Qt-Qt在WIN10上实现特效(着色、模糊、投影、透明)
相关资料:
https://blog.51cto.com/11496263/1866763 4种效果英文说明
https://download.csdn.net/download/zhujianqiangqq/13092433 代码包下载
.pro

1 QT += core gui 2 3 greaterThan(QT_MAJOR_VERSION, 4): QT += widgets 4 5 CONFIG += c++11 6 7 # The following define makes your compiler emit warnings if you use 8 # any Qt feature that has been marked deprecated (the exact warnings 9 # depend on your compiler). Please consult the documentation of the 10 # deprecated API in order to know how to port your code away from it. 11 DEFINES += QT_DEPRECATED_WARNINGS 12 13 # You can also make your code fail to compile if it uses deprecated APIs. 14 # In order to do so, uncomment the following line. 15 # You can also select to disable deprecated APIs only up to a certain version of Qt. 16 #DEFINES += QT_DISABLE_DEPRECATED_BEFORE=0x060000 # disables all the APIs deprecated before Qt 6.0.0 17 18 SOURCES += \ 19 main.cpp \ 20 mainwindow.cpp 21 22 HEADERS += \ 23 mainwindow.h 24 25 FORMS += \ 26 mainwindow.ui 27 28 # Default rules for deployment. 29 qnx: target.path = /tmp/$${TARGET}/bin 30 else: unix:!android: target.path = /opt/$${TARGET}/bin 31 !isEmpty(target.path): INSTALLS += target 32 33 RESOURCES +=
main.cpp

1 #include "mainwindow.h" 2 3 #include <QApplication> 4 5 int main(int argc, char *argv[]) 6 { 7 QApplication a(argc, argv); 8 MainWindow w; 9 w.show(); 10 return a.exec(); 11 }
mainWindow.h

1 #ifndef MAINWINDOW_H 2 #define MAINWINDOW_H 3 4 #include <QMainWindow> 5 6 #include <QHBoxLayout> 7 #include <QPushButton> 8 #include <QPainter> 9 #include <QStyle> 10 #include <QStyleOption> 11 #include <QGraphicsOpacityEffect> 12 #include <QDebug> 13 #include <QTimer> 14 15 QT_BEGIN_NAMESPACE 16 namespace Ui { class MainWindow; } 17 QT_END_NAMESPACE 18 19 class myWidget: public QWidget 20 { 21 Q_OBJECT 22 public: 23 myWidget(QWidget* parent = nullptr); 24 ~myWidget(); 25 26 void setGraphicsColorizeEffect(); 27 void setGraphicsBlurEffect(); 28 void setGraphicsDropShadowEffect(); 29 void setGraphicsOpacityEffect(); 30 protected: 31 virtual void paintEvent(QPaintEvent* pEvent) override; 32 private: 33 QHBoxLayout *m_pHl; 34 QPushButton *m_pPb; 35 }; 36 37 class MainWindow : public QMainWindow 38 { 39 Q_OBJECT 40 41 public: 42 MainWindow(QWidget *parent = nullptr); 43 ~MainWindow(); 44 protected: 45 virtual void paintEvent(QPaintEvent* pEvent) override; 46 private slots: 47 void on_pushButton_clicked(); 48 void on_pushButton_2_clicked(); 49 void on_pushButton_3_clicked(); 50 void on_pushButton_4_clicked(); 51 private: 52 Ui::MainWindow *ui; 53 myWidget *m_pWidget; 54 }; 55 #endif // MAINWINDOW_H
mainWindow.cpp

1 #include "mainwindow.h" 2 #include "ui_mainwindow.h" 3 4 MainWindow::MainWindow(QWidget *parent) 5 : QMainWindow(parent) 6 , ui(new Ui::MainWindow) 7 { 8 ui->setupUi(this); 9 10 m_pWidget = new myWidget(this); 11 m_pWidget->setGeometry(100, 30, 300, 200); 12 } 13 14 MainWindow::~MainWindow() 15 { 16 delete ui; 17 } 18 19 void MainWindow::paintEvent(QPaintEvent *pEvent) 20 { 21 // 界面背景图片片 22 QPainter painter(this); 23 painter.drawPixmap(0, 0, this->width(), this->height(), QPixmap("D:/Image/12954363.jpg")); 24 } 25 26 27 myWidget::myWidget(QWidget *parent) 28 : QWidget(parent) 29 { 30 m_pHl = new QHBoxLayout(this); 31 this->setLayout(m_pHl); 32 33 m_pPb = new QPushButton(this); 34 m_pPb->setText("132"); 35 36 m_pHl->addWidget(m_pPb); 37 } 38 39 myWidget::~myWidget() 40 { 41 42 } 43 44 void myWidget::setGraphicsColorizeEffect() 45 { 46 QGraphicsColorizeEffect *e1 = new QGraphicsColorizeEffect(this); 47 e1->setColor(QColor(0,0,192)); 48 this->setGraphicsEffect(e1); 49 } 50 51 void myWidget::setGraphicsBlurEffect() 52 { 53 QGraphicsBlurEffect *e0 = new QGraphicsBlurEffect(this); 54 e0->setBlurRadius(10); 55 this->setGraphicsEffect(e0); 56 } 57 58 void myWidget::setGraphicsDropShadowEffect() 59 { 60 QGraphicsDropShadowEffect *e2 = new QGraphicsDropShadowEffect(this); 61 e2->setOffset(8,8); 62 this->setGraphicsEffect(e2); 63 } 64 65 void myWidget::setGraphicsOpacityEffect() 66 { 67 QGraphicsOpacityEffect *e4 = new QGraphicsOpacityEffect(this); 68 e4->setOpacity(0.4); 69 this->setGraphicsEffect(e4); 70 } 71 72 void myWidget::paintEvent(QPaintEvent *pEvent) 73 { 74 // 界面背景图片片 75 QPainter painter(this); 76 painter.drawPixmap(0, 0, this->width(), this->height(), QPixmap("D:/Image/1.jpg")); 77 } 78 79 void MainWindow::on_pushButton_clicked() 80 { 81 m_pWidget->setGraphicsColorizeEffect(); 82 } 83 84 void MainWindow::on_pushButton_2_clicked() 85 { 86 m_pWidget->setGraphicsBlurEffect(); 87 } 88 89 void MainWindow::on_pushButton_3_clicked() 90 { 91 m_pWidget->setGraphicsDropShadowEffect(); 92 } 93 94 void MainWindow::on_pushButton_4_clicked() 95 { 96 m_pWidget->setGraphicsOpacityEffect(); 97 }
mainWindow.ui

