1 #include<cstdio> 2 #include<cstring> 3 #include<vector> 4 #include<algorithm> 5 typedef __int64 LL; 6 using namespace std; 7 #define MAXN 1<<11 8 #define MAXM 15 9 int n,m; 10 vector<int>G[MAXN]; 11 LL dp[MAXM][MAXN]; 12 bool OK(int a,int b) 13 { 14 int i,temp,cnt; 15 temp=a|b; 16 for(i=0;i<m;i++) 17 { 18 if(!(temp&(1<<i))) 19 return false; 20 } 21 temp=a&b; 22 for(i=cnt=0;i<m;i++) 23 { 24 if(temp&(1<<i)) 25 cnt++; 26 else 27 { 28 if(cnt&1) 29 return false; 30 cnt=0; 31 } 32 } 33 return !(cnt&1); 34 } 35 void DoIt() 36 { 37 int i,j,k; 38 memset(dp,0,sizeof(dp)); 39 dp[0][(1<<m)-1]=1; 40 for(i=1;i<=n+1;i++) 41 { 42 for(j=0;j<(1<<m);j++) 43 { 44 for(k=0;k<G[j].size();k++) 45 dp[i][j]+=dp[i-1][G[j][k]]; 46 } 47 } 48 printf("%I64d\n",dp[n+1][0]); 49 } 50 void Init() 51 { 52 int i,j; 53 for(i=0;i<(1<<m);i++) 54 { 55 G[i].clear(); 56 for(j=0;j<(1<<m);j++) 57 { 58 if(OK(i,j)) 59 G[i].push_back(j); 60 } 61 } 62 } 63 int main() 64 { 65 while(scanf("%d%d",&n,&m),n||m) 66 { 67 if(n&m&1) 68 puts("0"); 69 else 70 { 71 if(m>n) 72 swap(n,m); 73 Init(); 74 DoIt(); 75 } 76 } 77 return 0; 78 }
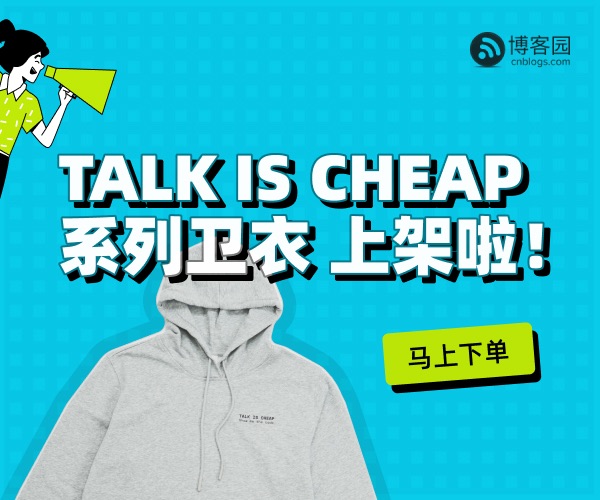