JavaGUI-02-Swing
Swing简介
Swing封装了AWT(AWT更底层)
1.窗口-JFrame
public class JFrameDemo {
public static void main(String[] args) {
MyJFrame jf = new MyJFrame("jf");
jf.init();
}
}
class MyJFrame extends JFrame{
public MyJFrame(String title){
this.setTitle(title);
}
//初始化
public void init(){
//获得一个容器
Container container = this.getContentPane();
container.setBackground(Color.black);
this.setBounds(300,300,200,200);
this.setVisible(true);
JLabel jlabel = new JLabel(this.getTitle());
//设置文本居中
jlabel.setHorizontalAlignment(SwingConstants.CENTER);
this.add(jlabel);
//设置关闭事件
this.setDefaultCloseOperation(WindowConstants.EXIT_ON_CLOSE);
}
}
2.弹窗-JDialog
public class DialogDemo {
public static void main(String[] args) {
new MainJFrame().init();
}
}
//主窗口
class MainJFrame extends JFrame{
public void init(){
this.setBounds(200,200,500,400);
this.setVisible(true);
//添加窗口关闭事件
this.setDefaultCloseOperation(WindowConstants.EXIT_ON_CLOSE);
//拿到容器(用容器来放组件)
Container container = this.getContentPane();
//设置为绝对布局(通过坐标指定组件的位置)
container.setLayout(null);
JButton jButton = new JButton("Button");
jButton.setBounds(0,0,100,50);
//点击这个按钮打开一个弹窗
jButton.addActionListener(new ActionListener() {//监听器
@Override
public void actionPerformed(ActionEvent actionEvent) {
//实例化弹窗
MyDialog myDialog = new MyDialog();
myDialog.init();
}
});
container.add(jButton);
