Eclipse快捷键
Eclipse中的快捷键:
* 1.补全代码的声明:alt + /
* 2.快速修复: ctrl + 1
* 3.批量导包:ctrl + shift + o
* 4.使用单行注释:ctrl + /
* 5.使用多行注释: ctrl + shift + /
* 6.取消多行注释:ctrl + shift + \
* 7.复制指定行的代码:ctrl + alt + down 或 ctrl + alt + up
* 8.删除指定行的代码:ctrl + d
* 9.上下移动代码:alt + up 或 alt + down
* 10.切换到下一行代码空位:shift + enter
* 11.切换到上一行代码空位:ctrl + shift + enter
* 12.如何查看源码:ctrl + 选中指定的结构 或 ctrl + shift + t
* 13.退回到前一个编辑的页面:alt + left
* 14.进入到下一个编辑的页面(针对于上面那条来说的):alt + right
* 15.光标选中指定的类,查看继承树结构:ctrl + t
* 16.复制代码: ctrl + c
* 17.撤销: ctrl + z
* 18.反撤销: ctrl + y
* 19.剪切:ctrl + x
* 20.粘贴:ctrl + v
* 21.保存: ctrl + s
* 22.全选:ctrl + a
* 23.格式化代码: ctrl + shift + f
* 24.选中数行,整体往后移动:tab
* 25.选中数行,整体往前移动:shift + tab
* 26.在当前类中,显示类结构,并支持搜索指定的方法、属性等:ctrl + o
* 27.批量修改指定的变量名、方法名、类名等:alt + shift + r
* 28.选中的结构的大小写的切换:变成大写: ctrl + shift + x
* 29.选中的结构的大小写的切换:变成小写:ctrl + shift + y
* 30.调出生成getter/setter/构造器等结构: alt + shift + s
* 31.显示当前选择资源(工程 or 文件)的属性:alt + enter
* 32.快速查找:参照选中的Word快速定位到下一个 :ctrl + k
*
* 33.关闭当前窗口:ctrl + w
* 34.关闭所有的窗口:ctrl + shift + w
* 35.查看指定的结构使用过的地方:ctrl + alt + g
* 36.查找与替换:ctrl + f
* 37.最大化当前的View:ctrl + m
* 38.直接定位到当前行的首位:home
* 39.直接定位到当前行的末位:end
项目二
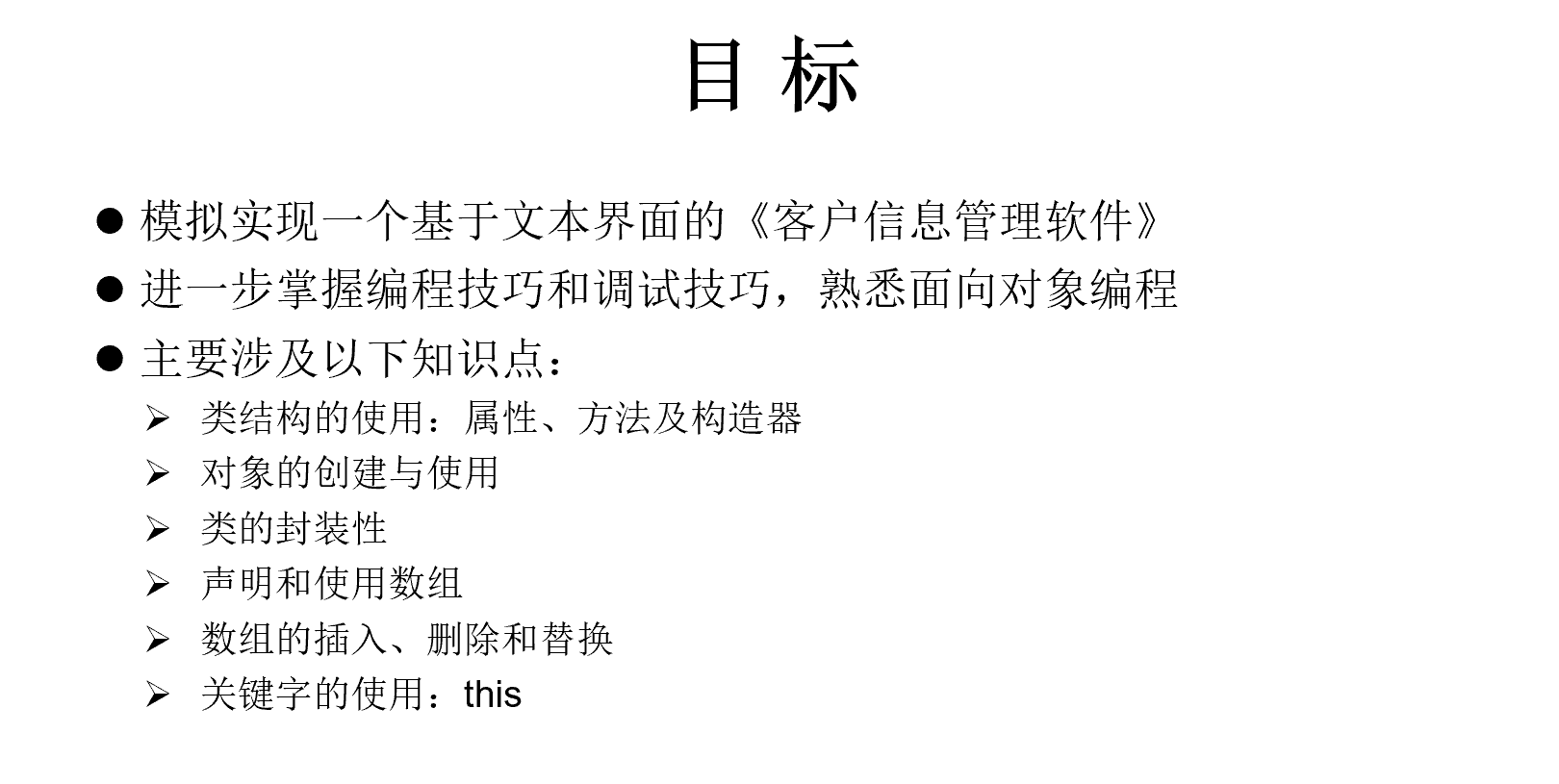
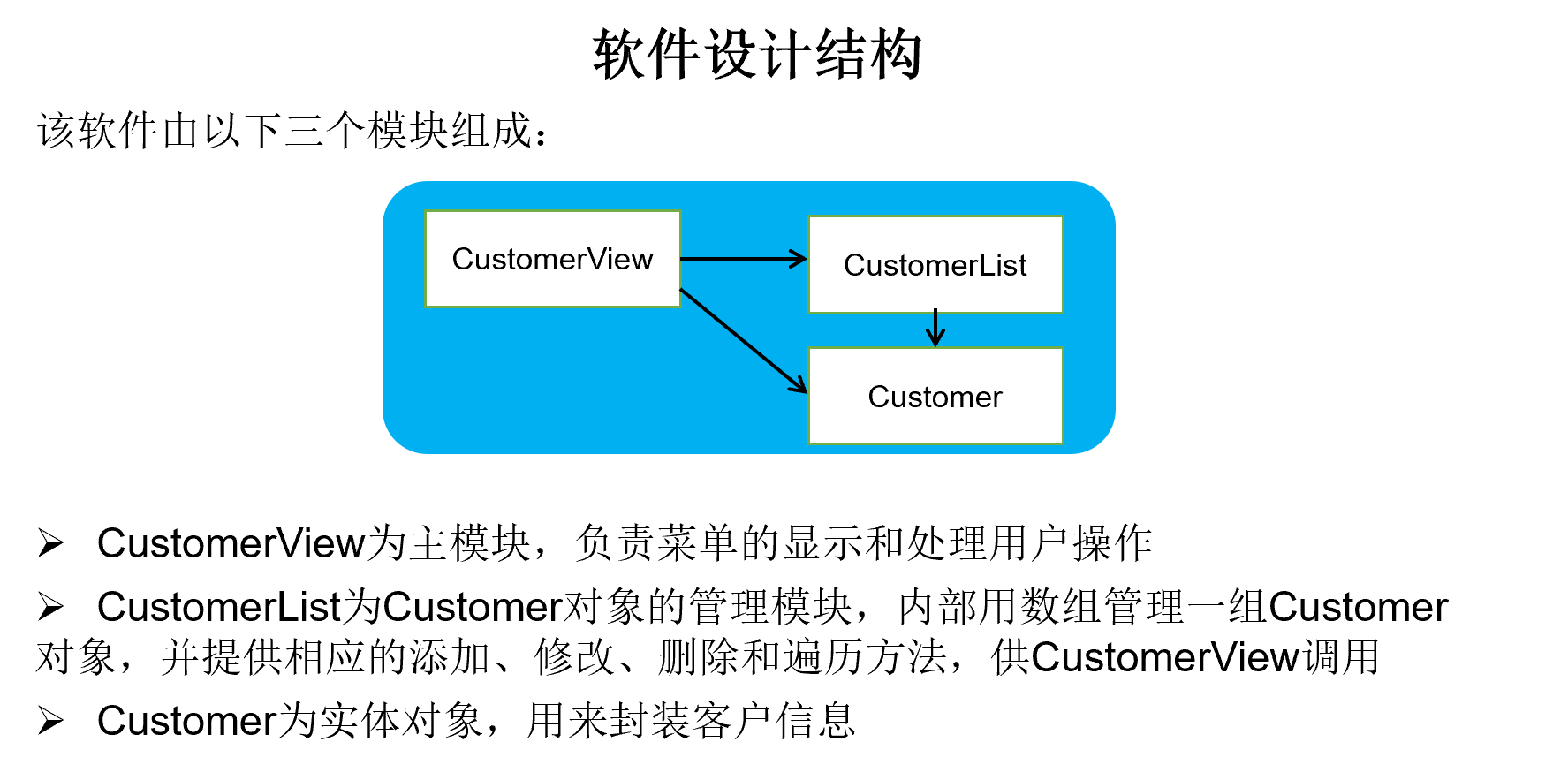
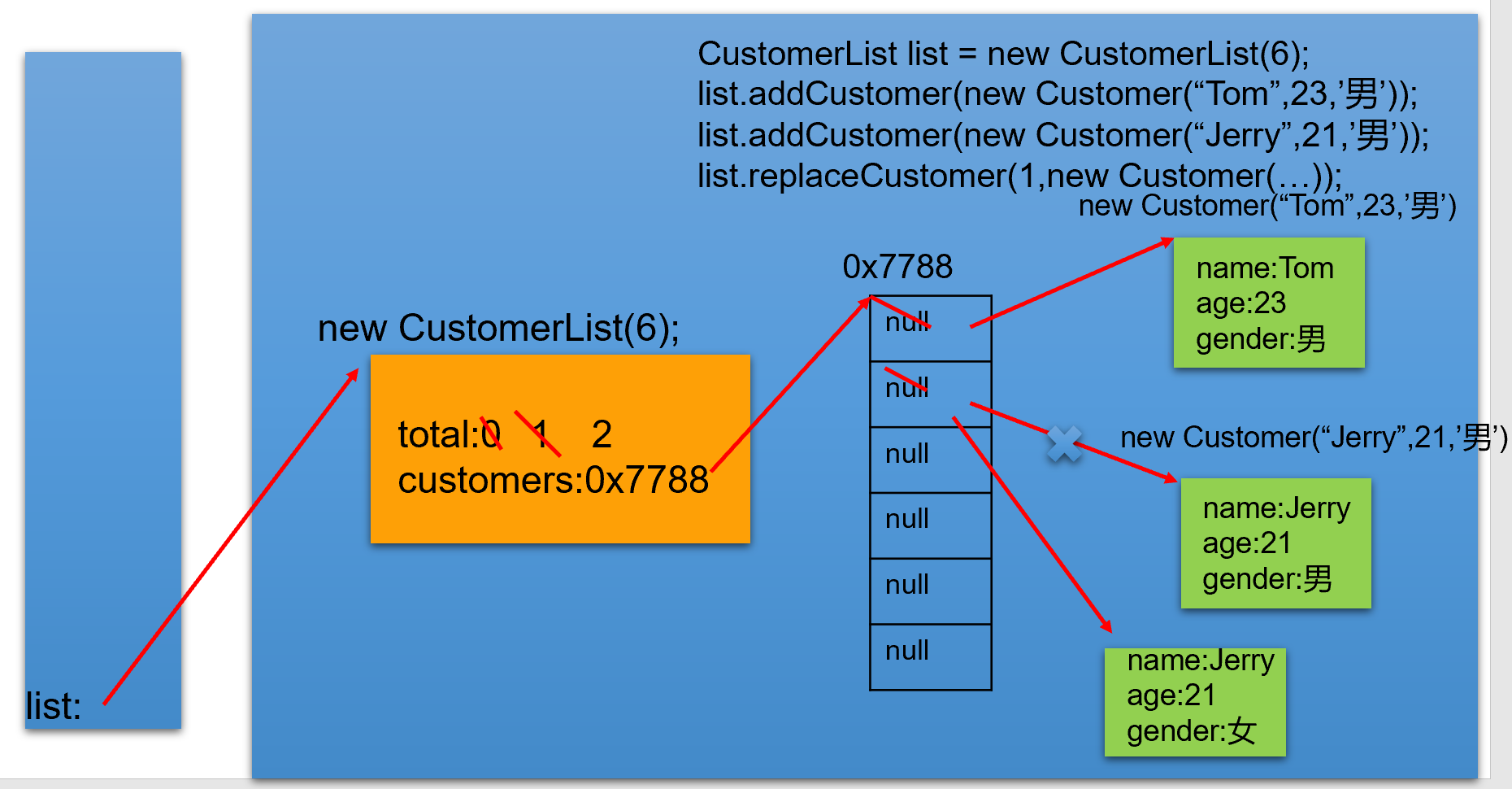
Customer
package com.atguigu.java.cust;
/**
*
* @Description 存储客户个人信息
* @author xiaojie Email:863828095@qq.com
* @version
* @date 2021年2月11日下午12:33:40
*
*/
public class Customer {
private String name;// 客户姓名
private char gender;// 性别
private int age;// 年龄
private String phone;// 电话号码
private String email;// 电子邮箱
public Customer() {
}
public Customer(String name, char gender, int age, String phone, String email) {
this.name = name;
this.gender = gender;
this.age = age;
this.phone = phone;
