最近我们团队完成了项目开发,要我做一个安装程序.以前从来没有进行过,哈哈^先花了半天学习,最后做了一个安装程序.但是出现在了一个问题,希望能得到高手的指教:
在我安装在最后的关头出现了这样的错误:未将对象设置引用到对象的实例!
安装程序又无法进行调试,找了好多次还是找不到哪里出现了问题.后面附上了安装类库的代码:
我的解决方案:
UI设计(1):
UI设计(2):
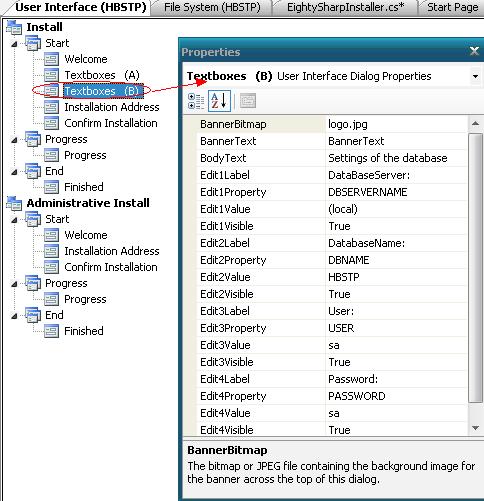
类库的代码有点长:
using System;
using System.IO;
using System.Data;
using System.Configuration.Install;
using System.DirectoryServices;
using System.Reflection;
using System.Data.SqlClient;
using System.Collections.Specialized;
using System.Xml;
using Microsoft.Win32;
using System.ComponentModel;
using System.Management;
using System.Collections;

namespace EightySharplibary


{
[RunInstaller(true)]
public partial class EightySharpInstaller : Installer

{
private SqlConnection sqlConn = null;
private SqlCommand Command;
private static string DBName;
private string AnotherDB;
private string ServerName;
private string AdminName;
private string AdminPwd;
private string iis;
private string port;
private string dir;
private string _target;
private DirectoryEntry _iisServer;
private ManagementScope _scope;
private ConnectionOptions _connection;

public EightySharpInstaller()

{
InitializeComponent();
}
//connect the db.

ConnectionDatabase#region ConnectionDatabase
private bool ConnectDatabase()

{
if (Command.Connection.State != ConnectionState.Open)

{
try

{
Command.Connection.Open();
}
catch

{
return false;
}
}
return true;
}
#endregion
//Get the db file

GetSQLFiles#region GetSQLFiles
private string GetSql(string name)

{
try

{
Assembly ass = Assembly.GetExecutingAssembly();
Stream strm = ass.GetManifestResourceStream(ass.GetName().Name + "." + name);
StreamReader reader = new StreamReader(strm);
return reader.ReadToEnd();
}
catch (Exception exp)

{
throw new ApplicationException(exp.Message);
}
}
#endregion
//ExecuteSQL statement

Execute sql statement.#region Execute sql statement.
private void ExecuteSql(string DataBaseName, string sqlstring)

{
Command = new SqlCommand(sqlstring, sqlConn);
if (ConnectDatabase())

{
try

{
Command.Connection.ChangeDatabase(DataBaseName);
Command.ExecuteNonQuery();
}
catch (Exception exp)

{
throw new Exception(exp.Message);
}
finally

{
Command.Connection.Close();
}
}
}
#endregion
//Create database and attach the files.

Create DB and attach the file.#region Create DB and attach the file.
protected bool CreateDBAndTable(string DBName)

{
AnotherDB = DBName + "Profile";
bool result = false;
try

{
ExecuteSql("master", "USE MASTER IF EXISTS (SELECT NAME FROM SYSDATABASES WHERE NAME='" + DBName + "') DROP DATABASE " + DBName);
ExecuteSql("master", "CREATE DATABASE " + DBName);
ExecuteSql(DBName, GetSql("HBSTPSQL.txt"));

ExecuteSql("master", "USE MASTER IF EXISTS (SELECT NAME FROM SYSDATABASES WHERE NAME='" + AnotherDB + "') DROP DATABASE " + AnotherDB);
ExecuteSql("master", "CREATE DATABASE " + AnotherDB);
ExecuteSql(AnotherDB, GetSql("ASPNETDBSQL.txt"));
result = true;
}
catch

{
File.Delete(dir + @"HBSTPSQl.txt");
File.Delete(dir + @"ASPNETDBSQL.txt");
result = false;
}
return result;
}
#endregion

RestoreDB Backup the data from the files .bak.#region RestoreDB Backup the data from the files .bak.
protected bool RestoreDB(string DBName)

