Qt-Qt使用QWebsocket实现服务端和客户端通信
相关资料:
https://blog.csdn.net/qq_40110291/article/details/103734258 Qt websocket 服务端和客户端通信
实例代码(服务端):
.pro

1 QT += core gui 2 QT += websockets 3 4 greaterThan(QT_MAJOR_VERSION, 4): QT += widgets 5 6 CONFIG += c++11 7 8 # The following define makes your compiler emit warnings if you use 9 # any Qt feature that has been marked deprecated (the exact warnings 10 # depend on your compiler). Please consult the documentation of the 11 # deprecated API in order to know how to port your code away from it. 12 DEFINES += QT_DEPRECATED_WARNINGS 13 14 # You can also make your code fail to compile if it uses deprecated APIs. 15 # In order to do so, uncomment the following line. 16 # You can also select to disable deprecated APIs only up to a certain version of Qt. 17 #DEFINES += QT_DISABLE_DEPRECATED_BEFORE=0x060000 # disables all the APIs deprecated before Qt 6.0.0 18 19 SOURCES += \ 20 main.cpp \ 21 mainwindow.cpp 22 23 HEADERS += \ 24 mainwindow.h 25 26 FORMS += \ 27 mainwindow.ui 28 29 # Default rules for deployment. 30 qnx: target.path = /tmp/$${TARGET}/bin 31 else: unix:!android: target.path = /opt/$${TARGET}/bin 32 !isEmpty(target.path): INSTALLS += target
main.cpp

1 #include "mainwindow.h" 2 3 #include <QApplication> 4 5 int main(int argc, char *argv[]) 6 { 7 QApplication a(argc, argv); 8 MainWindow w; 9 w.show(); 10 return a.exec(); 11 }
mainwindow.h

1 #ifndef MAINWINDOW_H 2 #define MAINWINDOW_H 3 4 #include <QMainWindow> 5 6 #include <QWebSocketServer> 7 #include <QWebSocket> 8 9 QT_BEGIN_NAMESPACE 10 namespace Ui { class MainWindow; } 11 QT_END_NAMESPACE 12 13 class MainWindow : public QMainWindow 14 { 15 Q_OBJECT 16 17 public: 18 MainWindow(QWidget *parent = nullptr); 19 ~MainWindow(); 20 21 public slots: 22 void onStartButtonClick(); 23 void onStopButtonClick(); 24 void onSendButtonClick(); 25 void onCleanButtonClick(); 26 27 Q_SIGNALS: 28 void closed(); 29 private Q_SLOTS: 30 void onNewConnection(); 31 void processTextMessage(QString message); 32 void socketDisconnected(); 33 34 private: 35 Ui::MainWindow *ui; 36 37 QWebSocketServer *m_WebSocketServer; 38 QList<QWebSocket *> m_clients; 39 bool m_debug; 40 QWebSocket *pSocket; 41 QDateTime *current_date_time; 42 43 }; 44 #endif // MAINWINDOW_H
mainwindow.cpp

