1、创建与设置
// 1. 创建时不添加按钮
// 实例化 alertController 对象
UIAlertController *alertController = [UIAlertController alertControllerWithTitle:@"警告" message:@"真的要关闭 !" preferredStyle:UIAlertControllerStyleAlert];
// 显示,模态视图显示
[self presentViewController:alertController animated:YES completion:nil];
// 2. 创建时添加按钮等信息
// 实例化 UIAlertController 对象
UIAlertController *alertController = [UIAlertController alertControllerWithTitle:@"警告" message:@"真的要关闭 !" preferredStyle:UIAlertControllerStyleAlert];
// 创建按钮
UIAlertAction *cancelAction = [UIAlertAction actionWithTitle:@"取消" style:UIAlertActionStyleCancel handler:nil];
UIAlertAction *okAction = [UIAlertAction actionWithTitle:@"确定" style:UIAlertActionStyleDefault handler:nil];
UIAlertAction *noAction = [UIAlertAction actionWithTitle:@"考虑一下" style:UIAlertActionStyleDestructive handler:nil];
// 向 alertController 上添加按钮
[alertController addAction:cancelAction];
[alertController addAction:okAction];
[alertController addAction:noAction];
// 显示 alertController 视图
[self presentViewController:alertController animated:YES completion:nil];
// 设置警告框类型
/*
UIAlertControllerStyleActionSheet = 0, 上拉菜单,操作表,底部弹出
UIAlertControllerStyleAlert 对话框,警告,中间弹出
*/
UIAlertController *alertController = [UIAlertController alertControllerWithTitle:@"警告" message:@"真的要关闭 ?" preferredStyle:UIAlertControllerStyleAlert];
// 设置按钮类型
/*
UIAlertActionStyleDefault = 0, 默认蓝色按钮,可以有多个
UIAlertActionStyleCancel, 取消按钮,显示在左侧或最下边,有且只能有一个
UIAlertActionStyleDestructive 红色警示按钮,可以有多个,
《iOS 用户界面指南》要求所有的 “警示” 样式按钮都必须排名第一
*/
UIAlertAction *cancelAction = [UIAlertAction actionWithTitle:@"取消" style:UIAlertActionStyleCancel handler:nil];
// 设置按钮点击响应事件
UIAlertAction *closeAction = [UIAlertAction actionWithTitle:@"关闭" style:UIAlertActionStyleDestructive handler:^(UIAlertAction * _Nonnull action) {
/*
点击了按钮时响应的事件
*/
}];
// 添加输入框
/*
只能添加到 UIAlertControllerStyleAlert 上,可以添加多个
*/
[alertController addTextFieldWithConfigurationHandler:^(UITextField * _Nonnull textField) {
/*
设置添加的 textField 属性
*/
}];
// 添加点击按钮
/*
添加警告框上的按钮,可以添加多个
取消按钮在左侧或最下边,其它按钮按照添加的顺序排列
*/
[alertController addAction:cancelAction];
// 设置首选按钮
/*
必须在添加按钮(addAction)完成后设置,iOS 9 新添加
首选的按钮会有加粗效果,只能用在 UIAlertControllerStyleAlert 中
*/
alertController.preferredAction = okAction;
// 设置按钮激活状态
/*
YES 按钮激活,可点击。NO 按钮禁用,不可点击
*/
okAction.enabled = YES;
// 显示警告框视图
[self presentViewController:alertController animated:YES completion:nil];
// 设置警告框标题
alertController.title = @"登录";
// 设置警告框提示信息
alertController.message = @"请输入用户名和密码登录 !";
// 获取警告框标题
NSString *alertTitle = alertController.title;
// 获取警告框提示信息
NSString *alertMessage = alertController.message;
// 获取警告框类型,readonly
UIAlertControllerStyle alertStyle = alertController.preferredStyle;
// 获取所有输入框,readonly
NSArray<UITextField *> *textFieldArray = alertController.textFields;
// 获取所有按钮,readonly
NSArray<UIAlertAction *> *actionsArray = alertController.actions;
// 获取按钮标题,readonly
NSString *actionTitle = okAction.title;
// 获取按钮类型,readonly
