Osg-OsgEarth读取Tif格式的高程数据(Qt5.14.2+osgEart3.6.5+win10)-No13-ReadtifFile
相关资料:
https://blog.csdn.net/qq_42570058/article/details/119984000
https://blog.51cto.com/u_15127609/4146224
https://download.csdn.net/download/zhujianqiangqq/85521084
.pro

1 QT += core gui widgets 2 TARGET = TestOsgQt 3 TEMPLATE = app 4 DEFINES += QT_DEPRECATED_WARNINGS 5 CONFIG += c++11 6 7 SOURCES += \ 8 main.cpp 9 10 HEADERS += 11 12 OsgDir = D:\\RuanJian\\osg365R 13 CONFIG(release, debug|release) { 14 LIBS += -L$${OsgDir}/lib/ -losgDB -losgViewer -lOpenThreads -losgAnimation -losg \ 15 -losgEarth -losgEarthAnnotation -losgEarthFeatures -losgEarthSymbology -losgEarthUtil \ 16 -losgQOpenGL -losgUtil -losgText -losgTerrain -losgSim \ 17 -losgShadow -losgParticle -losgManipulator -losgGA -losgFX \ 18 -losgWidget 19 } else { 20 LIBS += -L$${OsgDir}/debug/lib/ -losgDBd -losgViewerd -lOpenThreadsd -losgAnimationd -losgd \ 21 -losgEarthd -losgEarthAnnotationd -losgEarthFeaturesd -losgEarthSymbologyd -losgEarthUtild \ 22 -losgQOpenGLd -losgUtild -losgTextd -losgTerraind -losgSimd \ 23 -losgShadowd -losgParticled -losgManipulatord -losgGAd -losgFXd \ 24 } 25 26 27 INCLUDEPATH += $${OsgDir}/include 28 DEPENDPATH += $${OsgDir}/include
main.cpp

1 #include <QApplication> 2 #ifdef _WIN32 3 #include <Windows.h> 4 #endif // _WIN32 5 #include <iostream> 6 //#include <math.h> 7 8 #include <osg/Node> 9 #include <osg/Group> 10 #include <osgDB/ReadFile> 11 #include <osgViewer/Viewer> 12 #include <osg/Geode> 13 #include <osg/ShapeDrawable> 14 #include <osg/Material> 15 #include <osg/Image> 16 #include <osg/Texture2D> 17 #include <osg/BoundingSphere> 18 #include <osg/LineWidth> 19 #include <osg/Point> 20 #include <osg/TexGen> 21 #include <osg/TexEnv> 22 23 //#include <osg/TessellationHints> 24 //#include <osg/NodePath> 25 #include <osgGA/GUIEventHandler> 26 #include <osgGA/GUIEventAdapter> 27 28 #include <osg/PositionAttitudeTransform> 29 #include <osgViewer/ViewerEventHandlers> 30 #include <osg/MatrixTransform> 31 #include <OpenThreads/Thread> 32 #include <osg/LightSource> 33 #include <osg/Light> 34 35 //#include <gdal.h> 36 37 osg::ref_ptr<osg::Node> CreateNode() 38 { 39 osg::ref_ptr<osg::Group> _root = new osg::Group; 40 41 //定义并读取高程文件 42 //真实高程文件名称为:ASTGTM2_N34E110_dem.tif 43 //属于特殊的tiff格式,GEOTiff 44 //读取的时候使用osg的gdal插件进行读取,所以在路径后面加上了.gdal 45 //.gdal后缀名只要在这里加就可以了,真实的高程文件后缀名不需要修改 46 //osg::ref_ptr<osg::HeightField> heightMap = osgDB::readHeightFieldFile("E:\\OpenSourceGraph\\osgearth_install20190830\\data\\world.tif.gdal"); 47 osg::ref_ptr<osg::HeightField> heightMap = osgDB::readHeightFieldFile("D:/Gitee/mapdata/world.tif.gdal"); 48 49 50 //创建一个叶结点对象 51 osg::ref_ptr<osg::Geode> geode = new osg::Geode; 52 53 if (heightMap != nullptr) 54 { 55 //由于原始数据过大,创建三维对象会失败,所以重新构造一个对象 56 //相当于数据抽稀了一次。