Qt操作XML文件
PS:需要在工程文件.pro中增加xml
.pro

QT += core xml QT -= gui TARGET = xmltest CONFIG += console CONFIG -= app_bundle TEMPLATE = app SOURCES += main.cpp
main.cpp

1 #include <QtXml> 2 #include <QFile> 3 #include <windows.h> 4 5 //写xml 6 void WriteXml() 7 { 8 //打开或创建文件 9 QFile file("test.xml"); //相对路径、绝对路径、资源路径都可以 10 if(!file.open(QFile::WriteOnly|QFile::Truncate)) //可以用QIODevice,Truncate表示清空原来的内容 11 return; 12 13 QDomDocument doc; 14 //写入xml头部 15 QDomProcessingInstruction instruction; //添加处理命令 16 instruction=doc.createProcessingInstruction("xml","version=\"1.0\" encoding=\"UTF-8\""); 17 doc.appendChild(instruction); 18 //添加根节点 19 QDomElement root=doc.createElement("library"); 20 doc.appendChild(root); 21 //添加第一个子节点及其子元素 22 QDomElement book=doc.createElement("book"); 23 book.setAttribute("id",1); //方式一:创建属性 其中键值对的值可以是各种类型 24 QDomAttr time=doc.createAttribute("time"); //方式二:创建属性 值必须是字符串 25 time.setValue("2013/6/13"); 26 book.setAttributeNode(time); 27 QDomElement title=doc.createElement("title"); //创建子元素 28 QDomText text; //设置括号标签中间的值 29 text=doc.createTextNode("C++ primer"); 30 book.appendChild(title); 31 title.appendChild(text); 32 QDomElement author=doc.createElement("author"); //创建子元素 33 text=doc.createTextNode("Stanley Lippman"); 34 author.appendChild(text); 35 book.appendChild(author); 36 root.appendChild(book); 37 38 //添加第二个子节点及其子元素,部分变量只需重新赋值 39 book=doc.createElement("book"); 40 book.setAttribute("id",2); 41 time=doc.createAttribute("time"); 42 time.setValue("2007/5/25"); 43 book.setAttributeNode(time); 44 title=doc.createElement("title"); 45 text=doc.createTextNode("Thinking in Java"); 46 book.appendChild(title); 47 title.appendChild(text); 48 author=doc.createElement("author"); 49 text=doc.createTextNode("Bruce Eckel"); 50 author.appendChild(text); 51 book.appendChild(author); 52 root.appendChild(book); 53 54 // 输出到文件 55 QTextStream out_stream(&file); 56 doc.save(out_stream,4); //缩进4格 