2020十一届蓝桥杯-七月省赛 不是CB组的
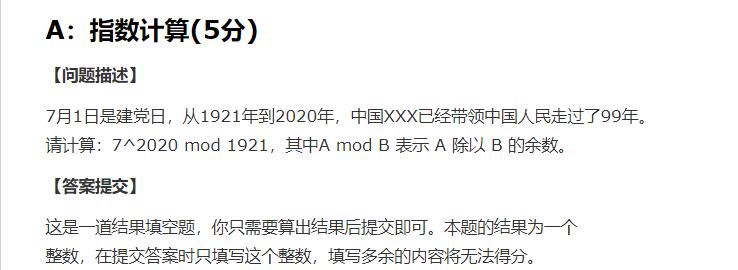
两种做法
#include <stdio.h>
#include <iostream>
using namespace std;
int dfs(int n) {
if (n == 1)
return 7;
else if (n % 2 == 0) {
return (dfs(n / 2) * dfs(n / 2)) % 1921;
}
else {
return (dfs(n / 2) * dfs(n / 2) * 7) % 1921;
}
}
int main() {
cout << dfs(2020);
return 0;
}
#include <stdio.h>
#include <iostream>
#include <map>
using namespace std;
int main() {
int ans = 1;
for (int i = 1; i <= 2020; i++) {
ans = (ans * 7) % 1921;
}
cout << ans;
return 0;
}
第二题
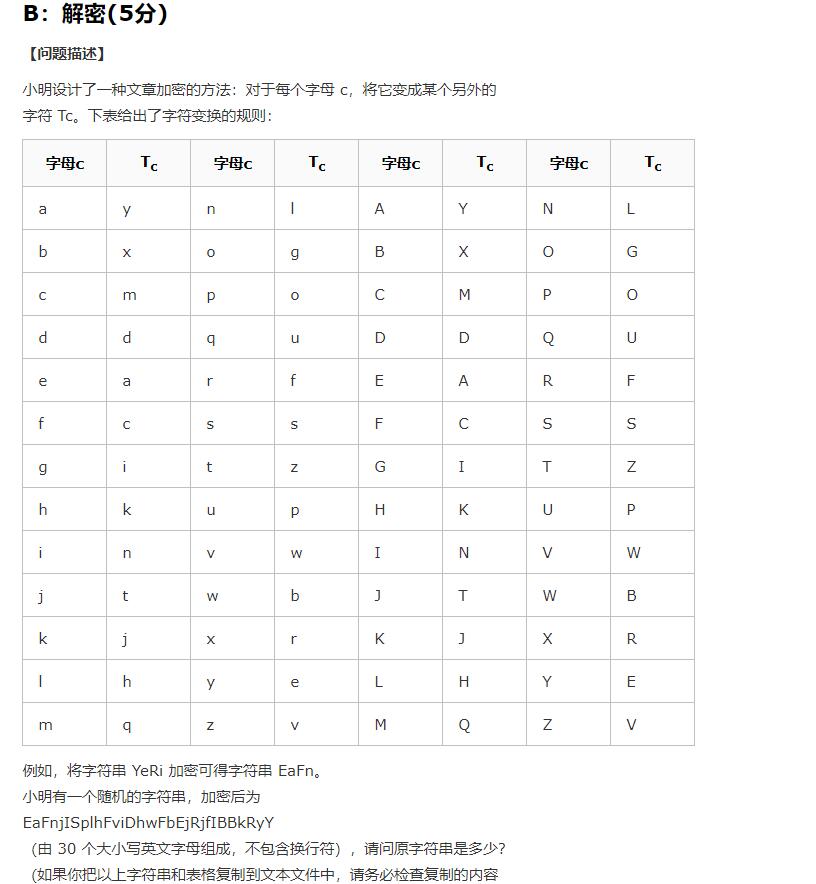
#include <stdio.h>
#include <iostream>
