实现效果
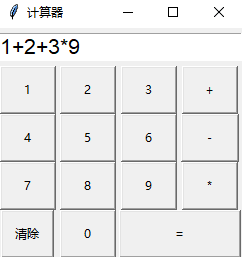
具体代码
"""
简易计算器
有一个“显示屏”可以实时显示输入内容
可以进行多元运算
可以接受键盘输入,也能通过鼠标点击按键输入
"""
import tkinter as tk
# 初始化计算器的值
calculation = ""
# 在按下按键时调用此函数
def button_click(number):
global calculation
calculation += str(number)
update_screen()
# 清除显示屏上的数字
def clear_screen():
global calculation
calculation = ""
update_screen()
# 运行计算器计算结果
def calculate():
global calculation
try:
result = str(eval(calculation))
clear_screen()
calculation = result
update_screen()
except:
clear_screen()
update_screen()
calculation = ""
# 更新显示屏
def update_screen():
screen.delete(0, tk.END)
screen.insert(0, calculation)
# 创建主窗口
root = tk.Tk()
root.title("计算器")
# 创建显示屏
screen = tk.Entry(root, width=20, font=("Arial", 16))
screen.grid(row=0, column=0, columnspan=4, pady=5)
# 创建按键
button_1 = tk.Button(root, text="1", padx=20, pady=10, command=lambda: button_click(1))
button_2 = tk.Button(root, text="2", padx=20, pady=10, command=lambda: button_click(2))
button_3 = tk.Button(root, text="3", padx=20, pady=10, command=lambda: button_click(3))
button_4 = tk.Button(root, text="4", padx=20, pady=10, command=lambda: button_click(4))
button_5 = tk.Button(root, text="5", padx=20, pady=10, command=lambda: button_click(5))
button_6 = tk.Button(root, text="6", padx=20, pady=10, command=lambda: button_click(6))
button_7 = tk.Button(root, text="7", padx=20, pady=10, command=lambda: button_click(7))
button_8 = tk.Button(root, text="8", padx=20, pady=10, command=lambda: button_click(8))
button_9 = tk.Button(root, text="9", padx=20, pady=10, command=lambda: button_click(9))
button_0 = tk.Button(root, text="0", padx=20, pady=10, command=lambda: button_click(0))
button_add = tk.Button(root, text="+", padx=19, pady=10, command=lambda: button_click("+"))
button_subtract = tk.Button(root, text="-", padx=22, pady=10, command=lambda: button_click("-"))
button_multiply = tk.Button(root, text="*", padx=21, pady=10, command=lambda: button_click("*"))
button_divide = tk.Button(root, text="/", padx=22, pady=10, command=lambda: button_click("/"))
button_equal = tk.Button(root, text="=", padx=52, pady=10, command=calculate)
button_clear = tk.Button(root, text="清除", padx=10, pady=10, command=clear_screen)
# 将按键添加到主窗口
button_1.grid(row=1, column=0)
button_2.grid(row=1, column=1)
button_3.grid(row=1, column=2)
button_4.grid(row=2, column=0)
button_5.grid(row=2, column=1)
button_6.grid(row=2, column=2)
button_7.grid(row=3, column=0)
button_8.grid(row=3, column=1)
button_9.grid(row=3, column=2)
button_0.grid(row=4, column=1)
button_add.grid(row=1, column=3)
button_subtract.grid(row=2, column=3)
button_multiply.grid(row=3, column=3)
button_divide.grid(row=4, column=3)
button_equal.grid(row=4, column=2, columnspan=2)
button_clear.grid(row=4, column=0)
root.mainloop()