1、模式介绍
typedef enum {
/** 默认模式,使用系统自带的指示器 ,不能显示进度,只能不停地转呀转*/
MBProgressHUDModeIndeterminate,
/** 用饼图显示进度 */
MBProgressHUDModeDeterminate,
/** 进度条 */
MBProgressHUDModeDeterminateHorizontalBar,
/** 圆环 */
MBProgressHUDModeAnnularDeterminate,
/** 自定义视图 */
MBProgressHUDModeCustomView,
/** 只显示文字 */
MBProgressHUDModeText
} MBProgressHUDMode;
2、实际效果
-
2.1 只显示文字:MBProgressHUDModeText
// 只显示文字
- (void)TextButtonAction {
self.HUD = [[MBProgressHUD alloc] initWithView:self.view];
[self.view addSubview:_HUD];
_HUD.labelText = @"加载中,请稍等...";
_HUD.mode = MBProgressHUDModeText;
[_HUD showAnimated:YES whileExecutingBlock:^{
sleep(2);
}
completionBlock:^{
[_HUD removeFromSuperview];
_HUD = nil;
}];
}
-
2.2 圆形进度条:MBProgressHUDModeDeterminate
// 圆形进度条
- (void)CircularButtonAction {
// 圆形进度条
self.HUD = [[MBProgressHUD alloc] initWithView:self.view];
[self.view addSubview:_HUD];
_HUD.mode = MBProgressHUDModeDeterminate;
_HUD.delegate = self;
_HUD.labelText = @"等待中";
[_HUD showWhileExecuting:@selector(ProgressBar) onTarget:self withObject:nil animated:YES];
}
// 进度条 计算
- (void)ProgressBar {
// 进度指示器,从0.0到1.0,默认值为0.0
float progress = 0.0f;
while (progress < 1.0f) {
progress += 0.01f;
_HUD.progress = progress;
// usleep函数能把进程挂起一段时间, 单位是微秒(千分之一毫秒)
usleep(50000);
}
}
-
2.3 文字 加 菊花
// 通常情况 文字 加 菊花
- (void)GeneralButtonAction {
//初始化进度框,置于当前的View当中
self.HUD = [[MBProgressHUD alloc] initWithView:self.view];
[self.view addSubview:_HUD];
// 如果设置此属性则当前的view置于后台
_HUD.dimBackground = YES;
// 设置对话框文字
_HUD.labelText = @"加载中";
// 细节文字
_HUD.detailsLabelText = @"请耐心等待";
// 显示对话框
[_HUD showAnimated:YES whileExecutingBlock:^{
// 对话框显示时需要执行的操作
sleep(3);
}
// 在HUD被隐藏后的回调
completionBlock:^{
// 操作执行完后取消对话框
[_HUD removeFromSuperview];
_HUD = nil;
}];
}
-
2.4 自定义动图:MBProgressHUDModeCustomView
// 自定义 动图
- (void)CustomButtonAction{
// 自定义view
self.HUD = [[MBProgressHUD alloc] initWithView:self.view];
// 取消背景框
self.HUD.color = [UIColor whiteColor];
[self.view addSubview:_HUD];
UIImageView *images = [[UIImageView alloc] initWithFrame:CGRectMake(0, 0, 200, 300)];
NSMutableArray *imageArray = [[NSMutableArray alloc] init];
for(int i = 1; i < 8 ; i++){
NSString *imgName = [NSString stringWithFormat:@"%d.tiff",i];
[imageArray addObject:[UIImage imageNamed:imgName]];
}
images.animationDuration = 0.7;
images.animationImages = imageArray;
// 开始播放
[images startAnimating];
// 自定义
_HUD.mode = MBProgressHUDModeCustomView;
_HUD.delegate = self;
_HUD.customView = images;
[_HUD show:YES];
// 延迟
[_HUD hide:YES afterDelay:2];
}
-
2.5 默认使用的系统自带指示器:MBProgressHUDModeIndeterminate;
-
2.6 进度条:MBProgressHUDModeDeterminateHorizontalBar
-
2.7 圆环:MBProgressHUDModeAnnularDeterminate;
3、结构介绍
- MBProgressHUD由指示器,文本框,详情文本框,背景框4个部分组成.
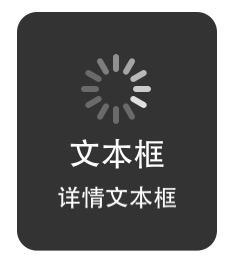
-
结构组成
// 文本框和其相关属性
@property (copy) NSString *labelText;
@property (MB_STRONG) UIFont* labelFont;
@property (MB_STRONG) UIColor* labelColor;
// 详情文本框和其相关属性
@property (copy) NSString *detailsLabelText;
@property (MB_STRONG) UIFont* detailsLabelFont;
@property (MB_STRONG) UIColor* detailsLabelColor;
// 背景框的透明度,默认值是0.8
@property (assign) float opacity;
// 背景框的颜色, 如果设置了这个属性,则opacity属性会失效,即不会有半透明效果
@property (MB_STRONG) UIColor *color;
// 背景框的圆角半径。默认值是10.0
@property (assign) float cornerRadius;
// 菊花的颜色,默认是白色
@property (MB_STRONG) UIColor *activityIndicatorColor;