- 引入 jar 包,包含了如下的相关 jar
- Spring 开发的基本 jar 包
- 数据库驱动
- Spring JDBC模板的 jar 包
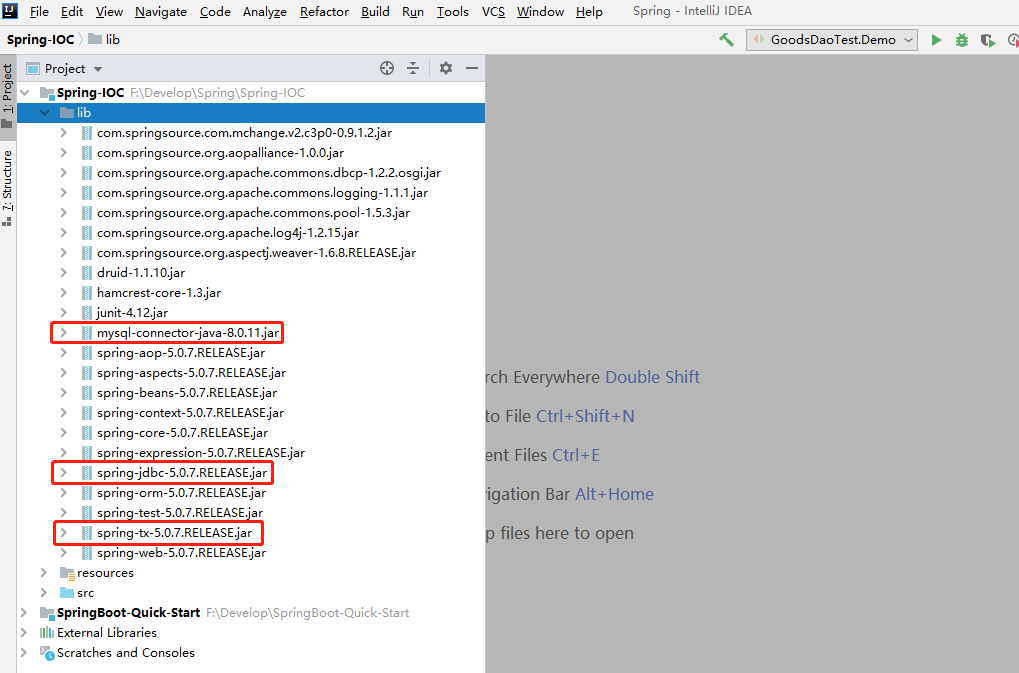
创建数据库和表
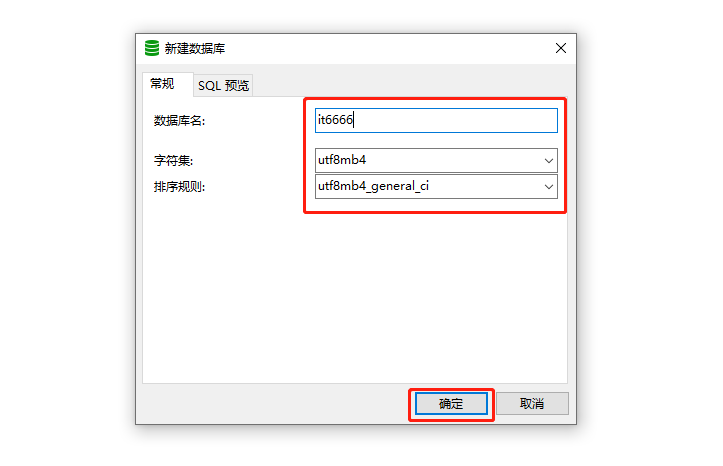
drop table if exists account;
create table account(
id int primary key auto_increment,
name varchar(20),
money double
);
使用JDBC模板
/**
* @author: BNTang
**/
public class Demo {
@Test
public void insertDemo() {
// 1.创建连接池
DriverManagerDataSource dataSource = new DriverManagerDataSource();
// 2.配置相关连接信息
dataSource.setDriverClassName("com.mysql.cj.jdbc.Driver");
dataSource.setUrl("jdbc:mysql://localhost:3306/it6666?useUnicode=true&characterEncoding=utf8&serverTimezone=GMT%2B8&useSSL=false");
dataSource.setUsername("root");
dataSource.setPassword("root");
// 3.创建JDBC模板
JdbcTemplate jdbcTemplate = new JdbcTemplate(dataSource);
jdbcTemplate.update("insert into account values (null, ?, ?)", "BNTang",10000d);
}
}
将连接池和模板交给Spring管理
配置文件配置Bean
- 修改
applicationContext.xml
...
<bean id="dataSource" class="org.springframework.jdbc.datasource.DriverManagerDataSource">
<property name="driverClassName" value="com.mysql.cj.jdbc.Driver"/>
<property name="url" value="jdbc:mysql://localhost:3306/it6666?useUnicode=true&characterEncoding=utf8&serverTimezone=GMT%2B8&useSSL=false"/>
<property name="username" value="root"/>
<property name="password" value="root"/>
</bean>
<bean id="jdbcTemplate" class="org.springframework.jdbc.core.JdbcTemplate">
<property name="dataSource" ref="dataSource"/>
</bean>
...
