Qt-Qt之QSS文件使用
相关资料
https://blog.csdn.net/github_55295425/article/details/126091163 原文地址
https://download.csdn.net/download/zhujianqiangqq/86663074 CSDN代码包下载
实例代码
.pro

1 QT += core gui 2 3 greaterThan(QT_MAJOR_VERSION, 4): QT += widgets 4 5 CONFIG += c++11 6 7 # The following define makes your compiler emit warnings if you use 8 # any Qt feature that has been marked deprecated (the exact warnings 9 # depend on your compiler). Please consult the documentation of the 10 # deprecated API in order to know how to port your code away from it. 11 DEFINES += QT_DEPRECATED_WARNINGS 12 13 # You can also make your code fail to compile if it uses deprecated APIs. 14 # In order to do so, uncomment the following line. 15 # You can also select to disable deprecated APIs only up to a certain version of Qt. 16 #DEFINES += QT_DISABLE_DEPRECATED_BEFORE=0x060000 # disables all the APIs deprecated before Qt 6.0.0 17 18 SOURCES += \ 19 main.cpp \ 20 mainwindow.cpp 21 22 HEADERS += \ 23 mainwindow.h 24 25 FORMS += \ 26 mainwindow.ui 27 28 # Default rules for deployment. 29 qnx: target.path = /tmp/$${TARGET}/bin 30 else: unix:!android: target.path = /opt/$${TARGET}/bin 31 !isEmpty(target.path): INSTALLS += target 32 33 RESOURCES += \ 34 Resource.qrc
main.cpp

1 #include "mainwindow.h" 2 3 #include <QApplication> 4 #include <QFile>// 引入 5 #include <QTextStream>// 引入 6 7 int main(int argc, char *argv[]) 8 { 9 QApplication a(argc, argv); 10 // ***********QSS文件开始********* 11 QFile file(":/QSS/myQSS.qss"); 12 QString styleSheet; 13 if (file.open(QIODevice::ReadOnly)) 14 { 15 16 styleSheet = QLatin1String(file.readAll()); 17 a.setStyleSheet(styleSheet); 18 } 19 file.close(); 20 // ***********QSS文件结束********* 21 MainWindow w; 22 w.show(); 23 return a.exec(); 24 }
mainwindow.h

1 #ifndef MAINWINDOW_H 2 #define MAINWINDOW_H 3 4 #include <QMainWindow> 5 6 QT_BEGIN_NAMESPACE 7 namespace Ui { class MainWindow; } 8 QT_END_NAMESPACE 9 10 class MainWindow : public QMainWindow 11 { 12 Q_OBJECT 13 14 public: 15 MainWindow(QWidget *parent = nullptr); 16 ~MainWindow(); 17 18 private: 19 Ui::MainWindow *ui; 20 }; 21 #endif // MAINWINDOW_H
mainwindow.cpp