}
}
//弹窗
class MyDialog extends JDialog{
public void init(){
//注意JDialog默认有窗口关闭事件 不用自己添加
this.setBounds(500,100,200,100);
this.setVisible(true);
Container container = this.getContentPane();
JLabel jLabel = new JLabel("this is a dialog");
container.add(jLabel);
}
}
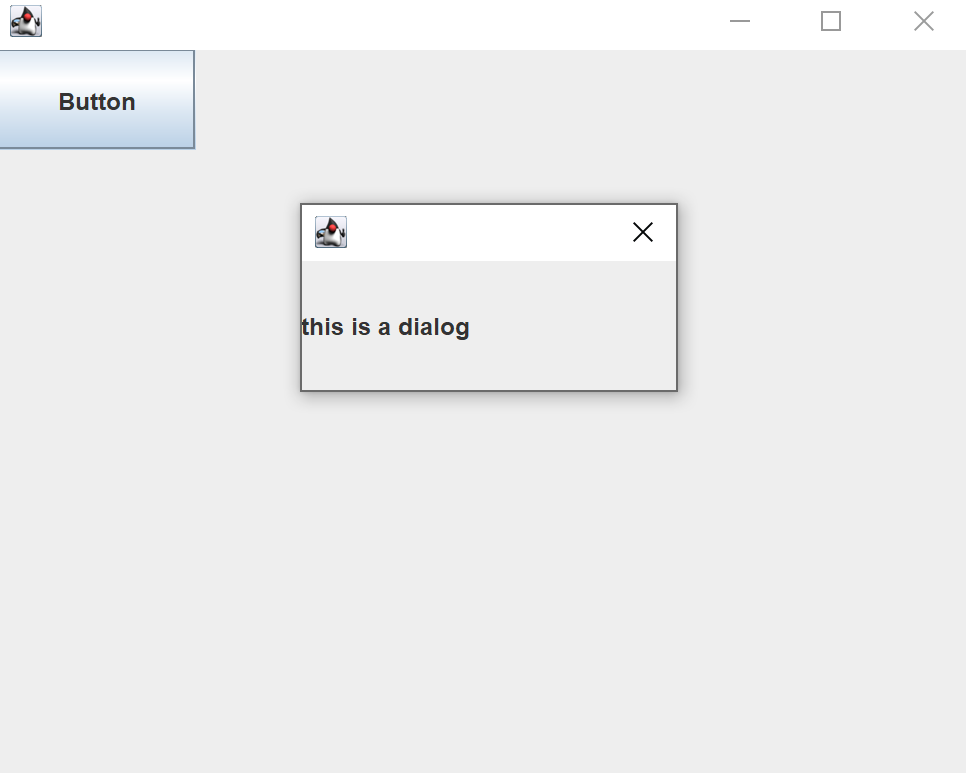
3.标签
文字lable
new JLable("xxx");
有ICON的Lable
1.用Java绘制的Icon
public class Lable_IconDemo {
public static void main(String[] args) {
new MainFrame().init();
}
}
class MainFrame extends JFrame{
public void init(){
this.setBounds(200,200,300,200);
this.setVisible(true);
//实例化一个带有Icon的JLable
//JLabel(标签的名字, Icon, 标签的位置)
JLabel jLabel = new JLabel(" Icon'name ", new MyIcon(15, 15), SwingConstants.CENTER);
Container container = this.getContentPane();
container.add(jLabel);
}
}
//implements Icon接口,需要实现接口的所有方法
class MyIcon implements Icon{
//参数
private int width = 0;
private int height = 0;
MyIcon(){}//无参构造
MyIcon(int width, int height){
this.width = width;
this.height = height;
}
@Override
public void paintIcon(Component component, Graphics graphics, int x, int y) {
//画Icon (xy坐标会根据jLabel的文字部分匹配,默认放在文字之前)
graphics.fillOval(x, y, this.width, this.height);
}
@Override
public int getIconWidth() {
return this.width;
}
@Override
public int getIconHeight() {
return this.height;
}
}
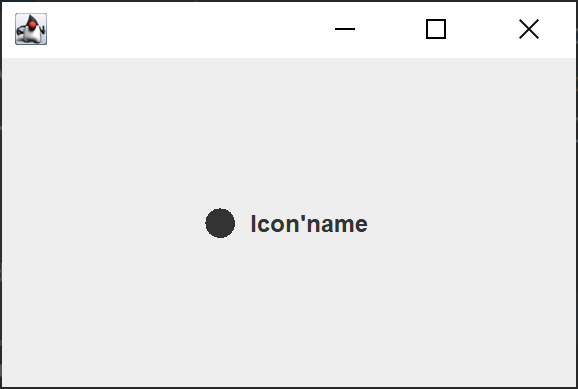
