LeetCode-28. Implement strStr() | 实现 strStr()
题目
Implement strStr().
Return the index of the first occurrence of needle in haystack, or -1 if needle is not part of haystack.
Clarification:
What should we return when needle is an empty string? This is a great question to ask during an interview.
For the purpose of this problem, we will return 0 when needle is an empty string. This is consistent to C's strstr() and Java's indexOf().
Example 1:
Input: haystack = "hello", needle = "ll"
Output: 2
Example 2:
Input: haystack = "aaaaa", needle = "bba"
Output: -1
Example 3:
Input: haystack = "", needle = ""
Output: 0
Constraints:
0 <= haystack.length, needle.length <= 5 * 104
haystack and needle consist of only lower-case English characters.
题解
难度简单。
这道题就是说要找到needle
在haystack
第一个出现的位置,如果没有出现就返回-1
。
解法一:暴力法
//Go
func strStr(haystack string, needle string) int {
//考虑特殊情况
if len(haystack) == 0 && len(needle) == 0 {
return 0
}
if len(haystack) == 0 {
return -1
}
if len(needle) == 0 {
return 0
}
if len(haystack) < len(needle) {
return -1
}
len_h := len(haystack) //获取haystack字符串的长度
len_n := len(needle) //获取needle字符串的长度
for i:=0;i<len_h-len_n+1;i++ {
j := 0; //子串每次都要重头开始遍历
for ;j<len_n;j++ {
if (haystack[i+j] != needle[j]) {
break;
}
}
if (j == len_n) {
return i;
}
}
return -1;
}
执行结果:
leetcode-cn:
执行用时:0 ms, 在所有 Go 提交中击败了100.00%的用户
内存消耗:2.2 MB, 在所有 Go 提交中击败了64.54%的用户
leetcode:
Runtime: 0 ms, faster than 100.00% of Go online submissions for Implement strStr().
Memory Usage: 2.3 MB, less than 100.00% of Go online submissions for Implement strStr().
参考资料
Golang
中的内置函数strings.Index也可以实现,可以参考它的源码实现。
//Go
import "strings"
func strStr(haystack string, needle string) int {
return strings.Index(haystack,needle)
}
版权声明:本文版权归作者和博客园共有,欢迎转载,但未经作者同意必须保留此段声明。
特此声明:所有评论和私信都会在第一时间回复。也欢迎园子里和园子外的大大们指正错误,共同进步。或者直接私信我 (^∀^)
声援博主:如果您觉得文章对您有帮助,可以点击文章右下角【推荐】一下。您的鼓励是作者坚持原创和持续写作的最大动力!
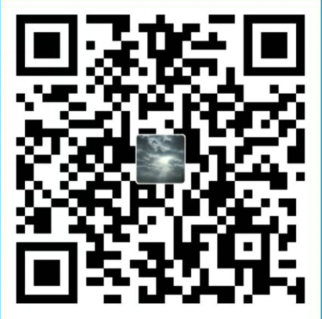
您的资助是我最大的动力!
金额随意,欢迎来赏!
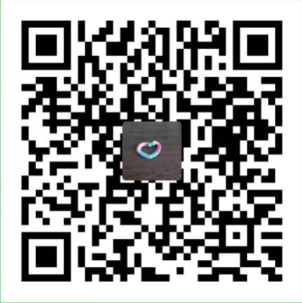
如果,您希望更容易地发现我的新博客,不妨点击一下绿色通道的
本博客的所有打赏均将用于博主女朋友的化妆品购买以及养肥计划O(∩_∩)O。我是【~不会飞的章鱼~】!