{
AnotherDB = DBName + "Profile";
dir = this.Context.Parameters["targetdir"];
bool Restult = false;

string MSQL1 = "RESTORE DATABASE " + DBName +
" FROM DISK = '" + dir + @"HBSTPDB.bak' " +
" WITH MOVE 'HBSTPDB' TO '" + @"C:"Program Files"Microsoft SQL Server"MSSQL.1"MSSQL"Data"" + DBName + ".mdf', " +
" MOVE 'HBSTPDB_log' TO '" + @"C:"Program Files"Microsoft SQL Server"MSSQL.1"MSSQL"Data"" + DBName + ".ldf' ";
string MSQL2 = "RESTORE DATABASE " + AnotherDB +
" FROM DISK = '" + dir + @"ASPNETDB.bak' " +
" WITH MOVE 'ADPNETDB' TO '" + @"C:"Program Files"Microsoft SQL Server"MSSQL.1"MSSQL"Data"" + AnotherDB + ".mdf', " +
" MOVE 'aspnetdb_log' TO '" + @"C:"Program Files"Microsoft SQL Server"MSSQL.1"MSSQL"Data"" + AnotherDB + ".ldf' ";
try

{
ExecuteSql("master", "USE MASTER IF EXISTS (SELECT NAME FROM SYSDATABASES WHERE NAME='" + DBName + "') DROP DATABASE " + DBName);
ExecuteSql("master", MSQL1);
ExecuteSql("master", "USE MASTER IF EXISTS (SELECT NAME FROM SYSDATABASES WHERE NAME='" + AnotherDB + "') DROP DATABASE " + AnotherDB);
ExecuteSql("master", MSQL2);

Restult = true;
}
finally

{
// delete the backup files
try

{
File.Delete(dir + @"HBSTPDB.bak");
File.Delete(dir + @"ASPNETDB.bak");
}
catch

{
}
}
return Restult;
}
#endregion
//Modify the web.config

Modify teh web.config connectionstring.#region Modify teh web.config connectionstring.
private bool WriteWebConfig()

{
FileInfo fileInfo = new FileInfo(this.Context.Parameters["targetdir"] + "/web.config");
if (!fileInfo.Exists)

{
throw new InstallException("Missing config file:" + this.Context.Parameters["targetdir"] + "/web.config");
}
XmlDocument xmldoc = new XmlDocument();
xmldoc.Load(fileInfo.FullName);
bool connection1 = false;
bool connection2 = false;
bool foundIt = connection1 && connection2;
foreach(XmlNode node in xmldoc["configuration"]["connectionStrings"])

{
if (node.Name == "add")

{
if (node.Attributes.GetNamedItem("name").Value == "HBSTPConnection")

{
node.Attributes.GetNamedItem("connectionString").Value = String.Format("Persist Security Info=False; Data Source={0}; Initial Catalog={1}; User ID={2}; Password={3}; Packet Size=4096; Pooling=true; Max Pool Size=100; Min Pool Size=1", ServerName, DBName, AdminName, AdminPwd);
connection1 = true;
}
if (node.Attributes.GetNamedItem("name").Value == "ConnectionString")

{
node.Attributes.GetNamedItem("connectionString").Value = String.Format("Persist Security Info=False; Data Source={0};Initial Catalog={1}; User ID={2}; Password={3}; Packet Size=4096; Pooling=true; Max Pool Size=100; Min Pool Size=1", ServerName, AnotherDB, AdminName, AdminPwd);
connection2 = true;
}
}
}
if (!foundIt)

{
throw new InstallException("Error when writing the config file: web.config");
}
xmldoc.Save(fileInfo.FullName);
return foundIt;
}
#endregion
//Write the registrykey

Write RegistryKey.#region Write RegistryKey.
private void WriteRegistryKey()

{
//Write registrykey
RegistryKey rote = Registry.LocalMachine;
RegistryKey EightySharp = rote.OpenSubKey("SOFTWARE", true);
RegistryKey thirdSub = EightySharp.CreateSubKey("EightySharp");
thirdSub.SetValue("FilePath", "80Sharp");
}
#endregion
//Connect the iis server

Connect iis server#region Connect iis server
public bool Connect()

{
if (iis == null)

{
return false;
}
try

{
_iisServer = new DirectoryEntry("IIS://" + iis + "/W3SVC/1");
_target = iis;
_connection = new ConnectionOptions();
_scope = new ManagementScope(@"""" + iis + @""root"MicrosoftIISV2", _connection);
_scope.Connect();
}
catch

{
return false;
}
return IsConnected();
}
//
public bool IsConnected()

{
if (_target == null || _connection == null || _scope == null)

{
return false;
}
return _scope.IsConnected;
}
#endregion
//Is Web site Exists

Is web site exists#region Is web site exists
public bool IsWebSiteExists(string serverID)

{
bool result = false;
try

{
string siteName = "W3SVC/" + serverID;
ManagementObjectSearcher searcher = new ManagementObjectSearcher(_scope, new ObjectQuery("SELECT * FROM IIsWebServer"), null);
ManagementObjectCollection webSites = searcher.Get();
foreach (ManagementObject webSite in webSites)

{
if ((string)webSite.Properties["Name"].Value == siteName)

{
result = true ;
}
return false;
}
}
catch

{
result = false;
}
return result;
}
#endregion

//get next serverid

Get next openID#region Get next openID
private int GetNextOpenID()