1 #include "mainwindow.h" 2 #include "ui_mainwindow.h" 3 4 #include <QListView>// 引入 5 6 MainWindow::MainWindow(QWidget *parent) 7 : QMainWindow(parent) 8 , ui(new Ui::MainWindow) 9 { 10 ui->setupUi(this); 11 12 setWindowTitle(QStringLiteral("Qt之QSS文件使用")); 13 14 ui->comboBox->setView(new QListView()); 15 ui->comboBox->addItem("a"); 16 ui->comboBox->addItem("a"); 17 ui->comboBox->addItem("a"); 18 ui->comboBox->addItem("a"); 19 } 20 21 MainWindow::~MainWindow() 22 { 23 delete ui; 24 }
mainwindow.ui

1 <?xml version="1.0" encoding="UTF-8"?> 2 <ui version="4.0"> 3 <class>MainWindow</class> 4 <widget class="QMainWindow" name="MainWindow"> 5 <property name="geometry"> 6 <rect> 7 <x>0</x> 8 <y>0</y> 9 <width>593</width> 10 <height>357</height> 11 </rect> 12 </property> 13 <property name="windowTitle"> 14 <string>MainWindow</string> 15 </property> 16 <widget class="QWidget" name="centralwidget"> 17 <layout class="QHBoxLayout" name="horizontalLayout"> 18 <item> 19 <layout class="QVBoxLayout" name="verticalLayout"> 20 <item> 21 <layout class="QHBoxLayout" name="horizontalLayout_4"> 22 <item> 23 <widget class="QPushButton" name="pushButton"> 24 <property name="text"> 25 <string>PushButton</string> 26 </property> 27 </widget> 28 </item> 29 <item> 30 <widget class="QLineEdit" name="lineEdit"/> 31 </item> 32 <item> 33 <widget class="QPushButton" name="SP_dir_Button"> 34 <property name="text"> 35 <string>PushButton</string> 36 </property> 37 </widget> 38 </item> 39 </layout> 40 </item> 41 <item> 42 <layout class="QHBoxLayout" name="horizontalLayout_2"> 43 <item> 44 <widget class="QLabel" name="label"> 45 <property name="text"> 46 <string>TextLabel</string> 47 </property> 48 </widget> 49 </item> 50 <item> 51 <widget class="QLineEdit" name="lineEdit_2"/> 52 </item> 53 <item> 54 <widget class="QPushButton" name="pushButton_2"> 55 <property name="text"> 56 <string>PushButton</string> 57 </property> 58 </widget> 59 </item> 60 </layout> 61 </item> 62 <item> 63 <layout class="QHBoxLayout" name="horizontalLayout_3"> 64 <item> 65 <widget class="QComboBox" name="comboBox"/> 66 </item> 67 </layout> 68 </item> 69 <item> 70 <layout class="QHBoxLayout" name="horizontalLayout_5"> 71 <item> 72 <widget