Qt-Qt之QDesktopWidget的使用说明(屏幕个数、屏幕分辩率、屏幕PDI、设置屏幕分辩率)
相关资料:
https://download.csdn.net/download/zhujianqiangqq/86539703 CSDN代码包下载
https://blog.csdn.net/Superman___007/article/details/109450184
https://blog.csdn.net/chenyijun/article/details/104749632/
实例代码:
.pro

1 QT += core gui 2 3 greaterThan(QT_MAJOR_VERSION, 4): QT += widgets 4 5 CONFIG += c++11 6 7 # The following define makes your compiler emit warnings if you use 8 # any Qt feature that has been marked deprecated (the exact warnings 9 # depend on your compiler). Please consult the documentation of the 10 # deprecated API in order to know how to port your code away from it. 11 DEFINES += QT_DEPRECATED_WARNINGS 12 13 # You can also make your code fail to compile if it uses deprecated APIs. 14 # In order to do so, uncomment the following line. 15 # You can also select to disable deprecated APIs only up to a certain version of Qt. 16 #DEFINES += QT_DISABLE_DEPRECATED_BEFORE=0x060000 # disables all the APIs deprecated before Qt 6.0.0 17 18 SOURCES += \ 19 main.cpp \ 20 mainwindow.cpp 21 22 HEADERS += \ 23 mainwindow.h 24 25 FORMS += \ 26 mainwindow.ui 27 28 LIBS += -luser32 29 LIBS += -lgdi32 30 31 # Default rules for deployment. 32 qnx: target.path = /tmp/$${TARGET}/bin 33 else: unix:!android: target.path = /opt/$${TARGET}/bin 34 !isEmpty(target.path): INSTALLS += target
main.cpp

1 #include "mainwindow.h" 2 3 #include <QApplication> 4 5 int main(int argc, char *argv[]) 6 { 7 QApplication a(argc, argv); 8 MainWindow w; 9 w.show(); 10 return a.exec(); 11 }
mainwindow.h

1 // 相关资料: 2 // https://blog.csdn.net/Superman___007/article/details/109450184 3 // https://blog.csdn.net/chenyijun/article/details/104749632/ 4 5 #ifndef MAINWINDOW_H 6 #define MAINWINDOW_H 7 8 #include <Windows.h>//这个一定要添加,不然会报"No Target Architecture" 错误 9 #include <WinUser.h> 10 #include <wingdi.h> 11 12 #include <QMainWindow> 13 // 桌面对象引入 14 #include <QDesktopWidget> 15 #include <QDebug> 16 #include <QApplication> 17 18 QT_BEGIN_NAMESPACE 19 namespace Ui { class MainWindow; } 20 QT_END_NAMESPACE 21 22 class MainWindow : public QMainWindow 23 { 24 Q_OBJECT 25 26 public: 27 MainWindow(QWidget *parent = nullptr); 28 ~MainWindow(); 29 30 private slots: 31 void on_pushButton_4_clicked(); 32 33 void on_pushButton_3_clicked(); 