C++面向对象入门(三十)继承构造函数和析构函数
C++继承中的构造和析构
C++派生类能继承大多数基类的成员, 但不包括构造函数(包括拷贝函数), 析构函数
C++派生类能继承大多数基类的成员, 但不包括构造函数(包括拷贝函数), 析构函数
C++继承中构造函数的调用顺序(单继承)
1 调用基类的构造函数, 初始化基类成员, 如果基类也是派生类, 则递归调用基类的基类的构造函数
2 调用自身的构造函数, 初始化成员
1 调用基类的构造函数, 初始化基类成员, 如果基类也是派生类, 则递归调用基类的基类的构造函数
2 调用自身的构造函数, 初始化成员
C++继承中的析构函数调用顺序
1 派生类自身析构函数
2 派生类子对象成员的析构函数(如果有子对象)
3 普通基类的析构函数
4 虚基类的析构函数
1 派生类自身析构函数
2 派生类子对象成员的析构函数(如果有子对象)
3 普通基类的析构函数
4 虚基类的析构函数
继承关系图:
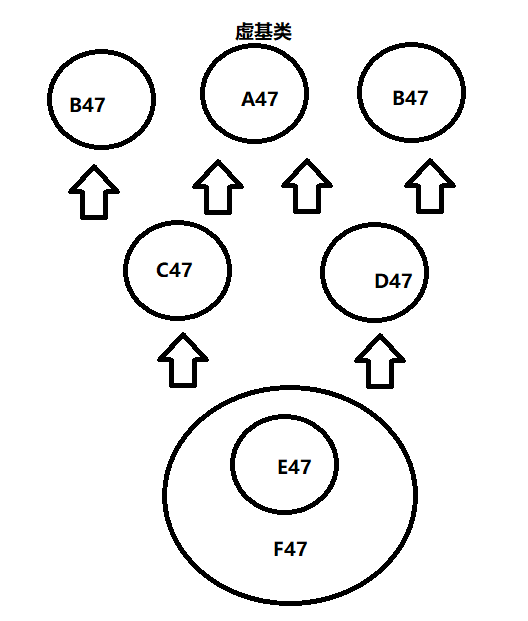
代码示例:
#include <iostream> #include "No47_singleInheritanceAndConstructorAndDestructor.h" using namespace std; /* C++继承中的构造和析构 C++派生类能继承大多数基类的成员, 但不包括构造函数(包括拷贝函数), 析构函数 C++继承中构造函数的调用顺序(单继承) 1 调用基类的构造函数, 初始化基类成员, 如果基类也是派生类, 则递归调用基类的基类的构造函数 2 调用自身的构造函数, 初始化成员 C++继承中的析构函数调用顺序 1 派生类自身析构函数 2 派生类子对象成员的析构函数(如果有子对象) 3 普通基类的析构函数 4 虚基类的析构函数 */ class A47 { public: int a; A47(); ~A47(); }; class B47 { public: int b; B47(); ~B47(); }; class C47 :public virtual A47, public B47 { public : int c; C47(); ~C47(); }; class D47 :public virtual A47, public B47 { public: int d; D47(); ~D47(); }; class E47 { public : E47(); ~E47(); int e; }; class F47 :public C47, public D47 { public: int f; E47 e47; F47(); ~F47(); void test(); }; void test() { F47 f; } int main() { test(); system("pause"); } A47::A47():a(1) { cout << "constructor of A47" << endl; } A47::~A47() { cout << "destructor of A47" << endl; } B47::B47():b(2) { cout << "constructor of B47" << endl; } B47::~B47() { cout << "destructor of B47" << endl; } C47::C47():A47(),B47(),c(3) { cout << "constructor of C47" << endl; } C47::~C47() { cout << "destructor of C47" << endl; } D47::D47():A47(),B47(),d(4) { cout << "constructor of D47" << endl; } D47::~D47() { cout << "destructor of D47" << endl; } E47::E47() :e(5) { cout << "constructor of E47" << endl; } E47::~E47() { cout << "destructor of E47" << endl; } F47::F47():C47(),D47(),e47(E47()),f(6) { cout << "constructor of F47" << endl; } F47::~F47() { cout << "destructor of F47" << endl; } void F47::test() { cout << "a = " << a << endl; cout << "C47::b = " << C47::b << endl; cout << "D47::b = " << D47::b << endl; cout << "c = " << c << endl; cout << "d = " << d << endl; cout << "e = " << e47.e << endl; cout << "f = " << f << endl; }
#include <iostream> #include "No47_singleInheritanceAndConstructorAndDestructor.h" using namespace std; /* C++继承中的构造和析构 C++派生类能继承大多数基类的成员, 但不包括构造函数(包括拷贝函数), 析构函数 C++继承中构造函数的调用顺序(单继承) 1 调用基类的构造函数, 初始化基类成员, 如果基类也是派生类, 则递归调用基类的基类的构造函数 2 调用自身的构造函数, 初始化成员 C++继承中的析构函数调用顺序 1 派生类自身析构函数 2 派生类子对象成员的析构函数(如果有子对象) 3 普通基类的析构函数 4 虚基类的析构函数 */ class A47 { public: int a; A47(); ~A47(); }; class B47 { public: int b; B47(); ~B47(); }; class C47 :public virtual A47, public B47 { public : int c; C47(); ~C47(); }; class D47 :public virtual A47, public B47 { public: int d; D47(); ~D47(); }; class E47 { public : E47(); ~E47(); int e; }; class F47 :public C47, public