#多元线性回归模型
from sklearn.datasets import load_boston# 导入boston房价数据集
from sklearn.linear_model import LinearRegression
from sklearn.preprocessing import PolynomialFeatures
import numpy
#1、读取数据集:波士顿房价数据集(加载数据)
data = load_boston()
data.keys()

#查看每一个key值
print(data.data[0])
print(data.target[0:20])#房价预测
print(data.feature_names)#人口密度犯罪率这些变量
print(data.DESCR)
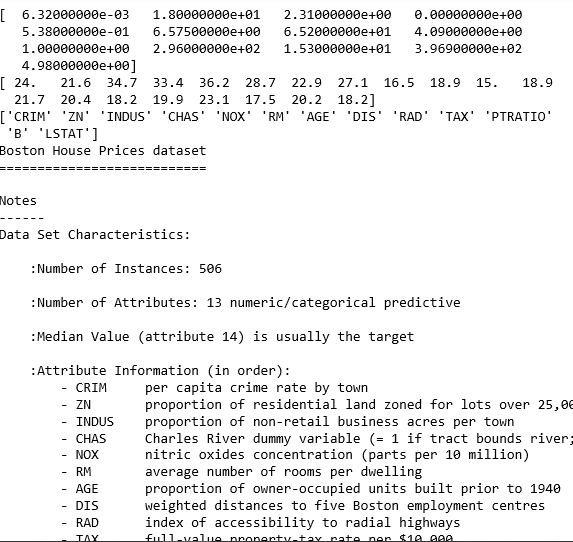
#2、训练集与测试集划分(划分数据集)
from sklearn.model_selection import train_test_split
x_train, x_test, y_train, y_test = train_test_split(data.data,data.target,test_size=0.3)
print(x_train.shape,y_train.shape)
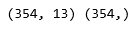

#3、线性回归模型:建立13个变量与房价之间的预测模型,并检测模型好坏(建立多元线性回归模型)
mlr = LinearRegression()
mlr.fit(x_train,y_train)
print('系数',mlr.coef_,"\n截距",mlr.intercept_)

# 检测模型好坏
from sklearn.metrics import regression
y_predict = mlr.predict(x_test)
# 计算模型的预测指标
print("预测的均方误差:", regression.mean_squared_error(y_test,y_predict))
print("预测的平均绝对误差:", regression.mean_absolute_error(y_test,y_predict))
# 打印模型的分数
print("模型的分数:",mlr.score(x_test, y_test))
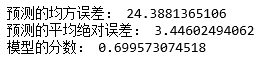
#4、多项式回归模型:建立13个变量与房价之间的预测模型,并检测模型好坏。(多元多项式回归模型)
# 多项式化
poly2 = PolynomialFeatures(degree=2)
x_poly_train = poly2.fit_transform(x_train)
x_poly_test = poly2.transform(x_test)
# 建立模型
mlrp = LinearRegression()
mlrp.fit(x_poly_train, y_train)

