重排链表
题目描述
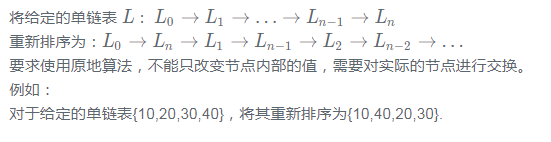
解题思路
- 我们知道
ArrayList
的底层就是用数组实现的,所以我们将链表储存在一个ArrayList
中,然后利用双指针,一个指向最前面,一个指向最后面,依次访问并向题目要求的链表形式进行转换!
public void reorderList_1(ListNode head) {
if (head == null){
return;
}
List<ListNode> list = new ArrayList<>();
while (head != null){
list.add(head);
head = head.next;
}
int i = 0,j = list.size() - 1;
while (i < j){
list.get(i).next = list.get(j);
i++;
if(i == j)
break;
list.get(j).next = list.get(i);
j--;
}
list.get(i).next = null;
}
- 三步走:1. 将链表分为两个链表 2. 将第二个链表逆序 3. 依次连接两个链表
public void reorderList(ListNode head){
if (head == null || head.next == null || head.next.next == null)
return;
ListNode slow = head;
ListNode fast = head.next;
while (fast != null && fast.next != null){
slow = slow.next;
fast = fast.next.next;
}
ListNode mid = slow.next;
slow.next = null;
ListNode newHead = reverse(mid);
while (newHead != null){
ListNode temp = newHead.next;
newHead.next = head.next;
head.next = newHead;
head = newHead.next;
newHead = temp;
}
}
private ListNode reverse(ListNode head) {
if (head == null)
return head;
ListNode tail = head;
head = head.next;
tail.next = null;
while (head != null){
ListNode tmp = head.next;
head.next = tail;
tail = head;
head = tmp;
}
return tail;
}