1 #include "mainwindow.h" 2 #include "ui_mainwindow.h" 3 4 MainWindow::MainWindow(QWidget *parent) 5 : QMainWindow(parent) 6 , ui(new Ui::MainWindow) 7 { 8 ui->setupUi(this); 9 10 m_WebSocketServer = new QWebSocketServer("server", QWebSocketServer::NonSecureMode); 11 connect(m_WebSocketServer, SIGNAL(newConnection()), this, SLOT(onNewConnection())); 12 connect(ui->startButton, SIGNAL(clicked(bool)), this, SLOT(onStartButtonClick())); 13 connect(ui->stopButton, SIGNAL(clicked(bool)), this, SLOT(onStopButtonClick())); 14 connect(ui->cleanButton, SIGNAL(clicked(bool)), this, SLOT(onCleanButtonClick())); 15 connect(ui->sendButton, SIGNAL(clicked(bool)), this, SLOT(onSendButtonClick())); 16 17 } 18 19 MainWindow::~MainWindow() 20 { 21 delete ui; 22 } 23 24 void MainWindow::onStartButtonClick() 25 { 26 int i_port = ui->monitorSpinBox->text().toInt(); 27 m_WebSocketServer->listen(QHostAddress::Any, i_port); 28 ui->startButton->setEnabled(false); 29 ui->stopButton->setEnabled(true); 30 } 31 32 void MainWindow::onStopButtonClick() 33 { 34 ui->startButton->setEnabled(true); 35 ui->stopButton->setEnabled(false); 36 m_WebSocketServer->close(); 37 } 38 39 void MainWindow::onSendButtonClick() 40 { 41 QString msg = ui->sendTextedit->document()->toPlainText(); 42 for (int i=0;i<m_clients.size();i++) 43 { 44 m_clients.at(i)->sendTextMessage(msg); 45 } 46 } 47 48 void MainWindow::onCleanButtonClick() 49 { 50 ui->receiveTextEdit->clear(); 51 } 52 53 void MainWindow::onNewConnection() 54 { 55 ui->startButton->setEnabled(false); 56 ui->stopButton->setEnabled(true); 57 ui->sendTextedit->setEnabled(true); 58 ui->sendButton->setEnabled(true); 59 ui->cleanButton->setEnabled(true); 60 61 pSocket = m_WebSocketServer->nextPendingConnection(); 62 63 connect(pSocket, SIGNAL(textMessageReceived(QString)), this, SLOT(processTextMessage(QString))); 64 connect(pSocket, SIGNAL(disconnected()), this, SLOT(socketDisconnected())); 65 66 QString item = pSocket->peerAddress().toString(); 67 ui->linkclientListWidget->addItem(item); 68 m_clients << pSocket; 69 } 70 71 void MainWindow::processTextMessage(QString message) 72 { 73 QString time = current_date_time->currentDateTime().toString("yyyy.MM.dd hh:mm:ss.zzz ddd"); 74 QString item = pSocket->peerAddress().toString(); 75 ui->receiveTextEdit->append(time + "" + item + "\n" + message); 76 } 77 78 void MainWindow::socketDisconnected() 79 { 80 ui->startButton->setEnabled(true); 81 ui->stopButton->setEnabled(false); 82 ui->sendButton->setEnabled(false); 83 ui->sendTextedit->setEnabled(false); 84 ui->receiveTextEdit->setEnabled(false); 85 ui->cleanButton->setEnabled(false); 86 }
