TreeMap相关
Map接口
Map集合的特点
1.能够存储唯一的列的数据(唯一,不可重复) Set
2.能够存储可以重复的数据(可重复) List
3.值的顺序取决于键的顺序
4.键和值都是可以存储null元素的
TreeMap
本质上就是红黑树
的实现
1.每个节点要么是红色,要么是黑色。
2.根节点必须是黑色
3.每个叶子节点【NIL】是黑色
4.每个红色节点的两个子节点必须是黑色
5.任意节点到每个叶子节点的路径包含相同数量的黑节点
K key; // key
V value; // 值
Entry<K,V> left; // 左子节点
Entry<K,V> right; // 右子节点
Entry<K,V> parent; // 父节点
boolean color = BLACK; // 节点的颜色 默认是黑色
put为例
public V put(K key, V value) {
// 将root赋值给局部变量 null
Entry<K,V> t = root;
if (t == null) {
// 初始操作
// 检查key是否为空
compare(key, key); // type (and possibly null) check
// 将要添加的key、 value封装为一个Entry对象 并赋值给root
root = new Entry<>(key, value, null);
size = 1;
modCount++;
return null;
}
int cmp;
Entry<K,V> parent; // 父节点
// split comparator and comparable paths
Comparator<? super K> cpr = comparator; // 获取比较器
if (cpr != null) {
// 一直找到插入节点的父节点
do {
// 将root赋值给了parent
parent = t;
// 和root节点比较值得大小
cmp = cpr.compare(key, t.key);
if (cmp < 0)
// 将父节点的左子节点付给了t
t = t.left;
else if (cmp > 0)
t = t.right; // 将父节点的右节点付给了t
else
// 直接和父节点的key相等,直接修改值
return t.setValue(value);
} while (t != null);
}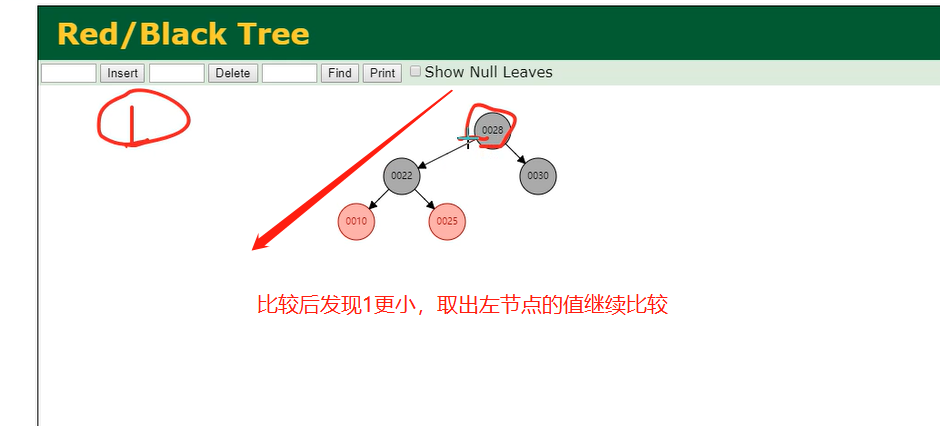
else {
if (key == null)
throw new NullPointerException();