0、前言
- NSComboBox是Cocoa框架中的一个控件,用于在下拉列表中显示可选项,允许用户从中选择一个选项或输入自定义内容。
- 它可以用于许多场景,例如选择器、搜索框、标签选择等。
1、创建示例
// 创建
NSComboBox *combo_box = [[NSComboBox alloc] init];
// 添加
[self.window.contentView addSubview:combo_box];
// 设置位置尺寸
combo_box.frame = NSMakeRect(20, 400, 150, 30);
// 用于控制是否显示垂直滚动条。
// 如果设置为YES,则当下拉列表中的项目数超过numberOfVisibleItems时,将会显示垂直滚动条。
combo_box.hasVerticalScroller = NO;
// 用于设置下拉列表中单元格之间的间距。
combo_box.intercellSpacing = NSMakeSize(60, 20);
// 用于设置下拉列表中每个项目的高度。
combo_box.itemHeight = 80;
// 用于控制下拉按钮的边框样式。如果设置为YES,则下拉按钮将显示边框。
combo_box.buttonBordered = YES;
// 用于设置下拉列表中可见的项目数。
combo_box.numberOfVisibleItems = 3;
// 用于获取下拉列表中项目的数量。
NSInteger numberOfItems = combo_box.numberOfItems;
NSLog(@"用于获取下拉列表中项目的数量 == %@", @(numberOfItems));
// 用于控制是否启用自动完成功能。如果设置为YES,则用户在输入时会自动匹配下拉列表中的项目。
combo_box.completes = NO;
// 用于获取当前选中的项目的索引值。
NSInteger indexOfSelectedItem = combo_box.indexOfSelectedItem;
NSLog(@"用于获取当前选中的项目的索引值 == %@", @(indexOfSelectedItem));
// 返回指定索引处的项的对象值。
id item_obj = [combo_box itemObjectValueAtIndex:2];
NSLog(@"item_obj == %@", item_obj);
// 用于获取当前选中的项目的值。
id objectValueOfSelectedItem = combo_box.objectValueOfSelectedItem;
NSLog(@"用于获取当前选中的项目的值 == %@", objectValueOfSelectedItem);
// 返回指定对象值的项的索引。如果没有找到匹配的项,则返回NSNotFound。
NSInteger index = [combo_box indexOfItemWithObjectValue:@(23)];
NSLog(@"index == %@", @(index));
// 选择下拉列表中指定索引处的项目。
[combo_box selectItemAtIndex:2];
// 取消选择下拉列表中指定索引处的项目。
[combo_box deselectItemAtIndex:2];
// 选择下拉列表中与指定对象值匹配的项目。
[combo_box selectItemWithObjectValue:@(23)];
// 将下拉列表中指定索引处的项目滚动到顶部。
[combo_box scrollItemAtIndexToTop:2];
// 将下拉列表中指定索引处的项目滚动到可见区域。
[combo_box scrollItemAtIndexToVisible:2];
// 重新加载数据,可以用于更新下拉列表中的选项内容。
[combo_box reloadData];
// 通知下拉列表的数据源中的项目数量已更改,以便更新下拉列表的大小。
[combo_box noteNumberOfItemsChanged];
// 用于设置下拉列表中的项目值。可以通过NSArray或NSMutableArray对象来设置。
NSArray *objectValues = combo_box.objectValues;
NSLog(@"用于设置下拉列表中的项目值 == %@", objectValues);
// 用于设置NSComboBox的代理对象,以便处理与下拉列表相关的事件和操作。
combo_box.delegate = self;
#pragma mark - NSComboBoxDelegate
// 表示下拉列表即将弹出时调用的方法,可以在该方法中进行一些初始化操作或者修改下拉列表的内容。
- (void)comboBoxWillPopUp:(NSNotification *)notification {
}
// 表示下拉列表即将消失时调用的方法,可以在该方法中进行一些清理操作或者记录用户所选的内容。
- (void)comboBoxWillDismiss:(NSNotification *)notification {
}
// 示下拉列表中选择的内容已经发生变化时调用的方法,可以在该方法中响应用户的选择,并进行相应的操作。
- (void)comboBoxSelectionDidChange:(NSNotification *)notification {
NSComboBox *comboBox = notification.object;
NSInteger selectedIndex = comboBox.indexOfSelectedItem;
NSLog(@"comboBoxSelectionDidChange selected item %@", self.datas[selectedIndex]);
}
// 表示用户正在改变下拉列表中选择的内容时调用的方法,可以在该方法中实时响应用户的操作,例如根据用户输入实时更新下拉列表的内容。
- (void)comboBoxSelectionIsChanging:(NSNotification *)notification {
NSComboBox *comboBox = notification.object;
NSInteger selectedIndex = comboBox.indexOfSelectedItem;
NSLog(@"comboBoxSelectionIsChanging selected item %@",self.datas[selectedIndex]);
}
-
1.6 效果
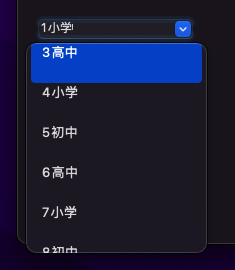
2、不使用数据源
// 设置是否使用数据源来提供下拉列表中的项目。
combo_box.usesDataSource = NO;
// 向下拉列表中添加一个项目。
[combo_box addItemWithObjectValue:@(23)];
// 向下拉列表中添加多个项目。
[combo_box addItemsWithObjectValues:@[@(23), @"桂林"]];
