Qt-Qt之使用Activate控件(程序内显示需要安装Adobe PDF Reader)
相关资料
https://download.csdn.net/download/zhujianqiangqq/86665828 csdn代码包下载
.pro

1 QT += core gui 2 3 4 greaterThan(QT_MAJOR_VERSION, 4): QT += widgets 5 6 CONFIG += c++11 7 CONFIG += qaxcontainer 8 9 # The following define makes your compiler emit warnings if you use 10 # any Qt feature that has been marked deprecated (the exact warnings 11 # depend on your compiler). Please consult the documentation of the 12 # deprecated API in order to know how to port your code away from it. 13 DEFINES += QT_DEPRECATED_WARNINGS 14 15 # You can also make your code fail to compile if it uses deprecated APIs. 16 # In order to do so, uncomment the following line. 17 # You can also select to disable deprecated APIs only up to a certain version of Qt. 18 #DEFINES += QT_DISABLE_DEPRECATED_BEFORE=0x060000 # disables all the APIs deprecated before Qt 6.0.0 19 20 SOURCES += \ 21 main.cpp \ 22 mainwindow.cpp 23 24 HEADERS += \ 25 mainwindow.h 26 27 FORMS += \ 28 mainwindow.ui 29 30 # Default rules for deployment. 31 qnx: target.path = /tmp/$${TARGET}/bin 32 else: unix:!android: target.path = /opt/$${TARGET}/bin 33 !isEmpty(target.path): INSTALLS += target
main.cpp

1 #include "mainwindow.h" 2 3 #include <QApplication> 4 5 int main(int argc, char *argv[]) 6 { 7 QApplication a(argc, argv); 8 MainWindow w; 9 w.show(); 10 return a.exec(); 11 }
mainwindow.h

1 #ifndef MAINWINDOW_H 2 #define MAINWINDOW_H 3 4 #include <QMainWindow> 5 #include <QDebug> 6 #include <QMessageBox> 7 #include "QAxWidget" 8 #include "QDesktopServices" 9 #include "QUrl" 10 11 QT_BEGIN_NAMESPACE 12 namespace Ui { class MainWindow; } 13 QT_END_NAMESPACE 14 15 class MainWindow : public QMainWindow 16 { 17 Q_OBJECT 18 19 public: 20 MainWindow(QWidget *parent = nullptr); 21 ~MainWindow(); 22 23 private slots: 24 void on_pushButton_4_clicked(); 25 26 void on_pushButton_3_clicked(); 27 28 void on_pushButton_2_clicked(); 29 30 void on_pushButton_clicked(); 31 32 void on_pushButton_5_clicked(); 33 34 void on_pushButton_6_clicked(); 35 36 void on_pushButton_7_clicked(); 37 38 void on_pushButton_8_clicked(); 39 40 void on_pushButton_9_clicked(); 41 42 private: 43 Ui::MainWindow *ui; 44 QAxWidget* m_pOfficeContent; 45 }; 46 #endif // MAINWINDOW_H
mainwindow.cpp

