Osg-OsgShader着色器(Qt5.14.2+osgE3.6.5+win10)-No19-OsgShader
.pro

1 QT += core gui widgets 2 TARGET = TestOsgQt 3 TEMPLATE = app 4 DEFINES += QT_DEPRECATED_WARNINGS 5 CONFIG += c++11 6 7 QMAKE_CXXFLAGS_RELEASE = $$QMAKE_CFLAGS_RELEASE_WITH_DEBUGINFO 8 QMAKE_LFLAGS_RELEASE = $$QMAKE_LFLAGS_RELEASE_WITH_DEBUGINFO 9 10 SOURCES += \ 11 main.cpp 12 13 HEADERS += 14 15 OsgDir = D:\\Gitee\\osg365R 16 CONFIG(release, debug|release) { 17 LIBS += -L$${OsgDir}/lib/ -losgDB -losgViewer -lOpenThreads -losgAnimation -losg \ 18 -losgEarth -losgEarthAnnotation -losgEarthFeatures -losgEarthSymbology -losgEarthUtil \ 19 -losgQOpenGL -losgUtil -losgText -losgTerrain -losgSim \ 20 -losgShadow -losgParticle -losgManipulator -losgGA -losgFX \ 21 -losgWidget 22 } else { 23 LIBS += -L$${OsgDir}/debug/lib/ -losgDBd -losgViewerd -lOpenThreadsd -losgAnimationd -losgd \ 24 -losgEarthd -losgEarthAnnotationd -losgEarthFeaturesd -losgEarthSymbologyd -losgEarthUtild \ 25 -losgQOpenGLd -losgUtild -losgTextd -losgTerraind -losgSimd \ 26 -losgShadowd -losgParticled -losgManipulatord -losgGAd -losgFXd \ 27 } 28 29 30 INCLUDEPATH += $${OsgDir}/include 31 DEPENDPATH += $${OsgDir}/include
main.cpp

1 // 原文 2 // https://www.baidu.com/link?url=gbmmOlk8c213ybwxNnnYTwLqvK3vZajPVLLSImrBOG1ovEN1eYnkxoJUkMidHz7efRheoF9g_XQM_Nx5r70hI_&wd=&eqid=b5d5f9fc00012dcf0000000362ce309e 3 4 #include <osg/Node> 5 #include <osg/Geometry> 6 #include <osg/Notify> 7 #include <osg/MatrixTransform> 8 #include <osg/Texture2D> 9 #include <osg/DrawPixels> 10 #include <osg/PolygonOffset> 11 #include <osg/Geode> 12 13 #include <osgDB/Registry> 14 #include <osgDB/ReadFile> 15 16 #include <osgText/Text> 17 18 #include <osgViewer/Viewer> 19 20 #include <osg/ShapeDrawable> 21 22 #ifdef _DEBUG 23 #pragma comment(lib,"osgd.lib") 24 #pragma comment(lib,"osgDBd.lib") 25 #pragma comment(lib,"osgTextd.lib") 26 #pragma comment(lib,"osgViewerd.lib") 27 #endif 28 29 class TextureCoordUpdateCallback : public osg::NodeCallback 30 { 31 public: 32 33 TextureCoordUpdateCallback(double delay = 1.0) : 34 _delay(delay), 35 _prevTime(0.0) 36 { 37 } 38 39 virtual void operator()(osg::Node* node, osg::NodeVisitor* nv) 40 { 41 if (nv->getFrameStamp()) 42 { 43 double currTime = nv->getFrameStamp()->getSimulationTime(); 44 if (currTime - _prevTime > _delay) 45 { 46 osg::Geode* geode = node->asGeode(); 47 osg::Geometry* geom = geode->getDrawable(0)->asGeometry(); 48 // 获取纹理坐标数组 49 osg::Array* tmp = geom->getTexCoordArray(0); 50 osg::Vec2Array* coorArray = (osg::Vec2Array*) tmp; 51 auto it = coorArray->begin(); 52 for (; it < coorArray->end(); it++) 53 { 54 // 动起来 55 it->x() += 0.001; 56 } 57 // 更新纹理坐标数组 58 geom->setTexCoordArray(0, coorArray); 59 60 // record time 61 _prevTime = currTime; 62 } 63 } 64 } 65 66 67 protected: 68 double _delay; 69 double _prevTime; 70 71 }; 72 73 74 osg::Node* createPyramidModel() 75 { 76 // create the root node which will hold the model. 77 osg::Group* root = new osg::Group(); 78 79 // turn off lighting 80 root->getOrCreateStateSet()->setMode(GL_LIGHTING, osg::StateAttribute::OFF); 81 82 osg::Geode* pyramidGeode = new osg::Geode(); 83 osg::Geometry* pyramidGeometry = new osg::Geometry(); 84 pyramidGeode->setUpdateCallback(new TextureCoordUpdateCallback(0.01)); 85 pyramidGeode->setDataVariance(osg::Object::DYNAMIC); 86 pyramidGeode->addDrawable(pyramidGeometry); 87 root->addChild(pyramidGeode); 88 89 osg::Vec3Array* pyramidVertices = new osg::Vec3Array; 90 pyramidVertices->push_back(osg::Vec3(0, 0, 0)); // 左前 91 pyramidVertices->push_back(osg::Vec3(10, 0, 0)); // 右前 92 pyramidVertices->push_back(osg::Vec3(10, 10, 0)); // 右后 93 pyramidVertices->push_back(osg::Vec3(0, 