mainwindow.ui

1 <?xml version="1.0" encoding="UTF-8"?> 2 <ui version="4.0"> 3 <class>MainWindow</class> 4 <widget class="QMainWindow" name="MainWindow"> 5 <property name="geometry"> 6 <rect> 7 <x>0</x> 8 <y>0</y> 9 <width>800</width> 10 <height>600</height> 11 </rect> 12 </property> 13 <property name="windowTitle"> 14 <string>MainWindow</string> 15 </property> 16 <widget class="QWidget" name="centralwidget"> 17 <widget class="QLabel" name="label"> 18 <property name="geometry"> 19 <rect> 20 <x>30</x> 21 <y>40</y> 22 <width>54</width> 23 <height>12</height> 24 </rect> 25 </property> 26 <property name="text"> 27 <string>TextLabel</string> 28 </property> 29 </widget> 30 <widget class="QSpinBox" name="monitorSpinBox"> 31 <property name="geometry"> 32 <rect> 33 <x>100</x> 34 <y>30</y> 35 <width>101</width> 36 <height>22</height> 37 </rect> 38 </property> 39 <property name="maximum"> 40 <number>50000</number> 41 </property> 42 </widget> 43 <widget class="QPushButton" name="startButton"> 44 <property name="geometry"> 45 <rect> 46 <x>220</x> 47 <y>30</y> 48 <width>80</width> 49 <height>20</height> 50 </rect> 51 </property> 52 <property name="text"> 53 <string>启动</string> 54 </property> 55 </widget> 56 <widget class="QPushButton" name="stopButton"> 57 <property name="geometry"> 58 <rect> 59 <x>320</x> 60 <y>30</y> 61 <width>80</width> 62 <height>20</height> 63 </rect> 64 </property> 65 <property name="text"> 66 <string>停止</string> 67 </property> 68 </widget> 69 <widget class="QTextEdit" name="sendTextedit"> 70 <property name="geometry"> 71 <rect> 72 <x>30</x> 73 <y>80</y> 74 <width>361</width> 75 <height>70</height> 76 </rect> 77 </property> 78 </widget> 79 <widget class="QPushButton" name="sendButton"> 80 <property name="geometry"> 81 <rect> 82 <x>410</x> 83 <y>80</y> 84 <width>80</width> 85 <height>20</height> 86 </rect> 87 </property> 88 <property name="text"> 89 <string>发送</string> 90 </property> 91 </widget> 92 <widget class="QTextEdit" name="receiveTextEdit"> 93 <property name="geometry"> 94 <rect> 95 <x>30</x> 96 <y>220</y> 97 <width>351</width> 98 <height>211</height> 99 </rect> 100 </property> 101 </widget> 102 <widget class="QLabel" name="label_2"> 103 <property