Java大作业 —— 面向对象设计(购物车)
团队介绍
名字 |
负责任务 |
许慎 |
DAO接口及代码编写 |
吴绍杰 |
前期调查与功能设计、博客制作 |
郑伊杰 |
UML类图及编码规范 |
一、前期调查
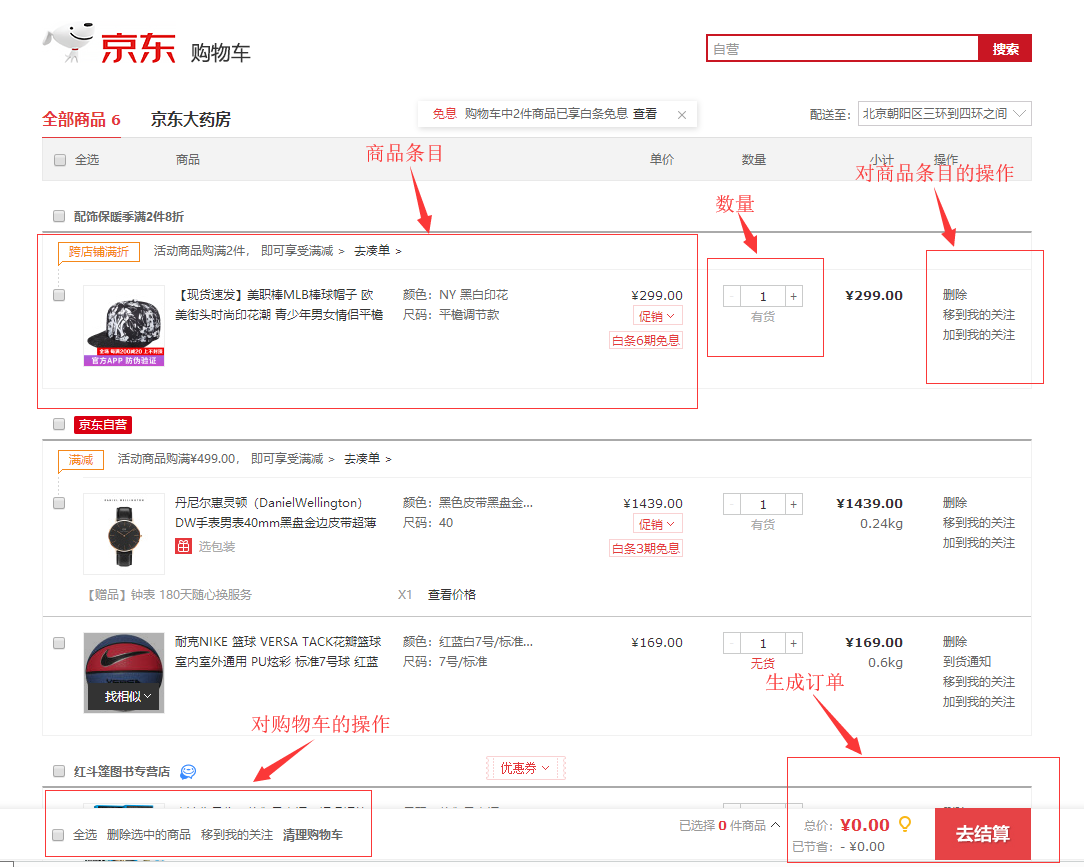
二、系统功能结构图
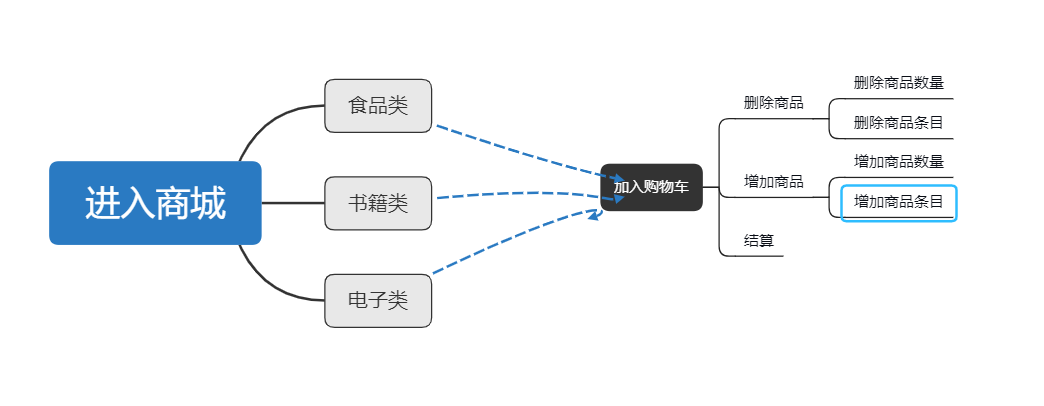
三、UML类图

四、运行截图
在购物车中添加商品:
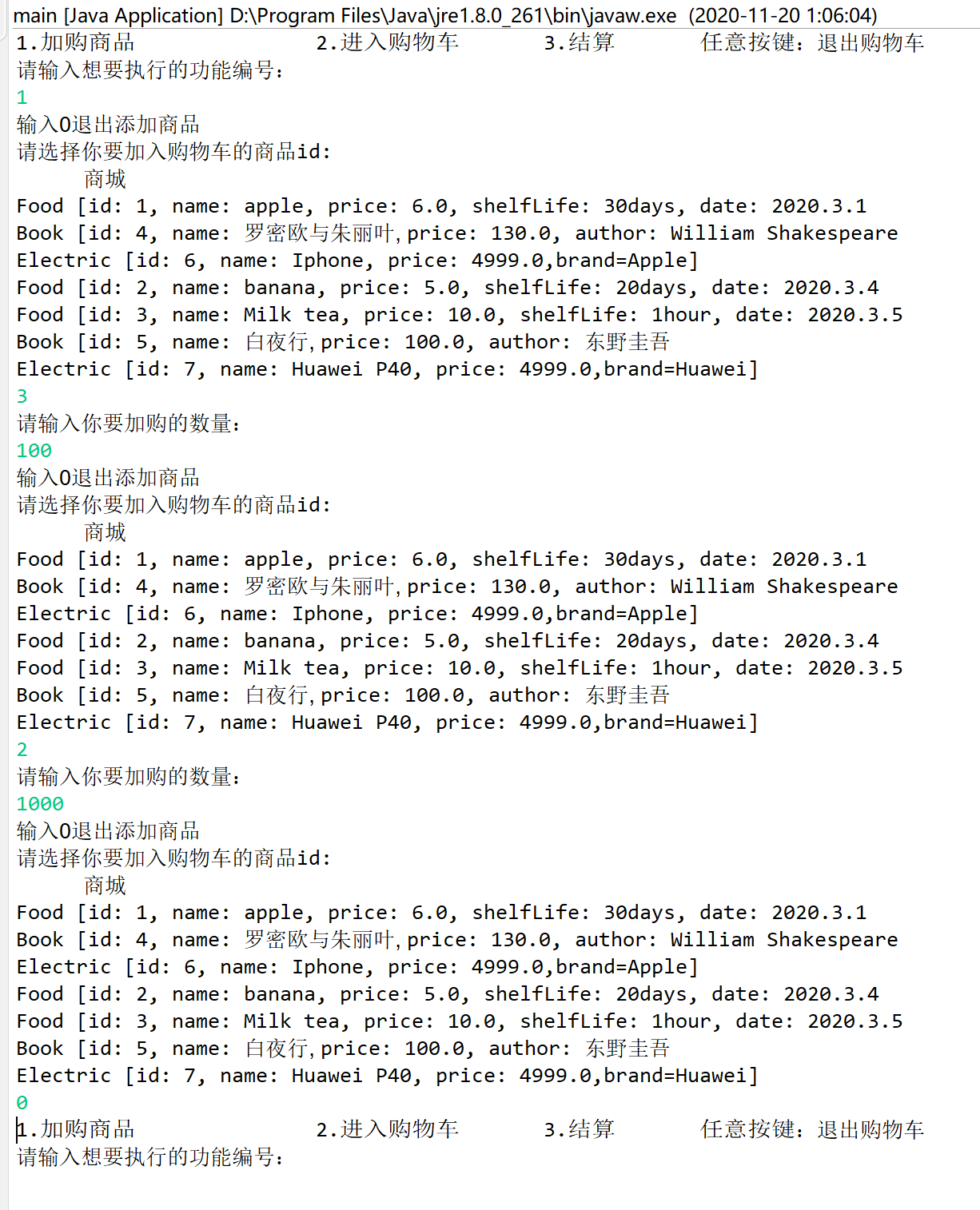
删除购物车中的商品:
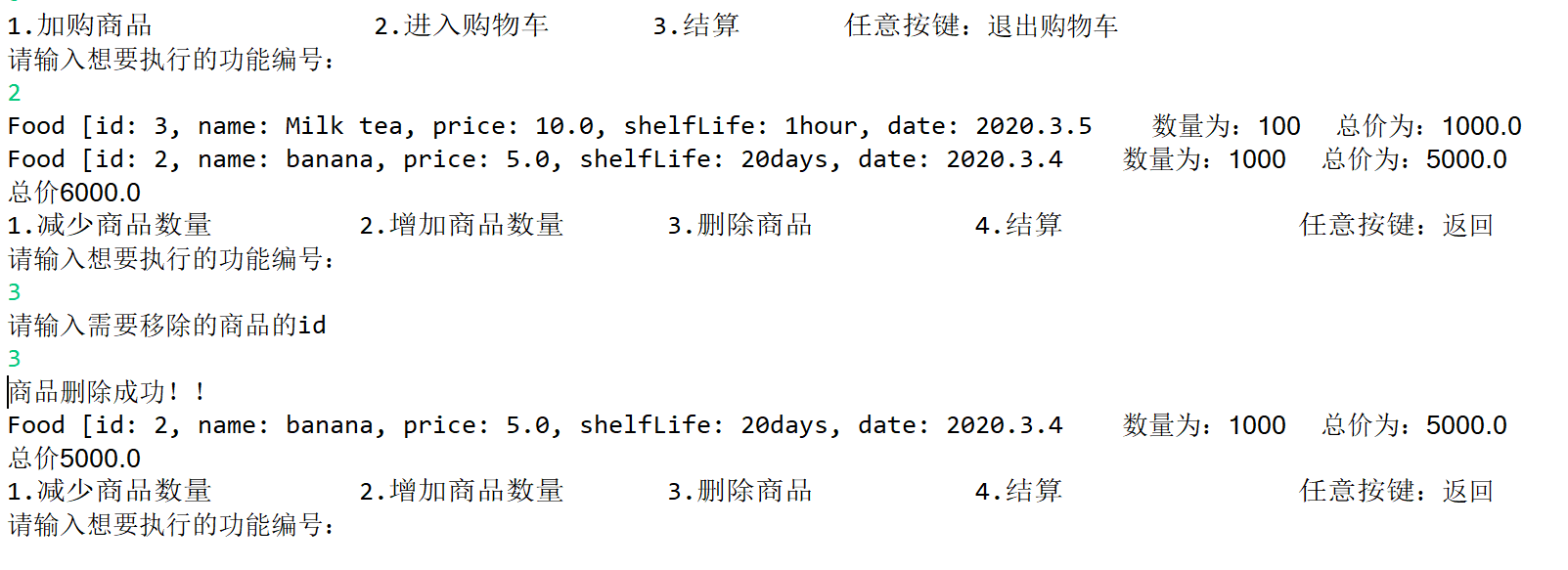
在购物车中增加和减少商品数量:
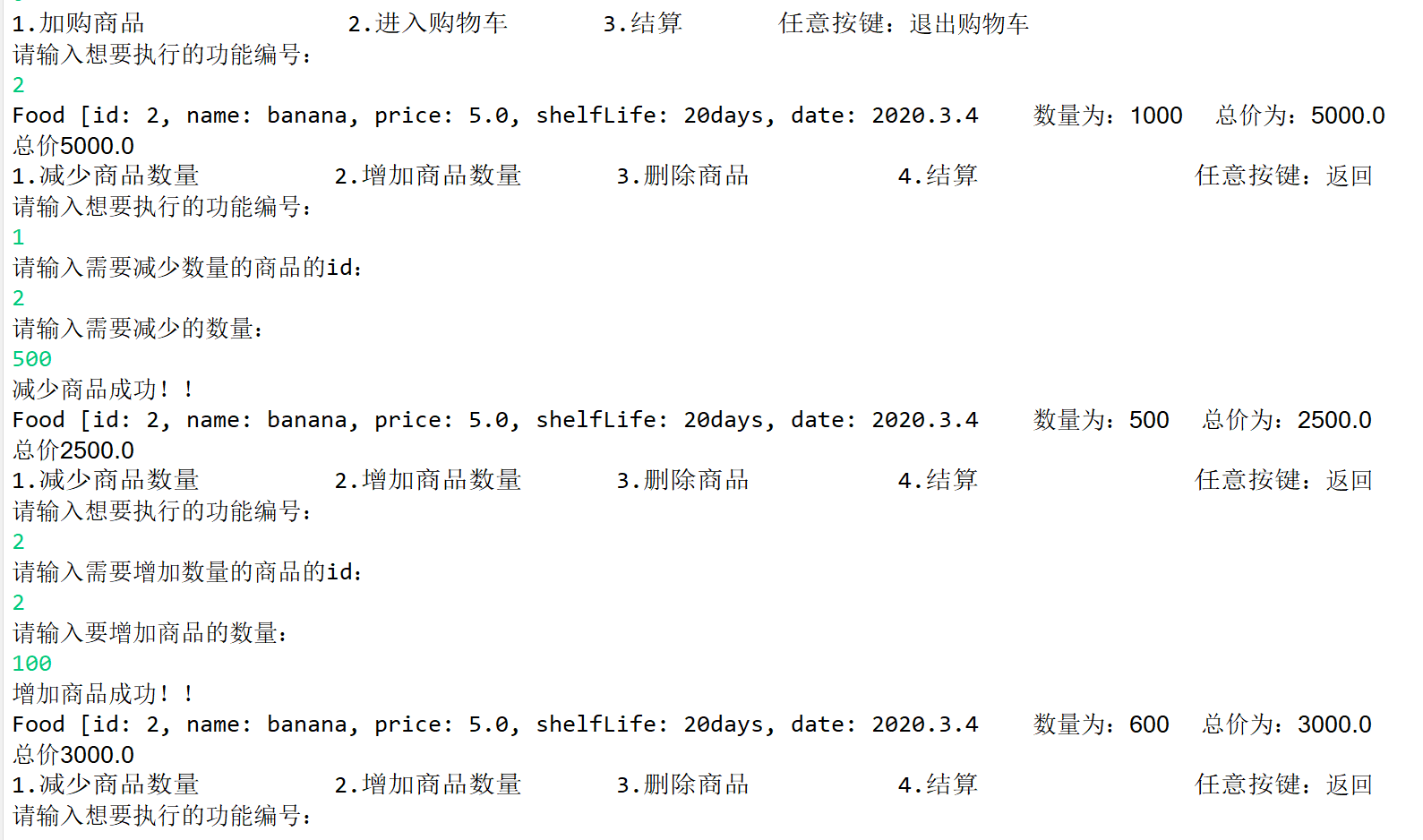
结算购物车:

五、DAO模式
public interface ShopDAO {
public boolean add(Commodity e,int count);//加入购物车
public boolean reduce(Integer id);//减少商品数量
public boolean increase(Integer id);//增加商品数量
public void diplayAll();//输出购物车内商品条目
public double checkout();//输出总金额
public boolean delete(Integer id);//删除条目
public int findById(Integer id);//查找ID
}
DAO模式,是将业务逻辑与数据的具体访问相分离的一种模式,它可以根据后台数据存储方式的不同,定义不同的实现方法。例如,编写GUI相关代码时,无需考虑数据操作的具体实现方法,只需要调用相应DAO方法。如果底层数据存储方式需要改变,只要增加DAO接口的新实现类即可,有利于不同人员的分工合作,提高效率
六、关键代码
---->Main
package shoppingcart;
import java.util.Scanner;
public class main {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
Commodity x1 = new Food("apple", 1, 6.0,"2020.3.1","30days");
Commodity x4 = new Food( "banana",2, 5.0, "2020.3.4", "20days");
Commodity x5 = new Food( "Milk tea",3, 10.0, "2020.3.5", "1hour");
Commodity x2 = new Book("罗密欧与朱丽叶",4, 130.0, "William Shakespeare");
Commodity x6 = new Book("白夜行",5, 100.0, "东野圭吾");
Commodity x3 = new Electric( "Iphone",6, 4999.0, "Apple");
Commodity x7 = new Electric( "Huawei P40",7, 4999.0, "Huawei");