当然,可以直接把原图使用特殊工具裁了 57 //创建一个新的HeightField对象,用来拷贝heightMap 58 osg::ref_ptr<osg::HeightField> heightMap1 = new osg::HeightField; 59 //从原对象中拷贝一些熟悉过来 60 heightMap1->setOrigin(heightMap->getOrigin()); 61 heightMap1->setRotation(heightMap->getRotation()); 62 heightMap1->setSkirtHeight(heightMap->getSkirtHeight()); 63 //XY方向的间隔设置为原来的两倍, 64 heightMap1->setXInterval(heightMap->getXInterval() * 2); 65 heightMap1->setYInterval(heightMap->getYInterval() * 2); 66 //设置新的高程数据量的行列数目为原来的一半 67 heightMap1->allocate(heightMap->getNumColumns() / 2, heightMap->getNumRows() / 2); 68 69 //把真实的数据值放进来 70 for (size_t r = 0; r < heightMap1->getNumRows(); ++r) 71 { 72 for (size_t c = 0; c < heightMap1->getNumColumns(); ++c) 73 { 74 //加载的数据中XY方向的间隔是0.0002左右(经纬度偏移),3600个格子,数量级太小,高程值动辄在千级别,如果没有进行坐标转换(GPS转换成米),显示出来之后结果会严重失常。所以此处简单的给高度值除以50000(这个是按照这个tif文件来试出来的,不同高程文件可能不同) 75 heightMap1->setHeight(c, r, heightMap->getHeight(c * 2, r * 2) / 500); 76 } 77 } 78 79 //添加到叶子节点中 80 geode->addDrawable(new osg::ShapeDrawable(heightMap1)); 81 82 83 osg::ref_ptr<osg::Material> material = new osg::Material; 84 material->setAmbient(osg::Material::FRONT_AND_BACK, osg::Vec4(1.0f, 1.0f, 1.0f, 1.0f)); 85 material->setDiffuse(osg::Material::FRONT_AND_BACK, osg::Vec4(1.0f, 1.0f, 1.0f, 1.0f)); 86 material->setSpecular(osg::Material::FRONT_AND_BACK, osg::Vec4(1.0f, 1.0f, 1.0f, 1.0f)); 87 material->setShininess(osg::Material::FRONT_AND_BACK, 60); 88 89 90 osg::ref_ptr<osg::Texture2D> texture2D = new osg::Texture2D; 91 //设置纹理 92 osg::ref_ptr<osg::Image> image1 = osgDB::readImageFile("D:/Gitee/OsgTestQt/src/No1-D/Earth_Color_16k.jpg"); 93 if (image1.valid()) 94 { 95 texture2D->setImage(image1.get()); 96 } 97 texture2D->setDataVariance(osg::Object::DYNAMIC); 98 geode->getOrCreateStateSet()->setAttributeAndModes(material.get(), osg::StateAttribute::ON); 99 geode->getOrCreateStateSet()->setTextureAttributeAndModes(0, texture2D, osg::StateAttribute::ON); 100 101 } 102 103 _root->addChild(geode.get()); 104 return _root.get(); 105 } 106 107 osg::ref_ptr<osg::Light> createLight() 108 { 109 osg::ref_ptr<osg::Light> l = new osg::Light; 110 l->setLightNum(0);//启用第几个光源 OpenGL有8个光源 111 l->setDirection(osg::Vec3(0, 0, -1));//方向 112 l->setPosition(osg::Vec4(10.0, 10.0, 0.0, 0.0f));//位置 113 //osg::LightSource* ls = new osg::LightSource();//此处用超级指针 返回会发生错误 114 //ls->setLight(l); 115 return l; 116 } 117 118 119 120 int main() 121 { 122 osg::ref_ptr<osgViewer::Viewer> viewer1 = new osgViewer::Viewer; 123 osg::ref_ptr<osg::Group> group1 = new osg::Group; 124 125 group1->addChild(CreateNode()); 126 viewer1->setSceneData(group1.get()); 127 viewer1->setUpViewInWindow(200, 200, 600, 400, 0); 128 viewer1->setLight(createLight()); 129 130 131 return viewer1->run(); 132 }
作者:疯狂Delphi
本文版权归作者和博客园共有,欢迎转载,但未经作者同意必须保留此段声明,且在文章页面明显位置给出原文连接,否则保留追究法律责任的权利.