1 <?xml version="1.0" encoding="UTF-8"?> 2 <ui version="4.0"> 3 <class>MainWindow</class> 4 <widget class="QMainWindow" name="MainWindow"> 5 <property name="geometry"> 6 <rect> 7 <x>0</x> 8 <y>0</y> 9 <width>557</width> 10 <height>309</height> 11 </rect> 12 </property> 13 <property name="windowTitle"> 14 <string>MainWindow</string> 15 </property> 16 <widget class="QWidget" name="centralwidget"> 17 <widget class="QPushButton" name="pushButton"> 18 <property name="geometry"> 19 <rect> 20 <x>20</x> 21 <y>10</y> 22 <width>80</width> 23 <height>20</height> 24 </rect> 25 </property> 26 <property name="text"> 27 <string>着色</string> 28 </property> 29 </widget> 30 <widget class="QPushButton" name="pushButton_2"> 31 <property name="geometry"> 32 <rect> 33 <x>20</x> 34 <y>50</y> 35 <width>80</width> 36 <height>20</height> 37 </rect> 38 </property> 39 <property name="text"> 40 <string>模糊</string> 41 </property> 42 </widget> 43 <widget class="QPushButton" name="pushButton_3"> 44 <property name="geometry"> 45 <rect> 46 <x>20</x> 47 <y>90</y> 48 <width>80</width> 49 <height>20</height> 50 </rect> 51 </property> 52 <property name="text"> 53 <string>投影</string> 54 </property> 55 </widget> 56 <widget class="QPushButton" name="pushButton_4"> 57 <property name="geometry"> 58 <rect> 59 <x>20</x> 60 <y>130</y> 61 <width>80</width> 62 <height>20</height> 63 </rect> 64 </property> 65 <property name="text"> 66 <string>透明</string> 67 </property> 68 </widget> 69 </widget> 70 <widget class="QMenuBar" name="menubar"> 71 <property name="geometry"> 72 <rect> 73 <x>0</x> 74 <y>0</y> 75 <width>557</width> 76 <height>22</height> 77 </rect> 78 </property> 79 </widget> 80 <widget class="QStatusBar" name="statusbar"/> 81 </widget> 82 <resources/> 83 <connections/> 84 </ui>
作者:疯狂Delphi
本文版权归作者和博客园共有,欢迎转载,但未经作者同意必须保留此段声明,且在文章页面明显位置给出原文连接,否则保留追究法律责任的权利.
欢迎关注我,一起进步!扫描下方二维码即可加我
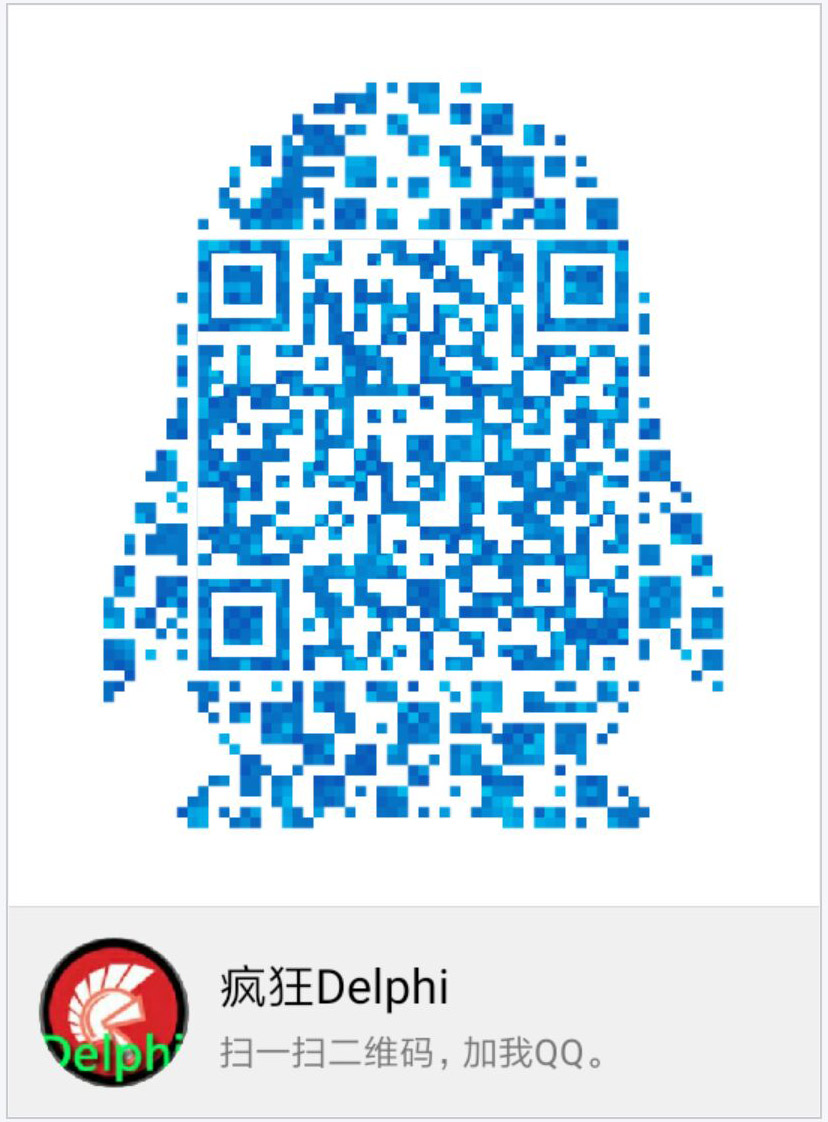
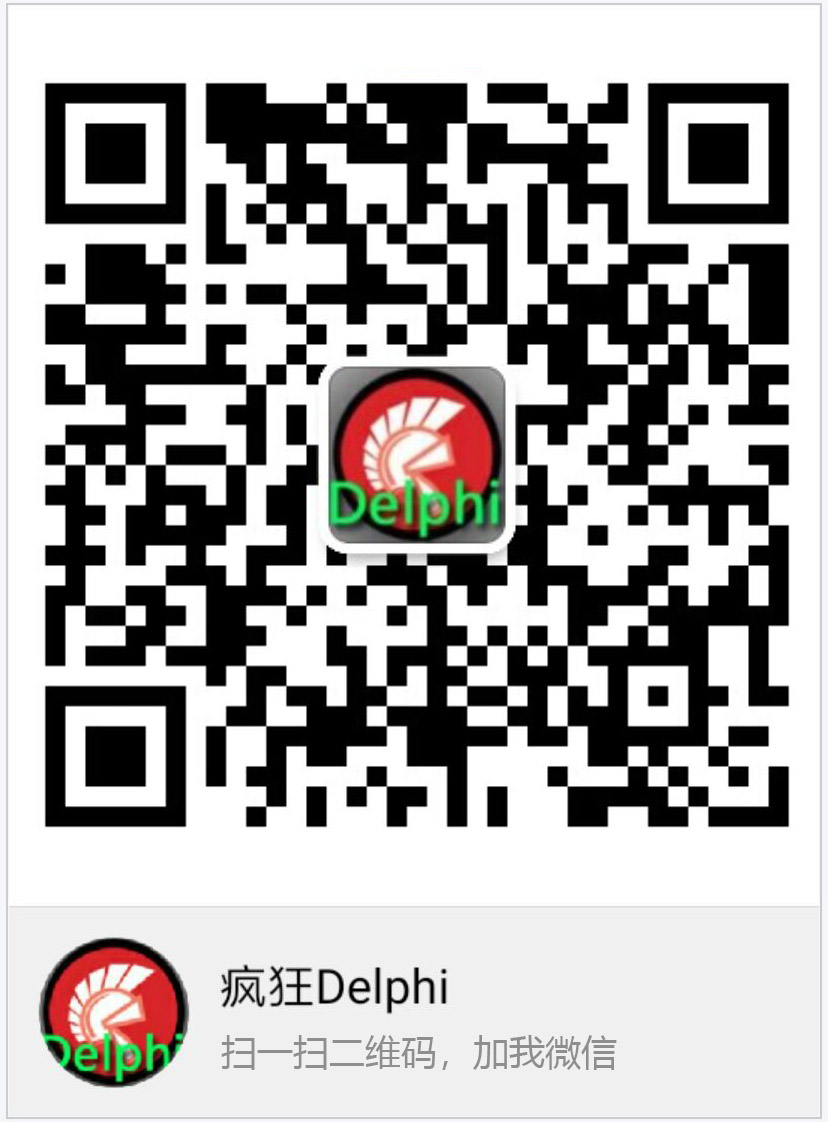