57 file.close(); 58 } 59 60 // string保存成XML文件 61 void StringToXml() 62 { 63 // 不加fromLocal8Bit这一句中文会乱码 64 QString ss = QString::fromLocal8Bit("<vs> <Title>test¥</Title> <A>you hao</A></vs>"); 65 QDomDocument doc; 66 if(!doc.setContent(ss)) 67 { 68 return; 69 } 70 71 QString folder = "E:/file"; 72 QDir dir; 73 if (!dir.exists(folder)) 74 { 75 dir.mkpath(folder); 76 } 77 78 QString str; 79 QTextStream stream(&str); 80 QDomElement root = doc.documentElement(); //返回根节点 81 root.save(stream, QDomElement::CDATASectionNode); 82 QFile ttfile("E:/file/xmlTest.xml"); 83 if (!ttfile.open(QFile::WriteOnly | QFile::Text)) 84 { 85 } 86 QTextStream out(&ttfile); 87 out.setCodec("UTF-8");// 不加这一句中文会乱码 88 out << str; 89 qDebug() << str; 90 } 91 92 // 读xml后保存成string 93 void XmlToStr() 94 { 95 //打开或创建文件 96 QFile file("E:/file/xmlTest.xml"); //相对路径、绝对路径、资源路径都可以 97 if(!file.open(QFile::ReadOnly)) 98 return; 99 100 QDomDocument doc; 101 if(!doc.setContent(&file)) 102 { 103 file.close(); 104 return; 105 } 106 107 QString str; 108 QTextStream stream(&str); 109 QDomElement root = doc.documentElement(); //返回根节点 110 root.save(stream, QDomElement::CDATASectionNode); 111 QFile ttfile("E:/file/txtTest.txt"); 112 if (!ttfile.open(QFile::WriteOnly | QFile::Text | QIODevice::Append)) 113 { 114 return; 115 } 116 QTextStream out(&ttfile); 117 out << str; 118 qDebug() << str; 119 } 120 121 //读xml 122 void ReadXml() 123 { 124 //打开或创建文件 125 QFile file("test.xml"); //相对路径、绝对路径、资源路径都行 126 if(!file.open(QFile::ReadOnly)) 127 return; 128 129 QDomDocument doc; 130 if(!doc.setContent(&file)) 131 { 132 file.close(); 133 return; 134 } 135 file.close(); 136 QDomElement root=doc.documentElement(); //返回根节点 137 qDebug()<<root.nodeName(); 138 QDomNode node=root.firstChild(); //获得第一个子节点 139 while(!node.isNull()) //如果节点不空 140 { 141 if(node.isElement()) //如果节点是元素 142 { 143 QDomElement e=node.toElement(); //转换为元素,注意元素和节点是两个数据结构,其实差不多 