// 在指定索引处插入一个项目。
[combo_box insertItemWithObjectValue:@(23) atIndex:2];
// 从下拉列表中删除指定项目。
[combo_box removeItemWithObjectValue:@(23)];
// 从下拉列表中删除指定索引处的项目。
[combo_box removeItemAtIndex:2];
// 从下拉列表中删除所有项目。
[combo_box removeAllItems];
// 添加选中事件
combo_box.target = self;
combo_box.action = @selector(comboBox_Selecte:);
- (void)comboBox_Selecte:(NSNotification *)notification {
NSComboBox *comboBox = [notification object];
NSInteger selectedIndex = [comboBox indexOfSelectedItem];
NSString *selectedItem = [comboBox itemObjectValueAtIndex:selectedIndex];
NSLog(@"Selected item: %@", selectedItem);
}
3、使用数据源
self.datas = @[@"小学", @"初中", @"高中",];
// 设置是否使用数据源来提供下拉列表中的项目。
combo_box.usesDataSource = YES;
// 用于设置NSComboBox的数据源对象,以便提供下拉列表中的项目数据。
combo_box.dataSource = self;
#pragma mark - NSComboBoxDataSource
// 这个方法用于返回下拉列表中的选项数量。
// 你需要在这个方法中返回一个整数,代表下拉列表中的选项数量。
// 例如,如果你的下拉列表中有5个选项,那么你应该返回5。
- (NSInteger)numberOfItemsInComboBox:(NSComboBox *)aComboBox {
return self.datas.count;
}
// 这个方法用于返回下拉列表中指定索引位置的选项。
// 你需要在这个方法中根据索引位置返回一个对象,这个对象代表下拉列表中指定索引位置的选项。
// 例如,如果你的下拉列表中有5个选项,你可以使用一个数组来存储这5个选项,
// 然后在这个方法中返回数组中指定索引位置的对象。
- (id)comboBox:(NSComboBox *)aComboBox objectValueForItemAtIndex:(NSInteger)index {
return self.datas[index];
}
// 开启时就选中第几个项目
- (NSUInteger)comboBox:(NSComboBox *)comboBox indexOfItemWithStringValue:(NSString *)string {
return 2;
}
// 用于在用户输入文本时提供自动补全功能。
// 举个例子,假设我们有一个 NSComboBox 对象,其中包含了一些字符串,比如 "apple", "banana", "orange"。
// 用户在输入框中输入 "a" 时,我们希望自动补全为 "apple"。
- (nullable NSString *)comboBox:(NSComboBox *)comboBox completedString:(NSString *)string {
// 遍历所有的字符串,寻找以输入的字符串开头的字符串
for (NSString *item in comboBox.objectValues) {
if ([item hasPrefix:string]) {
return item;
}
}
return nil;
}
4、API说明
// 下拉列表将要弹出通知
APPKIT_EXTERN NSNotificationName NSComboBoxWillPopUpNotification;
// 下拉列表将要关闭通知
APPKIT_EXTERN NSNotificationName NSComboBoxWillDismissNotification;
// 下拉列表选中行改变通知
APPKIT_EXTERN NSNotificationName NSComboBoxSelectionDidChangeNotification;
// 下拉列表选中行正在改变通知
APPKIT_EXTERN NSNotificationName NSComboBoxSelectionIsChangingNotification;
@protocol NSComboBoxDataSource <NSObject>
@optional
/**
* @brief 返回下拉列表中项目的数量
*
* @param comboBox 下拉列表
* @return 下拉列表中项目的数量
*/
- (NSInteger)numberOfItemsInComboBox:(NSComboBox *)comboBox NS_SWIFT_UI_ACTOR;
/**
* @brief 返回指定索引处的对象值
*
* @param comboBox 下拉列表
* @param index 要检索的对象值的索引
* @return 指定索引处的对象值,或者为nil(如果该对象不存在)
*/
- (nullable id)comboBox:(NSComboBox *)comboBox objectValueForItemAtIndex:(NSInteger)index NS_SWIFT_UI_ACTOR;
/**
* @brief 返回包含指定字符串值的下拉列表中的项目的索引
*
* @param comboBox 下拉列表
* @param string 用于查找项目的字符串值
* @return 包含指定字符串值的下拉列表中的项目的索引,或者为NSNotFound(如果没有找到)
*/
- (NSUInteger)comboBox:(NSComboBox *)comboBox indexOfItemWithStringValue:(NSString *)string NS_SWIFT_UI_ACTOR;
/**
* @brief 在用户输入完成后返回最匹配的已知字符串值
*
* @param comboBox 下拉列表
* @param string 用户输入完成的字符串
* @return 最匹配的已知字符串值
*/
- (nullable NSString *)comboBox:(NSComboBox *)comboBox completedString:(NSString *)string NS_SWIFT_UI_ACTOR;
@end
@protocol NSComboBoxDelegate <NSTextFieldDelegate>
@optional
/**
* @brief 下拉列表即将弹出时调用
*