class="QSlider" name="horizontalSlider"> 73 <property name="orientation"> 74 <enum>Qt::Horizontal</enum> 75 </property> 76 </widget> 77 </item> 78 <item> 79 <widget class="QSlider" name="verticalSlider"> 80 <property name="orientation"> 81 <enum>Qt::Vertical</enum> 82 </property> 83 </widget> 84 </item> 85 </layout> 86 </item> 87 </layout> 88 </item> 89 </layout> 90 </widget> 91 </widget> 92 <resources/> 93 <connections/> 94 </ui>
myQSS.qss

1 /*qss文件的书写格式类似css*/ 2 3 /*控件类名{样式声明;}*/ 4 /*设置整个Widget样式*/ 5 QWidget{ 6 background-color: rgb(255,255,255); 7 } 8 /*设置界面中所有QPushButton样式*/ 9 QPushButton{ 10 font: 25 14pt "微软雅黑 Light"; 11 color: rgb(255,255,255); 12 background-color: rgb(80,92,102); 13 border: none; 14 border-radius:8px; 15 } 16 17 /*设置控件处于某状态下(鼠标悬停/点击)的样式*/ 18 /*控件类名:控件状态{样式声明;}*/ 19 /*hover样式*/ 20 QPushButton:hover{ 21 background-color: rgb(95,107,117); 22 } 23 /*pressed样式*/ 24 QPushButton:pressed{ 25 background-color: rgb(54,54,54); 26 } 27 28 /*设定控件类的子控件样式*/ 29 /*设置QcomboBox的子控件下拉框样式*/ 30 QComboBox QAbstractItemView::item{ 31 height:40px; 32 } 33 /*注意:此处QcomboBox的子控件下拉框,无法直接被qss文件设定样式,需要在构造函数中设定如下语句:*/ 34 /*ui->filetype_choose_Box->setView(new QListView()); 之后qss样式就可以使用了*/ 35 36 /*设定控件类的子控件某种状态下样式*/ 37 /*设置QcomboBox的子控件下拉框hover时的样式*/ 38 QComboBox QAbstractItemView::item:hover{ 39 color: rgb(20,20,20); 40 background-color: rgb(255,209,71); 41 } 42 43 /*设定具体控件对象的样式*/ 44 /*控件类名#具体的对象名{样式声明;}*/ 45 /*设置SP_dir_Button控件的样式*/ 46 QPushButton#SP_dir_Button{ 47 font:75 13pt "微软雅黑"; 48 color: rgb(20,20,20); 49 background-color: rgba(255,209,71,0); 50 border:2px solid rgb(20,20,20); 51 } 52 QPushButton#SP_dir_Button:hover{ 53 color: rgb(255,255,255); 54 background-color: rgb(255,172,0); 55 border: none; 56 } 57 QPushButton#SP_dir_Button:pressed{ 58 color: rgb(20,20,20); 59 background-color: rgb(222,222,222); 60 } 61 62 /*QLineEdit设置显示图标*/ 63 QLineEdit 64 { 65 background: #f3f3f3; 66 background-image: url(:Images/search.svg); /* actual size, e.g. 16x16 */ 67 background-repeat: no-repeat; 68 background-position: left; 69 color: #252424; 70 font-family: SegoeUI; 71 font-size: 12px; 72 padding: 2 2 2 20; /* left padding (last number) must be more than