1 #include "mainwindow.h" 2 #include "ui_mainwindow.h" 3 4 MainWindow::MainWindow(QWidget *parent) 5 : QMainWindow(parent) 6 , ui(new Ui::MainWindow) 7 { 8 ui->setupUi(this); 9 10 setWindowTitle(QStringLiteral("Qt之使用Activate控件")); 11 12 m_pOfficeContent = new QAxWidget(ui->centralwidget); 13 m_pOfficeContent->setGeometry(0, 0, 1000, 1000); 14 ui->horizontalLayout_2->addWidget(m_pOfficeContent); 15 16 17 18 // 加载PDF(说的是安装Adobe PDF Reader就可以,但是我电脑上安装后也不行,加载为灰色背景) 19 // QString filename = "E:\\Gitee\\ZJQQtDemo\\src\\QtPDF\\A.pdf"; 20 // if(!officeContent->setControl("Adobe PDF Reader")) 21 // QMessageBox::critical(this, "Error", QStringLiteral("没有安装pdf!")); 22 // officeContent->dynamicCall("LoadFile(const QString&)", filename); 23 // officeContent->dynamicCall("SetVisible (bool Visible)", "true"); 24 // officeContent->setProperty("DisplayAlerts", true); 25 // officeContent->setProperty("DisplayScrollBars", true); // 显示滚动条 26 27 28 29 } 30 31 MainWindow::~MainWindow() 32 { 33 34 delete ui; 35 } 36 37 38 void MainWindow::on_pushButton_4_clicked() 39 { 40 m_pOfficeContent->setControl("E:\\Gitee\\ZJQQtDemo\\src\\QtPDF\\C.doc"); 41 } 42 43 void MainWindow::on_pushButton_3_clicked() 44 { 45 m_pOfficeContent->setControl("E:\\Gitee\\ZJQQtDemo\\src\\QtPDF\\B.docx"); 46 } 47 48 void MainWindow::on_pushButton_2_clicked() 49 { 50 m_pOfficeContent->setControl("E:\\Gitee\\ZJQQtDemo\\src\\QtPDF\\D.xlsx"); 51 } 52 53 void MainWindow::on_pushButton_clicked() 54 { 55 // 加载网页(百度) Microsoft Web Browser 56 m_pOfficeContent->setControl(QString::fromUtf8("{8856F961-340A-11D0-A96B-00C04FD705A2}")); 57 m_pOfficeContent->setObjectName(QString::fromUtf8("officeContent"));//设置控件的名称 58 m_pOfficeContent->setFocusPolicy(Qt::StrongFocus);//设置控件接收键盘焦点的方式:鼠标单击、Tab键 59 m_pOfficeContent->setProperty("DisplayAlerts",false); //不显示任何警告信息。 60 m_pOfficeContent->setProperty("DisplayScrollBars",true); // 显示滚动条 61 m_pOfficeContent->show(); 62 m_pOfficeContent->dynamicCall("Navigate(const QString&)", "https://www.baidu.com/"); 63 } 64 65 void MainWindow::on_pushButton_5_clicked() 66 { 67 // 远程桌面(没反映) 68 m_pOfficeContent->setControl(QString::fromUtf8("{8b918b82-7985-4c24-89df-c33ad2bbfbcd}")); 69 m_pOfficeContent->setProperty("Server","192.168.31.158"); // 远程连接 IP 70 m_pOfficeContent->setProperty("UserName", "administrator"); // 用户名 71 