Osg-Osg实例实现环境雾效果(Qt5.14.2+osgEarht3.6.5+win10)-No5-EnvironmentFog
相关资料:
https://blog.csdn.net/wb175208/article/details/88692393 osg 雾效果
https://blog.csdn.net/xiaoyuwang1994/article/details/105921857/ 实现osgEarth三维仿真场景模型雾的效果的添加解决方法
代码实例:
.pro

1 QT += core gui widgets 2 TARGET = TestOsgQt 3 TEMPLATE = app 4 DEFINES += QT_DEPRECATED_WARNINGS 5 CONFIG += c++11 6 7 SOURCES += \ 8 main.cpp 9 10 HEADERS += 11 12 OsgDir = D:\\RuanJian\\osg365R 13 CONFIG(release, debug|release) { 14 LIBS += -L$${OsgDir}/lib/ -losgDB -losgViewer -lOpenThreads -losgAnimation -losg \ 15 -losgEarth -losgEarthAnnotation -losgEarthFeatures -losgEarthSymbology -losgEarthUtil \ 16 -losgQOpenGL -losgUtil -losgText -losgTerrain -losgSim \ 17 -losgShadow -losgParticle -losgManipulator -losgGA -losgFX \ 18 -losgWidget 19 } else { 20 LIBS += -L$${OsgDir}/debug/lib/ -losgDBd -losgViewerd -lOpenThreadsd -losgAnimationd -losgd \ 21 -losgEarthd -losgEarthAnnotationd -losgEarthFeaturesd -losgEarthSymbologyd -losgEarthUtild \ 22 -losgQOpenGLd -losgUtild -losgTextd -losgTerraind -losgSimd \ 23 -losgShadowd -losgParticled -losgManipulatord -losgGAd -losgFXd \ 24 } 25 26 27 INCLUDEPATH += $${OsgDir}/include 28 DEPENDPATH += $${OsgDir}/include
main.cpp

1 #include <QApplication> 2 3 #include <osg/Node> 4 #include <osg/Group> 5 #include <osg/Geode> 6 #include <osg/Geometry> 7 #include <osg/Texture2D> 8 #include <osg/StateSet> 9 #include <osg/PositionAttitudeTransform> 10 #include <osgViewer/Viewer> 11 #include <osgDB/ReadFile> 12 #include <osgParticle/PrecipitationEffect> 13 // 雨雪效果 14 #include <osg/MatrixTransform> 15 // 粒子效果 16 #include <osgParticle/PrecipitationEffect> 17 #include <osgParticle/Particle> 18 #include <osgParticle/LinearInterpolator> 19 #include <osgParticle/ParticleSystem> 20 #include <osgParticle/RandomRateCounter> 21 #include <osgParticle/PointPlacer> 22 #include <osgParticle/RadialShooter> 23 #include <osgParticle/ModularEmitter> 24 #include <osgParticle/ParticleSystemUpdater> 25 #include <osgParticle/ModularProgram> 26 #include <osgUtil/Optimizer> 27 #include <osgUtil/Simplifier> 28 // 雾 29 #include <osg/Fog> 30 #include <osgDB/ReadFile> 31 #include <osgViewer/Viewer> 32 #include <osg/StateSet> 33 #include <osg/StateAttribute> 34 #include <osgViewer/ViewerEventHandlers> 35 #include <osgWidget/ViewerEventHandlers> 36 37 class FogCallBack : public osg::StateSet::Callback { 38 public: 39 FogCallBack(); 40 ~FogCallBack(); 41 42 virtual void operator() (osg::StateSet* ss, osg::NodeVisitor*nv)override; 43 }; 44 45 FogCallBack::FogCallBack() { 46 } 47 48 49 FogCallBack::~FogCallBack() { 50 } 51 52 void FogCallBack::operator() (osg::StateSet* ss, osg::NodeVisitor*nv) { 53 osg::Fog* fog = dynamic_cast<osg::Fog*>(ss->getAttribute(osg::StateAttribute::FOG)); 54 if (fog){ 55 float start = fog->getStart(); 56 if (start<fog->getEnd()){ 57 fog->setStart(start + 2.0); 58 } 59 } 60 } 61 62 int main(int argc, char *argv[]) 63 { 64 // ---------------------雾----------------------- 65 osg::ref_ptr<osg::Group> root = new osg::Group; 66 osg::ref_ptr<osg::Node> cowNode = osgDB::readNodeFile("D:\\osgFiles\\lz.osg"); 67 root->addChild(cowNode); 68 69 osg::ref_ptr<osg::Fog> fog = new osg::Fog; 70 fog->setColor(osg::Vec4(1.0, 1.0, 1.0, 0.5));//设置颜色 71 fog->setStart(1.0);//设置雾效的近距离 72 fog->setEnd(2000.0);//设置雾效的远距离 73 fog->setDensity(10.0);//设置雾效的密度 74 fog->setMode(osg::Fog::LINEAR);//设置雾效的类型-线型 