打开文件夹
using System; using System.Collections; using System.Collections.Generic; using System.Runtime.InteropServices; using UnityEngine; //unity3d调用win32打开对话框 [StructLayout(LayoutKind.Sequential, CharSet = CharSet.Auto)] public struct OpenFileName { public int structSize; public IntPtr dlgOwner; public IntPtr instance; public string filter; public string customFilter; public int maxCustFilter; public int filterIndex; public string file; public int maxFile; public string fileTitle; public int maxFileTitle; public string initialDir; //打开路径 null public string title; public int flags; public short fileOffset; public short fileExtension; public string defExt; public IntPtr custData; public IntPtr hook; public string templateName; public IntPtr reservedPtr; public int reservedInt; public int flagsEx; } public class OpenPDF:MonoBehaviour { [DllImport("Comdlg32.dll", SetLastError = true, ThrowOnUnmappableChar = true, CharSet = CharSet.Auto)] private static extern bool GetOpenFileName([In, Out] OpenFileName ofn); OpenFileName ofn; void Start() { ofn = new OpenFileName(); ofn.structSize = Marshal.SizeOf(ofn); ofn.filter = "PDF文件(*.pdf)\0*.pdf;"; ofn.file = new string(new char[256]); ofn.maxFile = ofn.file.Length; ofn.fileTitle = new string(new char[64]); ofn.maxFileTitle = ofn.fileTitle.Length; ofn.initialDir = "C:\\"; ofn.title = "打开课件"; ofn.defExt = "PDF"; ofn.flags = 0x00080000 | 0x00001000 | 0x00000800 | 0x00000200 | 0x00000008; //OFN_EXPLORER|OFN_FILEMUSTEXIST|OFN_PATHMUSTEXIST| OFN_ALLOWMULTISELECT|OFN_NOCHANGEDIR } public void OpenFile() { StartCoroutine(SelectFile()); } IEnumerator SelectFile() { yield return 1; List<string> fliePath = GetFilePath(); for (int i = 0; i < fliePath.Count; i++) { Debug.Log(fliePath[i]); } } /// <summary> /// 支持同时选择多个文件 /// </summary> /// <returns></returns> public List<string> GetFilePath() { List<string> fliePath = new List<string>(); if (GetOpenFileName(ofn)) { string[] filePath = ofn.file.Split('\0');// ‘\0’可表示C++中的NULL for (int i = 0; i < filePath.Length; i++) { if (!string.IsNullOrEmpty(filePath[i])&&i!=0) { fliePath.Add(filePath[0]+ filePath[i]); } } } else { Debug.Log("取消选择"); } return fliePath; } }
博客园Jason_c微信打赏码
如果本篇文档对你有帮助,打赏Jason_c根华子吧,他的私房钱被老婆没收了,呜呜!
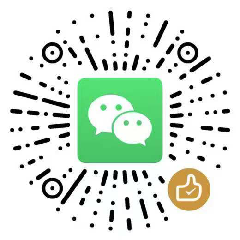