//编译器为每个函数自动添加一个prototype属性
//prototype.constructor 指向本函数定义
//prototype.__proto__ 指向Function.prototype
function Point(x, y) {
this.x = x;
this.y = y;
}
function Plane(x, y) {
this.x = x;
this.y = y;
}
//函数是对象实例
//对象实例就是无序key, value数组
//所有函数共享同一原型对象实例
//使用new时的行为
//1.创建一个新的空对象实例{}, 意味着新的存储空间
//2.为这个新的对象实例,添加一个__proto__属性,指向被调用函数的prototype属性
//3.以新的对象实例作为执行上下文(this),执行__proto__.constructor
// 也就是Point.prototype.constructor, 即Point函数的定义
//4.返回新对象实例的引用
//对象实例没有prototype属性
var p1 = new Point();
var p2 = new Point();
console.log("====================================================")
console.log("同类不同实例,共享__proto__")
console.log("----------------------------------------------------")
if(Object.is(p1.__proto__, p2.__proto__) && Object.is(p1.__proto__, Point.prototype)) {
console.log("p1.__proto__ === p2.__proto__");
console.log("p1.__proto__ === Point.prototype");
}
console.log("----------------------------------------------------")
console.log("====================================================")
console.log("类实例没有prototype属性")
console.log("----------------------------------------------------")
if(Object.is(p1.prototype, p2.prototype) && Object.is(p1.prototype, undefined) ) {console.log("p1.prototype , p2.prototype are undefined");}
console.log("----------------------------------------------------")
//Every JavaScript function is actually a Function object.
//This can be seen with the code (function(){}).constructor === Function, which returns true.
if(Object.is(Point.constructor, Function)) {console.log("Point.constructor === Function");}
if(Object.is(Point.__proto__.constructor, Function)) {console.log("Point.__proto__.constructor === Function");}
if(!Object.is(Point.prototype.constructor, Point.__proto__.constructor)) {console.log("Point.__proto__.constructor !== Point.prototype.constructor");}
console.log("====================================================")
console.log("Point.prototype")
console.log("----------------------------------------------------")
if(Object.is(Point.prototype.constructor, Point)) {console.log("Point.prototype.constructor === Point");}
if(Object.is(Point.prototype.__proto__, Object.prototype)) {console.log("Point.prototype.__proto__ === Object.prototype");}
console.log("----------------------------------------------------")
console.log("====================================================")
console.log("函数定义对象的__proto__都指向Function.prototype")
console.log("----------------------------------------------------")
if(Object.is(Point.__proto__, Function.prototype)) {console.log("Point.__proto__ === Function.prototype");}
if(Object.is(Object.__proto__, Function.prototype)) {console.log("Object.__proto__ === Function.prototype");}
if(Object.is(Function.__proto__, Function.prototype)) {console.log("Function.__proto__ === Function.prototype");}
Point.__proto__.FooAdded = "NewPropByFoo"
console.log(Object.FooAdded)
console.log(Function.FooAdded)
console.log(Plane.__proto__.FooAdded)
function newFunction() {};
console.log(newFunction.__proto__.FooAdded)
console.log("----------------------------------------------------")
console.log("====================================================")
console.log("Object.prototype")
console.log("----------------------------------------------------")
if(Object.is(Object.prototype.constructor, Object)) {console.log("Object.prototype.constructor === Object");}
console.log(Object.prototype.__proto__)
console.log("----------------------------------------------------")
console.log("====================================================")
console.log("Function.prototype")
console.log("----------------------------------------------------")
if(Object.is(Function.prototype.__proto__, Object.prototype)) {console.log("Function.prototype.__proto__ === Object.prototype");}
if(Object.is(Function.prototype.constructor, Function)) {console.log("Function.prototype.constructor === Function");}
console.log("----------------------------------------------------")
====================================================
同类不同实例,共享__proto__
----------------------------------------------------
p1.__proto__ === p2.__proto__
p1.__proto__ === Point.prototype
----------------------------------------------------
====================================================
类实例没有prototype属性
----------------------------------------------------
p1.prototype , p2.prototype are undefined
----------------------------------------------------
Point.constructor === Function
Point.__proto__.constructor === Function
Point.__proto__.constructor !== Point.prototype.constructor
====================================================
Point.prototype
----------------------------------------------------
Point.prototype.constructor === Point
Point.prototype.__proto__ === Object.prototype
----------------------------------------------------
====================================================
函数定义对象的__proto__都指向Function.prototype
----------------------------------------------------
Point.__proto__ === Function.prototype
Object.__proto__ === Function.prototype
Function.__proto__ === Function.prototype
NewPropByFoo
NewPropByFoo
NewPropByFoo
NewPropByFoo
----------------------------------------------------
====================================================
Object.prototype
----------------------------------------------------
Object.prototype.constructor === Object
null
----------------------------------------------------
====================================================
Function.prototype
----------------------------------------------------
Function.prototype.__proto__ === Object.prototype
Function.prototype.constructor === Function
----------------------------------------------------
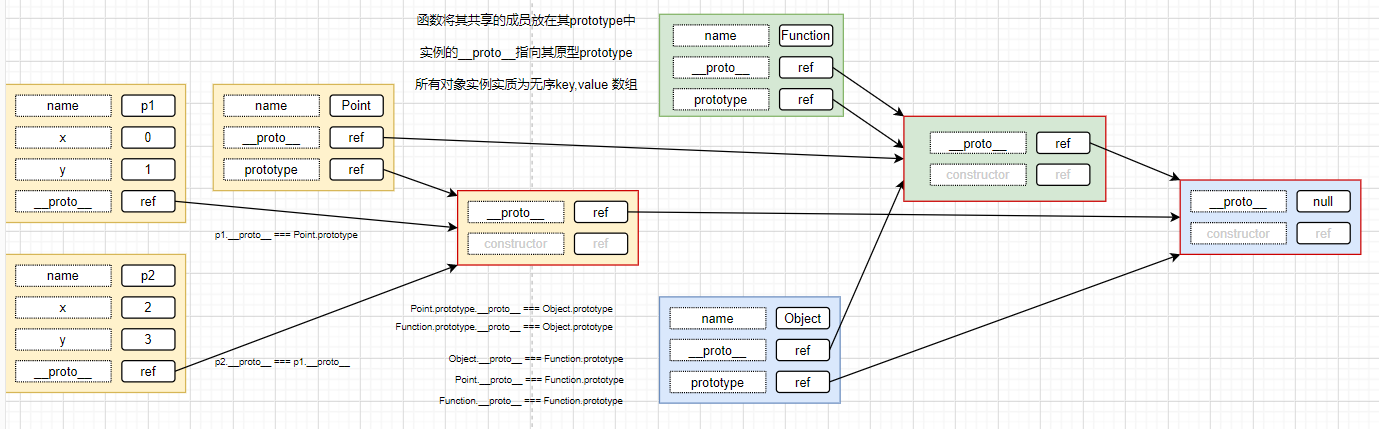