UIAlertActionStyle actionStyle = okAction.style;
// 获取首选按钮
UIAlertAction *preferredAction = alertController.preferredAction;
// 获取按钮激活状态
BOOL actionEnabled = okAction.enabled;
2、常用代码
@interface GC_Demo_Controller : UIViewController
/**
* @brief 系统弹出信息输入框
*
* @param title 标题
* @param message 详细说明
* @param one_placeholder 信息输入框占位文字
* @param one_tf_info 信息输入框文字
* @param one_secureText 是否密码安全输入模式
* @param two_placeholder 信息输入框占位文字
* @param two_tf_info 信息输入框文字
* @param two_secureText 是否密码安全输入模式
* @param ok_title 确定按钮文字
* @param ok_block 点击确定后得到输入框的文字的回调
* @param cancel_title 取消按钮文字
* @param cancel_block 点击取消后得到的回调
*/
- (void)alert_Tf:(NSString *)title
Message:(NSString *)message
One_Placeholder:(NSString *)one_placeholder
One_Tf_Info:(NSString *)one_tf_info
One_SecureText:(BOOL)one_secureText
Two_Placeholder:(NSString *)two_placeholder
Two_Tf_Info:(NSString *)two_tf_info
Two_SecureText:(BOOL)two_secureText
OK_Title:(NSString *)ok_title
Ok_Tap:(void (^)(NSString *one_tf_Info, NSString *two_tf_Info))ok_block
Cancel_Title:(NSString *)cancel_title
Cancel_Tap:(void (^)(void))cancel_block;
/**
* @brief 系统弹出信息输入框
*
* @param title 标题
* @param message 详细说明
* @param placeholder 信息输入框占位文字
* @param tf_Info 信息输入框文字
* @param secure_text 是否密码安全输入模式
* @param ok_title 确定按钮文字
* @param ok_block 点击确定后得到输入框的文字的回调
* @param cancel_title 取消按钮文字
* @param cancel_block 点击取消后得到的回调
*/
- (void)alert_Tf:(NSString *)title
Message:(NSString *)message
Placeholder:(NSString *)placeholder
Tf_Info:(NSString *)tf_Info
SecureText:(BOOL)secure_text
OK_Title:(NSString *)ok_title
Ok_Tap:(void (^)(NSString *tf_Info))ok_block
Cancel_Title:(NSString *)cancel_title
Cancel_Tap:(void (^)(void))cancel_block;
/**
* @brief 系统弹出信息输入框
*
* @param title 标题
* @param message 详细说明
* @param placeholder 信息输入框占位文字
* @param tf_Info 信息输入框文字
* @param secure_text 是否密码安全输入模式
* @param keyboardType 键盘类型
* @param ok_title 确定按钮文字
* @param ok_block 点击确定后得到输入框的文字的回调
* @param cancel_title 取消按钮文字
* @param cancel_block 点击取消后得到的回调
*/
- (void)alert_Tf:(NSString *)title
Message:(NSString *)message
Placeholder:(NSString *)placeholder
Tf_Info:(NSString *)tf_Info
SecureText:(BOOL)secure_text
KeyboardType:(UIKeyboardType)keyboardType
OK_Title:(NSString *)ok_title
Ok_Tap:(void (^)(NSString *tf_Info))ok_block
Cancel_Title:(NSString *)cancel_title
Cancel_Tap:(void (^)(void))cancel_block;
/**
* @brief 系统弹出信息输入框
*
* @param title 标题
* @param message 详细说明
* @param placeholder 信息输入框占位文字
* @param secure_text 是否密码安全输入模式
* @param ok_block 点击确定后得到输入框的文字的回调
*/
- (void)alert_Tf:(NSString *)title
Message:(NSString *)message
Placeholder:(NSString *)placeholder
SecureText:(BOOL)secure_text
Ok_Tap:(void (^)(NSString *tf_Info))ok_block;
/**
* @brief 系统弹出信息输入框
*
* @param title 标题
* @param placeholder 信息输入框占位文字
* @param secure_text 是否密码安全输入模式
* @param ok_block 点击确定后得到输入框的文字的回调
*/
- (void)alert_Tf:(NSString *)title
Placeholder:(NSString *)placeholder
SecureText:(BOOL)secure_text
Ok_Tap:(void (^)(NSString *tf_Info))ok_block;