D47 { public: int f; E47 e47; F47(); ~F47(); void test(); }; void test() { F47 f; } int main() { test(); system("pause"); } A47::A47():a(1) { cout << "constructor of A47" << endl; } A47::~A47() { cout << "destructor of A47" << endl; } B47::B47():b(2) { cout << "constructor of B47" << endl; } B47::~B47() { cout << "destructor of B47" << endl; } C47::C47():A47(),B47(),c(3) { cout << "constructor of C47" << endl; } C47::~C47() { cout << "destructor of C47" << endl; } D47::D47():A47(),B47(),d(4) { cout << "constructor of D47" << endl; } D47::~D47() { cout << "destructor of D47" << endl; } E47::E47() :e(5) { cout << "constructor of E47" << endl; } E47::~E47() { cout << "destructor of E47" << endl; } F47::F47():C47(),D47(),e47(E47()),f(6) { cout << "constructor of F47" << endl; } F47::~F47() { cout << "destructor of F47" << endl; } void F47::test() { cout << "a = " << a << endl; cout << "C47::b = " << C47::b << endl; cout << "D47::b = " << D47::b << endl; cout << "c = " << c << endl; cout << "d = " << d << endl; cout << "e = " << e47.e << endl; cout << "f = " << f << endl; }
#include <iostream> #include "No47_singleInheritanceAndConstructorAndDestructor.h" using namespace std; /* C++继承中的构造和析构 C++派生类能继承大多数基类的成员, 但不包括构造函数(包括拷贝函数), 析构函数 C++继承中构造函数的调用顺序(单继承) 1 调用基类的构造函数, 初始化基类成员, 如果基类也是派生类, 则递归调用基类的基类的构造函数 2 调用自身的构造函数, 初始化成员 C++继承中的析构函数调用顺序 1 派生类自身析构函数 2 派生类子对象成员的析构函数(如果有子对象) 3 普通基类的析构函数 4 虚基类的析构函数 */ class A47 { public: int a; A47(); ~A47(); }; class B47 { public: int b; B47(); ~B47(); }; class C47 :public virtual A47, public B47 { public : int c; C47(); ~C47(); }; class D47 :public virtual A47, public B47 { public: int d; D47(); ~D47(); }; class E47 { public : E47(); ~E47(); int e; }; class F47 :public C47, public D47 { public: int f; E47 e47; F47(); ~F47(); void test(); }; void test() { F47 f; } int main() { test(); system("pause"); } A47::A47():a(1) { cout << "constructor of A47" << endl; } A47::~A47() { cout << "destructor of A47" << endl; } B47::B47():b(2) { cout << "constructor of B47" << endl; } B47::~B47() { cout << "destructor of B47" << endl; } C47::C47():A47(),B47(),c(3) { cout << "constructor of C47" << endl; } C47::~C47() { cout << "destructor of C47" << endl; } D47::D47():A47(),B47(),d(4) { cout << "constructor of D47" << endl; } D47::~D47() { cout << "destructor of D47" << endl; } E47::E47() :e(5) { cout << "constructor of E47" << endl; } E47::~E47() { cout << "destructor of E47" << endl; } F47::F47():C47(),D47(),e47(E47()),f(6) { cout << "constructor of F47" << endl; } F47::~F47() { cout << "destructor of F47" << endl; } void F47::test() { cout << "a = " << a << endl; cout << "C47::b = " << C47::b << endl; cout << "D47::b = " << D47::b << endl; cout << "c = " << c << endl; cout << "d = " << d << endl; cout << "e = " << e47.e << endl; cout << "f = " << f << endl; }
运行结果:
路漫漫其修远兮,吾将上下而求索。