#include <map>
using namespace std;
int main() {
string str = "EaFnjISplhFviDhwFbEjRjfIBBkRyY";
string old = "abcdefghijklmnopqrstuvwxyzABCDEFGHIJKLMNOPQRSTUVWXYZ";
string New = "yxmdacikntjhqlgoufszpwbrevYXMDACIKNTJHQLGOUFSZPWBREV";
int index = 0;
for (int i = 0; i < str.size(); i++) {
index = New.find(str[i]);
cout << old[index];
}
return 0;
}
第三题
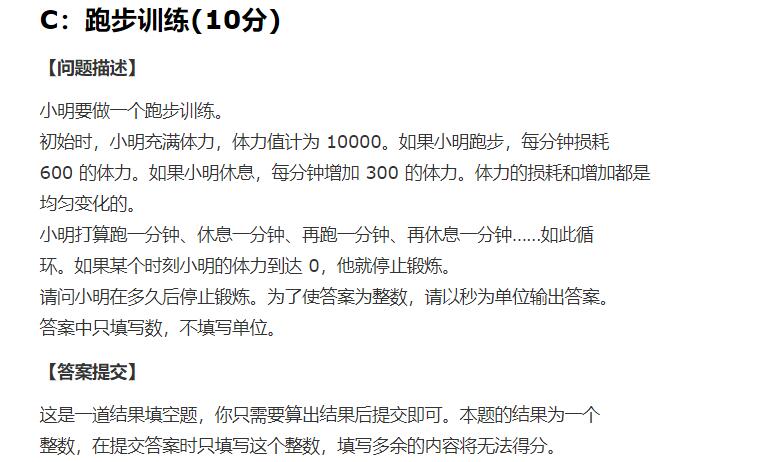
#include <stdio.h>
#include <iostream>
#include <map>
using namespace std;
int main() {
int start = 1000;
int flag = 1;// 1表示要跑
int cnt = 0;
while (start != 0) {
if (flag) {
if (start - 600 >= 0) {
