Qt-Qt之剪切板、热键应用(QClipboard、RegisterHotKey)
.pro

1 QT += core gui 2 3 greaterThan(QT_MAJOR_VERSION, 4): QT += widgets 4 5 CONFIG += c++11 6 7 # The following define makes your compiler emit warnings if you use 8 # any Qt feature that has been marked deprecated (the exact warnings 9 # depend on your compiler). Please consult the documentation of the 10 # deprecated API in order to know how to port your code away from it. 11 DEFINES += QT_DEPRECATED_WARNINGS 12 13 # You can also make your code fail to compile if it uses deprecated APIs. 14 # In order to do so, uncomment the following line. 15 # You can also select to disable deprecated APIs only up to a certain version of Qt. 16 #DEFINES += QT_DISABLE_DEPRECATED_BEFORE=0x060000 # disables all the APIs deprecated before Qt 6.0.0 17 18 SOURCES += \ 19 main.cpp \ 20 mainwindow.cpp 21 22 HEADERS += \ 23 mainwindow.h 24 25 FORMS += \ 26 mainwindow.ui 27 28 LIBS += \ 29 -lUser32 30 31 # Default rules for deployment. 32 qnx: target.path = /tmp/$${TARGET}/bin 33 else: unix:!android: target.path = /opt/$${TARGET}/bin 34 !isEmpty(target.path): INSTALLS += target
main.cpp

1 #include "mainwindow.h" 2 3 #include <QApplication> 4 5 int main(int argc, char *argv[]) 6 { 7 QApplication a(argc, argv); 8 MainWindow w; 9 w.show(); 10 return a.exec(); 11 }
mainwindow.h

1 #ifndef MAINWINDOW_H 2 #define MAINWINDOW_H 3 4 #include <windows.h> 5 6 #include <QMainWindow> 7 #include <QClipboard> 8 #include <QMimeData> 9 #include <QDebug> 10 #include <QTimer> 11 12 // 热键id 13 #define HOT_KEY_CTRL_2 0 14 #define HOT_KEY_CTRL_3 1 15 16 QT_BEGIN_NAMESPACE 17 namespace Ui { class MainWindow; } 18 QT_END_NAMESPACE 19 20 class MainWindow : public QMainWindow 21 { 22 Q_OBJECT 23 24 public: 25 MainWindow(QWidget *parent = nullptr); 26 ~MainWindow(); 27 28 bool nativeEvent(const QByteArray &eventType, void *message, long *result); 