作业要求
作业1要求
- 路由综合练习。默认显示首页,当单击导航中的导航项时,会导航到相应的页面。
作业2要求
- 页面间导航练习,在作业 1 的基础上,添加按钮来控制页面间的导航。
- 单击“后退”按钮可以后退一步记录,跳转到上一个页面。
- 单击“前进”按钮可以在浏览器记录中前进一步。
- 单击“跳步 - 2”按钮可以后退两步记录,跳转到上上个页面。
- 单击 push 按钮可以跳转到“陈兰英”组件 。
- 单击 replace 按钮可以使用“陈兰英”组件替换当前组件 。
源码
App.vue
<template>
<div id="app">
<ul class="nav">
<li>
<router-link to="/">黄子涵</router-link>
</li>
<li>
<router-link to="/huangkaizhuo">黄开卓</router-link>
</li>
<li>
<router-link to="/chenlanying">陈兰英</router-link>
</li>
<li>
<router-link to="/huangchunqin">黄春钦</router-link>
</li>
<li>
<router-link to="/shejiazi">佘佳梓</router-link>
</li>
</ul>
<router-view class="view"/>
<button class="hzhBtn" v-on:click="backOff">后退</button>
<button class="hzhBtn" v-on:click="forward">前进</button>
<button class="hzhBtn" v-on:click="skipStep">跳步-2</button>
<button class="hzhBtn" v-on:click="push">push</button>
<button class="hzhBtn" v-on:click="replace">replace</button>
</div>
</div>
</template>
<script>
export default {
name: "App",
methods: {
backOff () {
this.$router.go(-1);
},
forward () {
this.$router.go(1);
},
skipStep () {
this.$router.go(-2);
},
push () {
this.$router.push("/chenlanying");
},
replace () {
this.$router.replace("/chenlanying");
},
},
};
</script>
<style>
#app {
width: 500px;
}
.nav {
width: 450px;
height: 45px;
line-height: 45px;
background: #f8f8f8;
margin: 20px auto 0;
border: 1px solid #dfdfdf;
border-radius: 2px;
font-weight: 500;
}
.nav li {
float: left;
width: 80px;
text-align: center;
color: #666;
list-style: none;
}
.banner {
padding: 10px 0 ;
}
.banner h3 {
font-size: 24px;
}
a {
color: #303133;
text-decoration: none;
}
a:hover {
color: #40aaff;
}
.view {
padding-left: 200px;
}
.hzhBtn {
width: 70px;
height: 50px;
font-weight: 500;
padding: 5px 15px;
background: #3877eb;
color: #fff;
border-radius: 4px;
margin-right: 5px;
border: none;
margin-right: 20px;
}
</style>
组件
// huangZiHan.vue
<template>
<div class="banner">
<h3>黄子涵</h3>
</div>
</template>
<script>
export default {
}
</script>
<style>
</style>
// huangKaiZhuo.vue
<template>
<div class="banner">
<h3>黄开卓</h3>
</div>
</template>
<script>
export default {
}
</script>
<style>
</style>
// chenLanYing.vue
<template>
<div class="banner">
<h3>陈兰英</h3>
</div>
</template>
<script>
export default {};
</script>
<style>
</style>
// huangChunQin.vue
<template>
<div class="banner">
<h3>黄春钦</h3>
</div>
</template>
<script>
export default {
}
</script>
<style>
</style>
// sheJiaZi.vue
<template>
<div class="banner">
<h3>佘佳梓</h3>
</div>
</template>
<script>
export default {};
</script>
<style>
</style>
路由
// index.js
import Vue from 'vue'
import Router from 'vue-router'
Vue.use(Router)
export default new Router({
routes: [
{
path: '*',
redirect: '/'
},
{
path: '/',
name: 'huangZihan',
component: () => import('../components/huangZiHan.vue'),
meta: { title: '黄子涵'}
},
{
path: '/huangkaizhuo',
name: 'huangKaiZhuo',
component: () => import('../components/huangkaiZhuo.vue'),
meta: { title: '陈兰英'}
},
{
path: '/chenlanying',
name: 'chenLanYing',
component: () => import('../components/chenLanYing.vue'),
meta: { title: '陈兰英'}
},
{
path: '/huangchunqin',
name: 'huangChunQin',
component: () => import('../components/huangChunQin.vue'),
meta: { title: '黄春钦'}
},
{
path: '/shejiazi',
name: 'sheJiaZi',
component: () => import('../components/sheJiaZi.vue'),
meta: { title: '佘佳梓'}
},
]
})
main.js
// The Vue build version to load with the `import` command
// (runtime-only or standalone) has been set in webpack.base.conf with an alias.