10, 0)); // 左后 94 pyramidVertices->push_back(osg::Vec3(5, 5, 10)); // 塔尖 95 pyramidGeometry->setVertexArray(pyramidVertices); 96 osg::DrawElementsUInt* pyramidBase = new osg::DrawElementsUInt(osg::PrimitiveSet::QUADS, 0); 97 pyramidBase->push_back(3); 98 pyramidBase->push_back(2); 99 pyramidBase->push_back(1); 100 pyramidBase->push_back(0); 101 pyramidGeometry->addPrimitiveSet(pyramidBase); 102 osg::DrawElementsUInt* pyramidFaceOne = new osg::DrawElementsUInt(osg::PrimitiveSet::TRIANGLES, 0); 103 pyramidFaceOne->push_back(0); 104 pyramidFaceOne->push_back(1); 105 pyramidFaceOne->push_back(4); 106 pyramidGeometry->addPrimitiveSet(pyramidFaceOne); 107 osg::DrawElementsUInt* pyramidFaceTwo = new osg::DrawElementsUInt(osg::PrimitiveSet::TRIANGLES, 0); 108 pyramidFaceTwo->push_back(1); 109 pyramidFaceTwo->push_back(2); 110 pyramidFaceTwo->push_back(4); 111 pyramidGeometry->addPrimitiveSet(pyramidFaceTwo); 112 osg::DrawElementsUInt* pyramidFaceThree = new osg::DrawElementsUInt(osg::PrimitiveSet::TRIANGLES, 0); 113 pyramidFaceThree->push_back(2); 114 pyramidFaceThree->push_back(3); 115 pyramidFaceThree->push_back(4); 116 pyramidGeometry->addPrimitiveSet(pyramidFaceThree); 117 osg::DrawElementsUInt* pyramidFaceFour = new osg::DrawElementsUInt(osg::PrimitiveSet::TRIANGLES, 0); 118 pyramidFaceFour->push_back(3); 119 pyramidFaceFour->push_back(0); 120 pyramidFaceFour->push_back(4); 121 pyramidGeometry->addPrimitiveSet(pyramidFaceFour); 122 123 osg::Vec4Array* colors = new osg::Vec4Array; 124 colors->push_back(osg::Vec4(1.0f, 0.0f, 0.0f, 1.0f)); //红色 125 colors->push_back(osg::Vec4(0.0f, 1.0f, 0.0f, 1.0f)); //绿色 126 colors->push_back(osg::Vec4(0.0f, 0.0f, 1.0f, 1.0f)); //蓝色 127 colors->push_back(osg::Vec4(1.0f, 1.0f, 1.0f, 1.0f)); //白色 128 pyramidGeometry->setColorArray(colors); 129 