2.使用准备好的图片作为Icon
public class Lable_ImageIconDemo {
public static void main(String[] args) {
new MyFrame().init();
}
}
class MyFrame extends JFrame{
public void init(){
this.setBounds(200,200,300,200);
this.setVisible(true);
//Home标签
JLabel jLabel = new JLabel(" Home ");
//获取图片的地址(url)
//xxx.class.getResource() 获取与xxx类同级路径下的资源
URL home_png_url = MyFrame.class.getResource("home.png");
//实例化ImageIcon
ImageIcon Home_Icon = new ImageIcon(home_png_url);
//添加Icon
jLabel.setIcon(Home_Icon);
//设置居中
jLabel.setHorizontalAlignment(SwingConstants.CENTER);
Container container = this.getContentPane();
container.add(jLabel);
}
}
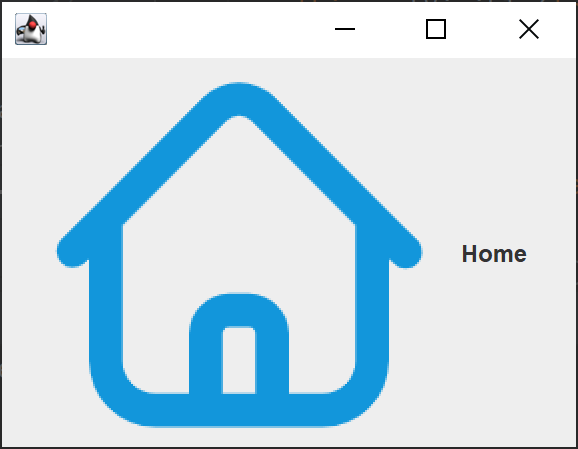
4.面板
简单使用
public class JPanelDemo {
public static void main(String[] args) {
new MyJF().init();
}
}
class MyJF extends JFrame{
public void init(){
this.setBounds(200,200,400,300);
this.setVisible(true);
Container container = this.getContentPane();
container.setLayout(new GridLayout(2,2,15,10));//后面两个参数是行列间距
JPanel jPanel1 = new JPanel(new GridLayout(1,1));
JPanel jPanel2 = new JPanel(new GridLayout(2,1));
JPanel jPanel3 = new JPanel(new GridLayout(3,1));
JPanel jPanel4 = new JPanel(new GridLayout(4,1));
jPanel1.add(new JButton("1"));
jPanel2.add(new JButton("2"));
jPanel2.add(new JButton("2"));
jPanel3.add(new JButton("3"));
jPanel3.add(new JButton("3"));
