public class TestHashMap { Map<String, Integer> tt = new HashMap<String, Integer>(); tt.put("aaa", 1); tt.put("bbb", 2); tt.put("ccc", 3); tt.put("ddd", 4); Set<Entry<String, Integer>> temp = tt.entrySet(); Iterator<Entry<String, Integer>> it = temp.iterator(); while (it.hasNext()) { Entry<String, Integer> entry = it.next(); System.out.println(entry.getKey() + " " + entry.getValue()); } } import java.util.HashMap; import java.util.Iterator; import java.util.Map; import java.util.Set; public class Student { private String name; private int id; public Student(int id, String name) { super(); this.name = name; this.id = id; } public String getName() { return name; } public void setName(String name) { this.name = name; } public int getId() { return id; } public void setId(int id) { this.id = id; } public static void main(String[] args) { Student st1 = new Student(0001, "张三"); Student st2 = new Student(0002, "李四"); Student st3 = new Student(0003, "王五"); Map<Integer, String> map = new HashMap<Integer, String>(); map.put(st1.getId(), st1.getName()); map.put(st2.getId(), st2.getName()); map.put(st3.getId(), st3.getName()); Set<Integer> set = map.keySet(); Iterator<Integer> it = set.iterator(); while (it.hasNext()) { int i = it.next(); String str = map.get(i); System.out.println("ID: " + i + "\t" + "姓名:" + str); } } }
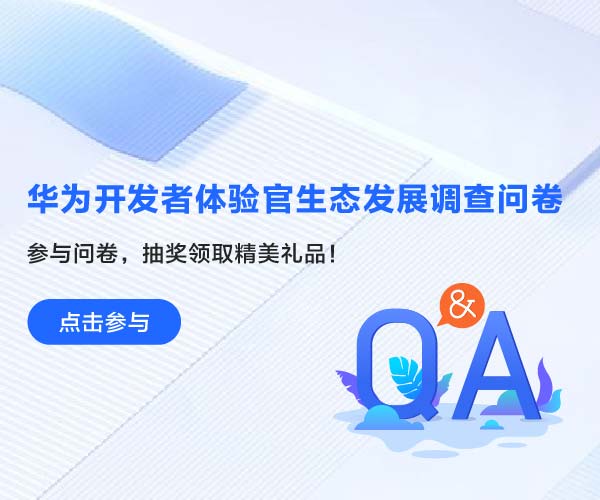