m_pOfficeContent->setProperty("ClearTextPassword", "123456"); // 用户密码 72 m_pOfficeContent->setProperty("DesktopWidth", 800); // 指定宽度 73 m_pOfficeContent->setProperty("DesktopHeight", 600); // 指定高度 74 m_pOfficeContent->dynamicCall("Connect()"); // 连接 75 } 76 77 void MainWindow::on_pushButton_6_clicked() 78 { 79 // 动画 80 QString swfPath = "E:\\Gitee\\ZJQQtDemo\\src\\QtPDF\\E.swf"; 81 m_pOfficeContent->setControl(QString::fromUtf8("{d27cdb6e-ae6d-11cf-96b8-444553540000}"));//设置此属性会初始化COM对象。 先前设置的任何COM对象都将关闭。 82 m_pOfficeContent->dynamicCall("LoadMovie(long,string)", 0, swfPath); 83 } 84 85 void MainWindow::on_pushButton_7_clicked() 86 { 87 // 加载PDF(完美加载) 88 QString filename = "E:\\Gitee\\ZJQQtDemo\\src\\QtPDF\\A.pdf"; 89 m_pOfficeContent->setControl("Microsoft Web Browser"); 90 m_pOfficeContent->dynamicCall("Navigate(const QString&)", filename); 91 } 92 93 void MainWindow::on_pushButton_8_clicked() 94 { 95 QString filename = "E:\\Gitee\\ZJQQtDemo\\src\\QtPDF\\A.pdf"; 96 QDesktopServices::openUrl(QUrl::fromLocalFile(filename )); 97 } 98 99 void MainWindow::on_pushButton_9_clicked() 100 { 101 m_pOfficeContent->setControl(QString::fromUtf8("{8856F961-340A-11D0-A96B-00C04FD705A2}")); 102 m_pOfficeContent->setObjectName(QString::fromUtf8("officeContent"));//设置控件的名称 103 m_pOfficeContent->setFocusPolicy(Qt::StrongFocus);//设置控件接收键盘焦点的方式:鼠标单击、Tab键 104 m_pOfficeContent->setProperty("DisplayAlerts",false); //不显示任何警告信息。 105 m_pOfficeContent->setProperty("DisplayScrollBars",true); // 显示滚动条 106 m_pOfficeContent->show(); 107 QString sUrl = "https://images.cnblogs.com/cnblogs_com/FKdelphi/1786386/o_200716061815Qt%E8%AE%BE%E7%BD%AE%E8%83%8C%E6%99%AF%E5%9B%BE%E7%89%87.png"; 108 m_pOfficeContent->dynamicCall("Navigate(const QString&)",sUrl); 109 }
mainwindow.ui

1 <?xml version="1.0" encoding="UTF-8"?> 2 <ui version="4.0"> 3 <class>MainWindow</class> 4 <widget class="QMainWindow" name="MainWindow"> 5 <property name="geometry"> 6 <rect> 7 <x>0</x> 8 <y>0</y> 9 <width>847</width> 10 <height>403</height> 11 </rect> 12 </property> 13 <property name="windowTitle"> 14 <string>MainWindow</string> 15 </property> 16 <widget class="QWidget" name="centralwidget"> 17 <layout class="QHBoxLayout" name="horizontalLayout_6"> 18 <item> 19 <layout class="QHBoxLayout" name="horizontalLayout_2"> 20 <item> 21 <layout class="QHBoxLayout" name="horizontalLayout_5"> 