name="geometry"> 104 <rect> 105 <x>30</x> 106 <y>200</y> 107 <width>54</width> 108 <height>12</height> 109 </rect> 110 </property> 111 <property name="text"> 112 <string>TextLabel</string> 113 </property> 114 </widget> 115 <widget class="QLabel" name="label_3"> 116 <property name="geometry"> 117 <rect> 118 <x>390</x> 119 <y>200</y> 120 <width>54</width> 121 <height>12</height> 122 </rect> 123 </property> 124 <property name="text"> 125 <string>TextLabel</string> 126 </property> 127 </widget> 128 <widget class="QPushButton" name="cleanButton"> 129 <property name="geometry"> 130 <rect> 131 <x>630</x> 132 <y>190</y> 133 <width>80</width> 134 <height>20</height> 135 </rect> 136 </property> 137 <property name="text"> 138 <string>清除</string> 139 </property> 140 </widget> 141 <widget class="QListWidget" name="linkclientListWidget"> 142 <property name="geometry"> 143 <rect> 144 <x>410</x> 145 <y>230</y> 146 <width>256</width> 147 <height>192</height> 148 </rect> 149 </property> 150 </widget> 151 </widget> 152 <widget class="QMenuBar" name="menubar"> 153 <property name="geometry"> 154 <rect> 155 <x>0</x> 156 <y>0</y> 157 <width>800</width> 158 <height>22</height> 159 </rect> 160 </property> 161 </widget> 162 <widget class="QStatusBar" name="statusbar"/> 163 </widget> 164 <resources/> 165 <connections/> 166 </ui>
客户端:
.pro

1 QT += core gui 2 QT += websockets 3 4 greaterThan(QT_MAJOR_VERSION, 4): QT += widgets 5 6 CONFIG += c++11 7 8 # The following define makes your compiler emit warnings if you use 9 # any Qt feature that has been marked deprecated (the exact warnings 10 # depend on your compiler). Please consult the documentation of the 11 # deprecated API in order to know how to port your code away from it. 12 DEFINES += QT_DEPRECATED_WARNINGS 13 14 # You can also make your code fail to compile if it uses deprecated APIs. 15 # In order to do so, uncomment the following line. 16 # You can also select to disable deprecated APIs only up to a certain version of Qt. 17 #DEFINES += QT_DISABLE_DEPRECATED_BEFORE=0x060000 # disables all the APIs deprecated before Qt 6.0.0 18 19 SOURCES += \ 20 main.cpp \ 21 mainwindow.cpp 22 23 HEADERS += \ 24 mainwindow.h 25 26 FORMS += \ 27 mainwindow.ui 28 29 # Default rules for deployment. 30 qnx: target.path = /tmp/$${TARGET}/bin 31 else: unix:!android: target.path = /opt/$${TARGET}/bin 32 !isEmpty(target.path): INSTALLS += target
