说明一下,所有代码需要:基础代码(通用)+ 关键代码才能运行。请自行结合
基础代码
import cv2 as cv
# 读路径下的图片
img = cv.imread("./cat.jpg")
# 在窗口显示
cv.imshow("color", img)
cv.waitKey(0)
平移图片
# 左右上下平移
# -x: 向左
# -y: 向上
# x: 向右
# y: 向下
def move(img, x ,y):
movemat = np.float32([ [1,0,x],[0,1,y] ])
# 1表示宽度
# 0表示高度
dimentions = (img.shape[1], img.shape[0])
return cv.warpAffine(img, movemat, dimentions)
new_img = move(img, 100, 100)
cv.imshow("moved", new_img)
效果
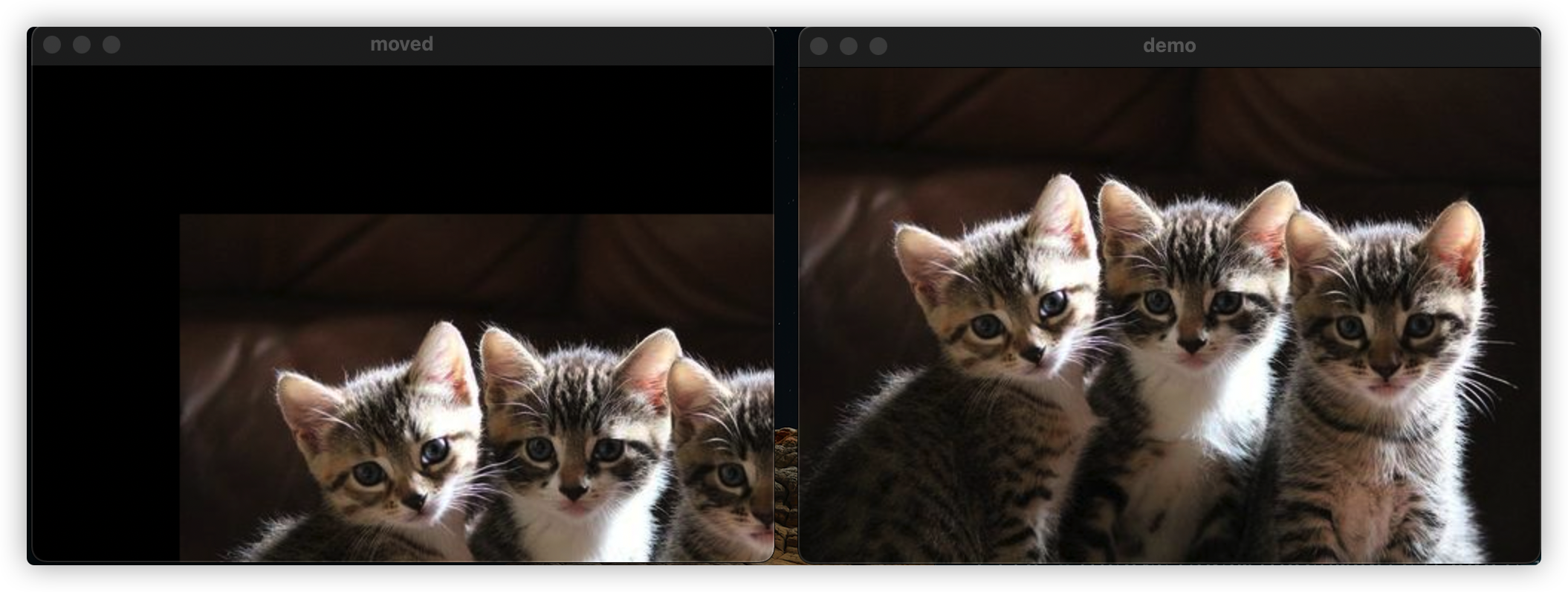
旋转图片
# 旋转
def rotate(img, angle, rotpoinet=None):
(height, width) = img.shape[:2]