34 35 void on_pushButton_2_clicked(); 36 37 void on_pushButton_clicked(); 38 39 void on_pushButton_5_clicked(); 40 41 void on_pushButton_7_clicked(); 42 43 void on_pushButton_6_clicked(); 44 45 void on_pushButton_8_clicked(); 46 47 private: 48 int GetTaskbarInfo(); 49 void GetTaskbarInfo(int &width, int &height, int &pos); 50 Ui::MainWindow *ui; 51 // 桌面对象 52 QDesktopWidget *m_pDeskdop; 53 54 }; 55 #endif // MAINWINDOW_H
mainwindow.cpp

1 #include "mainwindow.h" 2 #include "ui_mainwindow.h" 3 4 MainWindow::MainWindow(QWidget *parent) 5 : QMainWindow(parent) 6 , ui(new Ui::MainWindow) 7 { 8 ui->setupUi(this); 9 // 设置标题 10 setWindowTitle(QStringLiteral("Qt之QDesktopWidget的使用说明(屏幕个数、屏幕分辩率、屏幕PDI、设置屏幕分辩率)")); 11 // 设置界面大小 12 resize(600, 300); 13 // 为了测试,先把界面放在左100,顶200位置上。 14 move(100, 200); 15 // 桌面操作作 16 m_pDeskdop = QApplication::desktop(); 17 move((m_pDeskdop->width() - this->width())/2, (m_pDeskdop->height() - this->height())/2); 18 } 19 20 MainWindow::~MainWindow() 21 { 22 delete ui; 23 } 24 25 void MainWindow::on_pushButton_4_clicked() 26 { 27 ui->textEdit->append(QString::number(m_pDeskdop->screenCount())); 28 } 29 30 void MainWindow::on_pushButton_3_clicked() 31 { 32 ui->textEdit->append(QString::number(m_pDeskdop->numScreens())); 33 } 34 35 void MainWindow::on_pushButton_2_clicked() 36 { 37 ui->textEdit->append(QString::number(m_pDeskdop->screenNumber())); 38 } 39 40 void MainWindow::on_pushButton_clicked() 41 { 42 ui->textEdit->append(QString::number(m_pDeskdop->primaryScreen())); 43 } 44 45 void MainWindow::on_pushButton_5_clicked() 46 { 47 ui->textEdit->append(QString::number(m_pDeskdop->screen()->width())); 48 ui->textEdit->append(QString::number(m_pDeskdop->screen()->height())); 49 } 50 51 int MainWindow::GetTaskbarInfo() 52 { 53 QRect deskRect = m_pDeskdop->availableGeometry(); 54 QRect screenRect = m_pDeskdop->screenGeometry(); 55 56 int nLocation = 1; 57 if (deskRect.bottom() != screenRect.bottom()) //任务栏在下 58 { 59 nLocation = 1; 60 } 61 else if (deskRect.top() != screenRect.top()) //任务栏在上 62 { 63 nLocation = 2; 64 } 65 else if (deskRect.left() != screenRect.left()) //任务栏在左 66 { 67 nLocation = 3; 68 } 69 else if (deskRect.right() != screenRect.right()) //任务栏在右 70 { 71 nLocation = 4; 72 } 73 else //任务栏处于自动隐藏状态 74 { 75 nLocation = 5; 76 } 77 78 return nLocation; 79 } 80 81 void MainWindow::GetTaskbarInfo(int &width, int &height, int &pos) 82 { 83 // 得到被定义的系统数据或者系统配置信息 84 int wx = GetSystemMetrics(SM_CXSCREEN);// 主显示器屏幕的宽度,以像素为单位。 