this.email = email;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public char getGender() {
return gender;
}
public void setGender(char gender) {
this.gender = gender;
}
public int getAge() {
return age;
}
public void setAge(int age) {
this.age = age;
}
public String getPhone() {
return phone;
}
public void setPhone(String phone) {
this.phone = phone;
}
public String getEmail() {
return email;
}
public void setEmail(String email) {
this.email = email;
}
}
CustomerList
package com.atguigu.java.custlist;
import com.atguigu.java.cust.Customer;
/**
*
* @Description Customer对象的管理模块
* @author xiaojie Email:863828095@qq.com
* @version
* @date 2021年2月11日下午12:41:42
*
*/
public class CustomerList {
private Customer[] customers;// 用来保存客户对象的数组
private int total = 0;// 记录已保存客户对象的数量
public CustomerList() {
}
/**
*
* @param totalCustomer
* 根据用户的输入,创建数组长度
*/
public CustomerList(int totalCustomer) {
customers = new Customer[totalCustomer];
}
/**
*
* @Description 将用户存入customers数组中
* @author xiaojie
* @date 2021年2月11日下午12:58:17
* @param customer
* Customer对象
* @return true:添加成功 false:数组已满无法添加
*/
public boolean addCustomer(Customer customer) {
if (total >= customers.length) {
return false;
}
customers[total++] = customer;
return true;
}
/**
*
* @Description 用参数customer替换数组中由index指定的对象
* @author xiaojie
* @date 2021年2月11日下午1:27:33
* @param index
* 指定所替换的对象在数组中的位置
* @param cust
* 指定替换的新对象
* @return return:替换成功 false:index指定的数值超出cutomers数组的长度
*/
public boolean replaceCustomer(int index, Customer cust) {
if (index <= 0 || index > total) {
return false;
}
customers[index - 1] = cust;
return true;
}
/**
*
* @Description 从数组中删除参数index指定索引位置的客户对象记录
* @author xiaojie
* @date 2021年2月11日下午1:44:55
* @param index
* 指定删除对象在数组中的索引位置
* @return true:删除成功 false:表示index指定的索引位置不在数组中
*/