jPanel3.add(new JButton("3"));
jPanel4.add(new JButton("4"));
jPanel4.add(new JButton("4"));
jPanel4.add(new JButton("4"));
jPanel4.add(new JButton("4"));
container.add(jPanel1);
container.add(jPanel2);
container.add(jPanel3);
container.add(jPanel4);
}
}
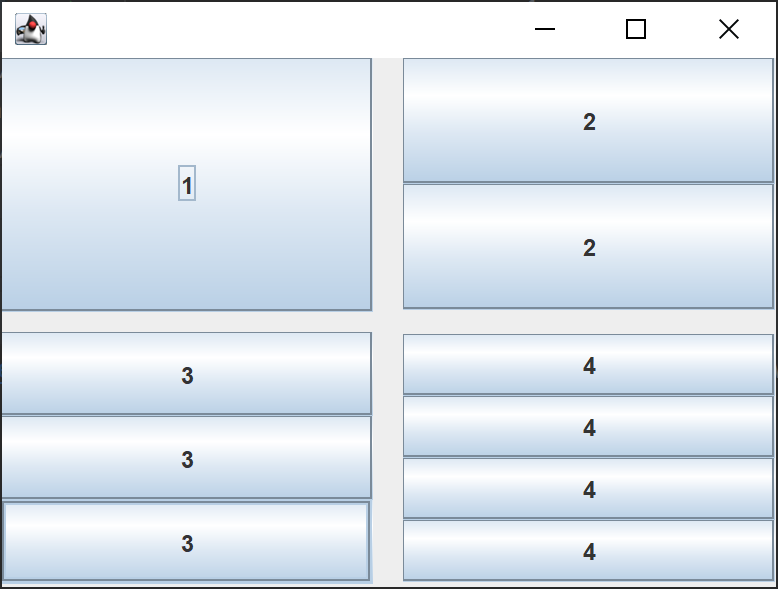
实现滚动条-JScrollPanel
public class JScrollPanel_Demo {
public static void main(String[] args) {
new MyTextJF().init();
}
}
class MyTextJF extends JFrame{
public void init(){
this.setBounds(200,200,300,200);
this.setVisible(true);
JTextArea jTextArea = new JTextArea();
jTextArea.setText("请输入文字:");
//把文本域放到面板
JScrollPane jScrollPane = new JScrollPane(jTextArea);
Container container = this.getContentPane();
//把面板放到文本域
container.add(jScrollPane);
}
}
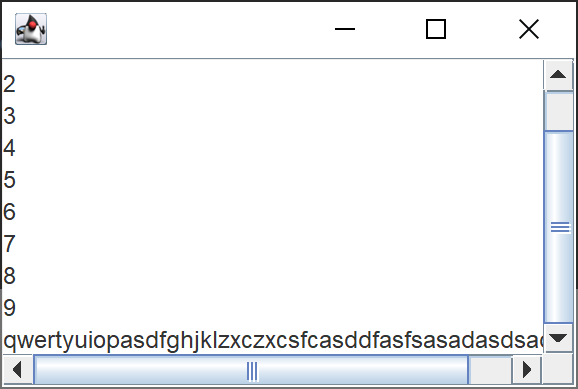
5.按钮
- 图片按钮
public class JButton_IconButton_Test {
public static void main(String[] args) {
new MyFrame01().init();
}
}
class MyFrame01 extends JFrame{
public void init(){
this.setBounds(200,200,600,500);
this.setVisible(true);
//把图片变成Icon
URL resource = MyFrame01.class.getResource("home.png");
ImageIcon home_Icon = new ImageIcon(resource);
//按钮
JButton jButton = new JButton();
//设置按钮Icon
jButton.setIcon(home_Icon);
//设置提示文字
jButton.setToolTipText("return home");
Container container = this.getContentPane();
container.add(jButton,BorderLayout.SOUTH);
}