/**
* @brief 系统弹出信息输入框
*
* @param title 标题
* @param placeholder 信息输入框占位文字
* @param secure_text 是否密码安全输入模式
* @param ok_block 点击确定后得到输入框的文字的回调
*/
- (void)alert_Num_Tf:(NSString *)title
Placeholder:(NSString *)placeholder
SecureText:(BOOL)secure_text
Ok_Tap:(void (^)(NSString *tf_Info))ok_block;
/**
* @brief 系统弹出包含确认、取消按钮的提示框框
*
* @param title 操作提示标题
* @param message 操作提示信息
* @param confirn 确认按钮文字
* @param cancle 取消按钮文字
* @param ok_block 点击确认的回调
* @param cancle_block 点击取消的回调
*/
- (void)alert:(NSString *)title
Message:(NSString *)message
Confirn:(NSString *)confirn
Cancel:(NSString *)cancle
Confirn_Job:(void (^)(UIAlertAction *action))ok_block
Cancel_Job:(void (^)(UIAlertAction *action))cancle_block;
/**
* @brief 系统弹出简单的确认操作提示框,含标题
*
* @param title 确认操作提示标题
* @param message 确认操作提示信息
* @param confirn 确认按钮文字
*/
- (void)alert:(NSString *)title
Message:(NSString *)message
Confirn:(NSString *)confirn;
/**
* @brief 系统弹出简单的确认操作提示框,不含标题
*
* @param message 确认操作提示信息
* @param confirn 确认按钮文字
*/
- (void)alert:(NSString *)message
Confirn:(NSString *)confirn;
@end
@implementation GC_Demo_Controller
/**
* @brief 系统弹出信息输入框
*
* @param title 标题
* @param message 详细说明
* @param one_placeholder 信息输入框占位文字
* @param one_tf_info 信息输入框文字
* @param one_secureText 是否密码安全输入模式
* @param two_placeholder 信息输入框占位文字
* @param two_tf_info 信息输入框文字
* @param two_secureText 是否密码安全输入模式
* @param ok_title 确定按钮文字
* @param ok_block 点击确定后得到输入框的文字的回调
* @param cancel_title 取消按钮文字
* @param cancel_block 点击取消后得到的回调
*/
- (void)alert_Tf:(NSString *)title
Message:(NSString *)message
One_Placeholder:(NSString *)one_placeholder
One_Tf_Info:(NSString *)one_tf_info
One_SecureText:(BOOL)one_secureText
Two_Placeholder:(NSString *)two_placeholder
Two_Tf_Info:(NSString *)two_tf_info
Two_SecureText:(BOOL)two_secureText
OK_Title:(NSString *)ok_title
Ok_Tap:(void (^)(NSString *one_tf_Info, NSString *two_tf_Info))ok_block
Cancel_Title:(NSString *)cancel_title
Cancel_Tap:(void (^)(void))cancel_block {
UIAlertController *alert_c = [UIAlertController alertControllerWithTitle:title
message:message
preferredStyle:UIAlertControllerStyleAlert];
UIAlertAction *ok_action = [UIAlertAction actionWithTitle:ok_title style:UIAlertActionStyleDefault
handler:(void (^)(UIAlertAction *)) ^ {
UITextField *one_tf = [alert_c.view viewWithTag:1];
UITextField *two_tf = [alert_c.view viewWithTag:2];
ok_block(one_tf.text, two_tf.text);
}];
[alert_c addAction:ok_action];
[alert_c addTextFieldWithConfigurationHandler:^(UITextField *textField) {
textField.placeholder = one_placeholder;
textField.keyboardType = UIKeyboardTypeDefault;
textField.tag = 1;
textField.secureTextEntry = one_secureText;
textField.clearButtonMode = UITextFieldViewModeWhileEditing;
if (one_tf_info.length) {
textField.text = one_tf_info;
}
}];
[alert_c addTextFieldWithConfigurationHandler:^(UITextField *textField) {
textField.placeholder = two_placeholder;
textField.keyboardType = UIKeyboardTypeDefault;
textField.tag = 2;