public boolean deleteCustomer(int index) {
if (index <= 0 || index > total) {
return false;
}
for (int i = index - 1; i < customers.length - 1; i++) {
customers[i] = customers[i + 1];
}
customers[customers.length - 1] = null;
return true;
total--;
}
/**
*
* @Description 返回数组中记录的所有客户对象
* @author xiaojie
* @date 2021年2月11日下午1:51:53
* @return 返回存储客户对象的数组
*/
public Customer[] getAllCustomers() {
return customers;
}
/**
*
* @Description 返回参数index索引位置的客户对象记录
* @author xiaojie
* @date 2021年2月11日下午2:04:52
* @param index
* 指定所要获得的客户在数组中的索引位置
* @return 返回参数index指定索引位置的客户记录,null表示参数index指定的索引位置不在匹配的数组中
*/
public Customer getCustomer(int index) {
if (index <= 0 || index > total) {
return null;
}
return customers[index - 1];
}
/**
*
* @Description 返回当前客户数量
* @author xiaojie
* @date 2021年2月11日下午2:12:57
* @return 放回当前客户数量
*/
public int getTotal() {
return total;
}
}
CustomerView
package com.atguigu.java.custview;
import com.atguigu.java.cust.Customer;
import com.atguigu.java.custlist.CustomerList;
import com.atguigu.p2.CMUtility;
public class CustomerView {
CustomerList customerList = new CustomerList(10);
public CustomerView() { //功能测试
String name = "华东";
char gender = '男';
int age = 19;
String phone = "13012341234";
String email = "1234@qq.com";
customerList.addCustomer(new Customer(name, gender, age, phone, email));
customerList.addCustomer(new Customer(name, gender, age, phone, email));
customerList.addCustomer(new Customer(name, gender, age, phone, email));
customerList.addCustomer(new Customer(name, gender, age, phone, email));
customerList.addCustomer(new Customer(name, gender, age, phone, email));
customerList.addCustomer(new Customer(name, gender, age, phone, email));
customerList.addCustomer(new Customer(name, gender, age, phone, email));
customerList.addCustomer(new Customer(name, gender, age, phone, email));
