Osg-OsgEarth实例圆锥体旋转效果(Qt5.14.2+osgEarht3.6.5+win10)-No12-OsgEarthModelRotate
相关资料:
https://www.cnblogs.com/coolbear/p/7765862.html osgEarth设置模型旋转角度
代码实例:
.pro

1 QT += core gui widgets 2 TARGET = TestOsgQt 3 TEMPLATE = app 4 DEFINES += QT_DEPRECATED_WARNINGS 5 CONFIG += c++11 6 7 SOURCES += \ 8 main.cpp 9 10 HEADERS += 11 12 OsgDir = D:\\RuanJian\\osg365R 13 CONFIG(release, debug|release) { 14 LIBS += -L$${OsgDir}/lib/ -losgDB -losgViewer -lOpenThreads -losgAnimation -losg \ 15 -losgEarth -losgEarthAnnotation -losgEarthFeatures -losgEarthSymbology -losgEarthUtil \ 16 -losgQOpenGL -losgUtil -losgText -losgTerrain -losgSim \ 17 -losgShadow -losgParticle -losgManipulator -losgGA -losgFX \ 18 -losgWidget 19 } else { 20 LIBS += -L$${OsgDir}/debug/lib/ -losgDBd -losgViewerd -lOpenThreadsd -losgAnimationd -losgd \ 21 -losgEarthd -losgEarthAnnotationd -losgEarthFeaturesd -losgEarthSymbologyd -losgEarthUtild \ 22 -losgQOpenGLd -losgUtild -losgTextd -losgTerraind -losgSimd \ 23 -losgShadowd -losgParticled -losgManipulatord -losgGAd -losgFXd \ 24 } 25 26 27 INCLUDEPATH += $${OsgDir}/include 28 DEPENDPATH += $${OsgDir}/include
main.cpp

1 #include <QApplication> 2 3 #include <osg/Node> 4 #include <osg/Group> 5 #include <osg/Geode> 6 #include <osg/Geometry> 7 #include <osg/Texture2D> 8 #include <osg/StateSet> 9 #include <osg/PositionAttitudeTransform> 10 #include <osgViewer/Viewer> 11 #include <osgDB/ReadFile> 12 #include <osgParticle/PrecipitationEffect> 13 // 粒子效果 14 #include <osgParticle/PrecipitationEffect> 15 #include <osgParticle/Particle> 16 #include <osgParticle/LinearInterpolator> 17 #include <osgParticle/ParticleSystem> 18 #include <osgParticle/RandomRateCounter> 19 #include <osgParticle/PointPlacer> 20 #include <osgParticle/RadialShooter> 21 #include <osgParticle/ModularEmitter> 22 #include <osgParticle/ParticleSystemUpdater> 23 #include <osgParticle/ModularProgram> 24 #include <osgUtil/Optimizer> 25 #include <osgUtil/Simplifier> 26 #include <osgParticle/FireEffect> 27 // 雾 28 #include <osg/Fog> 29 #include <osgDB/ReadFile> 30 #include <osgViewer/Viewer> 31 #include <osg/StateSet> 32 #include <osg/StateAttribute> 33 #include <osgViewer/ViewerEventHandlers> 34 #include <osgWidget/ViewerEventHandlers> 35 // 旋转 36 #include <osg/NodeCallback> 37 #include <osg/PositionAttitudeTransform> 38 #include <osgViewer/Viewer> 39 #include <osg/MatrixTransform> 40 #include <osgDB/ReadFile> 41 #include <osgGA/TrackballManipulator> 42 // 圆形 43 #include <osg/ShapeDrawable> 44 // 模型旋转角度 45 #include <osgEarthDrivers/gdal/GDALOptions> 46 #include <osgEarthUtil/EarthManipulator> 47 #include <osgEarthFeatures/ConvertTypeFilter> 48 #include <osgEarthDrivers/model_simple/SimpleModelOptions> 49 #include <osgGA/StateSetManipulator> 50 #include <osgEarth/ImageLayer> 51 #include <osgEarth/ModelLayer> 52 53 // lonlat1:雷达波圆锥顶点 54 // lonlat2:轨迹点 55 void rotateCone(osg::MatrixTransform* mt, const osgEarth::SpatialReference* sr, osg::Vec3d lonlat1, osg::Vec3d