链表数据结构
链表存储有序的元素集合,但不同于数组,链表中的元素在内存中并不是连续防止的。每个元素由一个存储元素本身的节点和一个只想下一个元素的引用(也称为指针或链接)组成
类似的例子
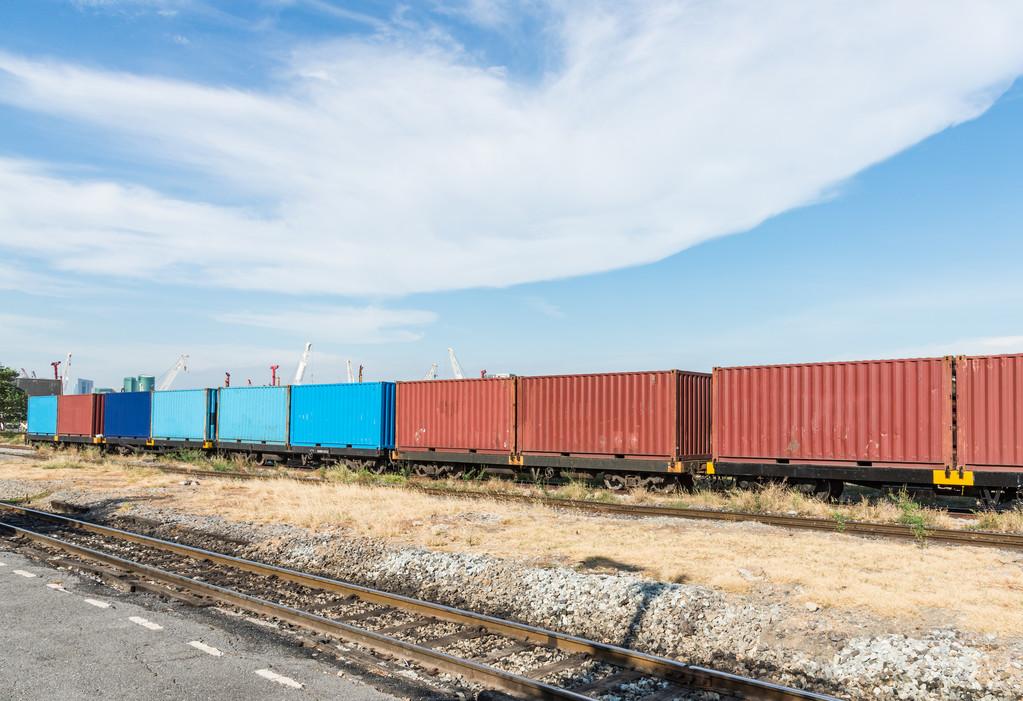
创建链表
- 创建一个类表示链表
- 链表内应该有一些方法
- 向链表尾部添加一个新元素
- 向链表特定位置添加一个新元素
- 返回链表中特定位置元素 不存在返回undefined
- 移除一个元素
- 返回元素在链表中的索引
- 从链表特定位置移除一个元素
- 如果链表中不包含任何元素,返回true,如果链表长度大于0返回false
- 返回链表包含的元素个数
- 返回整个链表的字符串
创建一个类列表链表
class Linkedlist {
constructor(equalsFn = defaultEquals) {
this.count = 0;
this.head = undefined;
this.equalsFn = equalsFn;
}
}
创建一个类表示链表元素
class Node{
constructor(el){
this.el = el
this.next = undefined
}
}
向链表尾部添加元素
push(el){
const node = new Node(el)
let current;
if(this.head == null){
this.head = node;
}else{
current = this.head;;
while(current.next != null){
current = current.next;
}
current.next = node;
}
this.count++;
}
从链表移中除元素
removeAt(index) {
if (index >= 0 && index < this.count) {
let current = this.head;
if (index === 0) {
this.head = this.next;
} else {
let previous;
for (let i = 0; i < index; i++) {
previous = current;
current = current.next;
}
previous.next = current.next
}
this.count--;
return current.element;
}
return undefined
}
逻辑复用方法 循环迭代链表直到目标位置
getElmentAt(index){
if(index >= 0 && index <= this.count){
let node = this.head;
for (let i = 0; i < index && node != null; i++) {
node = node.next;
}
return node;
}
return undefined;
}
在任意位置插入元素
insert(el,index){
if (index >= 0 && index <= this.count){
const node = new Node(el)
if(index === 0){
const current = this.head;
node.next = current;
this.head = node;
}else{
const previous = this.getElmentAt(index - 1)
const current = previous.next;
node.next = current;
previous.next = node;
}
this.count++;
return true;
}
return false;
}
返回一个元素的位置
indexOf(el){
let current = this.head;
for (let i = 0; i < this.count && current != null; i++) {
if(this.equalsFn(el,current.el)){
return i;
}
current = current.next;
}
return -1
}
从链表中移除元素
remove(el){
const index = this.indexOf(el);
return this.removeAt(index)
}
获取链表位置
size(){
return this.count;
}
判断是否为空
isEmpty(){
return this.size() === 0
}
获取链表
getHead(){
return this.head
}
返回整个链表的字符串
toString(){
if(this.head == null){
return '';
}
let objString = `${this.head.el}`;
let current = this.head.next;
for (let i = 0; i < this.size() && current != null; i++) {
objString = `${objString},${current.el}`;
current = current.next;
}
return objString;
}