144 qDebug()<<e.text()<< e.tagName()<<" "<<e.attribute("id")<<" "<<e.attribute("time"); //打印键值对,tagName和nodeName是一个东西 145 QDomNodeList list=e.childNodes(); 146 qDebug() <<"count:" << list.count(); 147 for(int i=0;i<list.count();i++) //遍历子元素,count和size都可以用,可用于标签数计数 148 { 149 QDomNode n=list.at(i); 150 if(n.isElement()) 151 { 152 QDomNodeList nList=n.childNodes(); 153 for(int j = 0; j < nList.count(); j++) 154 { 155 QDomNode nn = nList.at(j); 156 qDebug()<<nn.nodeName()<<":"<<nn.toElement().text(); 157 } 158 } 159 } 160 } 161 node=node.nextSibling(); //下一个兄弟节点,nextSiblingElement()是下一个兄弟元素,都差不多 162 } 163 } 164 165 //增加xml内容 166 void AddXml() 167 { 168 //打开文件 169 QFile file("test.xml"); //相对路径、绝对路径、资源路径都可以 170 if(!file.open(QFile::ReadOnly)) 171 return; 172 173 //增加一个一级子节点以及元素 174 QDomDocument doc; 175 if(!doc.setContent(&file)) 176 { 177 file.close(); 178 return; 179 } 180 file.close(); 181 182 QDomElement root=doc.documentElement(); 183 QDomElement book=doc.createElement("book"); 184 book.setAttribute("id",3); 185 book.setAttribute("time","1813/1/27"); 186 QDomElement title=doc.createElement("title"); 187 QDomText text; 188 text=doc.createTextNode("Pride and Prejudice"); 189 title.appendChild(text); 190 book.appendChild(title); 191 QDomElement author=doc.createElement("author"); 192 text=doc.createTextNode("Jane Austen"); 193 author.appendChild(text); 194 book.appendChild(author); 195 root.appendChild(book); 196 197 if(!file.open(QFile::WriteOnly|QFile::Truncate)) //先读进来,再重写,如果不用truncate就是在后面追加内容,就无效了 198 return; 199 //输出到文件 200 QTextStream out_stream(&file); 201 doc.save(out_stream,4); //缩进4格 202 file.close(); 203 } 204 205 //删减xml内容 206 void RemoveXml() 207 { 208 //打开文件 209 QFile file("test.xml"); //相对路径、绝对路径、资源路径都可以 210 if(!file.open(QFile::ReadOnly)) 211 return; 212 213 //删除一个一级子节点及其元素,外层节点删除内层节点于此相同 214 QDomDocument doc; 215 if(!doc.setContent(&file)) 216 { 217 