import Vue from 'vue'
import App from './App'
import router from './router'
Vue.config.productionTip = false
/* eslint-disable no-new */
new Vue({
el: '#app',
router: router,
components: { App },
template: '<App/>',
render:h => h(App),
})
效果
默认首页
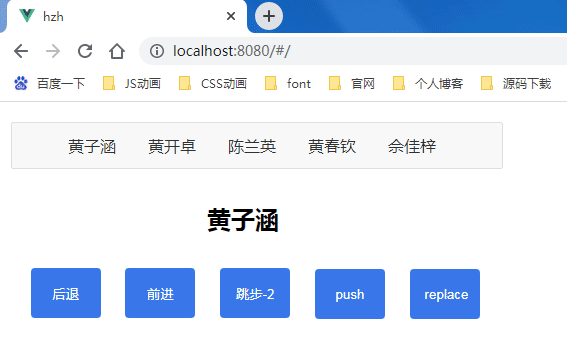
导航到页面
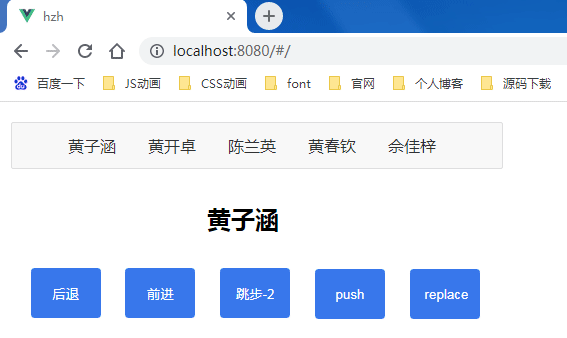
后退按钮
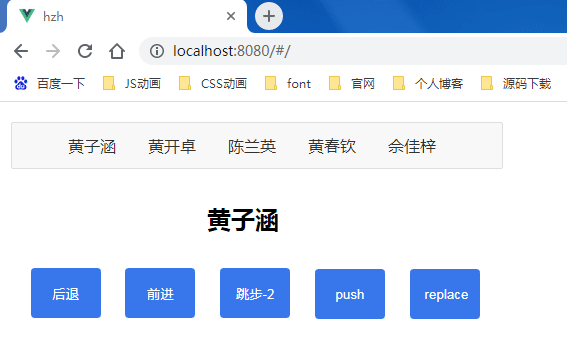
前进按钮
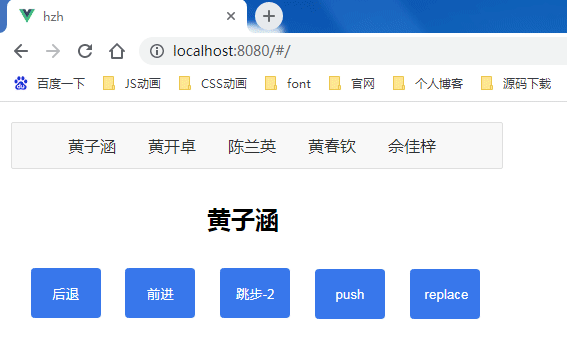
跳步按钮
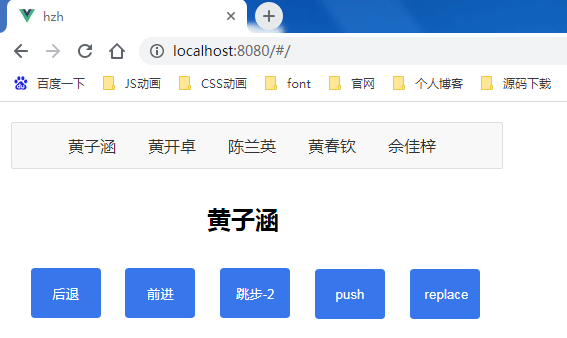
push 按钮
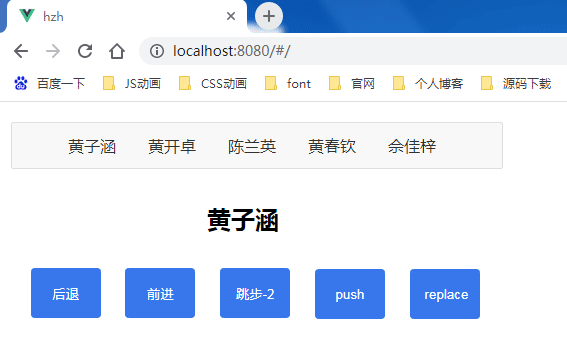
replace 按钮
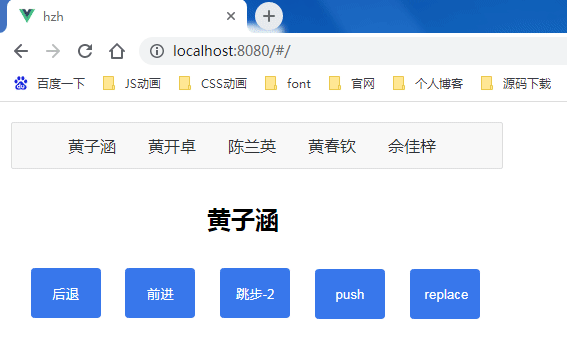