textField.secureTextEntry = two_secureText;
textField.clearButtonMode = UITextFieldViewModeWhileEditing;
if (two_tf_info.length) {
textField.text = two_tf_info;
}
}];
if (cancel_title.length) {
UIAlertAction *cancel_action = [UIAlertAction actionWithTitle:cancel_title style:UIAlertActionStyleCancel
handler:^(UIAlertAction * _Nonnull action) {
cancel_block();
}];
[alert_c addAction:cancel_action];
}
[self presentViewController:alert_c animated:YES completion:nil];
}
/**
* @brief 系统弹出信息输入框
*
* @param title 标题
* @param message 详细说明
* @param placeholder 信息输入框占位文字
* @param tf_Info 信息输入框文字
* @param secure_text 是否密码安全输入模式
* @param ok_title 确定按钮文字
* @param ok_block 点击确定后得到输入框的文字的回调
* @param cancel_title 取消按钮文字
* @param cancel_block 点击取消后得到的回调
*/
- (void)alert_Tf:(NSString *)title
Message:(NSString *)message
Placeholder:(NSString *)placeholder
Tf_Info:(NSString *)tf_Info
SecureText:(BOOL)secure_text
OK_Title:(NSString *)ok_title
Ok_Tap:(void (^)(NSString *tf_Info))ok_block
Cancel_Title:(NSString *)cancel_title
Cancel_Tap:(void (^)(void))cancel_block {
[self alert_Tf:title Message:message
Placeholder:placeholder Tf_Info:tf_Info
SecureText:secure_text KeyboardType:UIKeyboardTypeDefault
OK_Title:ok_title Ok_Tap:ok_block
Cancel_Title:cancel_title Cancel_Tap:cancel_block];
}
/**
* @brief 系统弹出信息输入框
*
* @param title 标题
* @param message 详细说明
* @param placeholder 信息输入框占位文字
* @param tf_Info 信息输入框文字
* @param secure_text 是否密码安全输入模式
* @param keyboardType 键盘类型
* @param ok_title 确定按钮文字
* @param ok_block 点击确定后得到输入框的文字的回调
* @param cancel_title 取消按钮文字
* @param cancel_block 点击取消后得到的回调
*/
- (void)alert_Tf:(NSString *)title
Message:(NSString *)message
Placeholder:(NSString *)placeholder
Tf_Info:(NSString *)tf_Info
SecureText:(BOOL)secure_text
KeyboardType:(UIKeyboardType)keyboardType
OK_Title:(NSString *)ok_title
Ok_Tap:(void (^)(NSString *tf_Info))ok_block
Cancel_Title:(NSString *)cancel_title
Cancel_Tap:(void (^)(void))cancel_block {
UIAlertController *alert_c = [UIAlertController alertControllerWithTitle:title
message:message
preferredStyle:UIAlertControllerStyleAlert];
UIAlertAction *ok_action = [UIAlertAction actionWithTitle:ok_title style:UIAlertActionStyleDefault
handler:(void (^)(UIAlertAction *)) ^ {
UITextField *textField = [alert_c.view viewWithTag:1];
ok_block(textField.text);
}];
[alert_c addAction:ok_action];
[alert_c addTextFieldWithConfigurationHandler:^(UITextField *textField) {
textField.placeholder = placeholder;
textField.keyboardType = keyboardType;
textField.tag = 1;
textField.secureTextEntry = secure_text;
textField.clearButtonMode = UITextFieldViewModeWhileEditing;
if (tf_Info.length) {
textField.text = tf_Info;
}
}];
if (cancel_title.length) {
UIAlertAction *cancel_action = [UIAlertAction actionWithTitle:cancel_title style:UIAlertActionStyleCancel
handler:^(UIAlertAction * _Nonnull action) {
cancel_block();
}];
[alert_c addAction:cancel_action];
}
[self presentViewController:alert_c animated:YES completion:nil];
}
/**