main.cpp

1 #include "mainwindow.h" 2 3 #include <QApplication> 4 5 int main(int argc, char *argv[]) 6 { 7 QApplication a(argc, argv); 8 MainWindow w; 9 w.show(); 10 return a.exec(); 11 }
mainwindow.h

1 #ifndef MAINWINDOW_H 2 #define MAINWINDOW_H 3 4 #include <QMainWindow> 5 6 #include <QWebSocket> 7 8 QT_BEGIN_NAMESPACE 9 namespace Ui { class MainWindow; } 10 QT_END_NAMESPACE 11 12 class MainWindow : public QMainWindow 13 { 14 Q_OBJECT 15 16 public: 17 MainWindow(QWidget *parent = nullptr); 18 ~MainWindow(); 19 20 Q_SIGNALS: 21 void closed(); 22 23 private Q_SLOTS: 24 void connectToServer(); 25 void onTextMessageReceived(const QString &message); 26 void closeConnection(); 27 28 public slots: 29 void stopClicked(); 30 void onconnected(); 31 void onSendButtonClicked(); 32 void onCleanButtonClicked(); 33 private: 34 Ui::MainWindow *ui; 35 36 QUrl m_url; 37 QWebSocket m_websocket; 38 bool m_debug; 39 QDateTime *current_date_time; 40 }; 41 #endif // MAINWINDOW_H
mainwindow.cpp

1 #include "mainwindow.h" 2 #include "ui_mainwindow.h" 3 4 MainWindow::MainWindow(QWidget *parent) 5 : QMainWindow(parent) 6 , ui(new Ui::MainWindow) 7 { 8 ui->setupUi(this); 9 connect(ui->linkbutton, SIGNAL(clicked(bool)), this, SLOT(connectToServer())); 10 connect(ui->disconnectbutton, SIGNAL(clicked(bool)), this, SLOT(stopClicked())); 11 connect(ui->sendbutton, SIGNAL(clicked(bool)), this, SLOT(onSendButtonClicked())); 12 connect(ui->cleanButton, SIGNAL(clicked(bool)), this, SLOT(onCleanButtonClicked())); 13 connect(&m_websocket, SIGNAL(connected()), this, SLOT(onconnected())); 14 connect(&m_websocket, SIGNAL(disconnected()), this, SLOT(closeConnection())); 15 connect(&m_websocket, SIGNAL(textMessageReceived(QString)), this, SLOT(onTextMessageReceived(QString))); 16 17 } 18 19 MainWindow::~MainWindow() 20 { 21 delete ui; 22 m_websocket.errorString(); 23 m_websocket.close(); 24 } 25 26 void MainWindow::connectToServer() 27 { 28 QString path = QString("ws://%1:%2").arg(ui->iplineedit->text()).arg(ui->portspinbox->text()); 29 QUrl url = QUrl(path); 30 m_websocket.open(url); 31 } 32 33 void MainWindow::onTextMessageReceived(const QString &message) 34 { 35 QString time = current_date_time->currentDateTime().toString("yyyy.MM.dd hh:mm:ss.zzz ddd"); 36 ui->receivemessageTextEdit->append(time + "\n" + message); 37 } 38 39 void MainWindow::closeConnection() 