Unity 文件压缩
public class ZIP { public void GetPaths(string rootPath, string[] whitelist, out List<string> dirs, out List<string> files) { rootPath = rootPath.Contains("/") ? rootPath.Replace("/", "\\") : rootPath; rootPath = rootPath.EndsWith("\\") ? rootPath : rootPath + "\\"; dirs = new List<string>(); files = new List<string>(); foreach (var item in whitelist) { string path = Path.Combine(rootPath, item); if (Directory.Exists(path)) { string dirName = item.EndsWith("\\") ? item : item + "\\"; if (!dirs.Contains(dirName)) { dirs.Add(dirName); } GetPaths(rootPath, path, dirs, files); } else if (File.Exists(path)) { if (!files.Contains(item)) { files.Add(item); } } } } public void GetPaths(string rootPath, string dirPath, List<string> dirs, List<string> files) { rootPath = rootPath.Contains("/") ? rootPath.Replace("/", "\\") : rootPath; rootPath = rootPath.EndsWith("\\") ? rootPath : rootPath + "\\"; foreach (var file in Directory.GetFiles(dirPath)) { string filePath = file.Contains("/") ? file.Replace("/", "\\") : file; string fileName = filePath.Replace(rootPath, ""); if (!files.Contains(fileName)) { files.Add(fileName); } } foreach (var subDir in Directory.GetDirectories(dirPath)) { string subDirPath = subDir.Contains("/") ? subDir.Replace("/", "\\") : subDir; string subDirName = subDirPath.Replace(rootPath, ""); subDirName = subDirName.EndsWith("\\") ? subDirName : subDirName + "\\"; if (!dirs.Contains(subDirName)) { dirs.Add(subDirName); } GetPaths(rootPath, subDir, dirs, files); } } public bool Zip(string rootPath, string[] _fileOrDirectoryArray, string _outputPathName, string _password = null, int level = 6) { if ((null == _fileOrDirectoryArray) || string.IsNullOrEmpty(_outputPathName)) { return false; } ZipOutputStream zipOutputStream = new ZipOutputStream(File.Create(_outputPathName)); zipOutputStream.SetLevel(level); // 压缩质量和压缩速度的平衡点 if (!string.IsNullOrEmpty(_password)) zipOutputStream.Password = _password; GetPaths(rootPath, _fileOrDirectoryArray, out List<string> dirs, out List<string> files); foreach (var dirName in dirs) { ZipEntry entry = new ZipEntry(dirName); entry.DateTime = DateTime.Now; entry.Size = 0; zipOutputStream.PutNextEntry(entry); zipOutputStream.Flush(); } foreach (var fileName in files) { ZipEntry entry = new ZipEntry(fileName); entry.DateTime = DateTime.Now; byte[] buffer = File.ReadAllBytes(Path.Combine(rootPath, fileName)); entry.Size = buffer.Length; zipOutputStream.PutNextEntry(entry); zipOutputStream.Write(buffer, 0, buffer.Length); } zipOutputStream.Finish(); zipOutputStream.Close(); return true; } public void Unzip(byte[] zipData,string unzipDirPath) { ZipEntry zip = null; Stream stream = new MemoryStream(zipData); ZipInputStream zipInStream = new ZipInputStream(stream); //循环读取Zip目录下的所有文件 while ((zip = zipInStream.GetNextEntry()) != null) { string path = Path.Combine(unzipDirPath, zip.Name); if (zip.IsDirectory) { if (!Directory.Exists(path)) { Directory.CreateDirectory(path); } } else if(zip.IsFile) { if (File.Exists(path)) { File.Delete(path); } byte[] data = new byte[(int)zipInStream.Length]; zipInStream.Read(data, 0, data.Length); File.WriteAllBytes(path, data); } } if (zipInStream != null) { zipInStream.Close(); } if (stream!=null) { stream.Close(); } } }
用到的库是:
using ICSharpCode.SharpZipLib.Zip;//文档:https://icsharpcode.github.io/SharpZipLib/help/api/ICSharpCode.SharpZipLib.Zip.ZipFile.html
博客园Jason_c微信打赏码
如果本篇文档对你有帮助,打赏Jason_c根华子吧,他的私房钱被老婆没收了,呜呜!
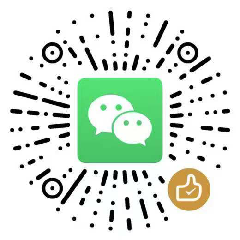