ShopDAO cart = new ShopAarrayDAO();// 根据需求选择
//ShopDAO cart = new ShopListDAO();
Shelf shelf = new Shelf();
// 加入货架
shelf.addCom(x1);
shelf.addCom(x2);
shelf.addCom(x3);
shelf.addCom(x4);
shelf.addCom(x5);
shelf.addCom(x6);
shelf.addCom(x7);
int key = 1;
while (key==1) {
System.out.println("1.加购商品 2.进入购物车 3.结算 任意按键:退出购物车");
System.out.println("请输入想要执行的功能编号:");
int a = sc.nextInt();
switch(a) {
case 1 :{// 添加商品
while(true) {
System.out.println("输入0退出添加商品");
System.out.println(" 商城");
shelf.showCommodity();// 展示货架内的商品
System.out.println("请选择你要加入购物车的商品id:");
int input = sc.nextInt();
if(input==0)break;
if(shelf.getItemById(input)==null) {
System.out.println("所要添加的商品不存在!");
continue;
}
System.out.println("请输入你要加购的数量:");
int n = sc.nextInt();
cart.add(shelf.getItemById(input),n);
}
}
break;
case 2 : {// 购物车
int b=1;
while (b==1) {
Integer x;
cart.diplayAll();
System.out.println("总价"+cart.checkout());
System.out.println("1.减少商品数量 2.增加商品数量 3.删除商品 4.结算 任意按键:返回");
System.out.println("请输入想要执行的功能编号:");
int nextChoice = sc.nextInt();
switch (nextChoice) {
case 1:
System.out.println("请输入需要减少数量的商品的id:");
x = sc.nextInt();
if(cart.findById(x)==-1) {
System.out.println("所要减少的商品不存在!");
continue;
}
System.out.println("请输入你要减少的数量:");
cart.reduce(x);
System.out.println("减少商品成功!!");
break;
case 2:
System.out.println("请输入需要增加数量的商品的id:");
x = sc.nextInt();
if(cart.findById(x)==-1) {
System.out.println("所要增加的商品不存在!");
continue;
}