* @param notification 通知对象,包含下拉列表
*/
- (void)comboBoxWillPopUp:(NSNotification *)notification NS_SWIFT_UI_ACTOR;
/**
* @brief 下拉列表即将关闭时调用
*
* @param notification 通知对象,包含下拉列表
*/
- (void)comboBoxWillDismiss:(NSNotification *)notification NS_SWIFT_UI_ACTOR;
/**
* @brief 下拉列表选中项发生更改时调用
*
* @param notification 通知对象,包含下拉列表
*/
- (void)comboBoxSelectionDidChange:(NSNotification *)notification NS_SWIFT_UI_ACTOR;
/**
* @brief 下拉列表选中项正在更改时调用
*
* @param notification 通知对象,包含下拉列表
*/
- (void)comboBoxSelectionIsChanging:(NSNotification *)notification NS_SWIFT_UI_ACTOR;
@end
@interface NSComboBox : NSTextField
/** 是否显示纵向滚动条 */
@property BOOL hasVerticalScroller;
/** 单元格之间的间隔大小 */
@property NSSize intercellSpacing;
/** 每个项的高度 */
@property CGFloat itemHeight;
/** 可见的行数 */
@property NSInteger numberOfVisibleItems;
/** 按钮是否有边框 */
@property(getter=isButtonBordered) BOOL buttonBordered;
/**
* @brief 重新加载下拉列表的数据
*/
- (void)reloadData;
/**
* @brief 通知下拉列表中的项目数量已更改
*/
- (void)noteNumberOfItemsChanged;
/** 是否使用数据源的方式来填充下拉列表 */
@property BOOL usesDataSource;
/**
* @brief 滚动 NSComboBox 中指定索引的项至顶部
*
* @param index 要滚动的项的索引
*/
- (void)scrollItemAtIndexToTop:(NSInteger)index;
/**
* @brief 将 NSComboBox 中指定索引的项滚动到可见区域内
*
* @param index 要滚动的项的索引
*/
- (void)scrollItemAtIndexToVisible:(NSInteger)index;
/**
* @brief 选中 NSComboBox 中指定索引的项
*
* @param index 要选中的项的索引
*/
- (void)selectItemAtIndex:(NSInteger)index;
/**
* @brief 取消选中 NSComboBox 中指定索引的项
*
* @param index 要取消选中的项的索引
*/
- (void)deselectItemAtIndex:(NSInteger)index;
/** 当前选中项在下拉列表中的索引 */
@property(readonly) NSInteger indexOfSelectedItem;
/** 下拉列表中的项目数量 */
@property(readonly) NSInteger numberOfItems;
/** 是否允许自动完成输入内容 */
@property BOOL completes;
/** 下拉列表代理对象,用于响应下拉列表相关事件 */
@property(nullable, weak) id<NSComboBoxDelegate> delegate;
/** 下拉列表数据源对象,提供下拉列表的数据内容 */
@property(nullable, assign) id<NSComboBoxDataSource> dataSource;
/**
* @brief 添加一个带有对象值的项到NSComboBox中
*
* @param object 要添加的对象值
*/
- (void)addItemWithObjectValue:(id)object;
/**
* @brief 通过NSArray中的对象值批量添加项到NSComboBox中
*
* @param objects 包含要添加的对象值的NSArray
*/
- (void)addItemsWithObjectValues:(NSArray *)objects;
/**
* @brief 在指定索引处插入一个带有对象值的项到NSComboBox中
*
* @param object 要插入的对象值
* @param index 要插入项的索引
*/
- (void)insertItemWithObjectValue:(id)object atIndex:(NSInteger)index;
/**
* @brief 从NSComboBox中删除具有指定对象值的项
*
* @param object 要删除的对象值
*/
- (void)removeItemWithObjectValue:(id)object;
/**
* @brief 删除指定索引处的项
*
* @param index 要删除的项的索引
*/
- (void)removeItemAtIndex:(NSInteger)index;
/**
* @brief 删除NSComboBox中的所有项
*/
- (void)removeAllItems;
/**
* @brief 选择具有指定对象值的项
*
* @param object 要选择的对象值
*/
- (void)selectItemWithObjectValue:(nullable id)object;
/**
* @brief 检索具有指定索引的项的对象值
*
* @param index 要检索对象值的项的索引
*
* @return 指定索引处项的对象值
*/
- (id)itemObjectValueAtIndex:(NSInteger)index;
/** 当前选中项的对象值 */
@property(nullable, readonly, strong) id objectValueOfSelectedItem;
/**
* @brief 查找给定对象值的列表项的索引
*
* @param object 要查找的对象值
*
* @return 对象值所在的列表项的索引,如果未找到,则返回NSNotFound
*/
- (NSInteger)indexOfItemWithObjectValue:(id)object;
/** 下拉列表框中的选项数组 */
@property(readonly, copy) NSArray *objectValues;
@end