lonlat2) 56 { 57 // 雷达波模型所在位置 58 osgEarth::GeoPoint geoPoint1( 59 sr, 60 lonlat1.x(), 61 lonlat1.y(), 62 lonlat1.z(), 63 osgEarth::ALTMODE_ABSOLUTE); 64 osg::Matrixd matrix1; 65 // 获取雷达波模型从原点变换到lonlat1位置的变换矩阵 66 geoPoint1.createLocalToWorld(matrix1); 67 // 经纬度高程到xyz的变换 68 osg::Vec3d world1, world2; 69 // geoPoint1.toWorld(world1);//等同于 sr->transformToWorld(lonlat1,world1); 70 sr->transformToWorld(lonlat2, world2); 71 // 计算轨迹点在雷达波模型坐标系下的位置 72 osg::Vec3 point2InRadarCoordinateSystem = world2*osg::Matrix::inverse(matrix1); 73 // 在雷达波模型坐标系下,对Z轴进行旋转,与连接原点指向轨迹点方向的矢量重合,计算出此旋转矩阵 74 osg::Matrixd rotMat = osg::Matrixd::rotate(osg::Z_AXIS, point2InRadarCoordinateSystem-osg::Vec3(0,0,0)); 75 // 将计算出的旋转矩阵赋给雷达波模型所在的mt 76 mt->setMatrix(rotMat); 77 } 78 79 int main(int argc, char *argv[]) 80 { 81 osgViewer::Viewer viewer; 82 std::string world_tif = "D:\\osgFiles\\world.tif"; 83 osgEarth::Map* map = new osgEarth::Map(); 84 // Start with a basemap imagery layer; we'll be using the GDAL driver 85 // to load a local GeoTIFF file: 86 osgEarth::Drivers::GDALOptions basemapOpt; 87 basemapOpt.url() = world_tif; 88 89 map->addLayer(new osgEarth::ImageLayer(osgEarth::ImageLayerOptions("basemap", basemapOpt))); 90 91 osgEarth::MapNodeOptions mapNodeOptions; 92 mapNodeOptions.enableLighting() = false; 93 osgEarth::MapNode* mapNode = new osgEarth::MapNode(map, mapNodeOptions); 94 95 osgEarth::Drivers::SimpleModelOptions opt; 96 opt.location() = osg::Vec3(118, 40, 10000); 97 //opt.url() = "cow.osg.1000,1000,1000.scale"; 98 osg::Geode* geode = new osg::Geode; 99 osg::ShapeDrawable* cone = new osg::ShapeDrawable(new osg::Cone(osg::Vec3(), 10000, 50000)); 100 //osg::ShapeDrawable* cone = new osg::ShapeDrawable(new osg::Box(osg::Vec3(), 50000)); 101 geode->addDrawable(cone); 102 osg::MatrixTransform* mtCone = new osg::MatrixTransform; 103 mtCone->addChild(geode); 104 opt.node() = mtCone; 105 map->addLayer(new osgEarth::ModelLayer("", opt)); 106 107 rotateCone(mtCone, map->getProfile()->getSRS(), osg::Vec3(118, 40, 100), osg::Vec3(120, 40, 100)); 108 109 osg::Group* root = new osg::Group(); 110 root->addChild(mapNode); 111 viewer.setSceneData(root); 112 viewer.setCameraManipulator(new osgEarth::Util::EarthManipulator()); 113 114 // Process cmdline args 115 //MapNodeHelper().parse(mapNode, arguments, &viewer, root, new LabelControl("Features Demo")); 116 117 //视点定位模型所在位置 118 osgEarth::Viewpoint vp("", 118, 40, 1000.0, -2.50, -90.0, 1.5e6); 119 (dynamic_cast<osgEarth::Util::EarthManipulator*>(viewer.getCameraManipulator()))->setViewpoint(vp); 120 121 // add some stock OSG handlers: 122 viewer.addEventHandler(new osgViewer::StatsHandler()); 123 viewer.addEventHandler(new osgViewer::WindowSizeHandler()); 124 viewer.addEventHandler(new osgGA::StateSetManipulator(viewer.getCamera()->getOrCreateStateSet())); 125 viewer.setUpViewInWindow(50,50,500,400); 126 return viewer.run(); 127 }
作者:疯狂Delphi
本文版权归作者和博客园共有,欢迎转载,但未经作者同意必须保留此段声明,且在文章页面明显位置给出原文连接,否则保留追究法律责任的权利.