29 private slots: 30 void on_pushButton_clicked(); 31 32 void on_pushButton_2_clicked(); 33 34 void on_pushButton_3_clicked(); 35 36 void on_pushButton_6_clicked(); 37 38 void on_pushButton_4_clicked(); 39 40 private: 41 void on_ClipboardChanged(); 42 void on_ClipboardAdd(); 43 private: 44 Ui::MainWindow *ui; 45 QClipboard *m_pClipboard = NULL; 46 bool m_bSwitch = false; 47 bool m_bReadFinish = false; 48 QString m_Txt = ""; 49 QTimer *m_pTimer = NULL; 50 }; 51 #endif // MAINWINDOW_H
mainwindow.cpp

1 // 开启监听Alt + 2 2 // 关闭监听Alt + 3 3 // 开启后可以复制、粘贴时,把\号改为/号。 4 5 #include "mainwindow.h" 6 #include "ui_mainwindow.h" 7 8 MainWindow::MainWindow(QWidget *parent) 9 : QMainWindow(parent) 10 , ui(new Ui::MainWindow) 11 { 12 ui->setupUi(this); 13 14 setWindowTitle(QStringLiteral("Qt之剪切板、热键应用")); 15 16 QClipboard *m_pClipboard = QApplication::clipboard(); //获取系统剪贴板指针 17 connect(m_pClipboard, &QClipboard::changed, this, &MainWindow::on_ClipboardChanged); 18 19 if (!RegisterHotKey(HWND(winId()), HOT_KEY_CTRL_2, MOD_ALT, 0x32))// MOD_ALT | MOD_CONTROL 20 qDebug() << "注册热键 ALT + 2"; 21 if (!RegisterHotKey(HWND(winId()), HOT_KEY_CTRL_3, MOD_ALT, 0x33)) 22 qDebug() << "注册热键 ALT + 3"; 23 // 24 m_pTimer = new QTimer(this); 25 m_pTimer->setSingleShot(false); 26 connect(m_pTimer, &QTimer::timeout, this, &MainWindow::on_ClipboardAdd); 27 m_pTimer->start(10); 28 } 29 30 MainWindow::~MainWindow() 31 { 32 delete ui; 33 } 34 35 bool MainWindow::nativeEvent(const QByteArray &eventType, void *message, long *result) 36 { 37 Q_UNUSED(eventType); 38 Q_UNUSED(result); 39 MSG* msg = static_cast<MSG*>(message); 40 if (msg->message == WM_HOTKEY) 41 { 42 switch (msg->wParam) { 43 case HOT_KEY_CTRL_2: 44 qDebug() << "触发了: ALT + 2"; 45 m_bSwitch = true; 46 break; 47 case HOT_KEY_CTRL_3: 48 qDebug() << "触发了: ALT + 3"; 49 m_bSwitch = false; 50 break; 51 default: 52 qDebug() << "被注入了其他热键."; 53 } 54 return true; 55 } 56 return false; 57 } 58 59 void MainWindow::on_ClipboardChanged() 60 { 61 const QMimeData *mimeData = m_pClipboard->mimeData(); 62 if (mimeData->hasImage()) 63 { 64 /* 获取剪切板图像数据。 */ 65 QPixmap pixmap = qvariant_cast<QPixmap>(mimeData->imageData()); 66 // 67 } 68 else if (mimeData->hasHtml()) 69 { 70 /* 获取剪切板html数据。 */ 71 QString html = mimeData->html(); 72 // 73 } 74 else if (mimeData->hasText()) { 75 /* 获取剪切板纯文本数据。 */ 76 QString text = mimeData->text(); 77 // 78 if (m_bSwitch) 79 { 80 text = text.replace("\\", "/"); 81 ui->textEdit->setText(text); 82 m_bReadFinish = true; 83 m_Txt = text; 84 } 85 } 86 else { 87 qDebug() << "Cannot display data"; 88 } 89 } 90 91 void MainWindow::on_ClipboardAdd() 92 { 93 if (m_bReadFinish && m_Txt != "") 94 { 95 m_bReadFinish = false; 96 m_pClipboard->setText(m_Txt); 97 } 98 } 99 100 void MainWindow::on_pushButton_clicked() 101 { 102 m_bSwitch = true; 103 } 104 105 void MainWindow::on_pushButton_2_clicked() 106 { 107 m_bSwitch = false; 108 } 109 110 void MainWindow::on_pushButton_3_clicked() 111 { 112 m_pClipboard->setText("AAA"); 113 } 114 115 void MainWindow::on_pushButton_6_clicked() 116 { 117 ui->textEdit->setText(m_pClipboard->text()); 118 } 119 120 void MainWindow::on_pushButton_4_clicked() 121 { 122 QMimeData mimeData; 123 mimeData.setImageData(QPixmap("hello.png")); // 图像数据 124 // mimeData.setHtml("<h1>Heool world.</h1>"); // Html数据 125 // mimeData.setText("Hello world."); // 纯文本数据 126 m_pClipboard->setMimeData(&mimeData); 127 }
mainwindow.ui

1 <?xml version="1.0" encoding="UTF-8"?> 2 <ui version="4.0"> 3 <class>MainWindow</class> 4 <widget class="QMainWindow" name="MainWindow"> 5 <property name="geometry"> 6 <rect> 7 <x>0</x> 8 <y>0</y> 9 <width>859</width> 10 <height>372</height> 11 </rect> 12 </property> 13 <property name="windowTitle"> 14 <string>MainWindow</string> 