System.out.println("请输入你要增加的数量:");
cart.increase(x);
System.out.println("增加商品成功!!");
break;
case 3:
System.out.println("请输入需要移除的商品的id");
x = sc.nextInt();
if(cart.findById(x)==-1) {
System.out.println("所要删除的商品不存在!");
continue;
}
cart.delete(x);
System.out.println("商品删除成功!!");
break;
case 4:{
System.out.println("总金额为:" + cart.checkout());
b = 0;
key=0;
break;
}
default:
b=0;
break;
}
}
}
break;
case 3:{
System.out.println("总金额为:" + cart.checkout());
key = 0;
break;
}
default:
key = 0;
break;
}
}
}
}
---->购物车的各个功能实现
package shoppingcart;
import java.util.Scanner;
public class ShopAarrayDAO implements ShopDAO{
Scanner sc = new Scanner(System.in);
//private ArrayList<CommodityItem> itemList = new ArrayList<>();
private CommodityItem[] itemList = new CommodityItem[20];
private int size=0;
public double checkout() {//结算
double x = 0;
for (int i = 0; i < size; i++) {
x = x + itemList[i].total();// 价格*数量
}
return x;
}
public boolean add(Commodity e,int count) {//将商品加入购物车
int index = findById(e.getId());
if (index == -1) {//购物车没有该商品
itemList[size++] = new CommodityItem(e,count);
//itemList.add(new CommodityItem(e,count));
} else {
itemList[index].increase(count);
//itemList.get(index).increase();
}
return true;
}
public boolean reduce(Integer id) {//从购物车减少商品
int index = findById(id);
int a = sc.nextInt();
CommodityItem entry = itemList[index];
if (entry.getCount()-a <= 0) {// 商品数量为0,删除
delete(index);
size--;
} else {
entry.decrease(a);