}
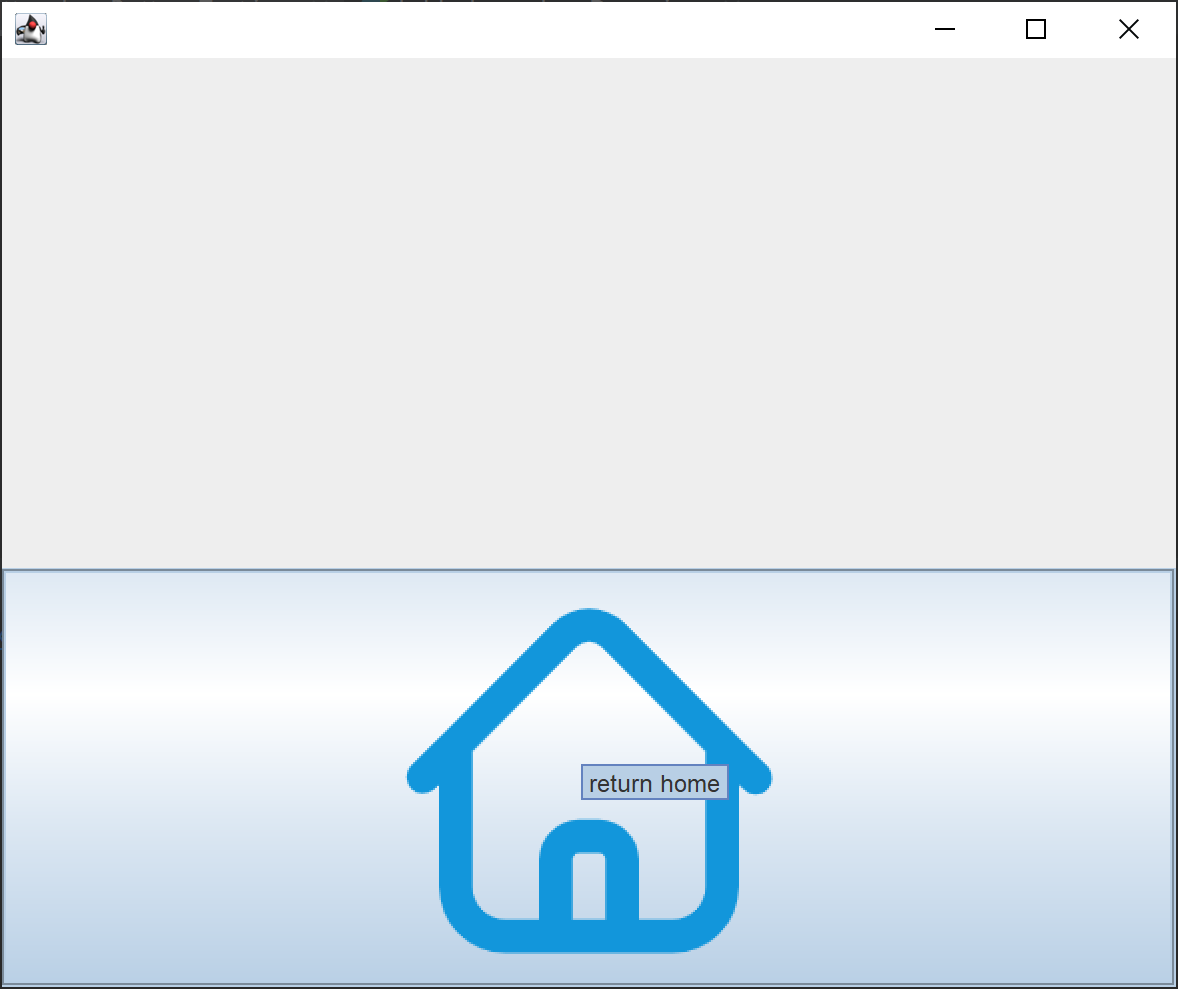
- 单选按钮-radioButton
public class JRadioButton_Demo02 {
public static void main(String[] args) {
new MyFrame02().init();
}
}
class MyFrame02 extends JFrame {
public void init(){
this.setBounds(200,200,600,500);
this.setVisible(true);
this.setDefaultCloseOperation(WindowConstants.EXIT_ON_CLOSE);
//单选按钮
JRadioButton jRadioButton1 = new JRadioButton("jRadioButton1");
JRadioButton jRadioButton2 = new JRadioButton("jRadioButton2");
JRadioButton jRadioButton3 = new JRadioButton("jRadioButton3");
//添加到同一个组中,一个组中只能选择一个
ButtonGroup buttonGroup = new ButtonGroup();
buttonGroup.add(jRadioButton1);
buttonGroup.add(jRadioButton2);
buttonGroup.add(jRadioButton3);
Container container = this.getContentPane();
container.add(jRadioButton1,BorderLayout.NORTH);
container.add(jRadioButton2,BorderLayout.CENTER);
container.add(jRadioButton3,BorderLayout.SOUTH);
}
}
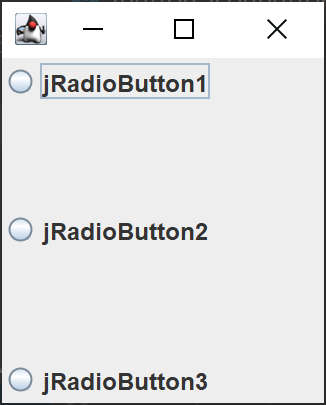
- 复选按钮
public class JCheckBox_Test {
public static void main(String[] args) {
new MyFrame03().init();
}
}
class MyFrame03 extends JFrame {
public void init(){
this.setBounds(200,200,600,500);
this.setVisible(true);
this.setDefaultCloseOperation(WindowConstants.EXIT_ON_CLOSE);
//复选框
JCheckBox jCheckBox1 = new JCheckBox("jCheckBox1");
JCheckBox jCheckBox2 = new JCheckBox("jCheckBox2");