# 如果没指定旋转的中心点,那么选择画面的中央作为旋转点
if rotpoinet is None:
rotpoinet = (width//2, height//2)
rotmat = cv.getRotationMatrix2D(rotpoinet, angle, 1)
dimentions = (width, height)
return cv.warpAffine(img, rotmat, dimentions)
rotate_img = rotate(img, 90)
cv.imshow("rotate", rotate_img)
效果
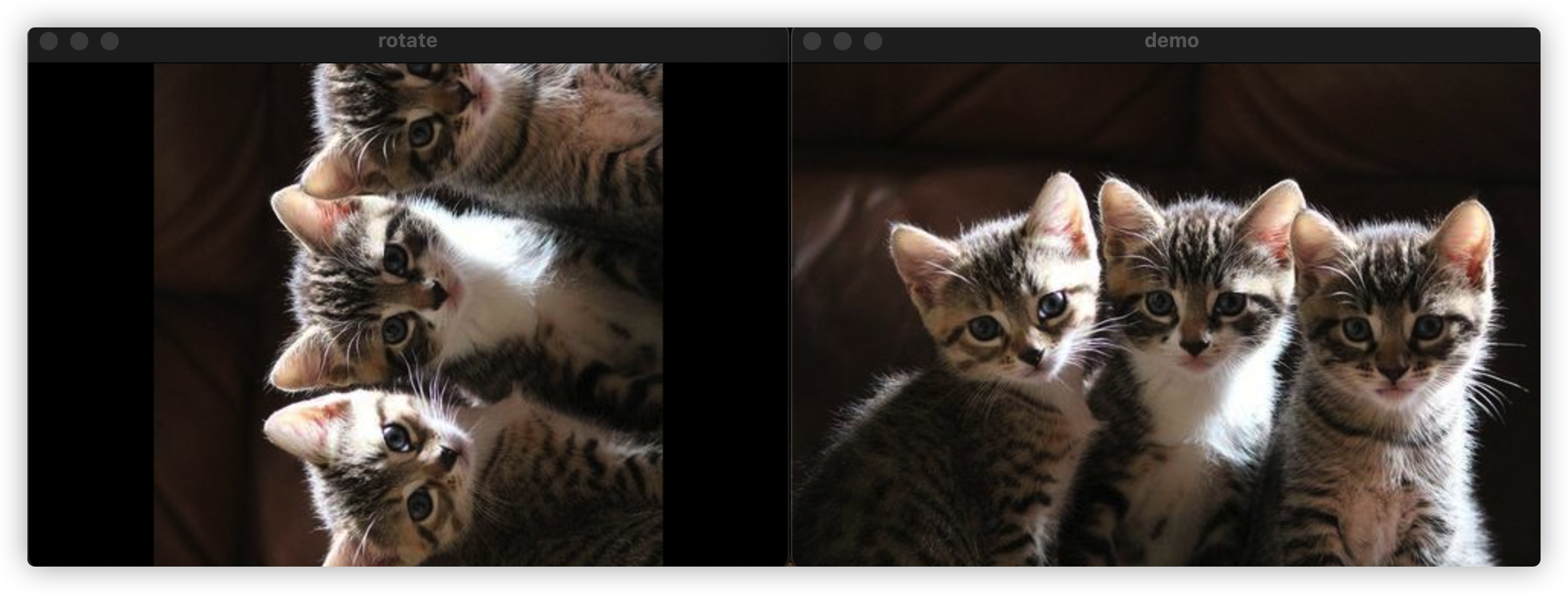
改变尺寸
# 改变尺寸
resized = cv.resize(img, (200, 200), interpolation=cv.INTER_CUBIC)
cv.imshow("resized", resized)
效果
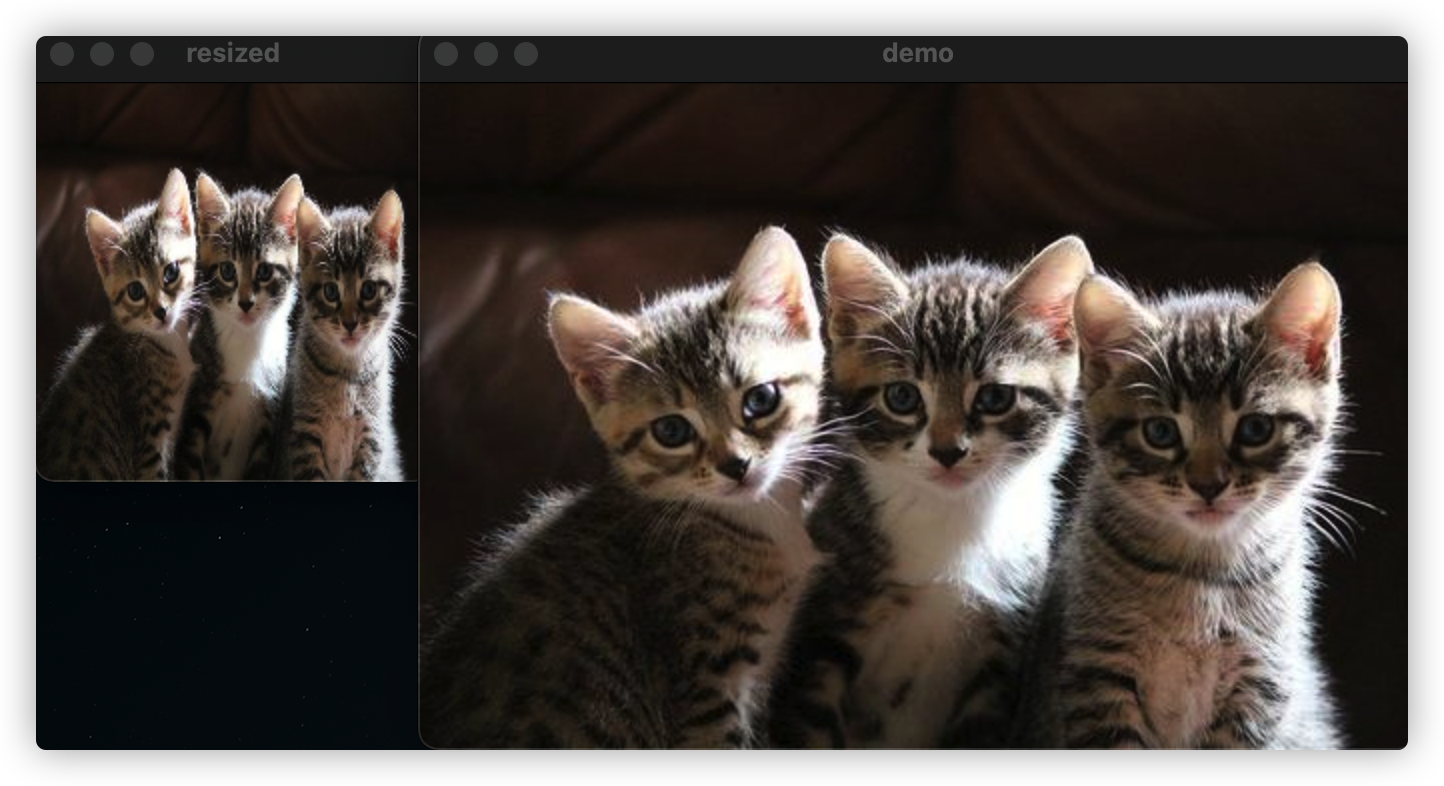
反转
# 反转
# 竖直方向--沿着x轴
filp_one = cv.flip(img ,0)
# 水平方向--沿着y轴
filp_two = cv.flip(img ,1)
cv.imshow("demoone", filp_one)
