-
文件夹目录
-
连接数据库
const mongoose = require("mongoose"); mongoose.connect("mongodb://localhost:27017/school",{ useNewUrlParser: true, useUnifiedTopology: true }); mongoose.connection.once("open",err=>{ if(err) throw err; console.log("database connction success!"); })
-
数据表映射对象
const mongoose = require("mongoose"); const studentSchema = new mongoose.Schema({ username:{ type:String, unique:true, require:true }, password:{ type:String, require:true }, sex:String, age:Number, hobby:[String], birthday:{ type:Date, default:Date.now } }); //创建Model映射对象 const studentSch = mongoose.model("students",studentSchema); module.exports = studentSch;
-
操作数据库
/** * 简单实现登录注册 * 相关技术点: * 1.get/post请求 * 注册 post请求 * 登录 get请求 * 2.请求响应过程 * reg.html --> reg * login.html --> login * 1.注册成功则跳转登录 * 2.注册失败则继续注册 * 3.登录成功跳转主页 * 4.登录失败则继续登录 * 3.登录与注册的过程 * 实现账号密码的非空验证 * 使用正则校验账号密码的格式 */ const express = require("_express@4.17.1@express"); const app = express(); app.listen(8080); //连接数据库 require("./dbutil/db"); //获取数据库映射对象 const studentModel = require("./model/studentModel"); const path = require("path"); /** * toRegister */ app.get("/toRegister",(req,res)=>{ const filePath = path.resolve(__dirname,"./static/register.html"); console.log("跳转到注册的路径为:",filePath); res.sendFile(filePath); }) /** * doRegister */ app.use(express.urlencoded({ extended:true })) app.post("/doRegister",async (req,res)=>{ // 1.获取账号密码 const {username,password,sex,age,hobby,birthday} = req.body; console.log(username,password,sex,age,hobby,birthday); // 2.非空校验 if(!username || !password || !sex || !age || !hobby || !birthday){ res.send("注册信息不能为空!"); return; } // 3.正则判断 const reg = /^[0-1a-zA-Z_]{6,16}$/; if(!reg.test(username)){ res.send("账号校验不通过!") return; } // 4.验证账号是否存在 const checkUsername = await studentModel.findOne(req.body); if(checkUsername){ res.send("当前账号已存在!") return; } // 5.进行注册处理 const result = await studentModel.create(req.body); // console.log(result); if(result){ const filePath = path.resolve(__dirname,"./static/login.html"); console.log("跳转路径:",filePath); res.sendFile(filePath); console.log("注册成功!"); }else{ console.log("注册失败!"); } }) /** * toLogin */ app.get("/toLogin",(req,res)=>{ const filePath = path.resolve(__dirname,"./static/login.html"); console.log("跳转到登录的路径为:",filePath); res.sendFile(filePath); }) /** * doLogin */ app.get("/doLogin",async (req,res)=>{ //1.获取账号密码 console.log(req.query); const {username,password} = req.query; //2.非空校验 if(!username || !password){ res.send("账号密码不能为空!"); } //3.正则判断 let reg = /^[0-9A-Za-z_]{6,16}$/; if(!reg.test(username) || !reg.test(password)){ res.send("账号密码格式不正确!") } //4.验证账号是否存在 const isEmpty = await studentModel.findOne(req.query); console.log(isEmpty); if(!isEmpty){ res.send("当前账号不存在!"); } //5.进行登录处理 if(isEmpty){ const filePath = path.resolve(__dirname,"./static/index.html"); console.log("跳转路径:",filePath); res.sendFile(filePath); } });
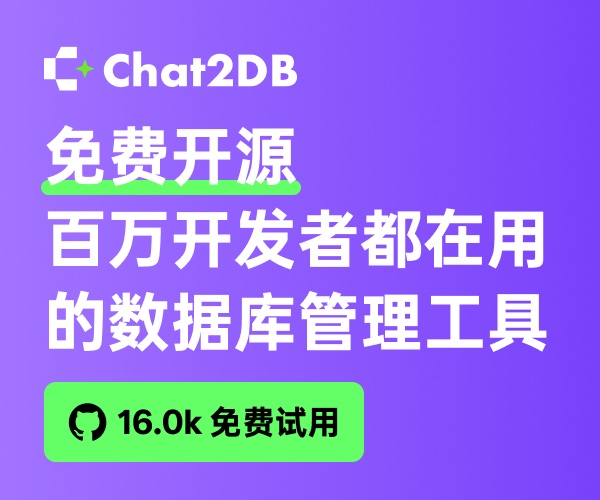
