两道算法题
2018-07-06 17:47 FuzhePan 阅读(142) 评论(0) 编辑 收藏 举报一,大整数加法,链表实现。
比如 123 + 456 = 579

代码实现
class LinkedNode{ public int value; public LinkedNode next; public static LinkedNode build(int num){ LinkedNode node = new LinkedNode(); LinkedNode tail = node; while(num > 0){ tail.value = num % 10; tail.next = new LinkedNode(); tail = tail.next; num = num / 10; } return node; } public String toString(){ StringBuilder sb = appendValue(new StringBuilder(),this); StringBuilder reversedSB = sb.reverse(); return reversedSB.toString(); } private StringBuilder appendValue(StringBuilder sb,LinkedNode node){ if(node == null){ return sb; } sb.append(node.value); return appendValue(sb,node.next); } } public class BigIntegerCaculator { /** * 基于链表实现大整数加法 * @param node0 * @param node1 * @return */ public LinkedNode add(LinkedNode node0,LinkedNode node1){ LinkedNode result = new LinkedNode(); LinkedNode tailNode = result; int carryValue = 0; while(node0 != null || node1 != null || carryValue > 0){ int node0Value = node0 == null ? 0 : node0.value; int node1Value = node1 == null ? 0 : node1.value; int sumedValue = node0Value + node1Value + carryValue; carryValue = sumedValue / 10; tailNode.value = sumedValue % 10; tailNode.next = new LinkedNode(); tailNode = tailNode.next; node0 = node0 == null ? null : node0.next; node1 = node1 == null ? null : node1.next; } return result; } /** * 测试 * @param args */ public static void main(String[] args) { /* LinkedNode node0 = LinkedNode.build(123); LinkedNode node1 = LinkedNode.build(456); BigIntegerCaculator caculator = new BigIntegerCaculator(); LinkedNode sumNode = caculator.add(node0,node1); System.out.println(node0.toString() + "+" +node1.toString() + "=" + sumNode.toString()); */ LinkedNode node2 = LinkedNode.build(789); LinkedNode node3 = LinkedNode.build(89); BigIntegerCaculator caculator = new BigIntegerCaculator(); LinkedNode sumNode = caculator.add(node2,node3); System.out.println(node2.toString() + "+" +node3.toString() + "=" + sumNode.toString()); } }
二,数组,统计环的个数,环都是独立的,不考虑不成环的情况。
比如下图,第0个元素存储的是下一个元素的索引,所以第0、2、5个元素构成一个环,1本身构成一个环。
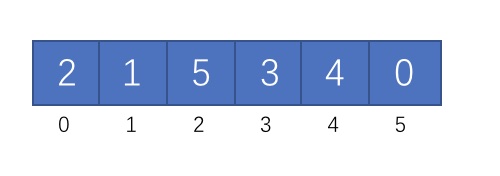
分析:首先需要遍历数组,遍历到每个元素时,递归查找指向的下一个元素,并把查找路径存储到set集合中,如果set集合中已经包含此元素,则构成一个环。复杂度是o(n)
代码实现
public class LoopCount { public int loopCount(int[] array){ Set<Integer> set = new HashSet<>(); int count = 0; for(int i = 0; i< array.length; i++){ if(set.contains(i)){ continue; } if(isLoop(array,set,i)){ count++; } } return count; } private boolean isLoop(int[] array,Set<Integer> set,int index){ set.add(index); if(set.contains(array[index])){ return true; } return isLoop(array,set,array[index]); } public static void main(String[] args) { int[] array = new int[]{2,1,5,3,4,0}; System.out.println(new LoopCount().loopCount(array)); } }