欢迎关注我,一起进步!扫描下方二维码即可加我
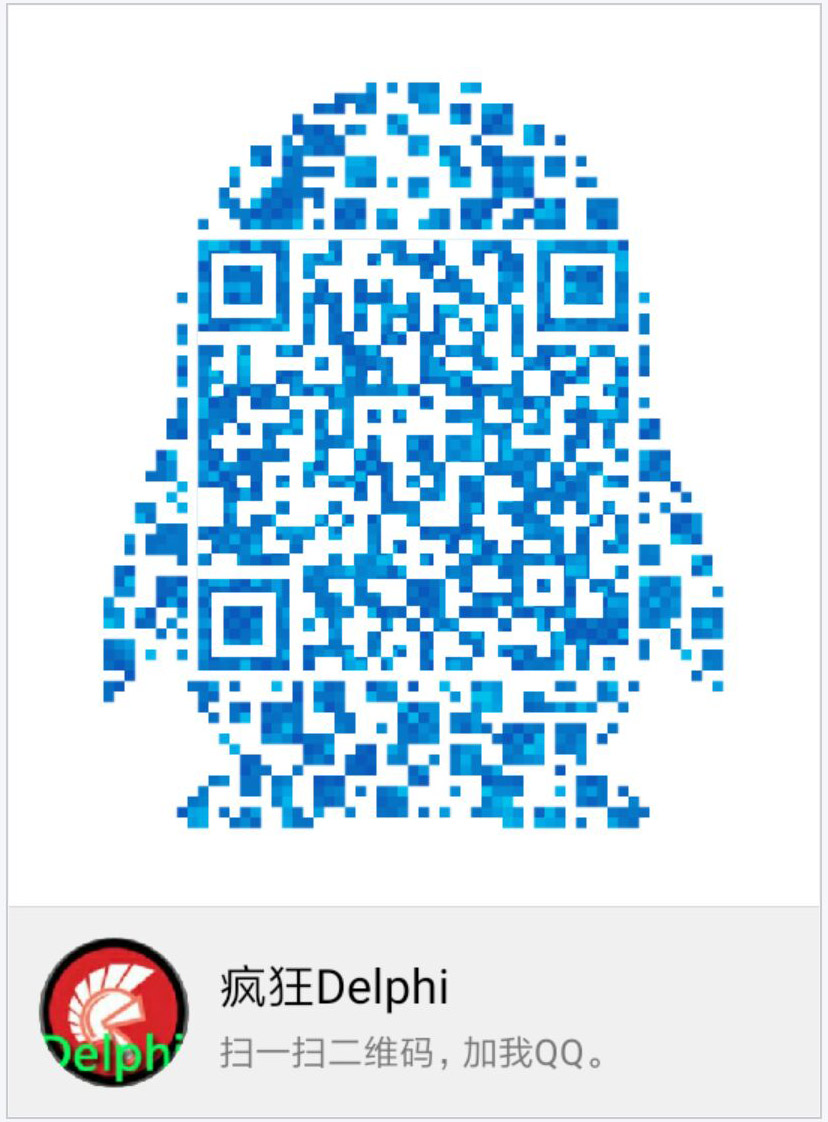
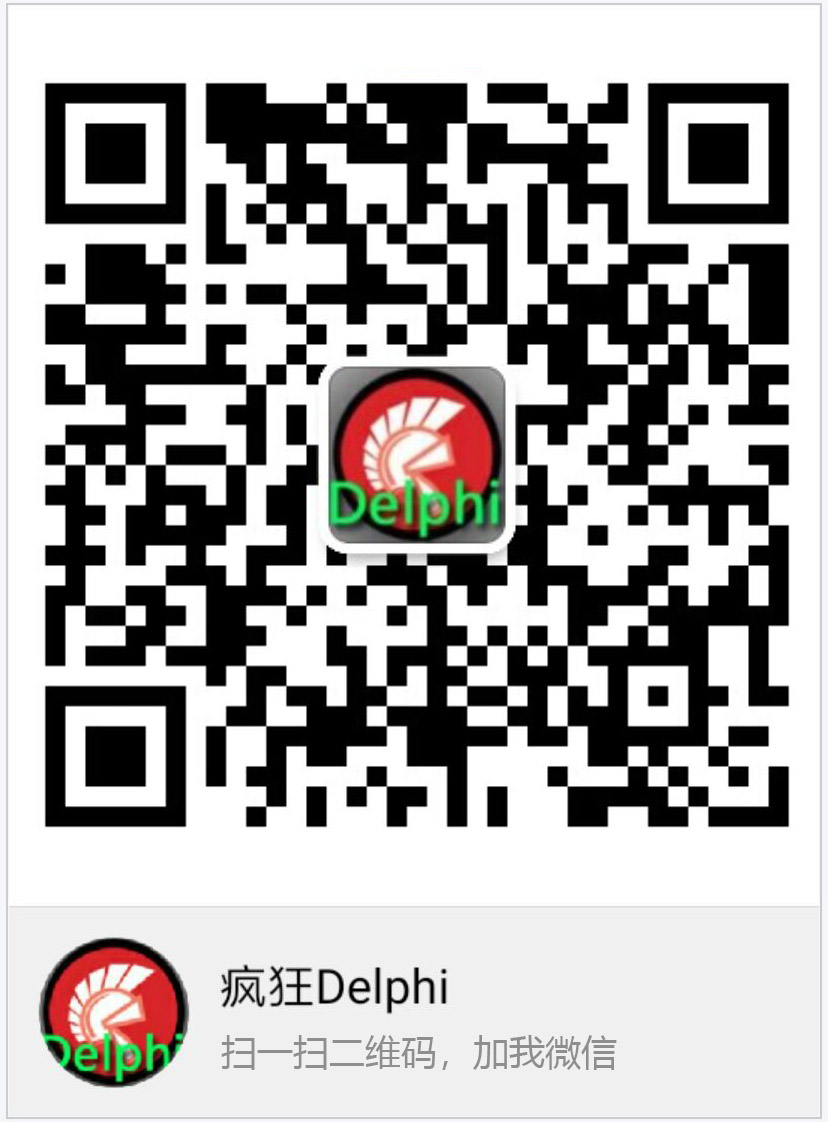