这是通过调用GetDeviceCaps获得的相同值 85 int wy = GetSystemMetrics(SM_CYSCREEN);// 主显示器屏幕的高度,以像素为单位。 86 87 RECT rtWorkArea; 88 // 函数查询或设置系统级参数 89 SystemParametersInfo(SPI_GETWORKAREA, 0, &rtWorkArea, 0);// 工作区是指屏幕上不被系统任务条或应用程序桌面工具遮盖的部分 90 //1.任务栏停靠在左边情况 91 if (0 != rtWorkArea.left) 92 { 93 width = wx - rtWorkArea.right; 94 height = wy; 95 pos = 0; 96 return; 97 } 98 //2.任务栏停靠在上边情况 99 if(0!=rtWorkArea.top) 100 { 101 width = wx; 102 height = wy - rtWorkArea.bottom; 103 pos = 1; 104 return; 105 } 106 //3.任务栏停靠在右边情况 107 if(0==rtWorkArea.left && wx!=rtWorkArea.right) 108 { 109 width = wx - rtWorkArea.right; 110 height = wy; 111 pos = 2; 112 return; 113 } 114 //4.任务栏停靠在下边情况 115 if(0==rtWorkArea.top && wy!=rtWorkArea.bottom) 116 { 117 width = wx; 118 height = wy - rtWorkArea.bottom; 119 pos = 3; 120 return; 121 } 122 //5.任务栏自动隐藏的情况,这样其宽高都是0 123 if(0==rtWorkArea.left && 0==rtWorkArea.top 124 && wx==rtWorkArea.right && wy==rtWorkArea.bottom) 125 { 126 width = 0; 127 height =0; 128 pos = 4; 129 return; 130 } 131 } 132 133 void MainWindow::on_pushButton_7_clicked() 134 { 135 ui->textEdit->append(QString::number(GetTaskbarInfo())); 136 } 137 138 void MainWindow::on_pushButton_6_clicked() 139 { 140 int w, h, pos; 141 GetTaskbarInfo(w, h, pos); 142 ui->textEdit->append(QStringLiteral("任务栏 宽:%1 高:%2 位置:%3").arg(w).arg(h).arg(pos)); 143 } 144 145 void MainWindow::on_pushButton_8_clicked() 146 { 147 int nScreenWidth = ::GetSystemMetrics(SM_CXSCREEN); 148 int nScreenHeight = ::GetSystemMetrics(SM_CYSCREEN); 149 150 HWND hwd = ::GetDesktopWindow(); 151 HDC hdc = ::GetDC(hwd); 152 int width = GetDeviceCaps(hdc, DESKTOPHORZRES); 153 int height = GetDeviceCaps(hdc, DESKTOPVERTRES); 154 155 double dWidth = (double)width; 156 double dScreenWidth = (double)nScreenWidth; 157 double scale = dWidth / dScreenWidth; 158 159 ui->textEdit->append(QStringLiteral("nScreenWidth:%1 nScreenHeight:%2 width:%3 height:%4 scale:%5") 160 .arg(nScreenWidth).arg(nScreenHeight) 161 .arg(width).arg(height) 162 .arg(scale)); 163 }
mainwindow.ui