40 { 41 ui->linkbutton->setEnabled(true); 42 ui->disconnectbutton->setEnabled(false); 43 ui->sendmessagetextedit->setEnabled(false); 44 ui->sendbutton->setEnabled(false); 45 ui->receivemessageTextEdit->setEnabled(false); 46 ui->cleanButton->setEnabled(false); 47 // ui->statusLabel->setText(tr("disconnected")); 48 } 49 50 void MainWindow::stopClicked() 51 { 52 m_websocket.close(); 53 } 54 55 void MainWindow::onconnected() 56 { 57 qDebug() << "hello word!"; 58 // ui->statusLabel->setText(tr("connected")); 59 ui->linkbutton->setEnabled(false); 60 ui->disconnectbutton->setEnabled(true); 61 ui->sendmessagetextedit->setEnabled(true); 62 ui->sendbutton->setEnabled(true); 63 ui->receivemessageTextEdit->setEnabled(true); 64 ui->cleanButton->setEnabled(true); 65 } 66 67 void MainWindow::onSendButtonClicked() 68 { 69 QString msg = ui->sendmessagetextedit->document()->toPlainText(); 70 m_websocket.sendTextMessage(msg); 71 } 72 73 void MainWindow::onCleanButtonClicked() 74 { 75 ui->receivemessageTextEdit->clear(); 76 }
mainwindow.ui

1 <?xml version="1.0" encoding="UTF-8"?> 2 <ui version="4.0"> 3 <class>MainWindow</class> 4 <widget class="QMainWindow" name="MainWindow"> 5 <property name="geometry"> 6 <rect> 7 <x>0</x> 8 <y>0</y> 9 <width>561</width> 10 <height>406</height> 11 </rect> 12 </property> 13 <property name="windowTitle"> 14 <string>MainWindow</string> 15 </property> 16 <widget class="QWidget" name="centralwidget"> 17 <widget class="QPushButton" name="linkbutton"> 18 <property name="geometry"> 19 <rect> 20 <x>330</x> 21 <y>20</y> 22 <width>80</width> 23 <height>20</height> 24 </rect> 25 </property> 26 <property name="text"> 27 <string>连接</string> 28 </property> 29 </widget> 30 <widget class="QLabel" name="label"> 31 <property name="geometry"> 32 <rect> 33 <x>30</x> 34 <y>20</y> 35 <width>54</width> 36 <height>12</height> 37 </rect> 38 </property> 39 <property name="text"> 40 <string>TextLabel</string> 41 </property> 42 </widget> 43 <widget class="QLineEdit" name="iplineedit"> 44 <property name="geometry"> 45 <rect> 46 <x>100</x> 47 <y>20</y> 48 <width>113</width> 49 <height>20</height> 50 </rect> 51 </property> 52 </widget> 53 <widget class="QSpinBox" name="portspinbox"> 54 <property name="geometry"> 55 <rect> 56 <x>230</x> 57 <y>20</y> 58 <width>81</width> 59 <height>22</height> 60 </rect> 61 </property> 62 <property