Container container = this.getContentPane();
container.add(jCheckBox1,BorderLayout.NORTH);
container.add(jCheckBox2,BorderLayout.SOUTH);
}
}
6.列表
- 下拉框
public class ComboBox_Test {
public static void main(String[] args) {
new MyFrame01().init();
}
}
class MyFrame01 extends JFrame {
public void init(){
this.setBounds(200,200,600,500);
this.setVisible(true);
this.setDefaultCloseOperation(WindowConstants.EXIT_ON_CLOSE);
JComboBox status = new JComboBox();
status.addItem(null);
status.addItem("A: Right");
status.addItem("B: Wrong");
Container container = this.getContentPane();
container.add(status, BorderLayout.NORTH);
}
}
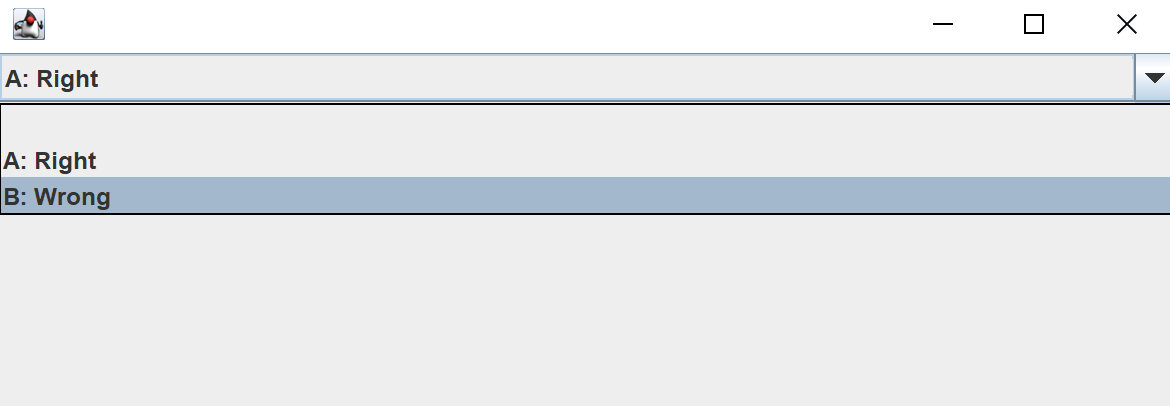
- 列表框
public class JList_Test {
public static void main(String[] args) {
new MyFrame02().init();
}
}
class MyFrame02 extends JFrame {
public void init(){
this.setBounds(200,200,600,500);
this.setVisible(true);
this.setDefaultCloseOperation(WindowConstants.EXIT_ON_CLOSE);
Vector contents = new Vector();
JList jList = new JList(contents);
contents.add("Godot");
contents.add("Unity");
contents.add("Unreal");
Container container = this.getContentPane();
container.add(jList);
}
}
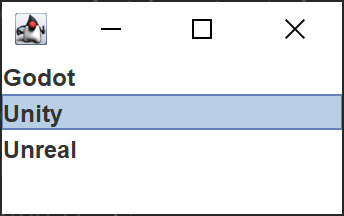
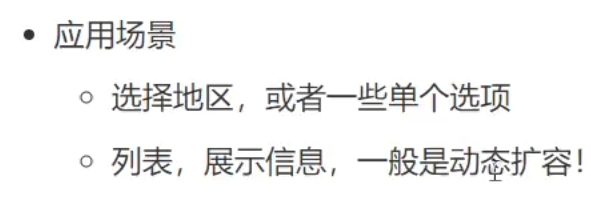
7.文本
- 文本框

- 密码框

- 文本域

GUI编程总结
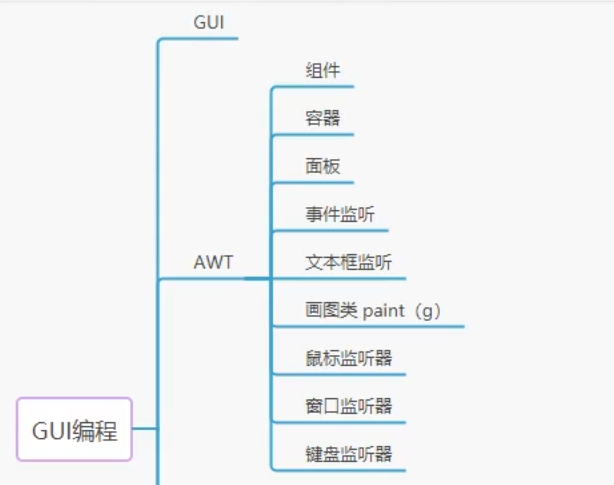
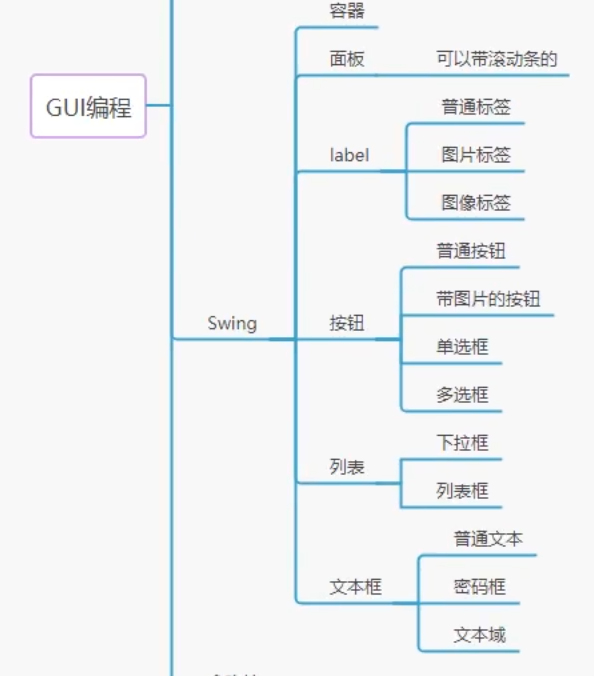