start -= 600;
cnt += 60;
}
else {
cnt += (start / 10);
start = 0;
}
flag = !flag;
}
else {
start += 300;
cnt += 60;
flag = !flag;
}
}
cout << cnt;
return 0;
}
D
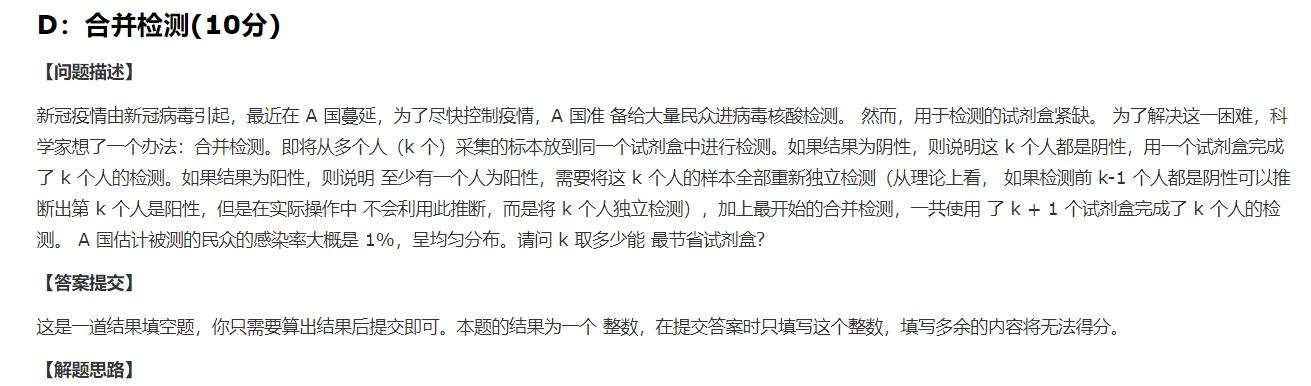
E
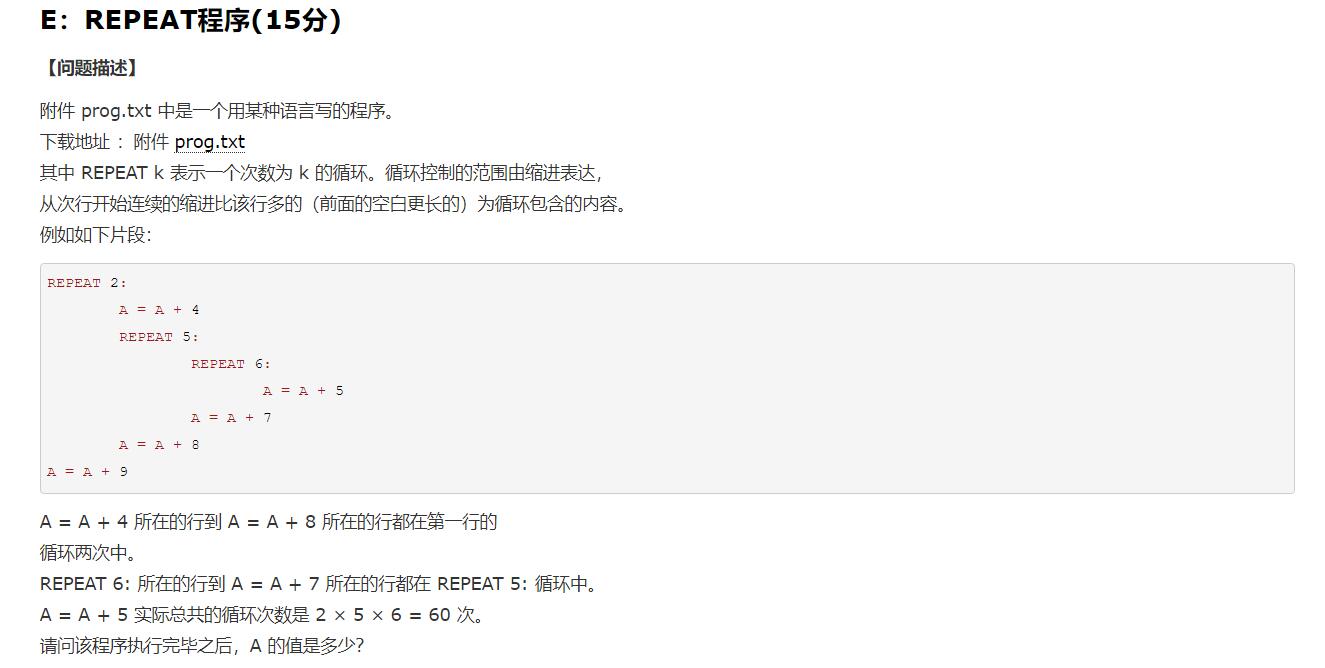
F
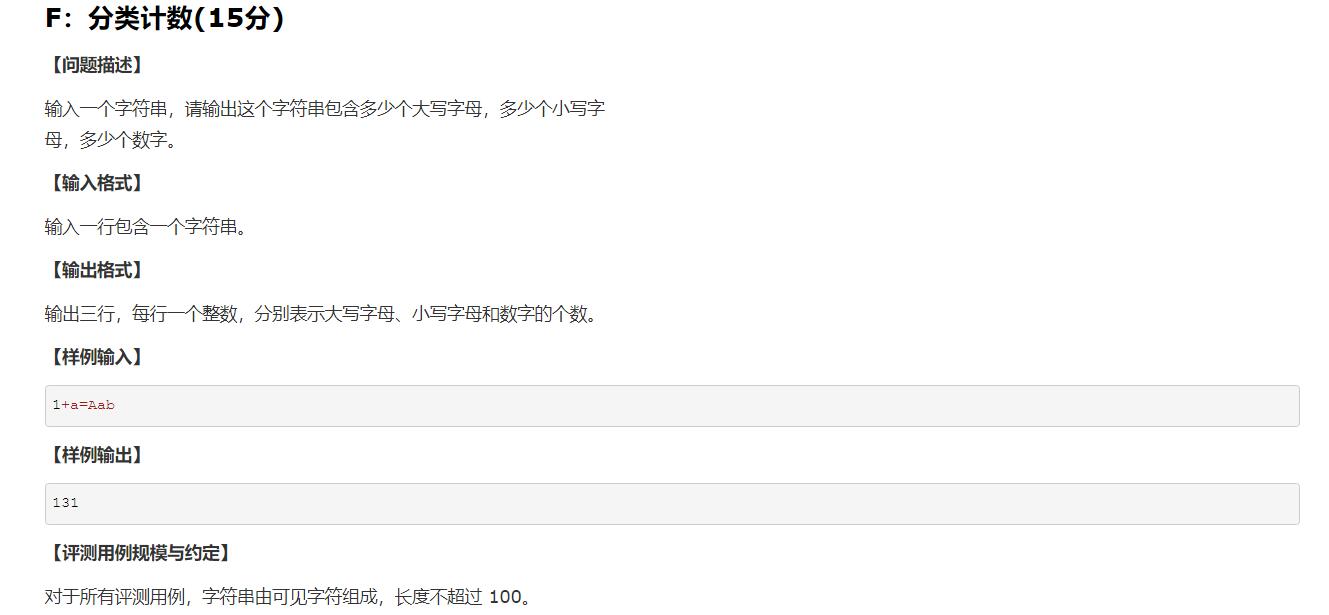
#include <iostream>
#include <stdio.h>
using namespace std;
int main() {
string str;
int cnt1 =0, cnt2 =0, cnt3 = 0;
cin >> str;
for (int i = 0; i < str.length(); i++) {
if (str[i] >= '0' && str[i] <= '9') {
cnt3++;
}
else if (str[i] >= 'A' && str[i] <= 'Z') {
cnt1++;
}
else if (str[i] >= 'a' && str[i] <= 'z') {
cnt2++;