15 </property> 16 <widget class="QWidget" name="centralwidget"> 17 <widget class="QPushButton" name="pushButton"> 18 <property name="geometry"> 19 <rect> 20 <x>640</x> 21 <y>10</y> 22 <width>80</width> 23 <height>20</height> 24 </rect> 25 </property> 26 <property name="text"> 27 <string>开启监听</string> 28 </property> 29 </widget> 30 <widget class="QPushButton" name="pushButton_2"> 31 <property name="geometry"> 32 <rect> 33 <x>640</x> 34 <y>30</y> 35 <width>80</width> 36 <height>20</height> 37 </rect> 38 </property> 39 <property name="text"> 40 <string>关闭监听</string> 41 </property> 42 </widget> 43 <widget class="QTextEdit" name="textEdit"> 44 <property name="geometry"> 45 <rect> 46 <x>20</x> 47 <y>10</y> 48 <width>601</width> 49 <height>241</height> 50 </rect> 51 </property> 52 </widget> 53 <widget class="QPushButton" name="pushButton_3"> 54 <property name="geometry"> 55 <rect> 56 <x>640</x> 57 <y>200</y> 58 <width>120</width> 59 <height>20</height> 60 </rect> 61 </property> 62 <property name="text"> 63 <string>向剪切板写入TXT</string> 64 </property> 65 </widget> 66 <widget class="QPushButton" name="pushButton_4"> 67 <property name="geometry"> 68 <rect> 69 <x>640</x> 70 <y>150</y> 71 <width>120</width> 72 <height>20</height> 73 </rect> 74 </property> 75 <property name="text"> 76 <string>向剪切板写入图片</string> 77 </property> 78 </widget> 79 <widget class="QPushButton" name="pushButton_6"> 80 <property name="geometry"> 81 <rect> 82 <x>640</x> 83 <y>220</y> 84 <width>120</width> 85 <height>20</height> 86 </rect> 87 </property> 88 <property name="text"> 89 <string>读取剪切板TXT内容</string> 90 </property> 91 </widget> 92 </widget> 93 </widget> 94 <resources/> 95 <connections/> 96 </ui>
作者:疯狂Delphi
本文版权归作者和博客园共有,欢迎转载,但未经作者同意必须保留此段声明,且在文章页面明显位置给出原文连接,否则保留追究法律责任的权利.
欢迎关注我,一起进步!扫描下方二维码即可加我
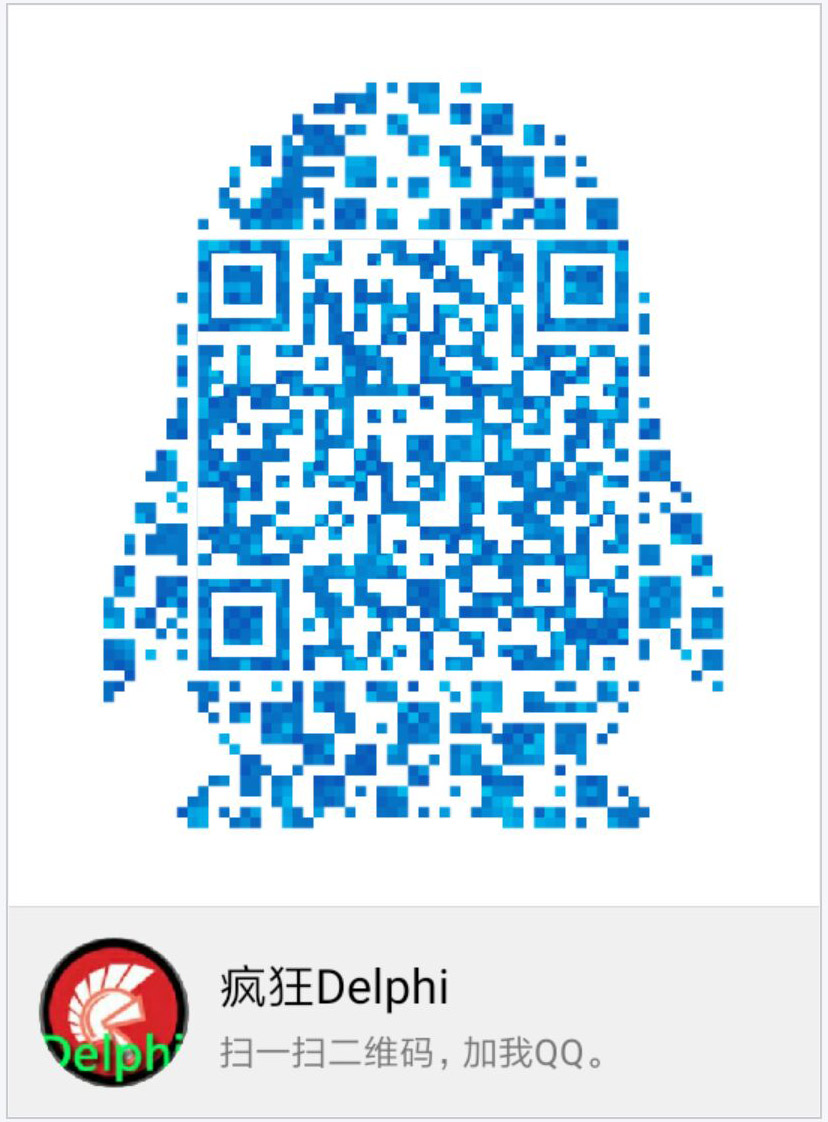
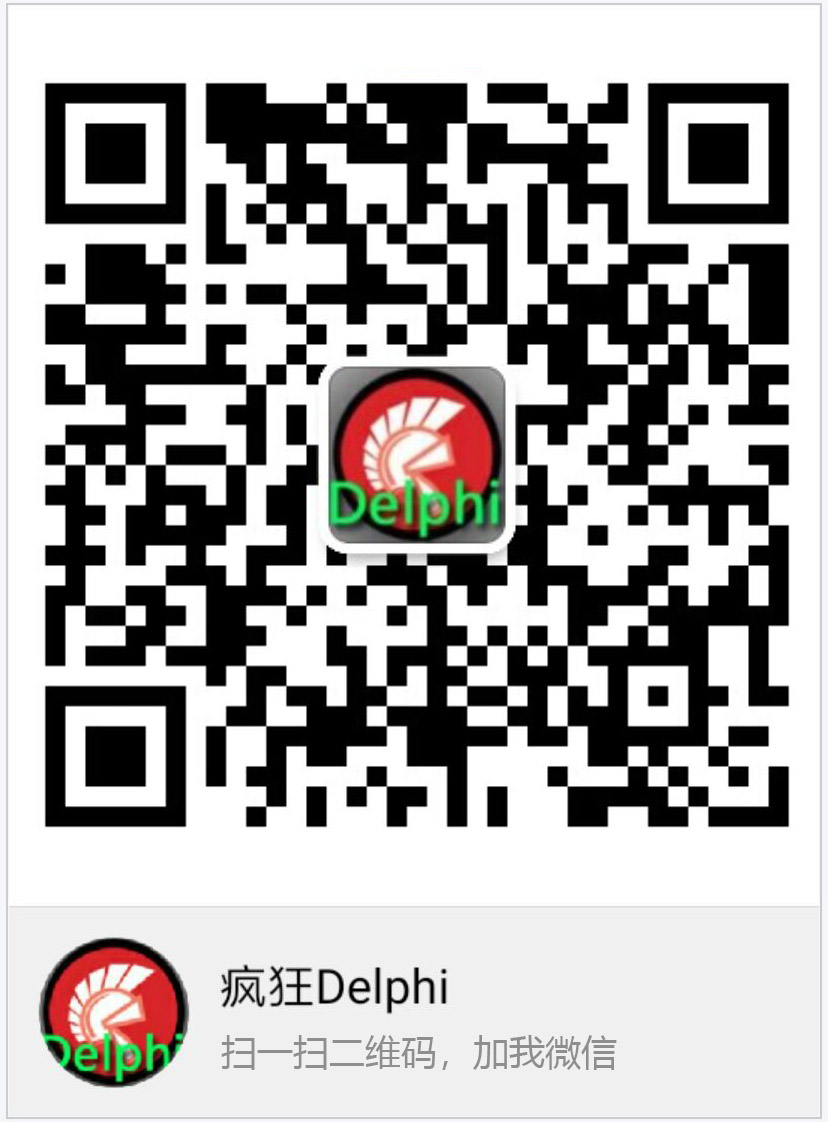