}
return true;
}
private void delete(int i) {
for (int j = i; j < size; j++) {
itemList[j] = itemList[j+1];
}
}
public boolean increase(Integer id) {//增加商品数量
int index = findById(id);
int a = sc.nextInt();
CommodityItem entry = itemList[index];
entry.increase(a);
return true;
}
public boolean delete(Integer id) {//删除商品
int index = findById(id);
delete(index);
size--;
return true;
}
public void diplayAll() {//购物车展示
for (int i = 0; i < size; i++) {
System.out.println(itemList[i].getProduct().toString()+" 数量为:"+itemList[i].getCount()+" 总价为:"+itemList[i].total());
}
}
public int findById(Integer id) {//id查找
for (int i = 0; i < size; i++) {
if (itemList[i].getProduct().getId().equals(id))
return i;
}
return -1;
}
}
商品类
package shoppingcart;
public class Commodity {//商品类
private String name;//商品名
private Integer id;//商品编号
private double price;//商品单价
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public Integer getId() {
return id;
}
public void setId(int id) {
this.id = id;
}
public double getPrice() {
return price;
}
public void setPrice(double price) {
this.price = price;
}
public Commodity(String name, Integer id, double price) {
this.name = name;
this.id = id;
this.price = price;
}
@Override
public String toString() {
return "Commodity [name=" + name + ", id=" + id + ", price=" + price + "]";
}
}
class Book extends Commodity{
private String author;//作者姓名
public Book(String name, Integer id, double price, String author) {