}
}
cout << cnt1 << endl << cnt2 << endl << cnt3 << endl;
return 0;
}
G
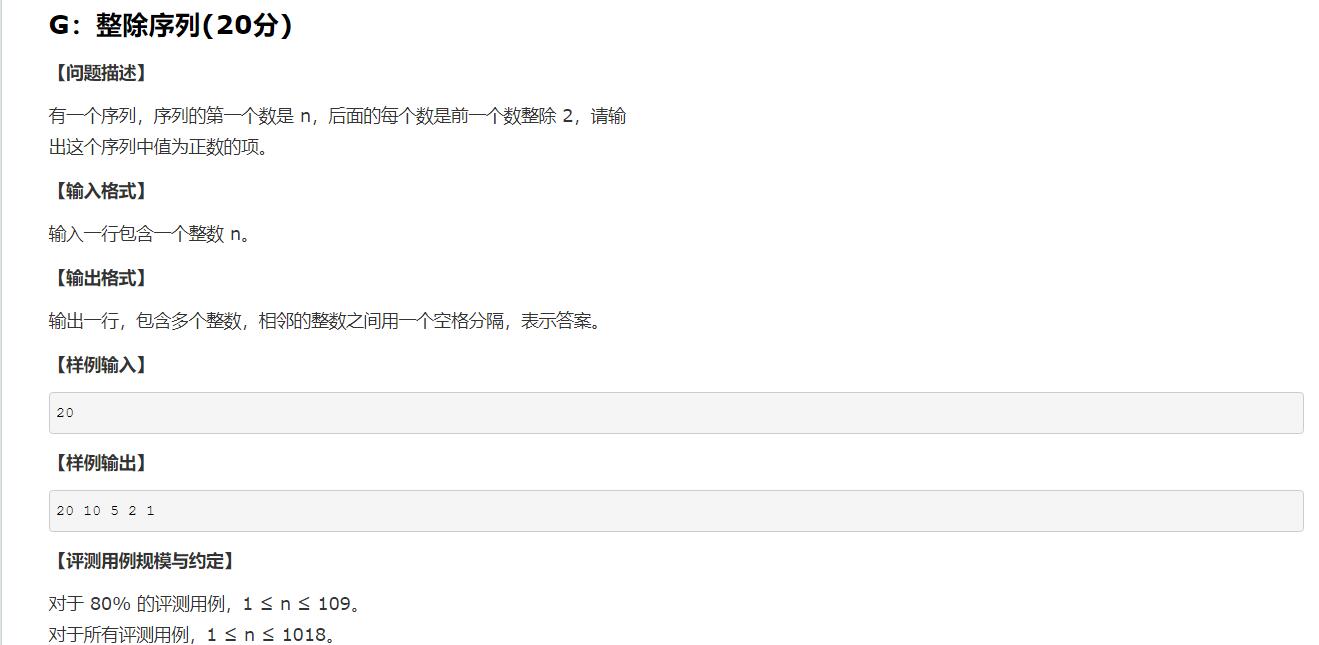
#include <iostream>
#include <stdio.h>
#include <vector>
using namespace std;
int main() {
int n;
vector<int> ans;
cin >> n;
ans.push_back(n);
while (n / 2 != 0) {
ans.push_back(n / 2);
n /= 2;
}
for (int i = 0; i < ans.size(); i++) {
if (i != ans.size() - 1)
cout << ans[i] << " ";
else {
cout << ans[i] << endl;
}
}
return 0;
}
H
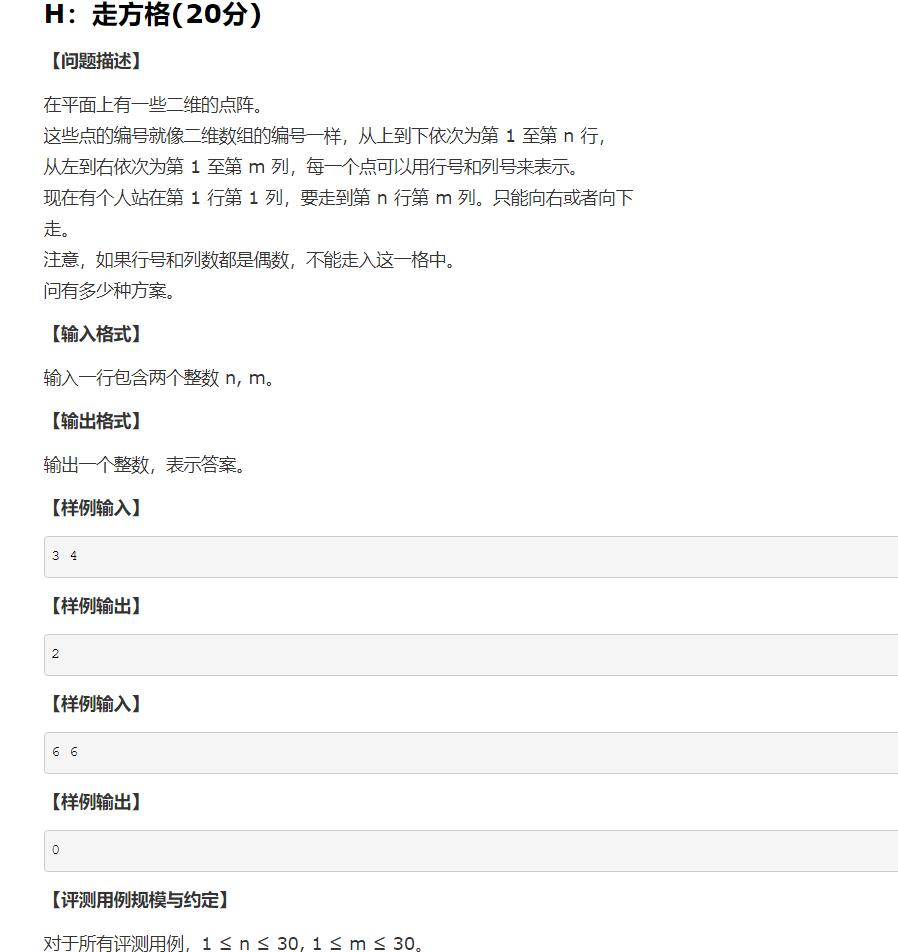
#include<iostream>
#include<algorithm>
using namespace std;
int a[35][35];
int main()
{
for(int i=0;i<35;i++){
