LeetCode问题4
Median of Two Sorted Arrays
问题描述如下:
There are two sorted arrays nums1 and nums2 of size m and n respectively.
Find the median of the two sorted arrays. The overall run time complexity should be O(log (m+n)).
Example 1:
nums1 = [1, 3] nums2 = [2] The median is 2.0
Example 2:
nums1 = [1, 2] nums2 = [3, 4] The median is (2 + 3)/2 = 2.5
很遗憾,我这个题不会做,怎么想都想不出怎么在Log(m+n)的复杂度下完成任务,看了Solution才发现其实并没有想像中很巧妙很难想的解法。
这里直接把Solution的核心思想放上吧:
两个序列分别分为左右两个部分,由于每个序列都已经按照从大到小的顺序排好,因此左边的序列的最大值与右边序列的最小值的平均值为所求结果。
因此问题转换成如何保证左右两边的序列长度相同,Solution巧妙的地方在于设定i以后,j可以通过计算得到,这样只有一个未知量,只需要确定i即可。
如何在Log(m+n)的复杂度下确定i,很自然的想法是二分搜索。Solution就是在这一核心思路下进行的,当然还涉及m和n的大小,当i或者j取到0如何处理的问题,不过总体思路有了这些都还容易解决。
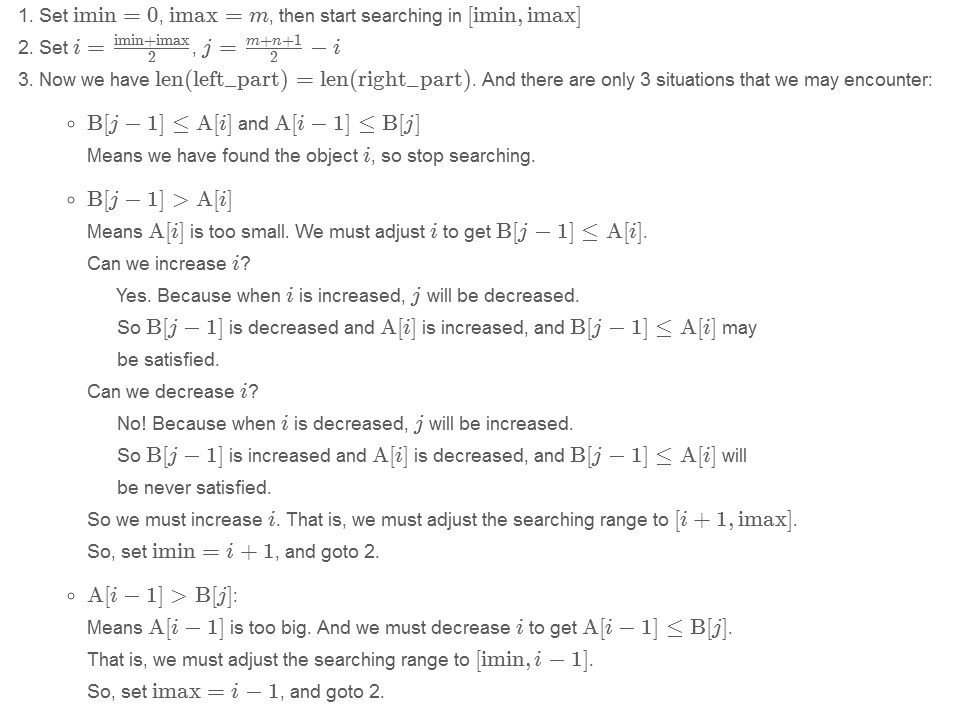
程序步骤如上,根据程序步骤,参考Solution完成代码如下:
class Solution { public double findMedianSortedArrays(int[] A, int[] B) { int m=A.length,n=B.length; if (m > n) { int[] temp = A; A = B; B = temp; int tmp = m; m = n; n = tmp; } int imin=0,imax=m; int stopflag=1,i=0,j=0,half=(m+n+1)/2; while(stopflag==1) { i=(imin+imax)/2; j=half-i; System.out.println("i"+i); System.out.println("j"+j); if((i==0||j==n||A[i-1]<=B[j])&&(i==m||j==0||B[j-1]<=A[i])) { stopflag=0; int maxLeft = 0; if (i == 0) { maxLeft = B[j-1]; } else if (j == 0) { maxLeft = A[i-1]; } else { maxLeft = Math.max(A[i-1], B[j-1]); } if ( (m + n) % 2 == 1 ) { return maxLeft; } int minRight = 0; if (i == m) { minRight = B[j]; } else if (j == n) { minRight = A[i]; } else { minRight = Math.min(B[j], A[i]); } return (maxLeft + minRight) / 2.0; } else if((i<imax)&&(B[j-1]>A[i])) imin++; else if((i>imin)&&(A[i-1]>B[j])) imax--; else stopflag=0; } return 0.0; } }
很遗憾,第一个遇到的Hard问题就不会,和CS专业的差距还是大的不可思议的。