file.close(); 218 return; 219 } 220 file.close(); //一定要记得关掉啊,不然无法完成操作 221 222 QDomElement root=doc.documentElement(); 223 QDomNodeList list=doc.elementsByTagName("book"); //由标签名定位 224 for(int i=0;i<list.count();i++) 225 { 226 QDomElement e=list.at(i).toElement(); 227 if(e.attribute("time")=="2007/5/25") //以属性名定位,类似于hash的方式,warning:这里仅仅删除一个节点,其实可以加个break 228 root.removeChild(list.at(i)); 229 } 230 231 if(!file.open(QFile::WriteOnly|QFile::Truncate)) 232 return; 233 //输出到文件 234 QTextStream out_stream(&file); 235 doc.save(out_stream,4); //缩进4格 236 file.close(); 237 } 238 239 //更新xml内容 240 void UpdateXml() 241 { 242 //打开文件 243 QFile file("test.xml"); //相对路径、绝对路径、资源路径都可以 244 if(!file.open(QFile::ReadOnly)) 245 return; 246 247 //更新一个标签项,如果知道xml的结构,直接定位到那个标签上定点更新 248 //或者用遍历的方法去匹配tagname或者attribut,value来更新 249 QDomDocument doc; 250 if(!doc.setContent(&file)) 251 { 252 file.close(); 253 return; 254 } 255 file.close(); 256 257 QDomElement root=doc.documentElement(); 258 QDomNodeList list=root.elementsByTagName("book"); 259 QDomNode node=list.at(list.size()-1).firstChild(); //定位到第三个一级子节点的子元素 260 QDomNode oldnode=node.firstChild(); //标签之间的内容作为节点的子节点出现,当前是Pride and Projudice 261 node.firstChild().setNodeValue("Emma"); 262 QDomNode newnode=node.firstChild(); 263 node.replaceChild(newnode,oldnode); 264 265 if(!file.open(QFile::WriteOnly|QFile::Truncate)) 266 return; 267 //输出到文件 268 QTextStream out_stream(&file); 269 doc.save(out_stream,4); //缩进4格 270 file.close(); 271 } 272 273 274 int main(int argc, char *argv[]) 275 { 276 // WriteXml(); 277 // qDebug()<<"write xml to file test.xml"; 278 279 StringToXml(); 280 qDebug()<<"string to xml file..."; 281 282 // XmlToStr(); 283 // qDebug()<<"Xml to string file..."; 284 285 // qDebug()<<"read xml to display..."; 286 // ReadXml(); 287 288 // qDebug()<<"add contents to xml..."; 289 // AddXml(); 290 291 // qDebug()<<"remove contents from xml..."; 292 // RemoveXml(); 293 294 // qDebug()<<"update contents to xml..."; 295 // UpdateXml(); 296 }