欢迎关注我,一起进步!扫描下方二维码即可加我
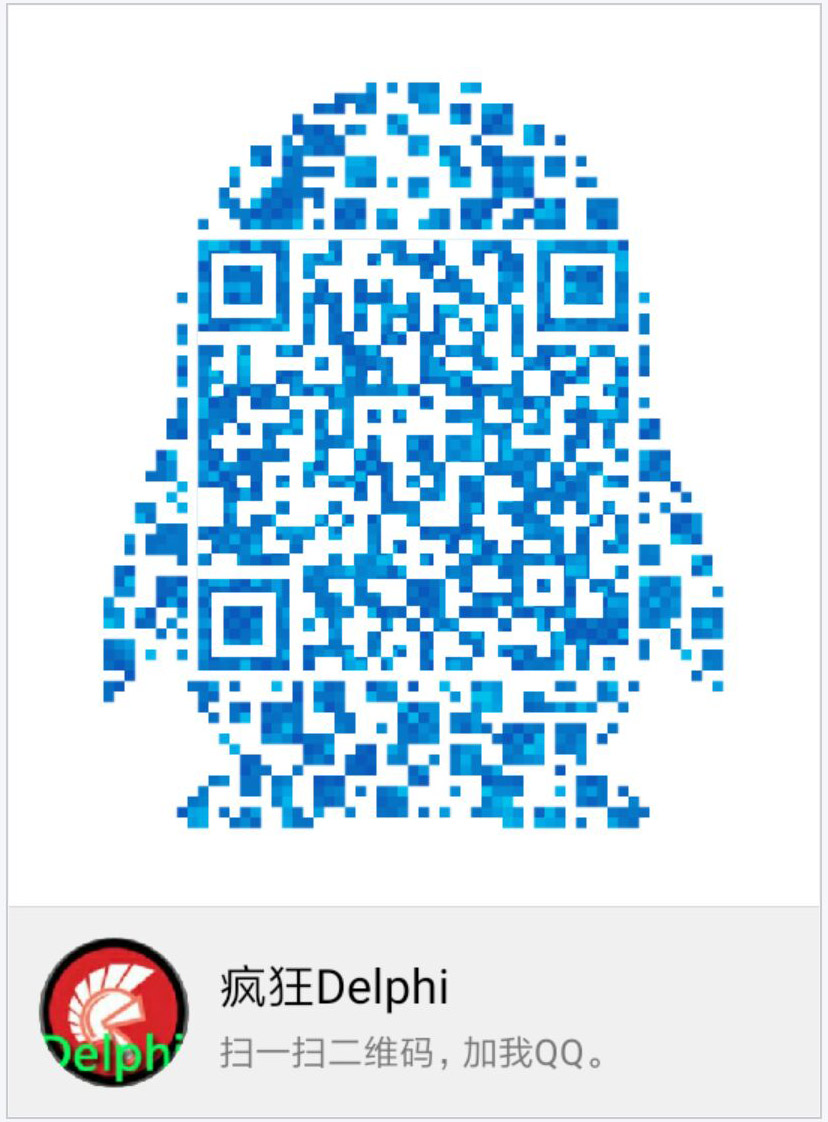
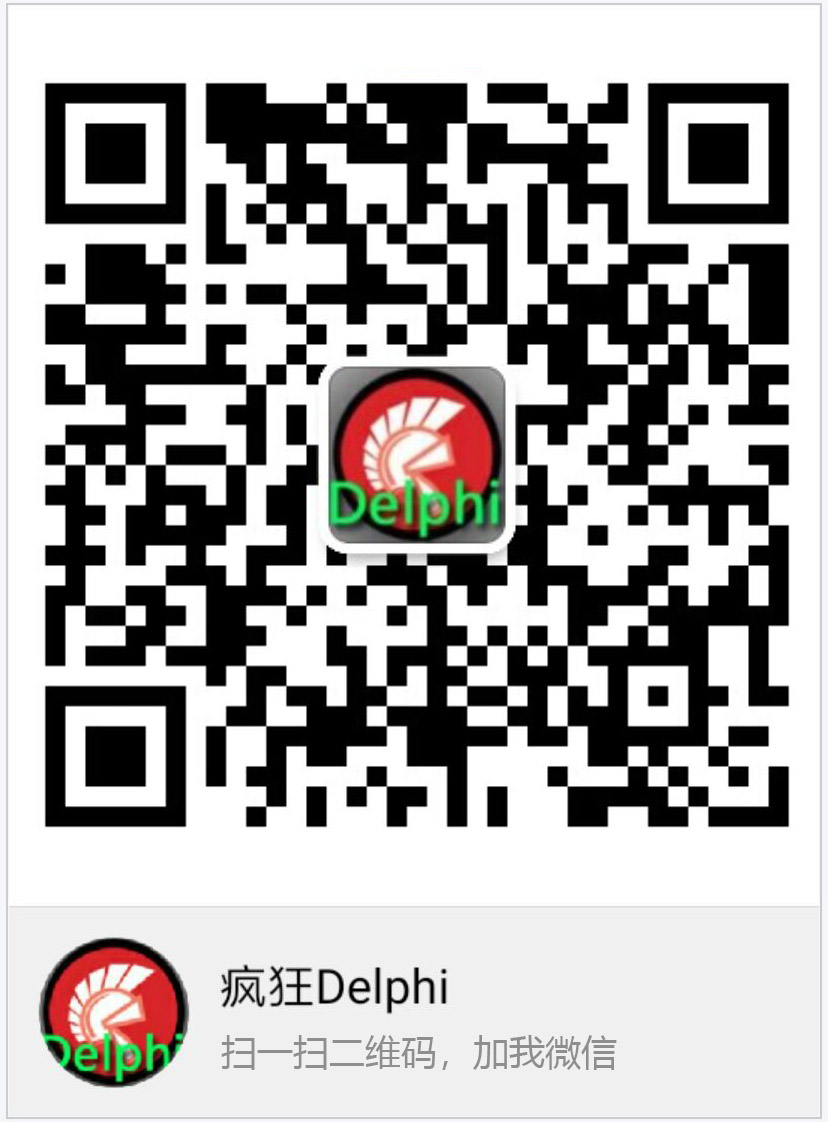