1、创建
// 创建
NSPopUpButton *popup_btn = [[NSPopUpButton alloc] init];
// NSPopUpButton的初始化方法之一,用于创建一个下拉菜单按钮。
// 参数buttonFrame表示按钮的位置和大小,参数flag表示是否允许下拉菜单的类型,
// 如果flag为YES,则按钮会展开下拉菜单;如果flag为NO,则按钮只显示当前选择的菜单项。
//NSPopUpButton *popup_btn = [[NSPopUpButton alloc] initWithFrame:NSMakeRect(20, 30, 150, 30) pullsDown:NO];
// 设置位置尺寸
popup_btn.frame = NSMakeRect(20, 30, 150, 30);
// 添加
[self.window.contentView addSubview:popup_btn];
// 指定NSPopUpButton是否显示弹出菜单。
// 如果设置为YES,则NSPopUpButton将显示一个下拉菜单。如果设置为NO,则NSPopUpButton将显示一个弹出菜单。
popup_btn.pullsDown = NO;
// 指定NSPopUpButton是否自动启用或禁用菜单项。
// 如果设置为YES,则NSPopUpButton将根据其绑定的数据源或其自身的状态自动启用或禁用菜单项。
// 如果设置为NO,则所有菜单项都将启用。
popup_btn.autoenablesItems = NO;
// 弹出菜单的相对位置。
popup_btn.preferredEdge = NSMinYEdge;
// 当NSPopUpButton准备弹出下拉菜单时发送。
// NSPopUpButtonWillPopUpNotification
[[NSNotificationCenter defaultCenter] addObserver:self selector:@selector(popUpButtonWillPopUpNotification:) name:NSPopUpButtonWillPopUpNotification object:popup_btn];
// 弹出菜单将要显示时的处理方法
- (void)popUpButtonWillPopUpNotification:(NSNotification *)notification {
// 在这里可以动态生成菜单项
NSPopUpButton *popUpButton = notification.object;
[popUpButton removeAllItems];
[popUpButton addItemWithTitle:@"上海"];
[popUpButton addItemWithTitle:@"北京"];
[popUpButton addItemWithTitle:@"广州"];
}
// 当不需要接收NSPopUpButtonWillPopUpNotification通知时,需要取消注册
- (void)dealloc {
[[NSNotificationCenter defaultCenter] removeObserver:self name:NSPopUpButtonWillPopUpNotification object:popup_btn];
}
// 返回NSPopUpButton中包含的选项数量。
NSInteger numberOfItems = popup_btn.numberOfItems;
NSLog(@"包含的选项数量 == %@", @(numberOfItems));
// 返回包含NSPopUpButton中所有选项的数组。
NSArray<NSMenuItem *> *itemArray = popup_btn.itemArray;
NSLog(@"所有选项的数组 == %@", itemArray);
// 返回NSPopUpButton中的最后一个选项。
NSMenuItem *lastItem = popup_btn.lastItem;
NSLog(@"lastItem == %@", lastItem);
// 返回当前下拉菜单中选中的选项。
NSMenuItem *selectedItem = popup_btn.selectedItem;
NSLog(@"返回当前下拉菜单中选中的选项 == %@", selectedItem.title);
// 返回当前下拉菜单中选中的选项的索引位置。
NSInteger indexOfSelectedItem = popup_btn.indexOfSelectedItem;
NSLog(@"选中的选项的索引位置 == %@", @(indexOfSelectedItem));
// 返回当前下拉菜单中选中的选项的标签。
NSInteger selectedTag = popup_btn.selectedTag;
NSLog(@"选中的选项的标签 == %@", @(selectedTag));
// 返回下拉菜单中所有选项的标题。
NSArray *itemTitles = popup_btn.itemTitles;
NSLog(@"所有选项的标题 == %@", itemTitles);
// 返回当前下拉菜单中选中的选项的标题。
NSString *titleOfSelectedItem = popup_btn.titleOfSelectedItem;
NSLog(@"选中的选项的标题 == %@", titleOfSelectedItem);
// 将具有指定索引的选项设置为被选择状态。
[popup_btn selectItem:selectedItem];
// 选择下拉菜单中指定索引位置的选项。
[popup_btn selectItemAtIndex:2];
// 选择下拉菜单中指定标题的选项。
[popup_btn selectItemWithTitle:@"广州"];
// 选择下拉菜单中指定标签的选项。
BOOL selecte_ok = [popup_btn selectItemWithTag:23];
NSLog(@"选择下拉菜单中指定标签的选项 == %@", @(selecte_ok));
// 创建菜单项
NSMenuItem *menuItem1 = [[NSMenuItem alloc] initWithTitle:@"北京" action:@selector(menu_item_Tap:) keyEquivalent:@""];
NSMenuItem *menuItem2 = [[NSMenuItem alloc] initWithTitle:@"天津" action:@selector(menu_item_Tap:) keyEquivalent:@""];
NSMenuItem *menuItem3 = [[NSMenuItem alloc] initWithTitle:@"佛山" action:@selector(menu_item_Tap:) keyEquivalent:@""];
// 将菜单项添加到菜单中
NSMenu *ns_menu = [[NSMenu alloc] initWithTitle:@"菜单"];