删除节点和增加节点
//更新xml内容 void UpdateXml() { //打开文件 QFile file("D:/QtDemo/QtXMLOK/test.xml"); //相对路径、绝对路径、资源路径都可以 if(!file.open(QFile::ReadOnly)) return; //更新一个标签项,如果知道xml的结构,直接定位到那个标签上定点更新 //或者用遍历的方法去匹配tagname或者attribut,value来更新 QDomDocument doc; if(!doc.setContent(&file)) { file.close(); return; } file.close(); QDomElement root = doc.documentElement(); QDomNodeList list = root.elementsByTagName(QStringLiteral("基本信息")); //// QDomNode node = list.at(list.size()-1).firstChild(); //定位到第三个一级子节点的子元素 // QDomNode oldnode = node.firstChild(); //标签之间的内容作为节点的子节点出现,当前是Pride and Projudice // node.firstChild().setNodeValue("Emma"); // QDomNode newnode=node.firstChild(); // node.replaceChild(newnode,oldnode); //删除方式一,通过标签book后面的属性删除<book>到</book> // for(int i=0;i<list.count();i++) // { // //转化为元素 // QDomElement e=list.at(i).toElement(); // //找到time是2007/5/25这一条数据将其删除 //// if(e.attribute("time")=="2013/6/13") // root.removeChild(list.at(i)); // } for(int i=list.count()-1;i>=0;i--) { //转化为元素 QDomElement e=list.at(i).toElement(); //找到time是2007/5/25这一条数据将其删除 // if(e.attribute("time")=="2013/6/13") root.removeChild(list.at(i)); } //创建next子节点book QDomElement book = doc.createElement("book"); book.setAttribute("id",3); book.setAttribute("time","1813/1/27"); // QDomElement title = doc.createElement("title"); // QDomText text; // text = doc.createTextNode("Pride and Prejudice"); //添加text内容到title节点 // title.appendChild(text); //添加title到book节点 // book.appendChild(title); //添加book到根节点 root.appendChild(book); if(!file.open(QFile::WriteOnly|QFile::Truncate)) return; //输出到文件 QTextStream out_stream(&file); doc.save(out_stream,4); //缩进4格 file.close(); }
作者:疯狂Delphi
本文版权归作者和博客园共有,欢迎转载,但未经作者同意必须保留此段声明,且在文章页面明显位置给出原文连接,否则保留追究法律责任的权利.
欢迎关注我,一起进步!扫描下方二维码即可加我
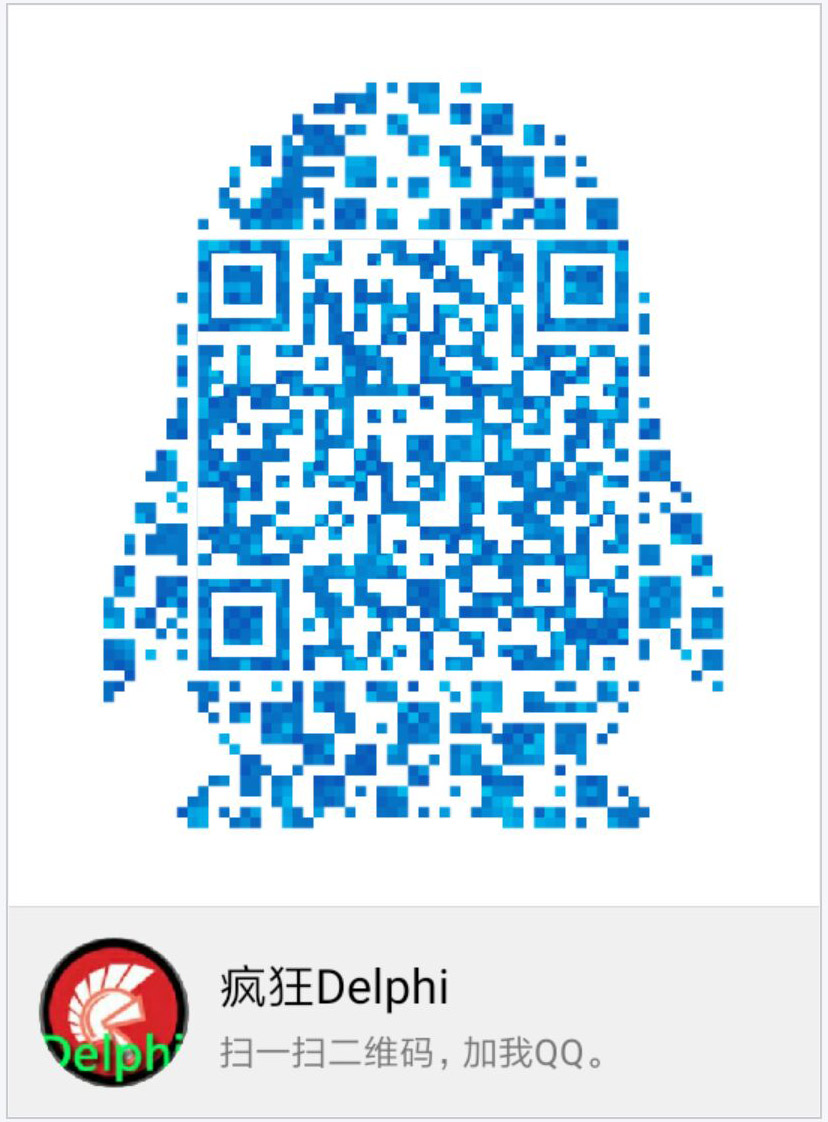
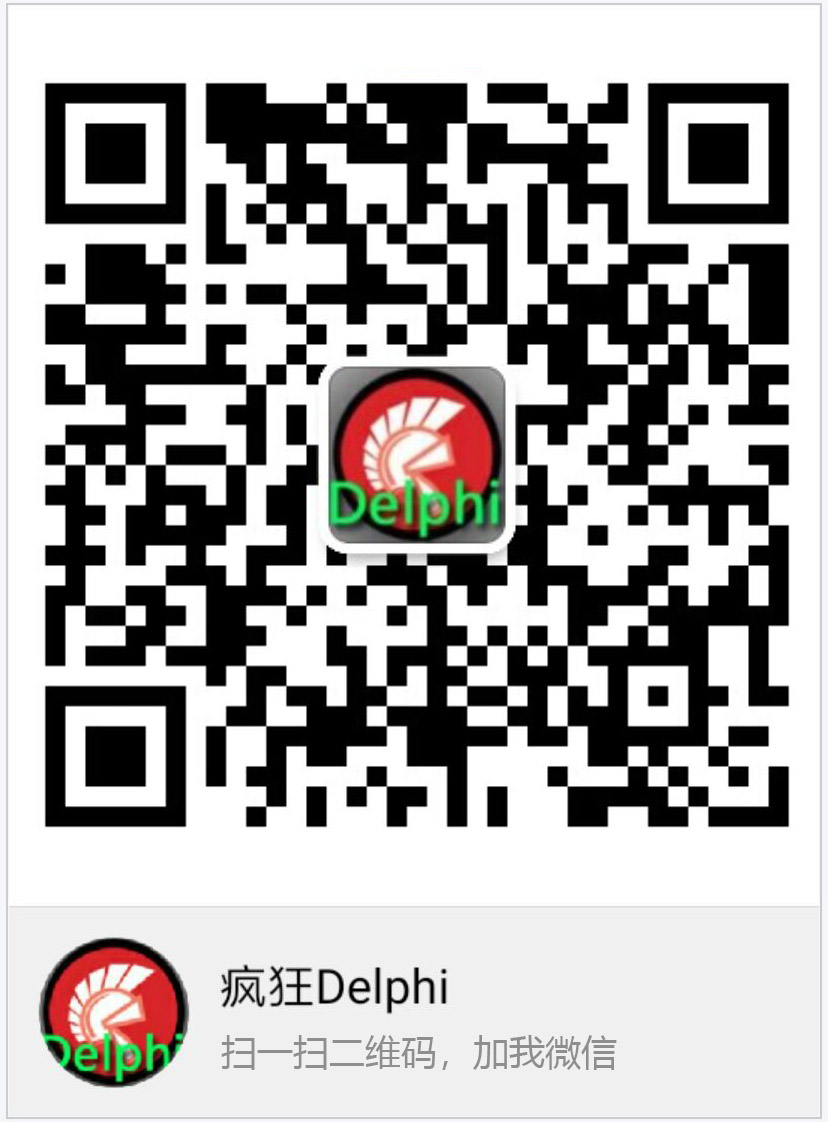