观察者模式(Observer)
1 /* 2 * 网上商店中如果商品(product)在名称(name)、价格(price)等 3 * 方面有变化,系统能自动通知会员,将是网上商店区别传统商店的一 4 * 大特色。应用外观模式,用C#控制台应用程序改进设计。 5 */ 6 using System; 7 using System.Collections.Generic; 8 using System.Linq; 9 using System.Text; 10 11 namespace Observer 12 { 13 //观察者-会员类 14 class Vip 15 { 16 private string name; 17 private string rcv_msg; 18 private ShopSystem shopsystem; 19 20 public Vip(ShopSystem shopsystem, string name) 21 { 22 this.shopsystem = shopsystem; 23 this.name = name; 24 } 25 public void RcvMsg() 26 { 27 rcv_msg = shopsystem.SndMsg; 28 Console.WriteLine("尊敬的{0},{1},预购从速哦~亲~",name,rcv_msg); 29 } 30 } 31 //通知者-系统类 32 class ShopSystem 33 { 34 private string snd_msg; 35 private IList<Vip> vips = new List<Vip>(); 36 public string SndMsg 37 { 38 get { return snd_msg; } 39 set { snd_msg = value; } 40 } 41 public void Attach(Vip vip) 42 { 43 vips.Add(vip); 44 } 45 public void Detach(Vip vip) 46 { 47 vips.Remove(vip); 48 } 49 public void Notify() 50 { 51 foreach (Vip vip in vips) 52 { 53 vip.RcvMsg(); 54 } 55 } 56 } 57 //Client-商品类 58 class Product 59 { 60 private string name; 61 private string price; 62 public Product(string name, string price) 63 { 64 this.name = name; 65 this.price = price; 66 } 67 public void SetName(ShopSystem shopsystem,string name) 68 { 69 shopsystem.SndMsg=("【"+this.name+"】改名为【"+name+"】"); 70 shopsystem.Notify(); 71 this.name = name; 72 } 73 public void SetPrice(ShopSystem shopsystem,string price) 74 { 75 shopsystem.SndMsg = ("【" + name + "】价格由【" + this.price + "】调整为【" + price + "】"); 76 shopsystem.Notify(); 77 this.price = price; 78 } 79 } 80 class Program 81 { 82 static void Main(string[] args) 83 { 84 Product pro1 = new Product("北京方便面","0.7¥"); 85 Product pro2 = new Product("武汉热干面","2.0¥"); 86 ShopSystem shopsystem = new ShopSystem(); 87 shopsystem.Attach(new Vip(shopsystem, "魏艾皮")); 88 shopsystem.Attach(new Vip(shopsystem, "卫蔼霹")); 89 90 pro1.SetName(shopsystem,"超级北京方便面"); 91 pro2.SetName(shopsystem,"无敌武汉热干面"); 92 pro1.SetPrice(shopsystem, "0.5¥"); 93 pro1.SetPrice(shopsystem, "1.5¥"); 94 } 95 } 96 }
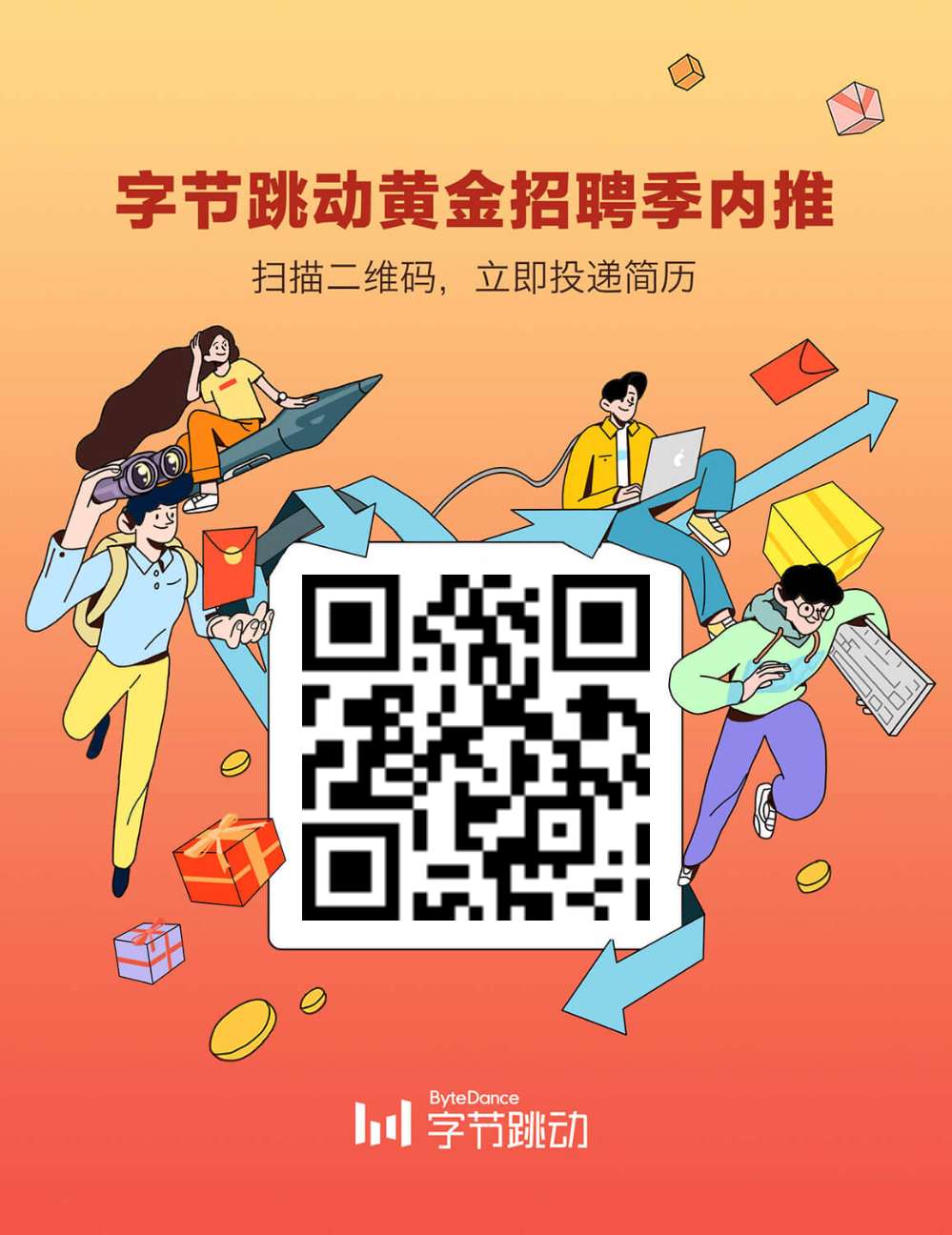
找我内推: 字节跳动各种岗位
作者:
ZH奶酪(张贺)
邮箱:
cheesezh@qq.com
出处:
http://www.cnblogs.com/CheeseZH/
*
本文版权归作者和博客园共有,欢迎转载,但未经作者同意必须保留此段声明,且在文章页面明显位置给出原文连接,否则保留追究法律责任的权利。