[LeetCode]Is Subsequence
392. Is Subsequence
动态规划
class Solution(object):
def isSubsequence(self, s, t):
"""
:type s: str
:type t: str
:rtype: bool
"""
if not s and not t:
return True
elif not s and t:
return True
elif not t and s:
return False
m, n = len(t), len(s)
dp = [[False for i in range(n + 1)] for j in range(m + 1)]
dp[0][0] = True
for i in range(1, m + 1):
dp[i][0] = True
for i in range(1, n + 1):
dp[0][i] = False
for i in range(1, m + 1):
for j in range(1, n + 1):
if t[i-1] == s[j-1]:
dp[i][j] = dp[i - 1][j - 1]
else:
dp[i][j] = dp[i - 1][j]
return dp[m][n]
class Solution(object):
def isSubsequence(self, s, t):
"""
:type s: str
:type t: str
:rtype: bool
"""
if not s and not t:
return True
elif not s and t:
return True
elif not t and s:
return False
m, n = len(t), len(s)
dp = [[False for j in range(m + 1)] for i in range(2)]
for i in range(m + 1):
dp[0][i] = True
for i in xrange(1, n+1):
for j in xrange(1, m+1):
if s[i-1] == t[j-1]:
dp[1][j] = dp[0][j-1]
else:
dp[1][j] = dp[1][j-1]
dp[0] = dp[1][:]
return dp[1][m]
不会
二分搜索
import collections
class Solution(object):
def isSubsequence(self, s, t):
"""
:type s: str
:type t: str
:rtype: bool
"""
def binary_search(nums, x):
low, high = 0, len(nums)
while low < high:
mid = (low + high) // 2
if nums[mid] < x:
low = mid + 1
else:
high = mid
return high
if not s and not t:
return True
elif not s and t:
return True
elif not t and s:
return False
pre_idx = 0
idxs = collections.defaultdict(list)
for i,n in enumerate(t):
idxs[n].append(i)
for i in range(len(s)):
idx = binary_search(idxs[s[i]], pre_idx)
if idx != len(idxs[s[i]]):
pre_idx = idxs[s[i]][idx] + 1
else:
return False
return True
贪心
class Solution(object):
def isSubsequence(self, s, t):
"""
:type s: str
:type t: str
:rtype: bool
"""
if not s and not t:
return True
elif not s and t:
return True
elif not t and s:
return False
pre_idx = -1
for i in range(len(s)):
idx = t.find(s[i], pre_idx+1)
if idx != -1:
pre_idx = idx
else:
return False
return True
滑动窗口
class Solution(object):
def isSubsequence(self, s, t):
"""
:type s: str
:type t: str
:rtype: bool
"""
if not s and not t:
return True
elif not s and t:
return True
elif not t and s:
return False
m, n = len(s), len(t)
si, ti = 0, 0
while si < m and ti < n:
if s[si] == t[ti]:
si += 1
ti += 1
return si == m
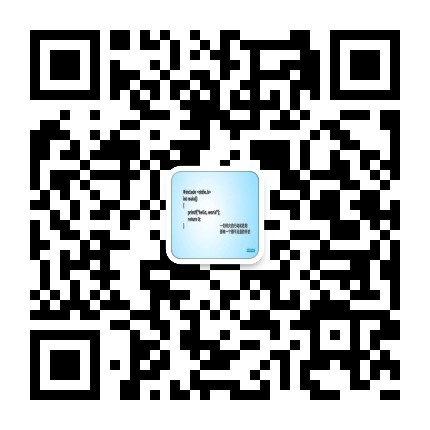
关注公众号:数据结构与算法那些事儿,每天一篇数据结构与算法