- 实际上 label 就是一个可以显示文字的视图控件。
1、Label 的创建
// 实例化 label 对象
UILabel *label = [[UILabel alloc] init];
// 将 label 加到父控件上
[self.view addSubview:label];
// 设置位置尺寸
label.frame = CGRectMake(10, 40, 100, 25);
2、Label 的设置
// label 文字
label.text = @"LabelLabelLabelLabelLabelLabelLabelLabelLabelLabel";
// 背景颜色
label.backgroundColor = [UIColor redColor];
// 文字颜色
label.textColor = [UIColor blackColor];
// 文字对齐方式
label.textAlignment = NSTextAlignmentCenter;
// 设置文字行数,0: 默认,行数不限
label.numberOfLines = 0;
// 文字阴影
// 阴影颜色
label.shadowColor = [UIColor greenColor];
// 阴影偏移量
label.shadowOffset = CGSizeMake(5, 5);
/*
==> 文字换行方式
NSLineBreakByWordWrapping = 0, 以单词为单位换行(最后一行显示不完以单词截断剩下的内容不显示也不会省略(没有...))
NSLineBreakByCharWrapping, 以字符为单位换行(最后一行显示不完以字符截断剩下的内容不显示也不会省略(没有...))
NSLineBreakByClipping, 以单词为单位换行(最后一行显示不完以字符截断剩下的内容不显示也不会省略(没有...))
NSLineBreakByTruncatingHead, 以单词换行,最后一行以字符截断,最后一行显示不完则省略最后一行的开头,以”...”显示
NSLineBreakByTruncatingTail, 以单词换行,最后一行以字符截断,最后一行显示不完则省略最后一行的中间,以”...”显示
NSLineBreakByTruncatingMiddle 以单词换行,最后一行以字符截断,最后一行显示不完则省略最后一行的最后,以”...”显示
*/
label.lineBreakMode = NSLineBreakByWordWrapping;
// 设置字体
// 系统样式
label.font = [UIFont systemFontOfSize:30];
// 加粗
label.font = [UIFont boldSystemFontOfSize:30];
// 倾斜
label.font = [UIFont italicSystemFontOfSize:30];
// 设置为指定字体类型的文字
label.font = [UIFont fontWithName:@"Zapfino" size:15];
// 获取系统字体库中的所有字体名称
NSArray *fontNameArray = [UIFont familyNames];
// 文字自动调整
label.adjustsFontSizeToFitWidth = YES;
// 文字自适应 frame 自适配文字,宽度不变,必须要在添加了显示文字之后设置
[label sizeToFit];
// 设置 label 每一行文字的最大宽度:在自动计算 label 高度时,为了保证计算出来的数值 跟 真正显示出来的效果 一致,若自动换行必须设置
label.preferredMaxLayoutWidth = [UIScreen mainScreen].bounds.size.width - 20;
3、可变属性 Label 的创建
NSString *str1 = @"NSMutable";
NSString *str2 = @"Attributed";
NSString *str3 = @"String";
// 设置 range 的大小
NSRange range1 = NSMakeRange(0, str1.length);
NSRange range2 = NSMakeRange(str1.length, str2.length);
NSRange range3 = NSMakeRange(str1.length + str2.length, str3.length);
// 实例化可变属性的字符串对象
NSMutableAttributedString *str = [[NSMutableAttributedString alloc]
initWithString:[NSString stringWithFormat:@"%@%@%@", str1, str2, str3]];
// 设置文字的颜色和文字的大小
[str addAttributes:@{NSForegroundColorAttributeName:[UIColor blueColor],
NSFontAttributeName:[UIFont boldSystemFontOfSize:15]}
range:range1];
[str addAttributes:@{NSForegroundColorAttributeName:[UIColor brownColor],
NSFontAttributeName:[UIFont systemFontOfSize:40]}
range:range2];
[str addAttributes:@{NSForegroundColorAttributeName:[UIColor greenColor],
NSFontAttributeName:[UIFont italicSystemFontOfSize:25]}
range:range3];
// 向 label 中添加文字
label.attributedText = str;
// frame 自适配文字,必须放在最后边,否则会不显示任何内容
[label sizeToFit];
4、常见需求
UIView *backView = [[UIView alloc] init];
[self.view addSubview:backView];
backView.frame = CGRectMake(10, 30, 300, 50);
backView.backgroundColor = [UIColor grayColor];
UILabel *label = [[UILabel alloc] init];
[backView addSubview:label];
label.frame = CGRectMake(0, 10, 120, 30);
label.backgroundColor = [UIColor yellowColor];
label.textColor = [UIColor greenColor];
label.text = @"Label Marquee";
// 子视图的范围不允许超过父视图的范围
backView.clipsToBounds = YES;
// 开始动画播放
// 设置 label 的起始位置
CGRect frame = label.frame;
frame.origin.x = backView.frame.size.width;
label.frame = frame;
// 开始简单动画
[UIView beginAnimations:nil context:nil];
// 设置动画时间(开始播放动画)
[UIView setAnimationDuration:5];
// 匀速
[UIView setAnimationCurve:UIViewAnimationCurveLinear];
// 无限重复
[UIView setAnimationRepeatCount:CGFLOAT_MAX];
// 设置 label 的结束位置
frame.origin.x = -frame.size.width;
label.frame = frame;
// 结束动画
[UIView commitAnimations];
// 一次播放动画结束
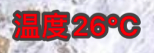
@interface UILabel (Extension)
@property (nonatomic) NSString *verticalText;
@end
#import "UILabel+Extension.h"
#import "objc/Runtime.h"
@implementation UILabel (Extension)
- (NSString *)verticalText {
// 利用runtime添加属性
return objc_getAssociatedObject(self, @selector(verticalText));
}
- (void)setVerticalText:(NSString *)verticalText {
objc_setAssociatedObject(self, &verticalText, verticalText, OBJC_ASSOCIATION_RETAIN_NONATOMIC);
NSMutableString *str = [[NSMutableString alloc] initWithString:verticalText];
NSInteger count = str.length;
for (NSInteger index = 1; index < count; index ++) {
[str insertString:@"\n" atIndex:index * 2 - 1];
}
self.text = str;
self.numberOfLines = 0;
}
@end