name="maximum"> 63 <number>50000</number> 64 </property> 65 </widget> 66 <widget class="QPushButton" name="disconnectbutton"> 67 <property name="geometry"> 68 <rect> 69 <x>430</x> 70 <y>20</y> 71 <width>80</width> 72 <height>20</height> 73 </rect> 74 </property> 75 <property name="text"> 76 <string>断开</string> 77 </property> 78 </widget> 79 <widget class="QLabel" name="label_2"> 80 <property name="geometry"> 81 <rect> 82 <x>30</x> 83 <y>80</y> 84 <width>54</width> 85 <height>12</height> 86 </rect> 87 </property> 88 <property name="text"> 89 <string>TextLabel</string> 90 </property> 91 </widget> 92 <widget class="QTextEdit" name="sendmessagetextedit"> 93 <property name="geometry"> 94 <rect> 95 <x>30</x> 96 <y>110</y> 97 <width>281</width> 98 <height>70</height> 99 </rect> 100 </property> 101 </widget> 102 <widget class="QPushButton" name="sendbutton"> 103 <property name="geometry"> 104 <rect> 105 <x>330</x> 106 <y>110</y> 107 <width>80</width> 108 <height>20</height> 109 </rect> 110 </property> 111 <property name="text"> 112 <string>发送</string> 113 </property> 114 </widget> 115 <widget class="QLabel" name="label_3"> 116 <property name="geometry"> 117 <rect> 118 <x>30</x> 119 <y>210</y> 120 <width>54</width> 121 <height>12</height> 122 </rect> 123 </property> 124 <property name="text"> 125 <string>TextLabel</string> 126 </property> 127 </widget> 128 <widget class="QTextEdit" name="receivemessageTextEdit"> 129 <property name="geometry"> 130 <rect> 131 <x>30</x> 132 <y>240</y> 133 <width>281</width> 134 <height>101</height> 135 </rect> 136 </property> 137 </widget> 138 <widget class="QPushButton" name="cleanButton"> 139 <property name="geometry"> 140 <rect> 141 <x>330</x> 142 <y>240</y> 143 <width>80</width> 144 <height>20</height> 145 </rect> 146 </property> 147 <property name="text"> 148 <string>清除</string> 149 </property> 150 </widget> 151 </widget> 152 <widget class="QMenuBar" name="menubar"> 153 <property name="geometry"> 154 <rect> 155 <x>0</x> 156 <y>0</y> 157 <width>561</width> 158 <height>22</height> 159 </rect> 160 </property> 161 </widget> 162 <widget class="QStatusBar" name="statusbar"/> 163 </widget> 164 <resources/> 165 <connections/> 166 </ui>
作者:疯狂Delphi
本文版权归作者和博客园共有,欢迎转载,但未经作者同意必须保留此段声明,且在文章页面明显位置给出原文连接,否则保留追究法律责任的权利.
欢迎关注我,一起进步!扫描下方二维码即可加我
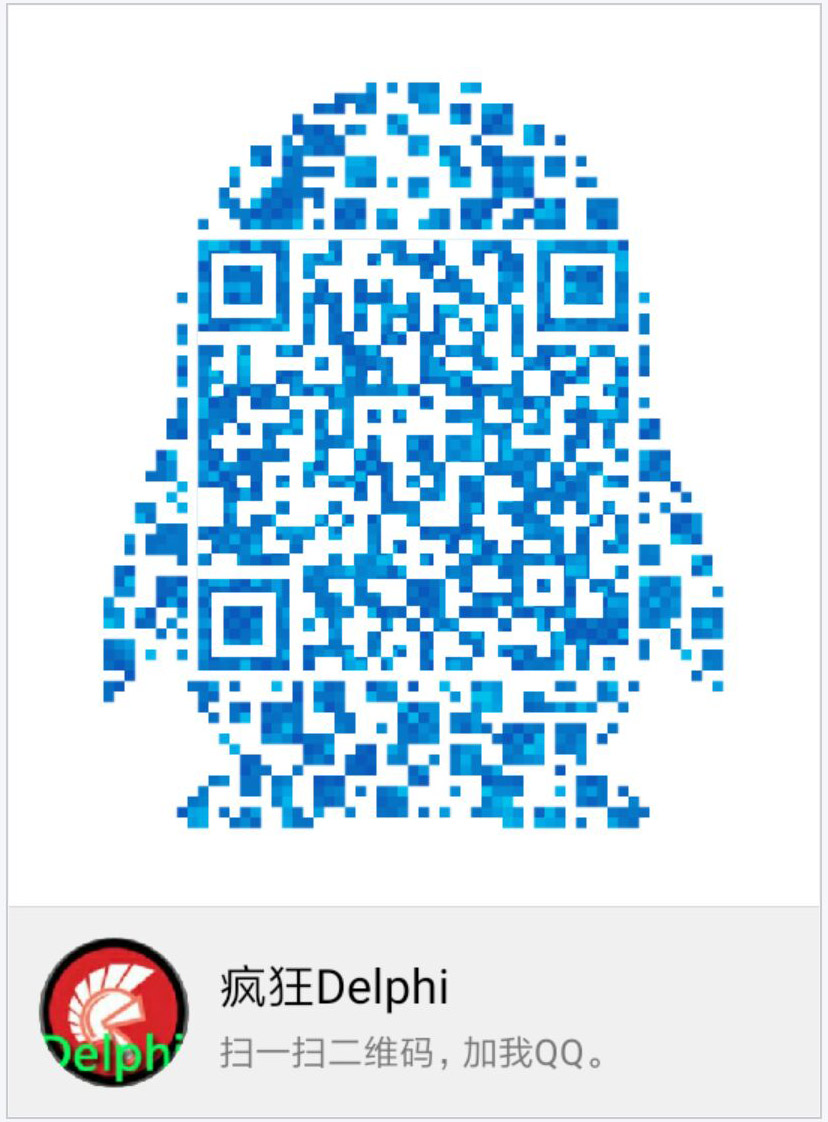
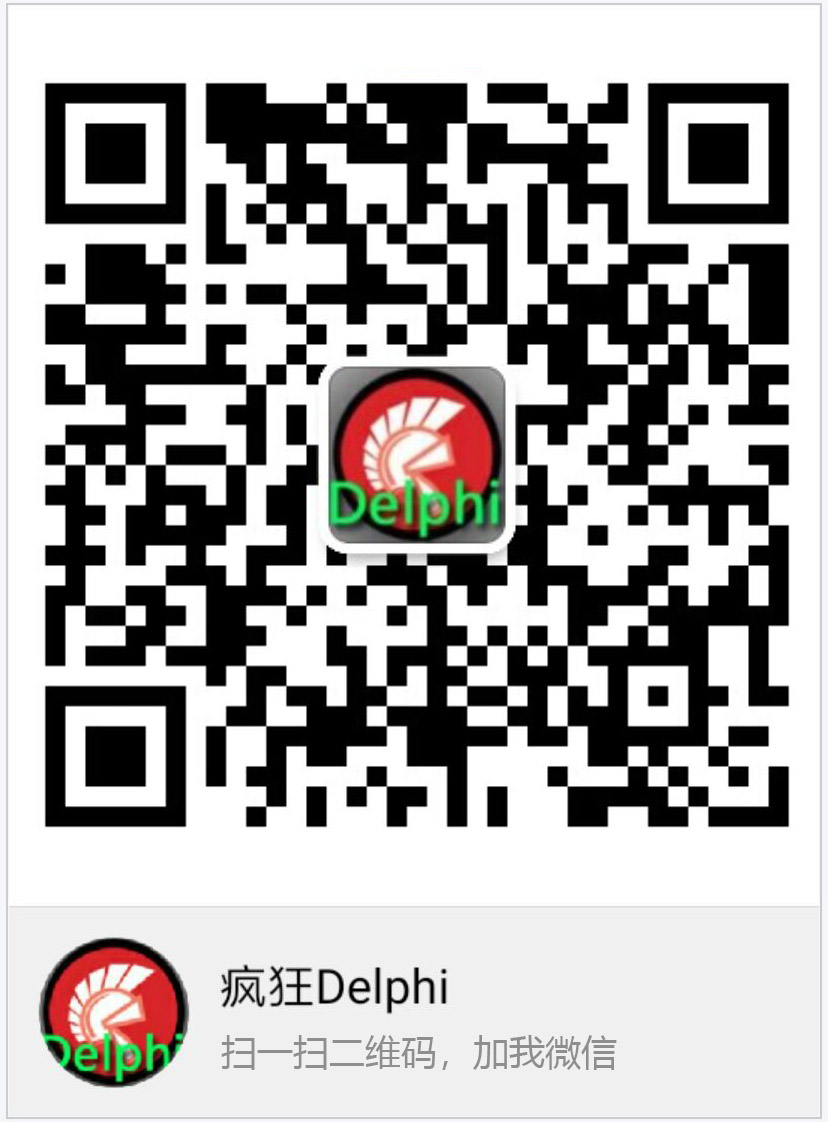