[LeetCode]232. Implement Queue using Stacks
232. Implement Queue using Stacks
class MyQueue(object):
def __init__(self):
"""
Initialize your data structure here.
"""
self.stack = []
self.stack_temp = []
def push(self, x):
"""
Push element x to the back of queue.
:type x: int
:rtype: void
"""
if self.stack_temp:
while self.stack_temp:
self.stack.append(self.stack_temp.pop())
self.stack.append(x)
def pop(self):
"""
Removes the element from in front of queue and returns that element.
:rtype: int
"""
while self.stack:
self.stack_temp.append(self.stack.pop())
if self.stack_temp:
return self.stack_temp.pop()
return None
def peek(self):
"""
Get the front element.
:rtype: int
"""
if self.stack_temp:
return self.stack_temp[len(self.stack_temp)-1]
elif self.stack:
while self.stack:
self.stack_temp.append(self.stack.pop())
if self.stack_temp:
return self.stack_temp[len(self.stack)-1]
return None
def empty(self):
"""
Returns whether the queue is empty.
:rtype: bool
"""
return False if self.stack or self.stack_temp else True
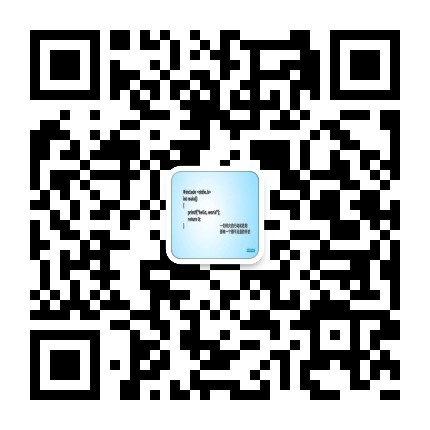
关注公众号:数据结构与算法那些事儿,每天一篇数据结构与算法