super(name, id, price);
this.author = author;
}
public String getAuthor() {
return author;
}
public void setAuthor(String author) {
this.author = author;
}
@Override
public int hashCode() {
final int prime = 31;
int result = 1;
result = prime * result + ((author == null) ? 0 : author.hashCode());
return result;
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (getClass() != obj.getClass())
return false;
Book other = (Book) obj;
if (author == null) {
if (other.author != null)
return false;
} else if (!author.equals(other.author))
return false;
return true;
}
@Override
public String toString() {
return "Book [id: " + this.getId() + ", name: " + this.getName() + ", price: " + this.getPrice() +", author: " + author + "";
}
}
class Food extends Commodity{
private String date;//生产日期
private String shelfLife;//保质期
public Food(String name, Integer id, double price, String date, String shelfLife) {
super(name, id, price);
this.date = date;
this.shelfLife = shelfLife;
}
public String getDate() {
return date;
}
public void setDate(String date) {
this.date = date;
}
public String getShelfLife() {
return shelfLife;
}
public void setShelfLife(String shelfLife) {
this.shelfLife = shelfLife;
}
@Override
public int hashCode() {
final int prime = 31;
int result = 1;
result = prime * result + ((date == null) ? 0 : date.hashCode());
result = prime * result + ((shelfLife == null) ? 0 : shelfLife.hashCode());
return result;
