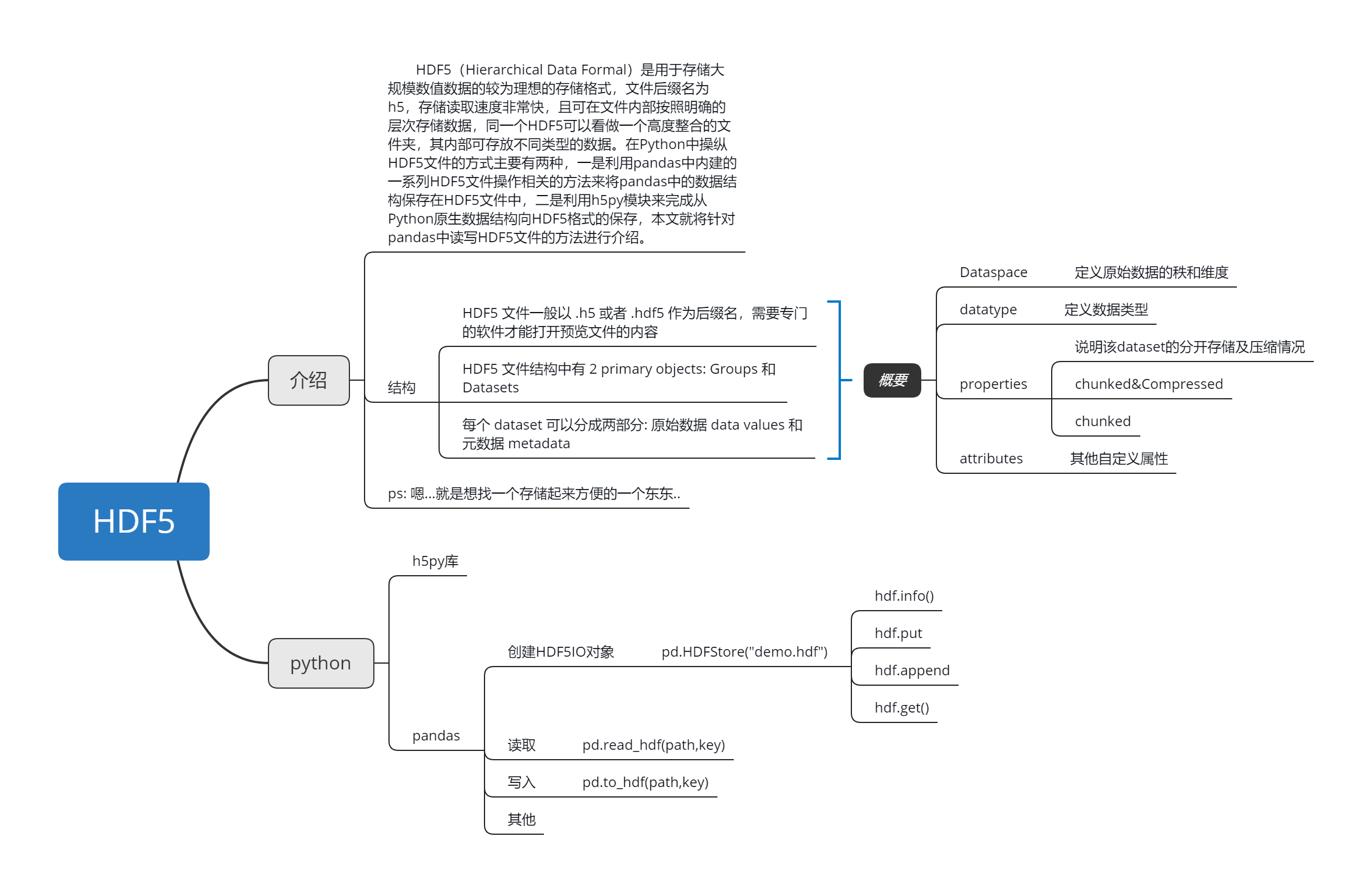
import numpy as np
import pandas as pd
import warnings
warnings.filterwarnings("ignore")
df1 = pd.DataFrame(np.random.standard_normal((30,2)), columns=['A','B'])
df2 = pd.DataFrame(np.random.standard_normal((30,2)), columns=['A','B'])
hdf=pd.HDFStore("Test.hdf","w")
# 写入
hdf.put(key="1",value=df1,format="table",data_columns=True)
hdf.put(key="2",value=df2,format="table",data_columns=True)
# 获取key
hdf.keys()
['/1', '/2']
hdf.append(key="1",value=df2)
# 输出信息
print(hdf.info())
<class 'pandas.io.pytables.HDFStore'>
File path: Test.hdf
/1 frame_table (typ->appendable,nrows->30,ncols->2,indexers->[index],dc->[A,B])
/2 frame_table (typ->appendable,nrows->30,ncols->2,indexers->[index],dc->[A,B])
# 根据key获取数据
t=hdf.get("1")
t[:4]
|
A |
B |
0 |
1.099650 |
0.799614 |
1 |
-0.806459 |
-0.773334 |
2 |
-0.238522 |
1.886817 |
3 |
1.200624 |
-0.082039 |
hdf.close()
df1.to_hdf("test2.hdf",key="2")
x=pd.read_hdf("Test.hdf",key="2")
new_hdf=pd.HDFStore("test2.hdf")
# 追加操作并不是很好..
# 存储的时候,要把数据整好了..