[ns_menu addItem:menuItem1];
[ns_menu addItem:menuItem2];
[ns_menu addItem:menuItem3];
// 指定NSPopUpButton弹出的菜单内容。
// 可以使用NSMenu对象或从nib文件中加载的NSMenuItem数组来设置。
popup_btn.menu = ns_menu;
- (void)menu_item_Tap:(NSMenuItem *)menu_item {
}
// 向NSPopUpButton中添加一个标题为指定字符串的选项。
[popup_btn addItemWithTitle:@"1234567"];
// 向NSPopUpButton中添加一个或多个标题为指定字符串数组中的字符串的选项。
[popup_btn addItemsWithTitles:@[@"北京", @"长沙", @"武汉"]];
// 向指定位置插入选项
[popup_btn insertItemWithTitle:@"香港" atIndex:2];
// 从NSPopUpButton中删除具有指定标题的选项。
[popup_btn removeItemWithTitle:@"北京"];
// 从NSPopUpButton中删除具有指定索引的选项。
[popup_btn removeItemAtIndex:1];
// 从NSPopUpButton中删除所有选项。
[popup_btn removeAllItems];
// 返回具有指定对象的选项的索引。
NSInteger index = [popup_btn indexOfItem:selectedItem];
// 返回具有指定标题的选项的索引。
index = [popup_btn indexOfItemWithTitle:@"桂林"];
// 返回具有指定标记的选项的索引。
index = [popup_btn indexOfItemWithTag:33];
// 返回具有指定代表对象的选项的索引。
index = [popup_btn indexOfItemWithRepresentedObject:selectedItem];
index = [popup_btn indexOfItemWithTarget:self andAction:@selector(popup_Tap)];
// 返回具有指定索引的选项。
selectedItem = [popup_btn itemAtIndex:2];
// 返回具有指定标题的选项。
selectedItem = [popup_btn itemWithTitle:@"西安"];
// 返回下拉菜单中指定索引位置的选项的标题。
NSString *title = [popup_btn itemTitleAtIndex:2];
NSLog(@"选项的标题 == %@", title);
// 置下拉菜单的标题。
[popup_btn setTitle:@"西藏"];
// 同步下拉菜单的标题和选中的选项。
[popup_btn synchronizeTitleAndSelectedItem];
2、自定义子项目
// 创建
NSPopUpButton *pop_btn = [[NSPopUpButton alloc] init];
// 位置尺寸
pop_btn.frame = CGRectMake(50, 200, 120, 50);
// 添加
[self.window.contentView addSubview:pop_btn];
// pullsDown 设置为 YES 只有向下的箭头
pop_btn.pullsDown = NO;
// 当交互事件发生时,是否禁用选项
pop_btn.autoenablesItems = YES;
// 弹出菜单的位置
pop_btn.preferredEdge = NSRectEdgeMaxX;
NSArray *pop_Items = @[@"广州", @"深圳", @"桂林", @"广州", @"深圳", @"桂林", @"广州", @"深圳", @"桂林", @"广州", @"深圳", @"桂林"];
for (NSInteger index = 0; index < pop_Items.count; index++) {
GC_MenuItem *menu_Item = [[GC_MenuItem alloc ] init];
menu_Item.title = pop_Items[index];
[pop_btn.menu addItem:menu_Item];
menu_Item.target = self;
menu_Item.action = @selector(pop_Tap:);
}
// 点击选中事件
- (void)pop_Tap:(NSPopUpButton *)pop_btn {
pop_btn.title = pop_btn.selectedItem.title;
NSLog(@"NSPopUpButton == %@", pop_btn.title);
}
-
2.2 自定义类
#import <Cocoa/Cocoa.h>
NS_ASSUME_NONNULL_BEGIN
@interface GC_MenuItem : NSMenuItem
@end
NS_ASSUME_NONNULL_END
#import "GC_MenuItem.h"
@interface GC_MenuView : NSView
@end
@implementation GC_MenuView
- (void)touchesBeganWithEvent:(NSEvent *)event {
[super touchesBeganWithEvent:event];
NSLog(@"1234567890");
}
@end
@implementation GC_MenuItem
- (void)setTitle:(NSString *)title {
[super setTitle:title];
GC_MenuView *menu_view = [[GC_MenuView alloc] init];
menu_view.frame = NSMakeRect(0, 0, 200, 30);
[self setView:menu_view];
menu_view.wantsLayer = YES;
if ([title isEqualToString:@"深圳"]) {
menu_view.layer.backgroundColor = [NSColor redColor].CGColor;
}
else if ([title isEqualToString:@"广州"]) {
menu_view.layer.backgroundColor = [NSColor lightGrayColor].CGColor;
}
else {
menu_view.layer.backgroundColor = [NSColor blueColor].CGColor;
}
}
@end
-
2.3 效果
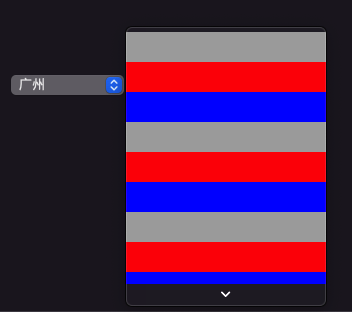