customerList.addCustomer(new Customer(name, gender, age, phone, email));
customerList.addCustomer(new Customer(name, gender, age, phone, email));
}
/**
*
* @Description 显示主菜单,响应用户输入,根据用户操作分别调用其他相应的成员方法
* @author xiaojie
* @date 2021年2月11日下午5:28:47
*/
public void enterMainMenu() {
while (true) {
System.out.println("\n-----------------客户信息管理软件-----------------\n");
System.out.println(" 1 添加客户");
System.out.println(" 2 修改客户");
System.out.println(" 3 删除客户");
System.out.println(" 4 客户列表");
System.out.println(" 5 退 出" + "\n");
System.out.print(" 请选择(1-5): ");
char custSelected = CMUtility.readMenuSelection();
switch (custSelected) {
case '1':
addNewCustomer();
break;
case '2':
modifyCustomer();
break;
case '3':
deleteCustomer();
break;
case '4':
listAllCustomers();
break;
case '5':
System.out.print("确认是否退出(Y/N): ");
char confirmResult = CMUtility.readConfirmSelection();
if (confirmResult == 'Y') {
System.out.println("\nBye~~");
return;
}
}
}
}
/**
*
* @Description 填写用户信息,将用户对象存储到Customers数组中
* @author xiaojie
* @date 2021年2月11日下午8:33:14
*/
private void addNewCustomer() {
if(customerList.getAllCustomers().length == customerList.getTotal()){
System.out.println("当前人数已满");
return;
}
System.out.println("\n---------------------添加客户--------------------");
System.out.print("姓名: ");
String name = CMUtility.readString(10);
System.out.print("性别: ");
char gender = CMUtility.readChar();
System.out.print("年龄: ");
int age = CMUtility.readInt();
System.out.print("电话: ");
String phone = CMUtility.readString(11);
System.out.print("邮箱: ");
String email = CMUtility.readString(30);
boolean isFlag = customerList.addCustomer(new Customer(name, gender, age, phone, email));
if (isFlag) {
System.out.println("---------------------添加完成--------------------");