* @brief 系统弹出信息输入框
*
* @param title 标题
* @param message 详细说明
* @param placeholder 信息输入框占位文字
* @param secure_text 是否密码安全输入模式
* @param ok_block 点击确定后得到输入框的文字的回调
*/
- (void)alert_Tf:(NSString *)title
Message:(NSString *)message
Placeholder:(NSString *)placeholder
SecureText:(BOOL)secure_text
Ok_Tap:(void (^)(NSString *tf_Info))ok_block {
[self alert_Tf:title
Message:message
Placeholder:placeholder
Tf_Info:@""
SecureText:secure_text
KeyboardType:UIKeyboardTypeDefault
OK_Title:MyLocal(@"SDR_0478")
Ok_Tap:ok_block
Cancel_Title:@"" Cancel_Tap:^{
}];
}
/**
* @brief 系统弹出信息输入框
*
* @param title 标题
* @param placeholder 信息输入框占位文字
* @param secure_text 是否密码安全输入模式
* @param ok_block 点击确定后得到输入框的文字的回调
*/
- (void)alert_Tf:(NSString *)title
Placeholder:(NSString *)placeholder
SecureText:(BOOL)secure_text
Ok_Tap:(void (^)(NSString *tf_Info))ok_block {
[self alert_Tf:title
Message:@""
Placeholder:placeholder
SecureText:secure_text
Ok_Tap:ok_block];
}
/**
* @brief 系统弹出信息输入框
*
* @param title 标题
* @param placeholder 信息输入框占位文字
* @param secure_text 是否密码安全输入模式
* @param ok_block 点击确定后得到输入框的文字的回调
*/
- (void)alert_Num_Tf:(NSString *)title
Placeholder:(NSString *)placeholder
SecureText:(BOOL)secure_text
Ok_Tap:(void (^)(NSString *tf_Info))ok_block {
[self alert_Tf:title Message:@""
Placeholder:placeholder
Tf_Info:@"" SecureText:secure_text
KeyboardType:UIKeyboardTypeNumberPad
OK_Title:MyLocal(@"SDR_0478")
Ok_Tap:ok_block
Cancel_Title:@"" Cancel_Tap:^{
}];
}
/**
* @brief 系统弹出包含确认、取消按钮的提示框框
*
* @param title 操作提示标题
* @param message 操作提示信息
* @param confirn 确认按钮文字
* @param cancle 取消按钮文字
* @param ok_block 点击确认的回调
* @param cancle_block 点击取消的回调
*/
- (void)alert:(NSString *)title
Message:(NSString *)message
Confirn:(NSString *)confirn
Cancel:(NSString *)cancle
Confirn_Job:(void (^)(UIAlertAction *action))ok_block
Cancel_Job:(void (^)(UIAlertAction *action))cancle_block {
UIAlertController *alert_c = [UIAlertController alertControllerWithTitle:title
message:message
preferredStyle:UIAlertControllerStyleAlert];
if (cancle && cancle.length) {
UIAlertAction *cancel_action = [UIAlertAction actionWithTitle:cancle style:UIAlertActionStyleCancel handler:cancle_block];
[alert_c addAction:cancel_action];
}
if (confirn && confirn.length) {
UIAlertAction *ok_action = [UIAlertAction actionWithTitle:confirn style:UIAlertActionStyleDefault handler:ok_block];
[alert_c addAction:ok_action];
}
// 显示,模态视图显示
[self presentViewController:alert_c animated:YES completion:nil];
}
/**
* @brief 系统弹出简单的确认操作提示框,含标题
*
* @param title 确认操作提示标题
* @param message 确认操作提示信息
* @param confirn 确认按钮文字
*/
- (void)alert:(NSString *)title
Message:(NSString *)message
Confirn:(NSString *)confirn {
[self alert:title
Message:message
Confirn:confirn
Cancel:@""
Confirn_Job:^(UIAlertAction * _Nonnull action) {
} Cancel_Job:^(UIAlertAction * _Nonnull action) {
}];
}
/**
* @brief 系统弹出简单的确认操作提示框,不含标题
*
* @param message 确认操作提示信息
* @param confirn 确认按钮文字
*/
- (void)alert:(NSString *)message
Confirn:(NSString *)confirn {