{
DirectoryEntry iisComputer = new DirectoryEntry("IIS://localhost/w3svc");
int nextID = 0;
foreach (DirectoryEntry iisWebServer in iisComputer.Children)

{
string sname = iisWebServer.Name;
try

{
int name = int.Parse(sname);
if (name > nextID)

{
nextID = name;
}
}
catch

{
}
}
return ++nextID;
}
#endregion
//create web site

Create Web site#region Create Web site
public string CreateWebSite(string serverID, string servercomment, string defaultVrootPath, string HostName, string IP, string Port)

{
try

{
ManagementObject oW3SVC = new ManagementObject(_scope, new ManagementPath(@"IIsWebService='W3SVC'"), null);
if (IsWebSiteExists(serverID))

{
return "Site Already exists
";
}
ManagementBaseObject inputParameters = oW3SVC.GetMethodParameters("CreateNewSite");
ManagementBaseObject[] serverBinding = new ManagementBaseObject[1];
serverBinding[0] = CreateServerBinding(HostName, IP, Port);
inputParameters["ServerComment"] = servercomment;
inputParameters["ServerBindings"] = serverBinding;
inputParameters["PathOfRootVirtualDir"] = defaultVrootPath;
inputParameters["ServerId"] = serverID;
ManagementBaseObject outParameter = null;
outParameter = oW3SVC.InvokeMethod("CreateNewSite", inputParameters, null);

//start the web site.
string serverName = "W3SVC/" + serverID;
ManagementObject webSite = new ManagementObject(_scope, new ManagementPath(@"IIsWebServer='" + ServerName + "'"), null);
webSite.InvokeMethod("Start", null);
return (string)outParameter.Properties["ReturnValue"].Value;
}
catch (Exception exp)

{
return exp.Message;
}
}
public ManagementObject CreateServerBinding(string HostName, string IP, string Port)

{
try

{
ManagementClass classBinding = new ManagementClass(_scope, new ManagementPath("ServerBinding"), null);
ManagementObject serverBinding = classBinding.CreateInstance();
serverBinding.Properties["Hostname"].Value = HostName;
serverBinding.Properties["IP"].Value = IP;
serverBinding.Properties["Port"].Value = port;
serverBinding.Put();
return serverBinding;
}
catch

{
return null;
}
}
#endregion
//Install

Install#region Install
public override void Install(System.Collections.IDictionary stateSaver)

{
base.Install(stateSaver);

dir = this.Context.Parameters["targetdir"];
DBName = this.Context.Parameters["dbname"].ToString();
ServerName = this.Context.Parameters["server"].ToString();
AdminName = this.Context.Parameters["user"].ToString();
AdminPwd = this.Context.Parameters["pwd"].ToString();
iis = this.Context.Parameters["iis"].ToString();
port = this.Context.Parameters["port"].ToString();

this.sqlConn.ConnectionString = "Packet size=4096;User ID=" + AdminName + ";Password=" + AdminPwd + ";Data Source=" + ServerName + ";Persist Security Info=False;Integrated Security=false";
//this.sqlConn.ConnectionString = "Packet size=4096; Data Source=" + ";database=master; Persist Security Info=False; Integrated Security=SSPI";
//Execute the sql create database.
if (!CreateDBAndTable(DBName))

{
throw new ApplicationException("A serions error been occured when create database!.");
}
//restore the database.
if (!RestoreDB(DBName))

{
throw new ApplicationException("A serions error been occured when backup the data!");
}
// create web site
Connect();
string serverID = GetNextOpenID().ToString();
string serverComment = "HBSTP";
string defaultVrootPath = this.Context.Parameters["targetdir"];
if (defaultVrootPath.EndsWith(@"""))
{
defaultVrootPath = defaultVrootPath.Substring(0, defaultVrootPath.Length - 1);
}
string HostName = this.Context.Parameters["hostname"];
string IP = this.Context.Parameters["ip"];
string Port = port;
string sReturn = CreateWebSite(serverID, serverComment, defaultVrootPath, HostName, IP, Port);

// modify web.config
if (!WriteWebConfig())

{
throw new ApplicationException("setting the connection string falied");
}
// write the registrykey!
WriteRegistryKey();
}
#endregion

Uninstall did not complete#region Uninstall did not complete
public override void Uninstall(IDictionary savedState)

{
if (savedState == null)

{
throw new ApplicationException("Uninstall failed!");
}
else

{
base.Uninstall(savedState);
}
}
#endregion
}
}

希望高手指点迷津.
感谢
(徐智雄):http://www.cnblogs.com/xuzhixiong/archive/2006/06/27/437056.html
http://weblogs.asp.net/scottgu/archive/2005/11/06/429723.aspx
http://www.c-sharpcorner.com/UploadFile/vishnuprasad2005/SetupProjects12022005022406AM/SetupProjects.aspx
SetupProjects12022005022406AM/SetupProjects.aspx