} else {
System.out.println("---------------------添加失败--------------------");
}
}
/**
*
* @Description 替换指定下标位置的对象的属性
* @author xiaojie
* @date 2021年2月11日下午9:49:32
*/
private void modifyCustomer() {
System.out.println("\n---------------------修改客户---------------------");
Customer temporary;
int isResult;
for (;;) {
System.out.print("请选择待修改客户编号(-1退出): ");
isResult = CMUtility.readInt();
if (isResult == -1) {
return;
}
if (customerList.getCustomer(isResult) == null) {
System.out.println("指定的索引不存在");
continue;
}
temporary = customerList.getCustomer(isResult);
break;
}
System.out.print("姓名(" + temporary.getName() + "): ");
String name = CMUtility.readString(10, temporary.getName());
System.out.print("性别(" + temporary.getGender() + "): ");
char gender = CMUtility.readChar(temporary.getGender());
System.out.print("年龄(" + temporary.getAge() + "): ");
int age = CMUtility.readInt(temporary.getAge());
System.out.print("电话(" + temporary.getPhone() + "): ");
String phone = CMUtility.readString(11, temporary.getPhone());
System.out.print("邮箱(" + temporary.getEmail() + "): ");
String email = CMUtility.readString(30, temporary.getEmail());
boolean isFlag = customerList.replaceCustomer(isResult, new Customer(name, gender, age, phone, email));
if (isFlag) {
System.out.println("---------------------修改完成---------------------");
} else {
System.out.println("---------------------修改失败---------------------");
}
}
/**
*
* @Description 删除指定下标位置的对象
* @author xiaojie
* @date 2021年2月11日下午10:19:59
*/
private void deleteCustomer() {
System.out.println("---------------------删除客户---------------------");
int isResult;
for (;;) {
System.out.print("请选择待删除客户编号(-1退出): ");
isResult = CMUtility.readInt();
if (isResult == -1) {
return;
}
System.out.print("确认是否删除(Y/N): ");
