using System; using System.Collections.Generic;//List<T>的命名空间 using System.Linq; using System.Text; using System.Threading;//线程所有空间 namespace Test { class TestThread { private static void ThreadFuncOne()//因为Main函数为static 的原因, 所以这边也要定义为Static { for (int i = 0; i < 10; i++) { Console.WriteLine(Thread.CurrentThread.Name + " i = " + i); } Console.WriteLine(Thread.CurrentThread.Name + " has finished"); } private static void ThreadFuncOne2(object status ) { for (int i = 0; i < 10; i++) { Console.WriteLine(Thread.CurrentThread.Name + " i = " + i); } Console.WriteLine(status.ToString() + Thread.CurrentThread.Name + " has finished"); } private void ThreadFuncOne3(object status)// { for (int i = 0; i < 10; i++) { Console.WriteLine(Thread.CurrentThread.Name + " i = " + i); } Console.WriteLine(status.ToString() + Thread.CurrentThread.Name + " has finished"); } static void Main(string[] args) { Thread.CurrentThread.Name = "MainThread"; Thread newThread = new Thread(new ThreadStart(TestThread.ThreadFuncOne));//这种不带参数 newThread.Name = "NewThread"; for (int j = 0; j < 20; j++) { if (j == 10) { newThread.Start(); //newThread.Join(); newThread.Join(); } else { Console.WriteLine(Thread.CurrentThread.Name + " j = " + j); } } newThread.Join();//阻塞主线程,直到NewThread 运行完成 //用线程池 WaitCallback BackLoop = new WaitCallback(ThreadFuncOne2 );//定义一个线程启动的回调 ThreadPool.QueueUserWorkItem(BackLoop, "我的线程");//以"我的线程作为一个参数" Console.ReadLine(); Thread t2 = new Thread(ThreadFuncOne2); Thread t1 = new Thread((object test) =>//这里定义具有参数的线程,用到Lambda表达式的定义方式 { Thread.Sleep(1000); Thread t = Thread.CurrentThread; Console.WriteLine("Name: " + t.Name); Console.WriteLine("ManagedThreadId: " + t.ManagedThreadId); Console.WriteLine("State: " + t.ThreadState); Console.WriteLine("Priority: " + t.Priority); Console.WriteLine("IsBackground: " + t.IsBackground); Console.WriteLine("IsThreadPoolThread: " + t.IsThreadPoolThread); Console.WriteLine("Thread Parm: " + test.ToString()); })//在括号后面接{} { Name = "Thread1",//通过这种方法 来设定它的属性 Priority = ThreadPriority.Highest }; t1.Start("线程参数");//带参数线程启动方式 Console.WriteLine("因为没有join ,先于Thread1调用"); t1.Join(); Console.WriteLine("因为有join ,后于Thread1调用"); //上面的动用如下方法 TestThread testTh = new TestThread(); Thread newThread3 = new Thread(testTh.ThreadFuncOne3) { Name ="带参数的线程"}; newThread3.Start("线程参数");//带参数线程启动方式 myClass myCS = new myClass() { _temp1 = "", _temp2 = "100" }; Console.ReadLine(); } } public class myClass { public string _temp1 { get; set; } public string _temp2 { get; set; } } }
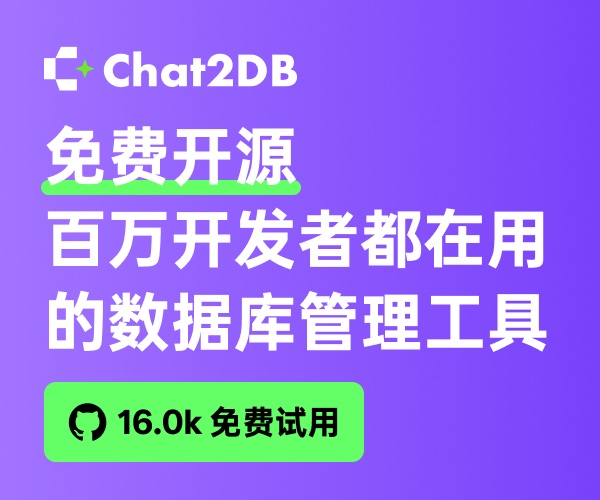