1 <?xml version="1.0" encoding="UTF-8"?> 2 <ui version="4.0"> 3 <class>MainWindow</class> 4 <widget class="QMainWindow" name="MainWindow"> 5 <property name="geometry"> 6 <rect> 7 <x>0</x> 8 <y>0</y> 9 <width>728</width> 10 <height>374</height> 11 </rect> 12 </property> 13 <property name="windowTitle"> 14 <string>MainWindow</string> 15 </property> 16 <widget class="QWidget" name="centralwidget"> 17 <layout class="QHBoxLayout" name="horizontalLayout"> 18 <item> 19 <layout class="QVBoxLayout" name="verticalLayout"> 20 <item> 21 <widget class="QTextEdit" name="textEdit"/> 22 </item> 23 <item> 24 <layout class="QHBoxLayout" name="horizontalLayout_2"> 25 <item> 26 <widget class="QPushButton" name="pushButton_7"> 27 <property name="text"> 28 <string>获取系统的任务栏位置以及高度</string> 29 </property> 30 </widget> 31 </item> 32 <item> 33 <widget class="QPushButton" name="pushButton_6"> 34 <property name="text"> 35 <string>获取系统任务栏位置宽高信息的方法</string> 36 </property> 37 </widget> 38 </item> 39 <item> 40 <widget class="QPushButton" name="pushButton_8"> 41 <property name="text"> 42 <string>设备的物理分辩率</string> 43 </property> 44 </widget> 45 </item> 46 </layout> 47 </item> 48 <item> 49 <layout class="QHBoxLayout" name="horizontalLayout_3"> 50 <item> 51 <widget class="QPushButton" name="pushButton_4"> 52 <property name="text"> 53 <string>获得屏幕数量</string> 54 </property> 55 </widget> 56 </item> 57 <item> 58 <widget class="QPushButton" name="pushButton_3"> 59 <property name="text"> 60 <string>获得屏幕数量2</string> 61 </property> 62 </widget> 63 </item> 64 <item> 65 <widget class="QPushButton" name="pushButton_2"> 66 <property name="text"> 67 <string>屏幕编号</string> 68 </property> 69 </widget> 70 </item> 71 <item> 72 <widget class="QPushButton" name="pushButton"> 73 <property name="text"> 74 <string>主屏幕编号</string> 75 </property> 76 </widget> 77 </item> 78 <item> 79 <widget class="QPushButton" name="pushButton_5"> 80 <property name="text"> 81 <string>屏幕宽高</string> 82 </property> 83 </widget> 84 </item> 85 </layout> 86 </item> 87 </layout> 88 </item> 89 </layout> 90 </widget> 91 </widget> 92 <resources/> 93 <connections/> 94 </ui>
作者:疯狂Delphi
本文版权归作者和博客园共有,欢迎转载,但未经作者同意必须保留此段声明,且在文章页面明显位置给出原文连接,否则保留追究法律责任的权利.
欢迎关注我,一起进步!扫描下方二维码即可加我
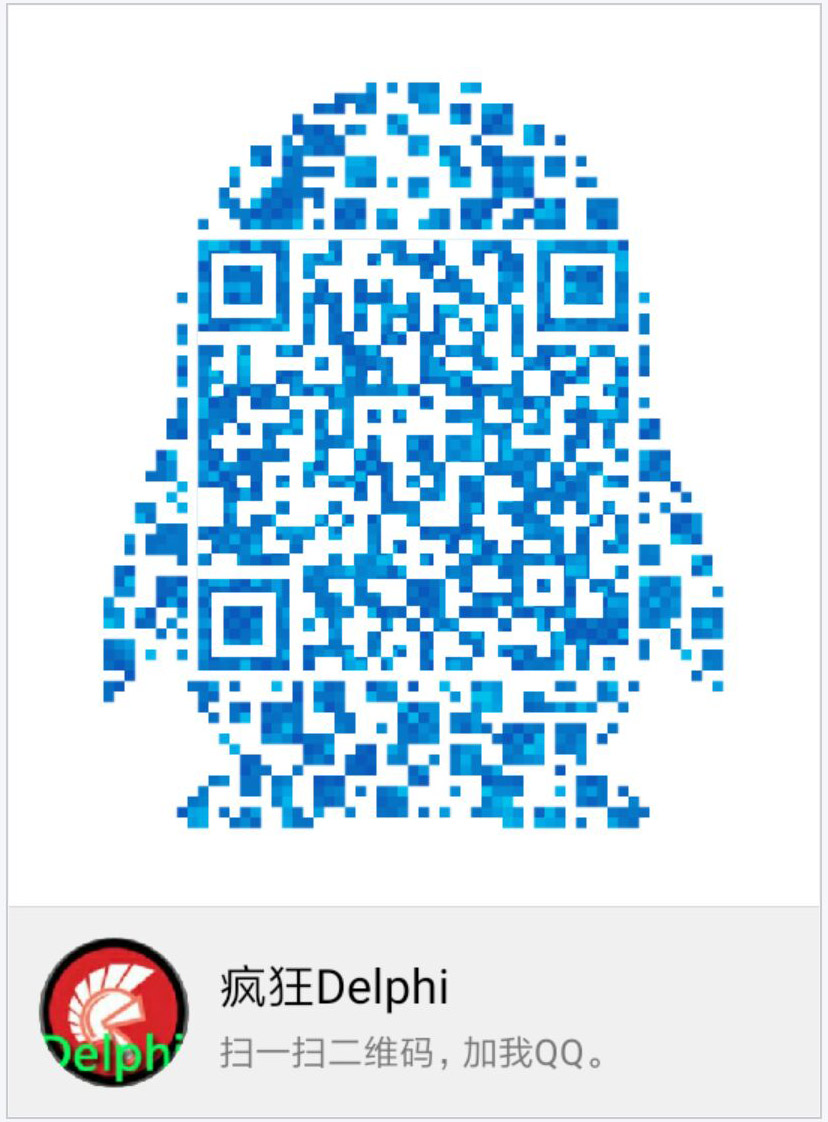
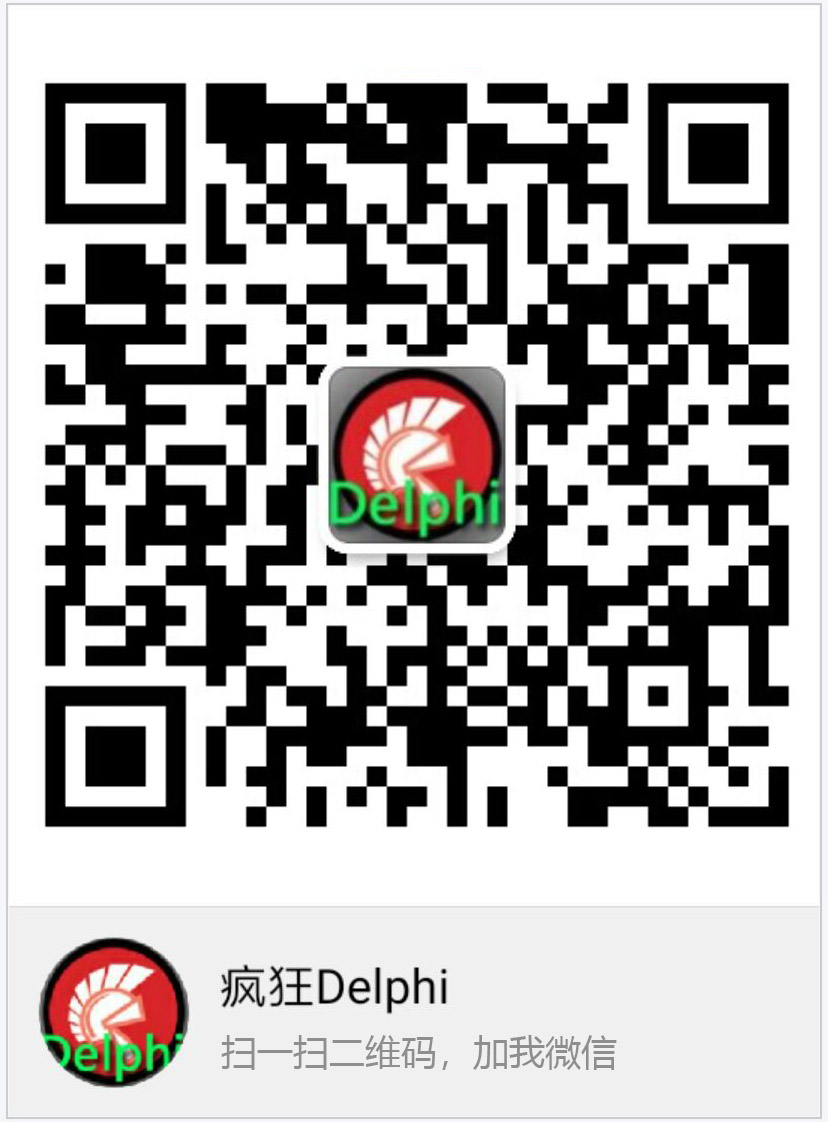