[self alert:@""
Message:message
Confirn:confirn
Cancel:@""
Confirn_Job:^(UIAlertAction * _Nonnull action) {
} Cancel_Job:^(UIAlertAction * _Nonnull action) {
}];
}
@end
3、富文本
-
3.1 源码
- 使用kvc的方式来自定义UIAlertController的样式。
UIAlertController *alert_c = [UIAlertController alertControllerWithTitle:@"标题" message:@"内容"
preferredStyle:UIAlertControllerStyleAlert];
// 使用富文本来改变alert的title字体大小和颜色
NSMutableAttributedString *title_attr = [[NSMutableAttributedString alloc] initWithString:@"这里是标题"];
[title_attr addAttribute:NSFontAttributeName value:[UIFont systemFontOfSize:24] range:NSMakeRange(0, 2)];
[title_attr addAttribute:NSForegroundColorAttributeName value:[UIColor redColor] range:NSMakeRange(0, 2)];
[alert_c setValue:title_attr forKey:@"attributedTitle"];
// 使用富文本来改变alert的message字体大小和颜色
// NSMakeRange(0, 2) 代表:从0位置开始 两个字符
NSMutableAttributedString *message_attr = [[NSMutableAttributedString alloc] initWithString:@"这里是正文信息"];
[message_attr addAttribute:NSFontAttributeName value:[UIFont systemFontOfSize:10] range:NSMakeRange(0, 6)];
[message_attr addAttribute:NSForegroundColorAttributeName value:[UIColor redColor] range:NSMakeRange(0, 2)];
[message_attr addAttribute:NSForegroundColorAttributeName value:[UIColor brownColor] range:NSMakeRange(3, 3)];
[alert_c setValue:message_attr forKey:@"attributedMessage"];
UIAlertAction *cancel_action = [UIAlertAction actionWithTitle:@"取消" style:UIAlertActionStyleCancel handler:nil];
// 设置按钮背景图片
UIImage *accessoryImage = [[UIImage imageNamed:@"selectRDImag.png"] imageWithRenderingMode:UIImageRenderingModeAlwaysOriginal];
[cancel_action setValue:accessoryImage forKey:@"image"];
// 设置按钮的title颜色
[cancel_action setValue:[UIColor lightGrayColor] forKey:@"titleTextColor"];
// 设置按钮的title的对齐方式
[cancel_action setValue:[NSNumber numberWithInteger:NSTextAlignmentLeft] forKey:@"titleTextAlignment"];
UIAlertAction *ok_action = [UIAlertAction actionWithTitle:@"确认" style:UIAlertActionStyleDefault handler:nil];
[alert_c addAction:ok_action];
[alert_c addAction:cancel_action];
[self presentViewController:alert_c animated:YES completion:nil];
-
3.2 效果
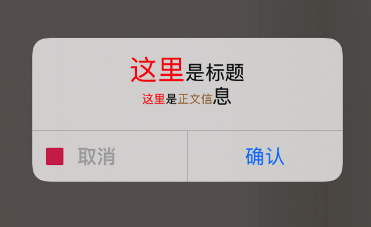
-
3.3 获取富文本属性
// 包含头文件
#import <objc/runtime.h>
@implementation GC_Demo_Controller
- (void)touchesBegan:(NSSet<UITouch *> *)touches withEvent:(UIEvent *)event {
[super touchesBegan:touches withEvent:event];
unsigned int outCount, index;
objc_property_t *properties = class_copyPropertyList([UIAlertController class], &outCount);
for (index = 0; index < outCount; index++) {
objc_property_t property = properties[index];
const char *char_name = property_getName(property);
NSString *c_name = [NSString stringWithCString:char_name encoding:NSUTF8StringEncoding];
const char *char_attr = property_getAttributes(property);
NSString *c_attr = [NSString stringWithCString:char_attr encoding:NSUTF8StringEncoding];
if ([c_name containsString:@"attribut"]) {
CHLog(@"属性名 = %@ 属性类型 = %@", c_name, c_attr)
}
}
}
@end