# 预测
y_predict2 = mlrp.predict(x_poly_test)
# 检测模型好坏
# 计算模型的预测指标
print("预测的均方误差:", regression.mean_squared_error(y_test,y_predict2))
print("预测的平均绝对误差:", regression.mean_absolute_error(y_test,y_predict2))
# 打印模型的分数
print("模型的分数:",mlrp.score(x_poly_test, y_test))
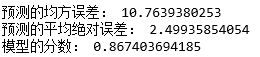
5、比较线性模型与非线性模型的性能,并说明原因。 性能比较:多项式回归做出来的模型显然会好一点。因为多项式模型不仅仅是一条直线,它是一条平滑的曲线,更贴合样本点的分布。并且多项式回归模型的误差也明显比线性的小。
二、中文文本分类:新闻文本分类(全部数据): 定义函数:读数据,清洗,分词。标签存入target_list,文本存入content_list
import os
import numpy as np
import sys
from datetime import datetime
import gc
path = 'F:\\大作业\\shuju'
# 导入结巴库,并将需要用到的词库加进字典
import jieba
# 导入(加载)停用词:
with open(r'F:\大作业\stopsCN.txt', encoding='utf-8') as f:
stopwords = f.read().split('\n')
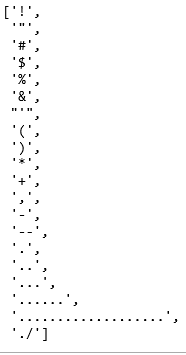
def processing(tokens):
tokens = "".join([char for char in tokens if char.isalpha()])
tokens = [token for token in jieba.cut(tokens,cut_all=True) if len(token) >=2]
tokens = " ".join([token for token in tokens if token not in stopwords])
return tokens
tokenList = []
targetList = []
for root,dirs,files in os.walk(path):
# print(root)
# print(dirs)
# print(files)
for filetext in files:
Filetext = os.path.join(root,filetext)
print(Filetext)
with open(Filetext,'r',encoding='utf-8') as f:
content = f.read() # 获取文本
target = Filetext.split('\\')[-2]
targetList.append(target)
# print(targetList)
tokenList.append(processing(content))
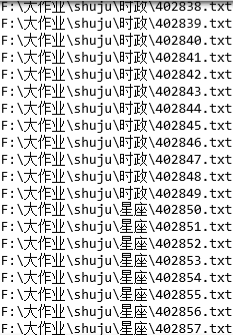
#将content_list列表向量化再建模,将模型用于预测并评估模型
from sklearn.feature_extraction.text import TfidfVectorizer#TF-IDF算法进行权值的计算
from sklearn.model_selection import train_test_split
from sklearn.naive_bayes import GaussianNB,MultinomialNB
from sklearn.model_selection import cross_val_score
from sklearn.metrics import classification_report
x_train,x_test,y_train,y_test = train_test_split(tokenList,targetList,test_size=0.2,stratify=targetList)
vectorizer = TfidfVectorizer()
X_train = vectorizer.fit_transform(x_train)
X_test = vectorizer.transform(x_test)
mnb = MultinomialNB()#多项式朴素贝叶斯建模
module = mnb.fit(X_train, y_train)
#拿到模型进行预测
y_predict = module.predict(X_test)
# 输出模型精确度
scores=cross_val_score(mnb,X_test,y_test,cv=5)
print("Accuracy:%.3f"%scores.mean())
# 输出模型评估报告
print("classification_report:\n",classification_report(y_predict,y_test))
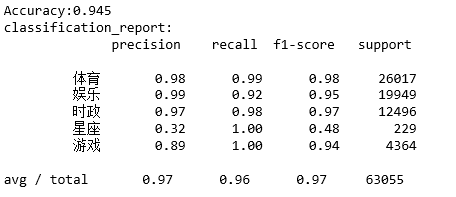
targetList.append(target)
print(targetList[0:10])
tokenList.append(processing(content))
tokenList[0:10]
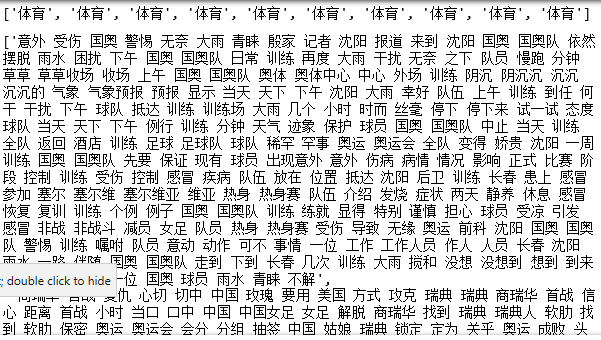
#预测和实际结果的可视化对比
import collections
# 统计测试集和预测集的各类新闻个数
testCount = collections.Counter(y_test)
predCount = collections.Counter(y_predict)
print('实际:',testCount,'\n', '预测', predCount)

#标签列表,实际结果列表,预测结果列表
nameList = list(testCount.keys())
testList = list(testCount.values())
predictList = list(predCount.values())
x = list(range(len(nameList)))
print("新闻类别:",nameList,'\n',"实际:",testList,'\n',"预测:",predictList)