the icon's width */ 73 border: 1px solid #dddddd; 74 } 75 76 /*QSlider滑块*/ 77 QSlider::groove:horizontal {border: 1px solid #4A708B;background: #C0C0C0;height: 5px;border-radius: 1px;padding-left:-1px;padding-right:-1px;} 78 79 QSlider::sub-page:horizontal { 80 background: qlineargradient(x1:0, y1:0, x2:0, y2:1, 81 stop:0 #B1B1B1, stop:1 #c4c4c4); 82 background: qlineargradient(x1: 0, y1: 0.2, x2: 1, y2: 1, 83 stop: 0 #5DCCFF, stop: 1 #1874CD); 84 border: 1px solid #4A708B; 85 height: 10px; 86 border-radius: 2px; 87 } 88 89 QSlider::add-page:horizontal {background: #575757;border: 0px solid #777;height: 10px;border-radius: 2px;} 90 91 QSlider::handle:horizontal 92 { 93 background: qradialgradient(spread:pad, cx:0.5, cy:0.5, radius:0.5, fx:0.5, fy:0.5, 94 stop:0.6 #45ADED, stop:0.778409 rgba(255, 255, 255, 255)); 95 96 width: 21px;margin-top: -3px;margin-bottom: -3px;border-radius: 5px; 97 } 98 99 QSlider::handle:horizontal:hover { 100 background: qradialgradient(spread:pad, cx:0.5, cy:0.5, radius:0.5, fx:0.5, fy:0.5, stop:0.6 #2A8BDA, 101 stop:0.778409 rgba(255, 255, 255, 255)); 102 width: 21px;margin-top: -3px;margin-bottom: -3px;border-radius: 5px; 103 } 104 105 QSlider::sub-page:horizontal:disabled {background: #808080;border-color: #999;} 106 107 QSlider::add-page:horizontal:disabled {background: #eee;border-color: #999;} 108 109 QSlider::handle:horizontal:disabled {background: #eee;border: 1px solid #aaa;border-radius: 4px;}
QSS类型选择器
通用选择器 * 匹配所有部件
类型选择器 QPushButton 匹配QPushButton及其子类的实例
属性选择器 QPushButton[flat="false"] 匹配QPushButton中flat属性为false的实例
类选择器 .QPushButton 匹配QPushButton的实例,但不包含子类。相当于* [class~="QPushButton"]
ID选择器 QPushButton#okButton 匹配所有objectName为okButton的QPushButton实例
后代选择器 QDialog QPushButton 匹配属于QDialog后代(孩子,孙子等)的QPushButton所有 实例
子选择器 QDialog > QPushButton 匹配属于QDialog直接子类的QPushButton所有实例
QSS文件的代码块高亮显示
默认 QtCreator 没有qss代码高亮提示,可以在设置中添加如下设置:
"工具"->"选项"->"环境"->"MIMF"类型
已注册的MIME类型输入框中输入:"text"->"查找"
MIME类型中找到 "text/css"
在下方"详情"->"模式"->追加";*qss"
无法加载QSS
1.文件路径不对
2.输入的格式不对
3.文件编码不对,如果有中文需要使用“UTF-8无BOM格式编码”,如果文件是“UTF-8格式编码”将无法加载。
欢迎关注我,一起进步!扫描下方二维码即可加我
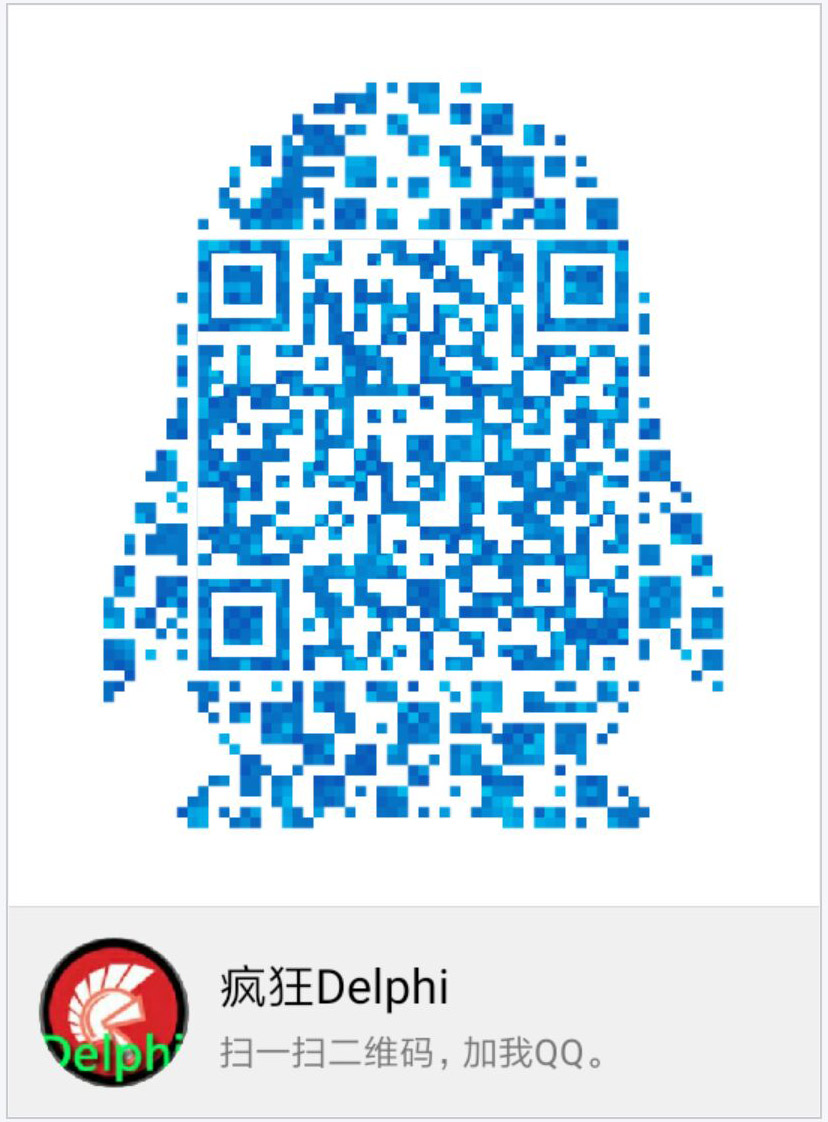
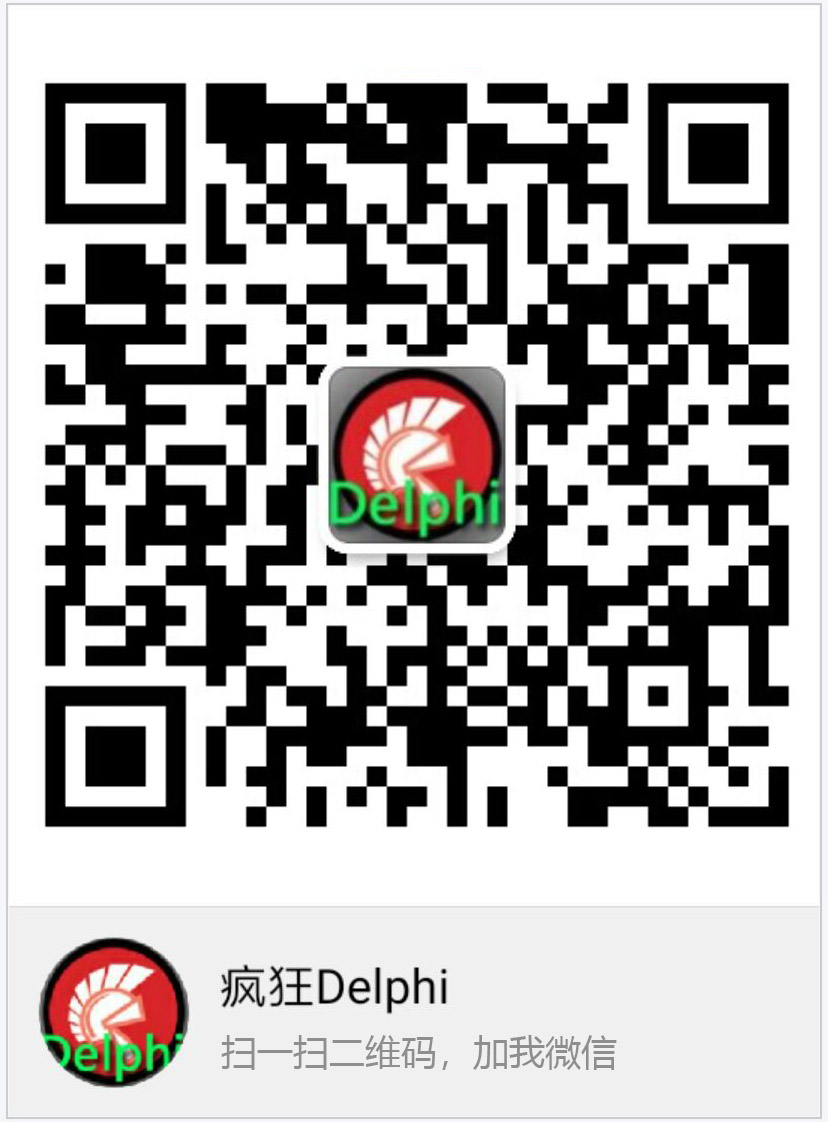