fill(a[i],a[i]+35,0);
}
for(int i=0;i<35;i++){
a[i][1]=1;
a[1][i]=1;
}
int n,m;
cin >> n >> m;
for(int i=1;i<=n;i++){
for(int j=1;j<=m;j++){
if(i==1||j==1){
a[i][j]=1;
}
else if(i%2==0&&j%2==0){
a[i][j]=0;
}
else {
a[i][j]=a[i-1][j]+a[i][j-1];
}
}
}
return 0;
}
I
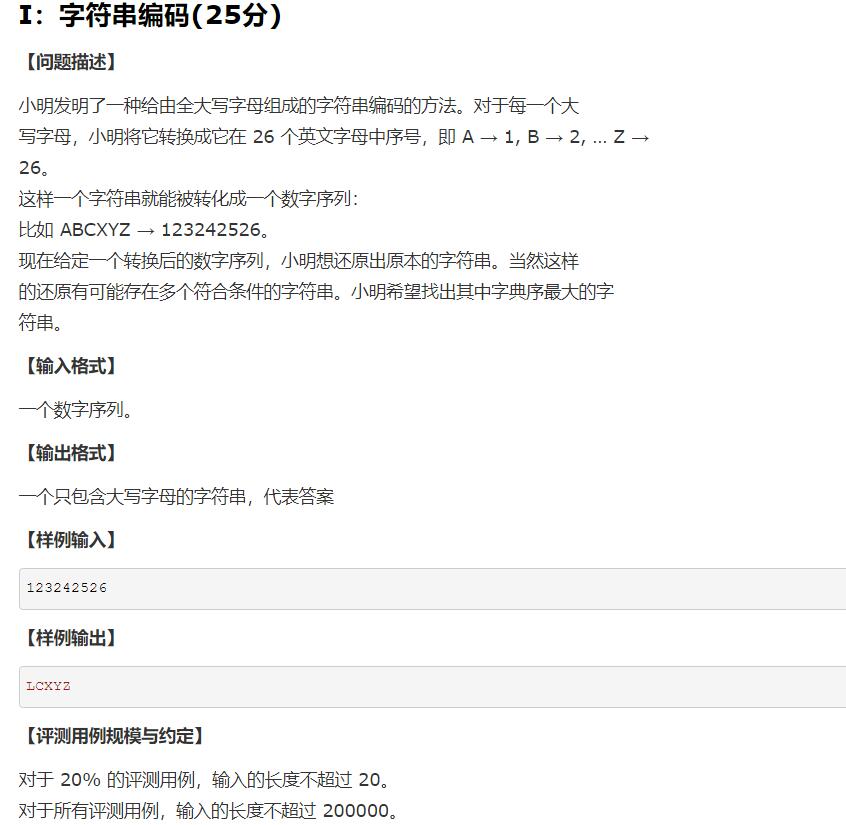
#include <iostream>
#include <stdio.h>
#include <vector>
#define max 35
using namespace std;
int maze[max][max];
int main() {
string target;
char Base = 'A';
string ans = "";
cin >> target;
int len = target.length();
for (int i = 0; i < len; i++) {
int offset = 0;
if (target[i] == '1') {