cv.imshow("demotwo", filp_two)
效果
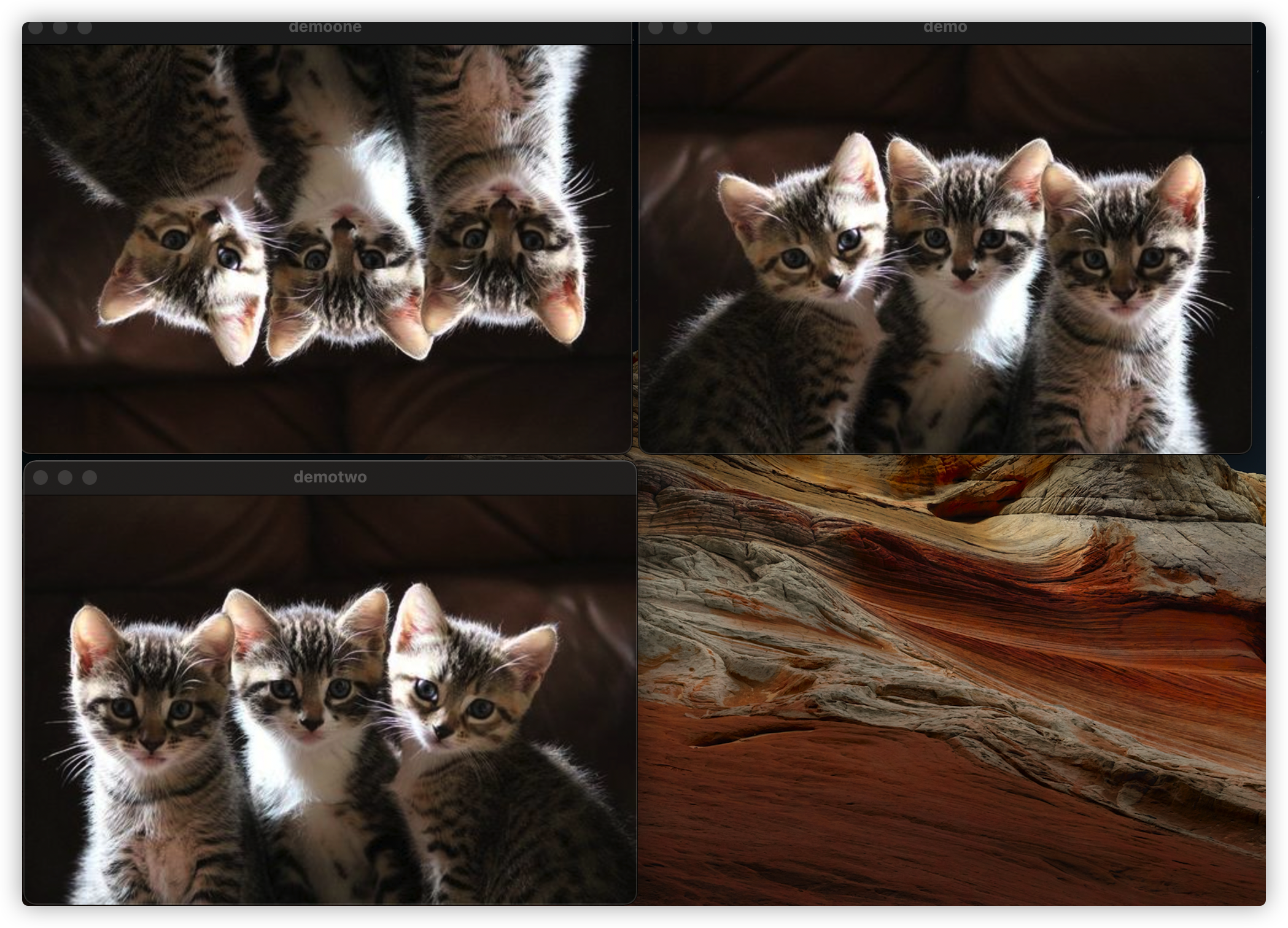
完整代码
import cv2 as cv
import numpy as np
from numpy.core.fromnumeric import shape
# 读路径下的图片
img = cv.imread("./cat.jpg")
# 左右上下平移
# -x: 向左
# -y: 向上
# x: 向右
# y: 向下
def move(img, x ,y):
movemat = np.float32([ [1,0,x],[0,1,y] ])
# 1表示宽度
# 0表示高度
dimentions = (img.shape[1], img.shape[0])
return cv.warpAffine(img, movemat, dimentions)
# new_img = move(img, 100, 100)
# cv.imshow("moved", new_img)
# 旋转
# def rotate(img, angle, rotpoinet=None):
# (height, width) = img.shape[:2]
# # 如果没指定旋转的中心点,那么选择画面的中央作为旋转点
# if rotpoinet is None:
# rotpoinet = (width//2, height//2)
# rotmat = cv.getRotationMatrix2D(rotpoinet, angle, 1)
# dimentions = (width, height)
# return cv.warpAffine(img, rotmat, dimentions)
# rotate_img = rotate(img, 90)
# cv.imshow("rotate", rotate_img)
# # 改变尺寸
# resized = cv.resize(img, (200, 200), interpolation=cv.INTER_CUBIC)
# cv.imshow("resized", resized)
# 反转
# 竖直方向--沿着x轴
filp_one = cv.flip(img ,0)
# 水平方向--沿着y轴
filp_two = cv.flip(img ,1)
cv.imshow("demoone", filp_one)
cv.imshow("demotwo", filp_two)
cv.imshow("demo", img)
cv.waitKey(0)