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (getClass() != obj.getClass())
return false;
Food other = (Food) obj;
if (date == null) {
if (other.date != null)
return false;
} else if (!date.equals(other.date))
return false;
if (shelfLife == null) {
if (other.shelfLife != null)
return false;
} else if (!shelfLife.equals(other.shelfLife))
return false;
return true;
}
@Override
public String toString() {
return "Food [id: " + this.getId() + ", name: " + this.getName() + ", price: " + this.getPrice() + ", shelfLife: " + shelfLife + ", date: " + date+"";
}
}
class Electric extends Commodity{
private String brand;//电器品牌
public String getBrand() {
return brand;
}
public void setBrand(String brand) {
this.brand = brand;
}
public Electric(String name, Integer id, double price, String brand) {
super(name, id, price);
this.brand = brand;
}
@Override
public int hashCode() {
final int prime = 31;
int result = 1;
result = prime * result + ((brand == null) ? 0 : brand.hashCode());
return result;
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (getClass() != obj.getClass())
return false;
Electric other = (Electric) obj;
if (brand == null) {
if (other.brand != null)
return false;
} else if (!brand.equals(other.brand))
return false;
return true;
}
@Override
public String toString() {
return "Electric [id: " + this.getId() + ", name: " + this.getName() + ", price: " + this.getPrice() + ",brand=" + brand + "]";
}
}