实验4 汇编应用编程和c语言程序反汇编分析
assume cs:code, ds:data data segment db 'welcome to masm!' db 00000010B,00100100B,01110001B ;颜色信息 绿色 绿底红色 白底蓝色 data ends code segment start: mov ax, data mov ds, ax mov ax, 0b800H mov es, ax ;设置显存段地址 mov cx,3 ;循环次数为3 mov bx,0 mov di, 1824 ;1764+64 附加短地址偏移地址 s0: push cx mov cx, 16 ;内存循环次数为16 mov si, 0 s1: mov al, [si] mov es:[di], al mov al, [16+bx] mov es:[di+1], al inc si add di, 2 loop s1 pop cx inc bx add di,128 loop s0 mov ah, 4ch int 21h code ends end start
结果如下:
分析:两个循环,外循环中颜色控制颜色信息的改变,内循环每次输入16个字符;
assume cs:code, ds:data data segment str db 'try', 0 data ends code segment start: mov ax, data mov ds, ax mov si, offset str mov al, 2 call printStr mov ah, 4ch int 21h printStr: push bx push cx push si push di mov bx, 0b800H mov es, bx mov di, 0 s: mov cl, [si] mov ch, 0 jcxz over mov ch, al mov es:[di], cx inc si add di, 2 jmp s over: pop di pop si pop cx pop bx ret code ends end start
结果:
str db 'another try', 0
把line12改为:
mov al, 4
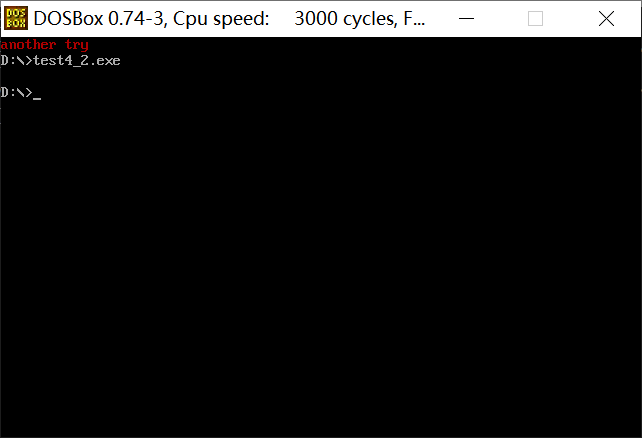
assume cs:code, ds:data data segment x dw 1984 str db 16 dup(0) data ends code segment start: mov ax, data mov ds, ax mov ax, x mov di, offset str call num2str mov ah, 4ch int 21h num2str: push ax push bx push cx push dx mov cx, 0 mov bl, 10 s1: div bl inc cx mov dl, ah push dx mov ah, 0 cmp al, 0 jne s1 s2: pop dx or dl, 30h mov [di], dl inc di loop s2 pop dx pop cx pop bx pop ax ret code ends end start
子任务1
对task3.asm进行汇编、链接,得到可执行程序后,在debug中使用u命令反汇编,使用g命令执行 到line15(程序退出之前),使用d命令查看数据段内容,观察是否把转换后的数字字符串'1984'存放 在数据段中str标号后面的单元。