22 <item> 23 <layout class="QVBoxLayout" name="verticalLayout"> 24 <item> 25 <layout class="QHBoxLayout" name="horizontalLayout_4"> 26 <item> 27 <widget class="QPushButton" name="pushButton_4"> 28 <property name="text"> 29 <string>加载DOC</string> 30 </property> 31 <property name="checkable"> 32 <bool>false</bool> 33 </property> 34 <property name="autoRepeatInterval"> 35 <number>100</number> 36 </property> 37 <property name="autoDefault"> 38 <bool>false</bool> 39 </property> 40 </widget> 41 </item> 42 <item> 43 <widget class="QPushButton" name="pushButton_3"> 44 <property name="text"> 45 <string>加载DOCX</string> 46 </property> 47 </widget> 48 </item> 49 <item> 50 <widget class="QPushButton" name="pushButton_2"> 51 <property name="text"> 52 <string>加载xlsx</string> 53 </property> 54 </widget> 55 </item> 56 </layout> 57 </item> 58 <item> 59 <layout class="QHBoxLayout" name="horizontalLayout"> 60 <item> 61 <widget class="QPushButton" name="pushButton_5"> 62 <property name="text"> 63 <string>远程桌</string> 64 </property> 65 </widget> 66 </item> 67 <item> 68 <widget class="QPushButton" name="pushButton"> 69 <property name="text"> 70 <string>加载网页(百度)</string> 71 </property> 72 </widget> 73 </item> 74 <item> 75 <widget class="QPushButton" name="pushButton_9"> 76 <property name="text"> 77 <string>下载网上图片</string> 78 </property> 79 </widget> 80 </item> 81 </layout> 82 </item> 83 <item> 84 <layout class="QHBoxLayout" name="horizontalLayout_3"> 85 <item> 86 <widget class="QPushButton" name="pushButton_8"> 87 <property name="text"> 88 <string>默认程序加载PDF</string> 89 </property> 90 </widget> 91 </item> 92 <item> 93 <widget class="QPushButton" name="pushButton_7"> 94 <property name="text"> 95 <string>本程序内加载PDF</string> 96 </property> 97 </widget> 98 </item> 99 <item> 100 <widget class="QPushButton" name="pushButton_6"> 101 <property name="text"> 102 <string>动画</string> 103 </property> 104 </widget> 105 </item> 106 </layout> 107 </item> 108 </layout> 109 </item> 110 </layout> 111 </item> 112 </layout> 113 </item> 114 </layout> 115 </widget> 116 </widget> 117 <resources/> 118 <connections/> 119 </ui>
作者:疯狂Delphi
本文版权归作者和博客园共有,欢迎转载,但未经作者同意必须保留此段声明,且在文章页面明显位置给出原文连接,否则保留追究法律责任的权利.
欢迎关注我,一起进步!扫描下方二维码即可加我
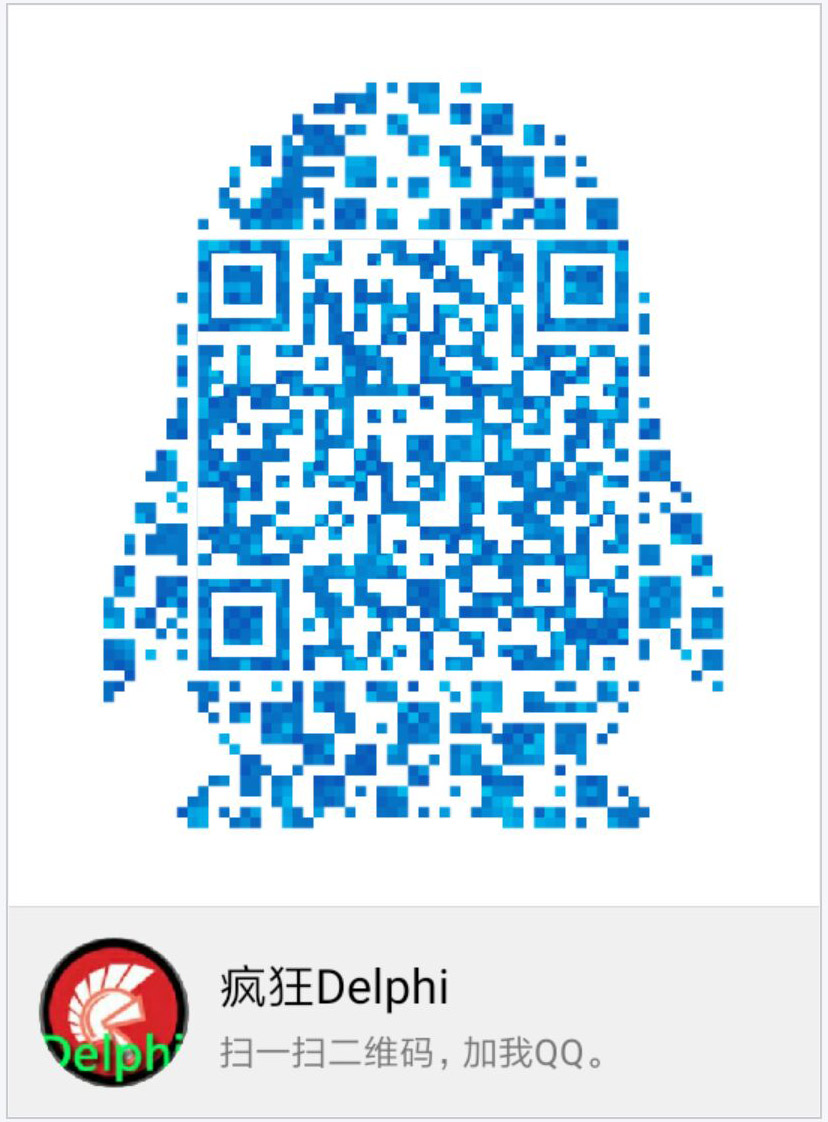
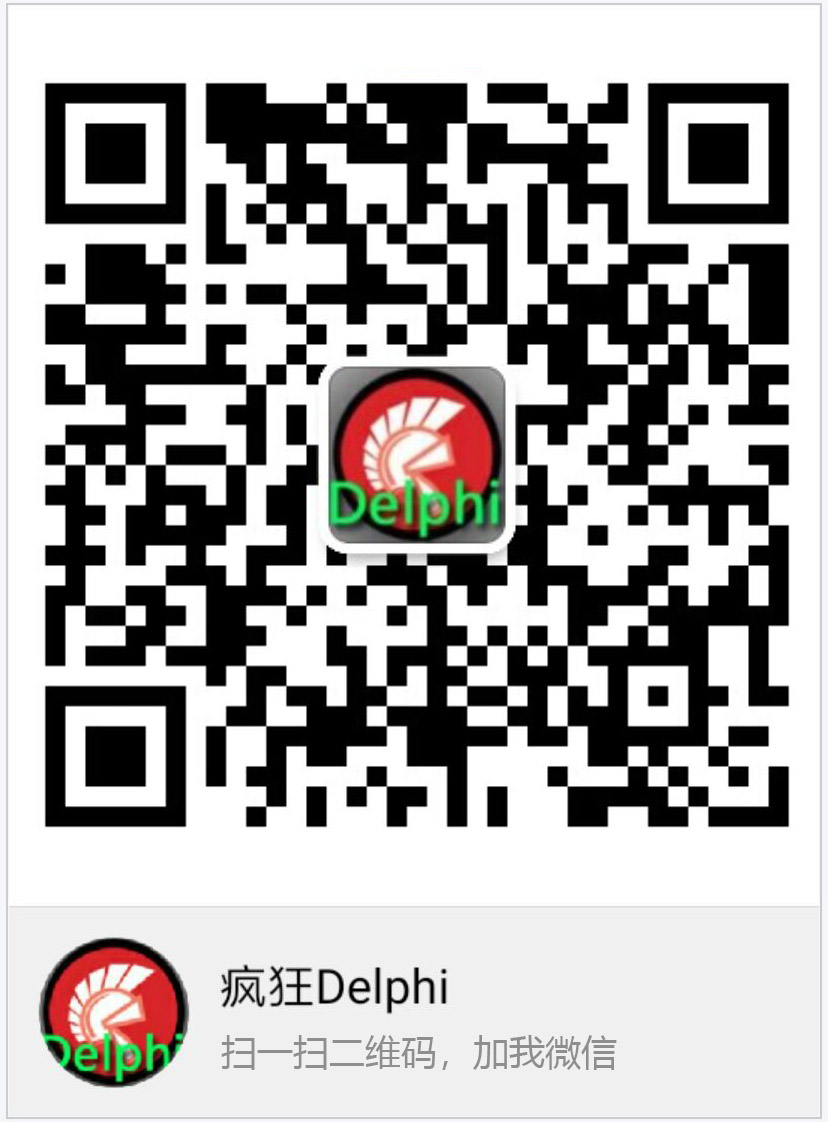