pyramidGeometry->setColorBinding(osg::Geometry::BIND_PER_VERTEX); 130 131 osg::Vec3Array* normals = new osg::Vec3Array(1); 132 (*normals)[0].set(0.0f, -1.0f, 0.0f); 133 pyramidGeometry->setNormalArray(normals, osg::Array::BIND_OVERALL); 134 135 osg::Vec2Array* texcoords = new osg::Vec2Array(5); 136 (*texcoords)[0].set(0.00f, 0.0f); 137 (*texcoords)[1].set(0.25f, 0.0f); 138 (*texcoords)[2].set(0.50f, 0.0f); 139 (*texcoords)[3].set(0.75f, 0.0f); 140 (*texcoords)[4].set(0.50f, 1.0f); 141 pyramidGeometry->setTexCoordArray(0, texcoords); 142 143 // set up the texture state. 144 osg::Texture2D* texture = new osg::Texture2D; 145 texture->setDataVariance(osg::Object::DYNAMIC); // protect from being optimized away as static state. 146 texture->setFilter(osg::Texture2D::MIN_FILTER, osg::Texture2D::LINEAR); 147 texture->setFilter(osg::Texture2D::MAG_FILTER, osg::Texture2D::LINEAR); 148 texture->setWrap(osg::Texture2D::WRAP_S, osg::Texture2D::REPEAT); 149 texture->setWrap(osg::Texture2D::WRAP_T, osg::Texture2D::REPEAT); 150 texture->setImage(osgDB::readImageFile("D:/Gitee/OsgTestQt/src/No19-OsgShader/road.png")); 151 osg::StateSet* stateset = pyramidGeometry->getOrCreateStateSet(); 152 stateset->setTextureAttributeAndModes(0, texture, osg::StateAttribute::ON); 153 154 return root; 155 } 156 157 static const char * fragShader = { 158 "varying vec4 color;\n" 159 "uniform sampler2D baseTex;\n" 160 "uniform int osg_FrameNumber;\n"//当前OSG程序运行的帧数; 161 "uniform float osg_FrameTime;\n"//当前OSG程序的运行总时间; 162 "uniform float osg_DeltaFrameTime;\n"//当前OSG程序运行每帧的间隔时间; 163 "uniform mat4 osg_ViewMatrix;\n"//当前OSG摄像机的观察矩阵; 164 "uniform mat4 osg_ViewMatrixInverse;\n"// 当前OSG摄像机观察矩阵的逆矩阵。 