75 76 root->getOrCreateStateSet()->setUpdateCallback(new FogCallBack); 77 root->getOrCreateStateSet()->setAttributeAndModes(fog.get(), osg::StateAttribute::ON| osg::StateAttribute::OVERRIDE); 78 79 osg::ref_ptr<osgViewer::Viewer> viewer = new osgViewer::Viewer(); 80 viewer->addEventHandler(new osgViewer::WindowSizeHandler()); 81 viewer->addEventHandler(new osgViewer::StatsHandler()); 82 // **********************************设置窗口******************************* 83 //设置图形环境特性 84 osg::ref_ptr<osg::GraphicsContext::Traits> traits = new osg::GraphicsContext::Traits(); 85 traits->x = 100; 86 traits->y = 200; 87 traits->width = 600; 88 traits->height = 500; 89 traits->windowDecoration = true; 90 traits->doubleBuffer = true; 91 traits->sharedContext = 0; 92 // 创建图形环境特性 93 osg::ref_ptr<osg::GraphicsContext> gc = osg::GraphicsContext::createGraphicsContext(traits.get()); 94 if (gc.valid()) 95 { 96 osg::notify(osg::INFO)<<" GraphicsWindow has been created successfully."<<std::endl; 97 98 //清除窗口颜色及清除颜色和深度缓冲 99 gc->setClearColor(osg::Vec4f(0.2f,0.2f,0.6f,1.0f)); 100 gc->setClearMask(GL_COLOR_BUFFER_BIT | GL_DEPTH_BUFFER_BIT); 101 } 102 else 103 { 104 osg::notify(osg::NOTICE)<<" GraphicsWindow has not been created successfully."<<std::endl; 105 } 106 //设置视口 107 viewer->getCamera()->setViewport(new osg::Viewport(0,0, traits->width, traits->height)); 108 //设置图形环境 109 viewer->getCamera()->setGraphicsContext(gc.get()); 110 viewer->setSceneData( root ); 111 return viewer->run(); 112 }
作者:疯狂Delphi
本文版权归作者和博客园共有,欢迎转载,但未经作者同意必须保留此段声明,且在文章页面明显位置给出原文连接,否则保留追究法律责任的权利.
欢迎关注我,一起进步!扫描下方二维码即可加我
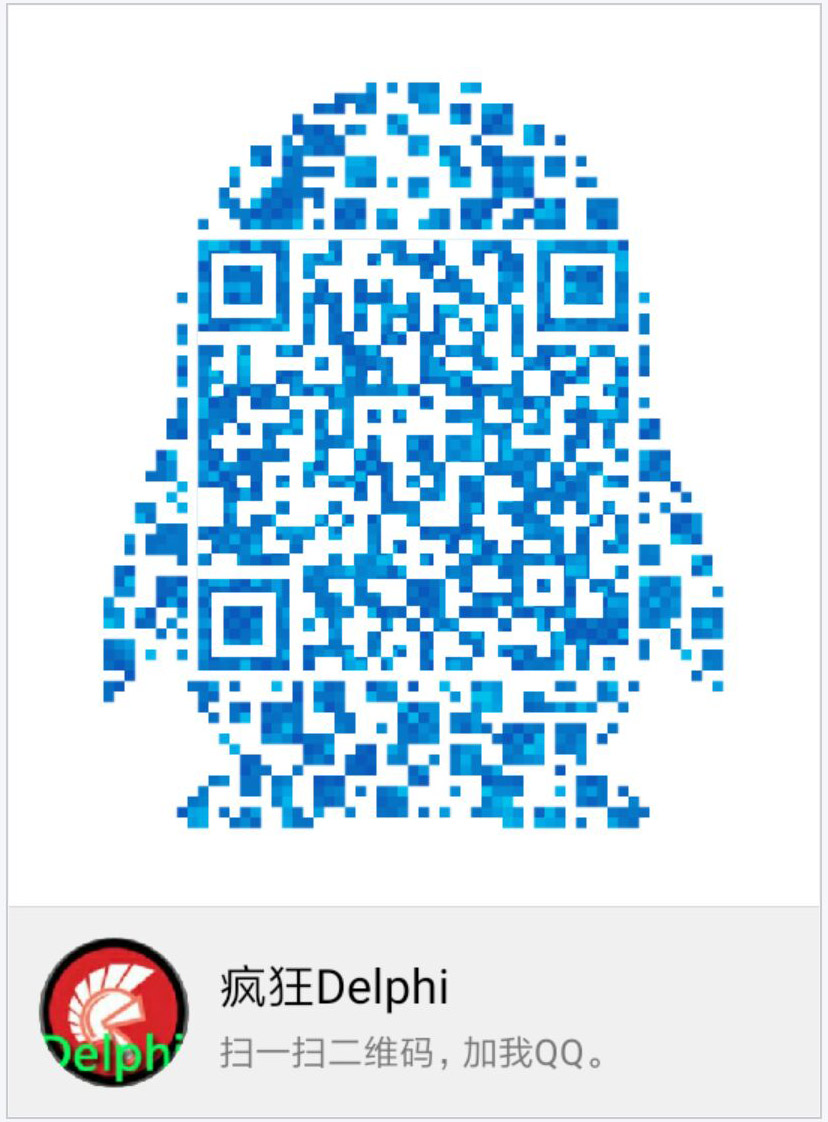
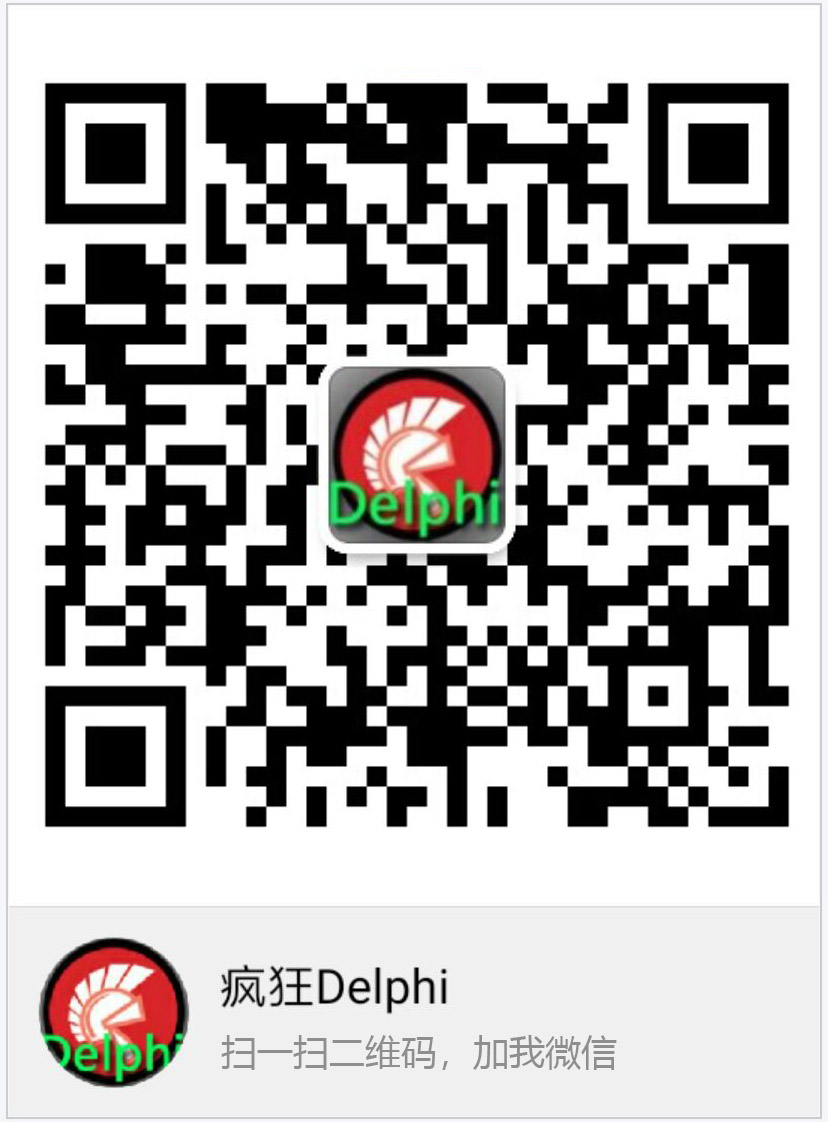