if (i + 1 != len) {
offset = 10 + target[i + 1] - '0';
i++;
}
else {
offset = 1;
}
}
else if (target[i] == '2' ) {
if (i + 1 != len && target[i + 1] <= '6') {
offset = 20 + target[i + 1] - '0';
i++;
}
else {
offset = 2;
}
}
else {
offset = target[i] - '0';
}
ans = ans + char( Base + offset - 1);
}
cout << ans << endl;
return 0;
}
J
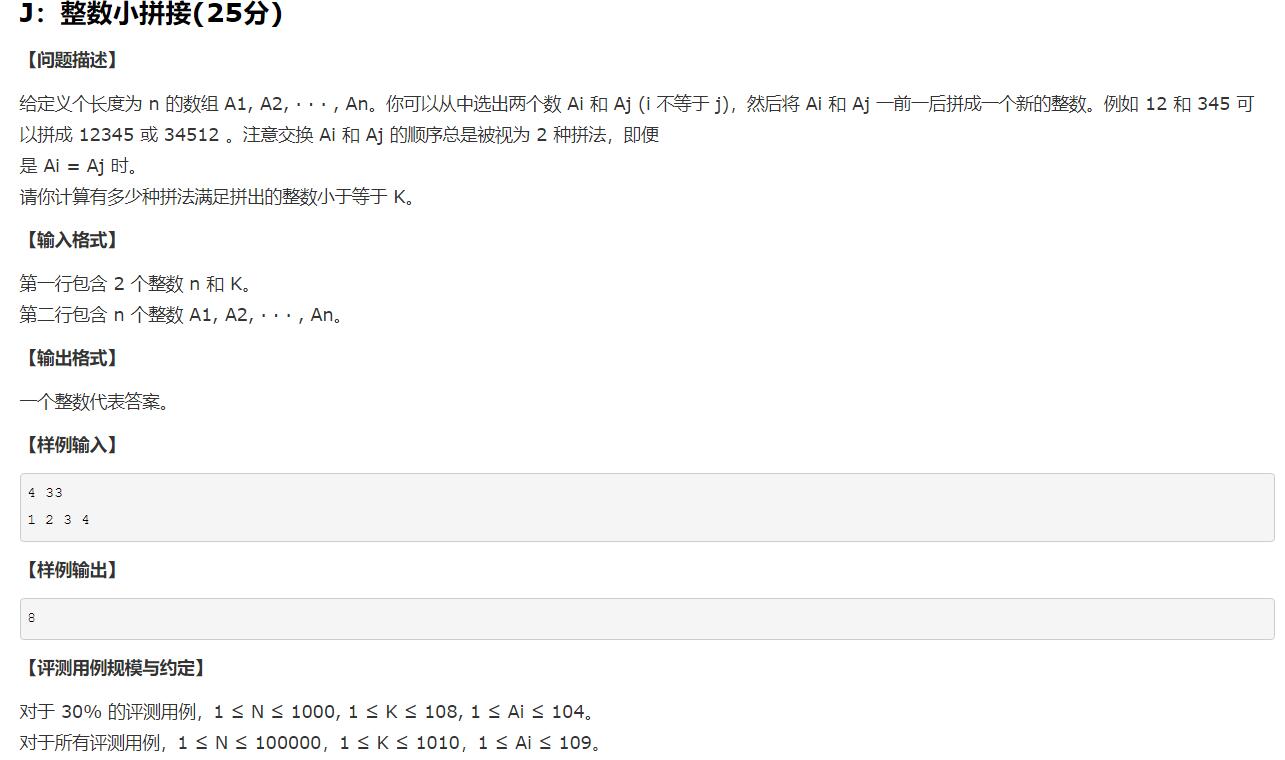
#include <iostream>
#include <stdio.h>
#include <vector>
#include <algorithm>
#define max 35
using namespace std;
int maze[max][max];
int digits(int k) {
int cnt = 0;
while (k != 0) {
cnt++;
k /= 10;
}
return cnt;
}
int cal(int x, int y,int z) {
while (y--) {
x *= 10;
}
x += z;
return x;
}
int main() {
int n, k;
cin >> n >> k;
int cnt = (digits(k) + 1) / 2 ;
vector<int> v;
int temp,ans = 0;
for (int i = 0; i < n; i++) {
cin >> temp;
v.push_back(temp);
}
sort(v.begin(), v.end());
int len = v.size();
for (int i = 0; i < len; i++) {
for (int j = 0; j < len; j++) {
if (i == j) continue;
if ((cal(v[i], digits(v[j]),v[j]) > k)||(digits(v[i]) > cnt && digits(v[j]) > cnt) ){
if ( j < i)
cout << i * (len - 1) + j << endl ;
else {
cout << i * (len - 1) + j - 1 << endl;
}
return 0;
}
}
}
cout << len * (len - 1) << endl;
return 0;
}