char confirmSelect = CMUtility.readConfirmSelection();
if (confirmSelect == 'N') {
return;
}
break;
}
boolean isFlag = customerList.deleteCustomer(isResult);
if (isFlag) {
System.out.println("---------------------删除完成---------------------");
} else {
System.out.println("---------------------删除失败---------------------");
}
}
/**
*
* @Description 打印数组中的用户对象信息
* @author xiaojie
* @date 2021年2月11日下午10:41:17
*/
private void listAllCustomers() {
System.out.println("---------------------------客户列表---------------------------");
System.out.println("编号\t姓名\t性别\t年龄\t电话\t\t邮箱");
for (int i = 1; i <= customerList.getTotal(); i++) {
if (customerList.getCustomer(i) == null) {
break;
}
System.out.println(i + "\t" + customerList.getCustomer(i).getName() + "\t"
+ customerList.getCustomer(i).getGender() + "\t" + customerList.getCustomer(i).getAge() + "\t"
+ customerList.getCustomer(i).getPhone() + "\t" + customerList.getCustomer(i).getEmail() + "\t");
}
System.out.println("-------------------------客户列表完成-------------------------");
}
public static void main(String[] args) {
CustomerView customerView = new CustomerView();
customerView.enterMainMenu();
}
}
CMUtility
package com.atguigu.p2;
import java.util.*;
/**
CMUtility工具类:
将不同的功能封装为方法,就是可以直接通过调用方法使用它的功能,而无需考虑具体的功能实现细节。
*/
public class CMUtility {
private static Scanner scanner = new Scanner(System.in);
/**
用于界面菜单的选择。该方法读取键盘,如果用户键入’1’-’5’中的任意字符,则方法返回。返回值为用户键入字符。
*/
public static char readMenuSelection() {
char c;
for (; ; ) {
String str = readKeyBoard(1, false);
c = str.charAt(0);
if (c != '1' && c != '2' &&
c != '3' && c != '4' && c != '5') {
System.out.print("选择错误,请重新输入:");
} else break;
}
return c;
}
/**
从键盘读取一个字符,并将其作为方法的返回值。
*/
public static char readChar() {
String str = readKeyBoard(1, false);
return str.charAt(0);
}
/**
从键盘读取一个字符,并将其作为方法的返回值。