assume cs:code, ds:data
data segment
x dw 1984
str db 16 dup(0)
data ends
code segment
start:
mov ax, data
mov ds, ax
mov ax, x
mov di, offset str
call num2str
mov si,offset str
mov al,2
call printStr
mov ah, 4ch
int 21h
num2str:
push ax
push bx
push cx
push dx
mov cx, 0
mov bl, 10
s1:
div bl
inc cx
mov dl, ah
push dx
mov ah, 0
cmp al, 0
jne s1
s2:
pop dx
or dl, 30h
mov [di], dl
inc di
loop s2
pop dx
pop cx
pop bx
pop ax
ret
printStr:
push bx
push cx
push si
push di
mov bx, 0b800H
mov es, bx
mov di, 0
s: mov cl, [si]
mov ch, 0
jcxz over
mov ch, al
mov es:[di], cx
inc si
add di, 2
jmp s
over: pop di
pop si
pop cx
pop bx
ret
code ends
end start
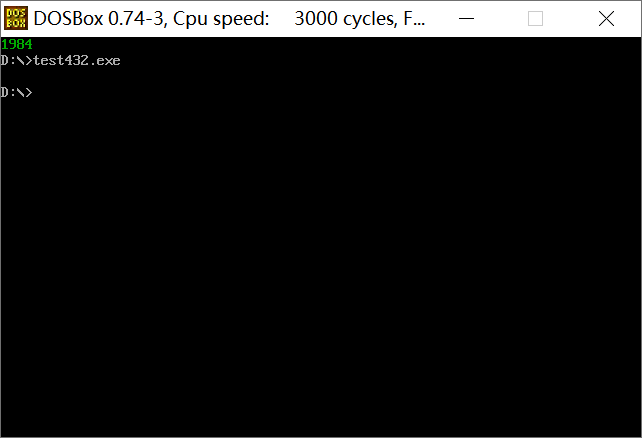
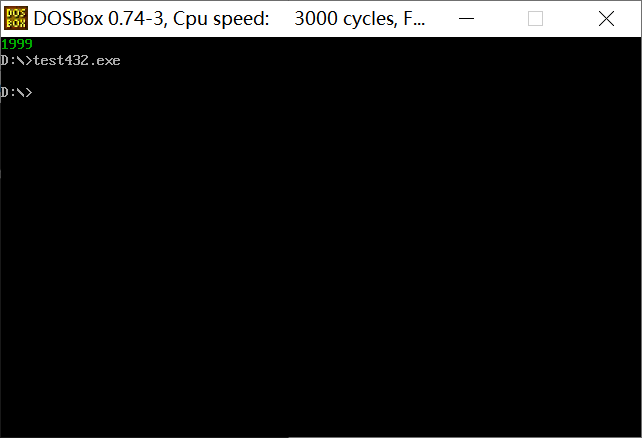
assume cs:code, ds:data data segment str db 80 dup(?) data ends code segment start: mov ax, data mov ds, ax mov si, 0 s1: mov ah, 1 int 21h mov [si], al cmp al, '#' je next inc si jmp s1 next: mov cx, si mov si, 0 s2: mov ah, 2 mov dl, [si] int 21h inc si loop s2 mov ah, 4ch int 21h code ends end start
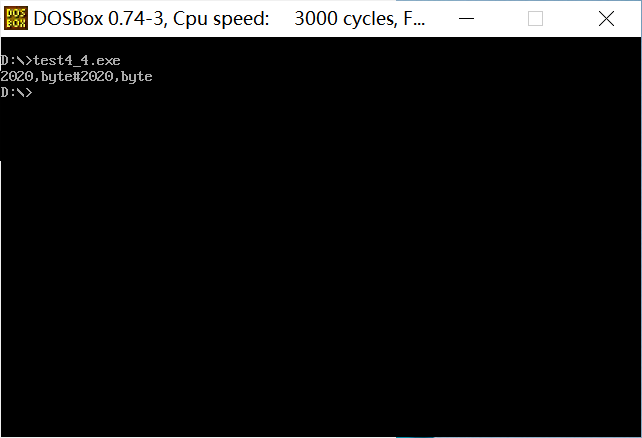
-
汇编、链接、运行程序,输入一个字符串并以#结束(比如,2020, bye#)观察运行结果。结合运行结果,理解程序功能,了解软中断指令。具体地:line12-19实现的功能是?
-
答:接收输入的字符串,“#”表示输入结束;cmp是比较指令,执行后对标志寄存器产生影响;je跳转函数,比较后相等则跳转。
-
line21-27实现的功能是?
- 答:将字符串输出到屏幕上。
#include<stdio.h> int sum(int,int); int main(){ int a=2,b=7,c; c=sum(a,b); return 0; } int sum(int x,int y){ return (x+y); }
设置断点:
反汇编:
总结对这个简单的c代码反汇编后,你对反汇编出来的汇编指令分析的内容总结。可以包括但不限于一下内容的总结:
答:参数传递和返回值是通过栈实现的;