165 "void main(void){\n" 166 "vec2 coord = gl_TexCoord[0].xy+vec2(0,osg_FrameTime*0.8);" 167 " gl_FragColor = texture2D(baseTex, coord);\n" 168 "}\n" 169 }; 170 osg::Node* createCone() 171 { 172 osg::ref_ptr<osg::Geode> geode = new osg::Geode; 173 osg::ref_ptr<osg::Cone> cone = new osg::Cone(osg::Vec3(0, 0, 0), 1.0f, 5); 174 osg::ref_ptr<osg::ShapeDrawable> shapeDrawable = new osg::ShapeDrawable(cone.get()); 175 geode->addDrawable(shapeDrawable.get()); 176 177 // set up the texture state. 178 osg::Texture2D* texture = new osg::Texture2D; 179 //texture->setDataVariance(osg::Object::DYNAMIC); // protect from being optimized away as static state. 180 texture->setFilter(osg::Texture2D::MIN_FILTER, osg::Texture2D::LINEAR); 181 texture->setFilter(osg::Texture2D::MAG_FILTER, osg::Texture2D::LINEAR); 182 texture->setWrap(osg::Texture2D::WRAP_S, osg::Texture2D::REPEAT); 183 texture->setWrap(osg::Texture2D::WRAP_T, osg::Texture2D::REPEAT); 184 texture->setImage(osgDB::readImageFile("D:/Gitee/OsgTestQt/src/No19-OsgShader/J20.jpg")); 185 186 osg::StateSet* stateset = shapeDrawable->getOrCreateStateSet(); 187 stateset->setTextureAttributeAndModes(0, texture, osg::StateAttribute::ON); 188 osg::Program * program = new osg::Program; 189 program->addShader(new osg::Shader(osg::Shader::FRAGMENT, fragShader)); 190 stateset->addUniform(new osg::Uniform("baseTex", 0)); 191 stateset->setAttributeAndModes(program, osg::StateAttribute::ON); 192 193 return geode.release(); 194 } 195 int main(int, char **) 196 { 197 // construct the viewer. 198 osgViewer::Viewer viewer; 199 200 // add model to viewer. 201 //viewer.setSceneData(createPyramidModel()); 202 viewer.setSceneData(createCone()); 203 viewer.setUpViewInWindow(200, 200, 800, 600, 0); 204 205 return viewer.run(); 206 }
作者:疯狂Delphi
本文版权归作者和博客园共有,欢迎转载,但未经作者同意必须保留此段声明,且在文章页面明显位置给出原文连接,否则保留追究法律责任的权利.
欢迎关注我,一起进步!扫描下方二维码即可加我
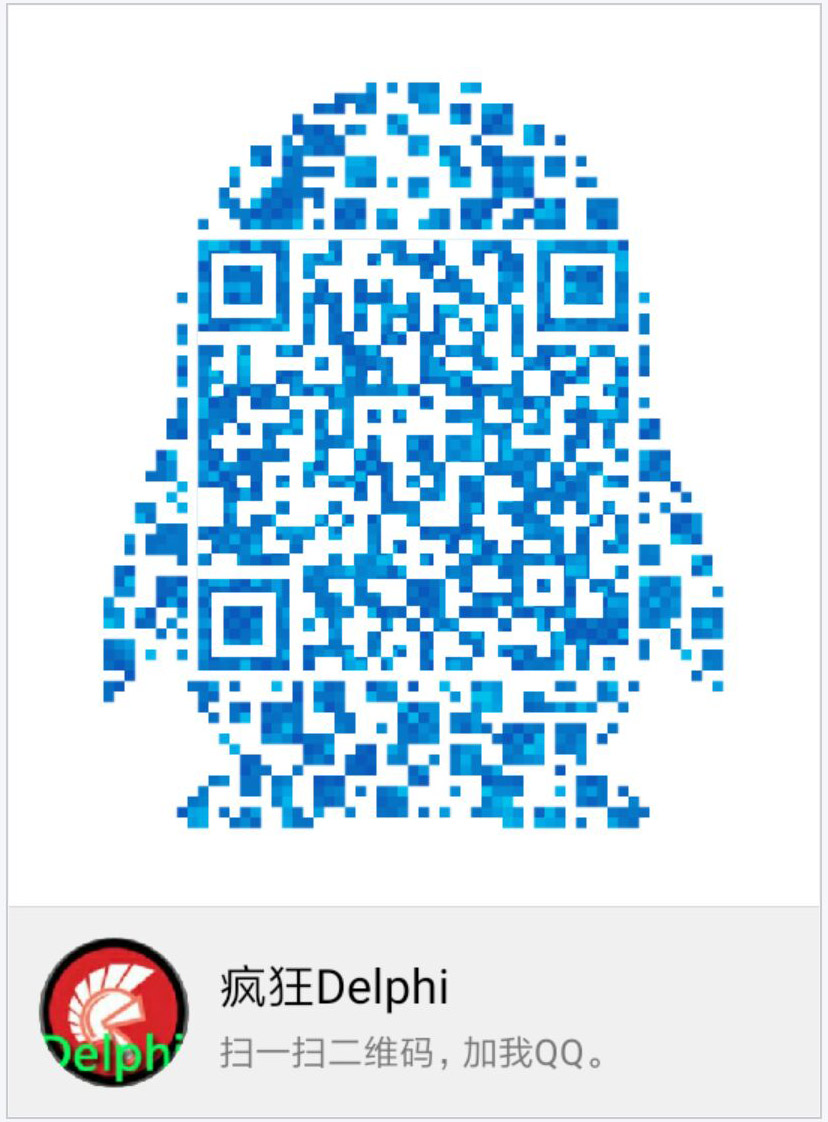
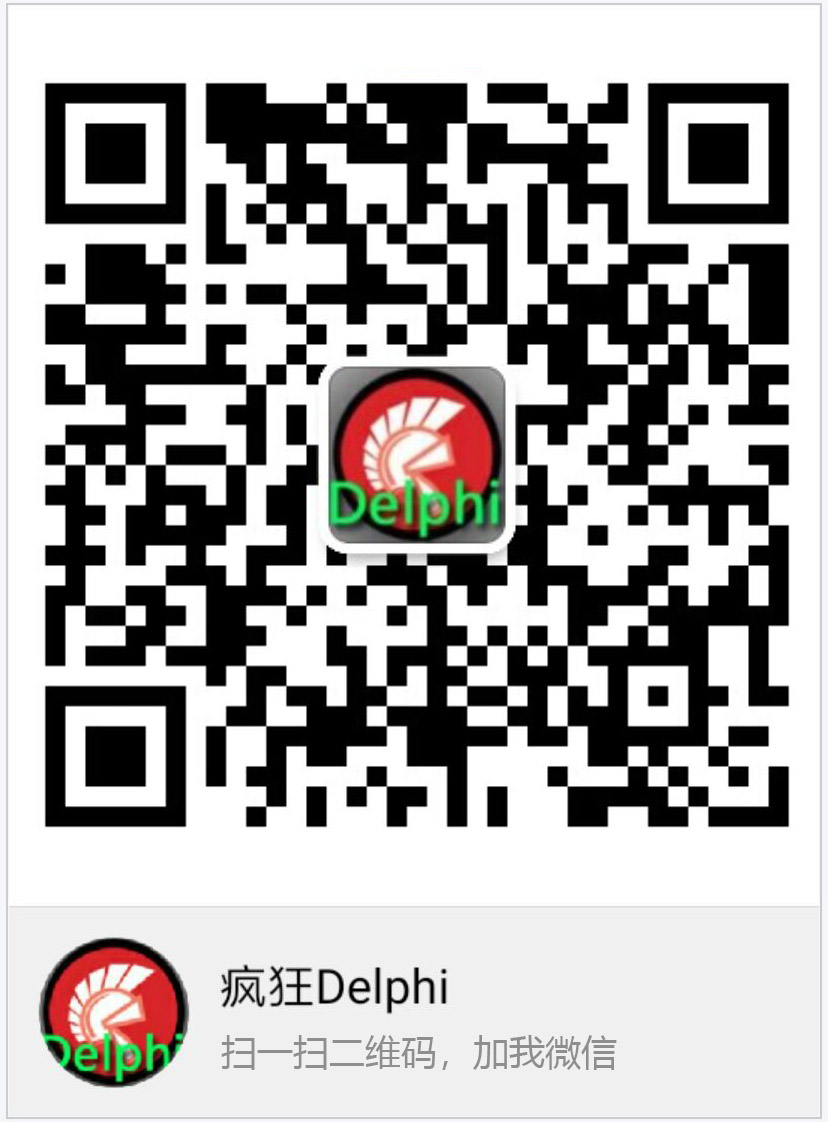