如果用户不输入字符而直接回车,方法将以defaultValue 作为返回值。
*/
public static char readChar(char defaultValue) {
String str = readKeyBoard(1, true);
return (str.length() == 0) ? defaultValue : str.charAt(0);
}
/**
从键盘读取一个长度不超过2位的整数,并将其作为方法的返回值。
*/
public static int readInt() {
int n;
for (; ; ) {
String str = readKeyBoard(2, false);
try {
n = Integer.parseInt(str);
break;
} catch (NumberFormatException e) {
System.out.print("数字输入错误,请重新输入:");
}
}
return n;
}
/**
从键盘读取一个长度不超过2位的整数,并将其作为方法的返回值。
如果用户不输入字符而直接回车,方法将以defaultValue 作为返回值。
*/
public static int readInt(int defaultValue) {
int n;
for (; ; ) {
String str = readKeyBoard(2, true);
if (str.equals("")) {
return defaultValue;
}
try {
n = Integer.parseInt(str);
break;
} catch (NumberFormatException e) {
System.out.print("数字输入错误,请重新输入:");
}
}
return n;
}
/**
从键盘读取一个长度不超过limit的字符串,并将其作为方法的返回值。
*/
public static String readString(int limit) {
return readKeyBoard(limit, false);
}
/**
从键盘读取一个长度不超过limit的字符串,并将其作为方法的返回值。
如果用户不输入字符而直接回车,方法将以defaultValue 作为返回值。
*/
public static String readString(int limit, String defaultValue) {
String str = readKeyBoard(limit, true);
return str.equals("")? defaultValue : str;
}
/**
用于确认选择的输入。该方法从键盘读取‘Y’或’N’,并将其作为方法的返回值。
*/
public static char readConfirmSelection() {
char c;
for (; ; ) {
String str = readKeyBoard(1, false).toUpperCase();
c = str.charAt(0);
if (c == 'Y' || c == 'N') {
break;
} else {
System.out.print("选择错误,请重新输入:");
}
}
return c;
}
private static String readKeyBoard(int limit, boolean blankReturn) {
String line = "";
while (scanner.hasNextLine()) {
line = scanner.nextLine();
if (line.length() == 0) {
if (blankReturn) return line;
else continue;
}
if (line.length() < 1 || line.length() > limit) {
System.out.print("输入长度(不大于" + limit + ")错误,请重新输入:");
continue;
}
break;
}
return line;
}
}