/**
* @author: BNTang
**/
@RunWith(SpringJUnit4ClassRunner.class)
@ContextConfiguration("classpath:applicationContext.xml")
public class Demo {
@Resource
private JdbcTemplate jdbcTemplate;
@Test
public void insertDemo() {
jdbcTemplate.update("insert into account values (null, ?, ?)", "BNTang",10000d);
}
}
使用开源连接池
DBCP
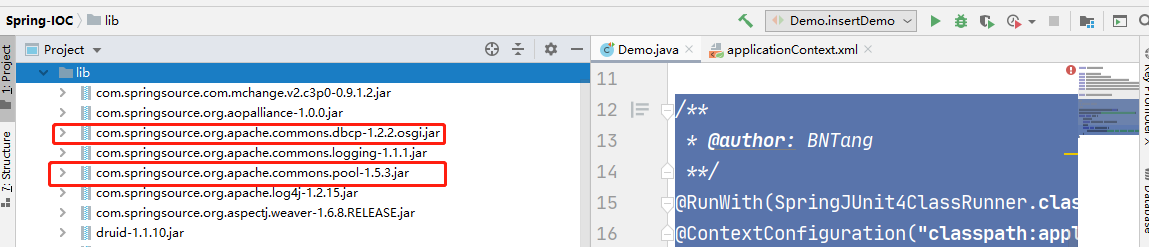
- 配置 DBCP 连接池
- 修改
applicationContext.xml
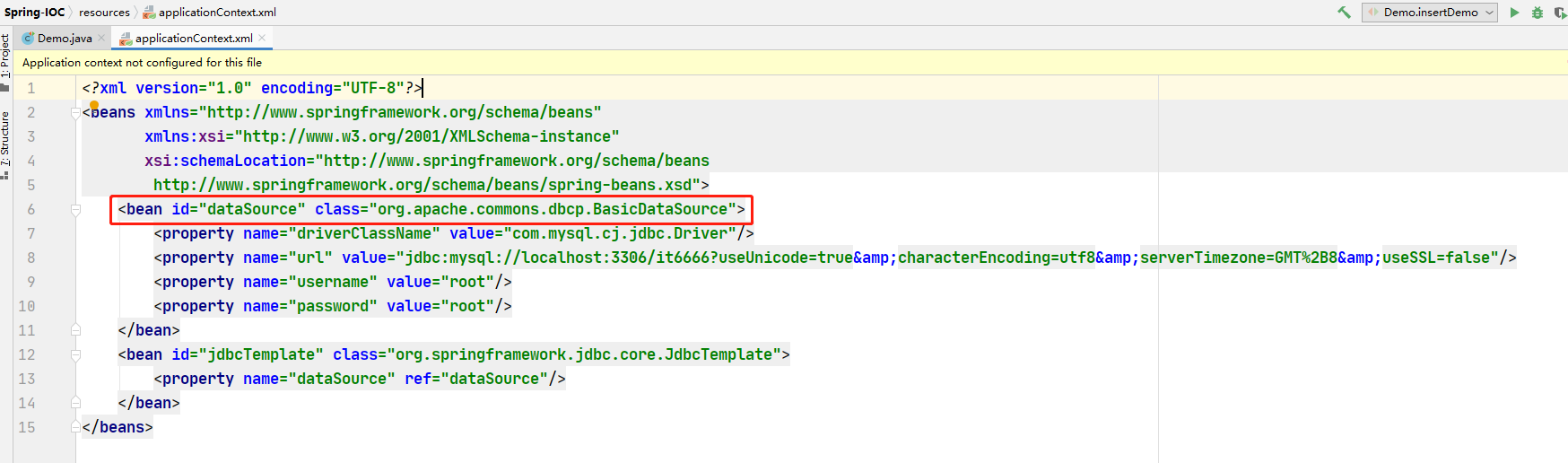
...
<bean id="dataSource" class="org.apache.commons.dbcp.BasicDataSource">
</bean>
...
C3P0
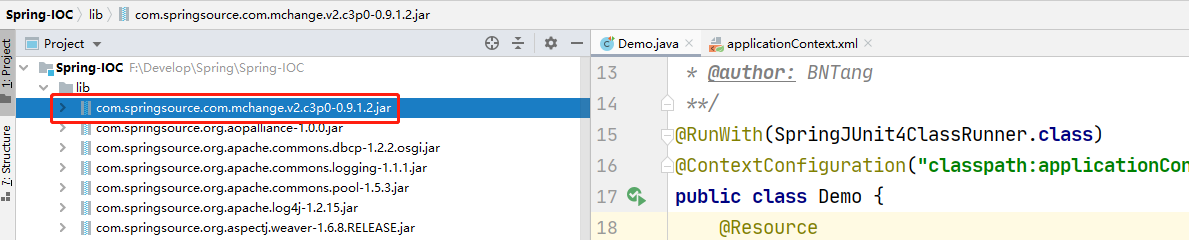
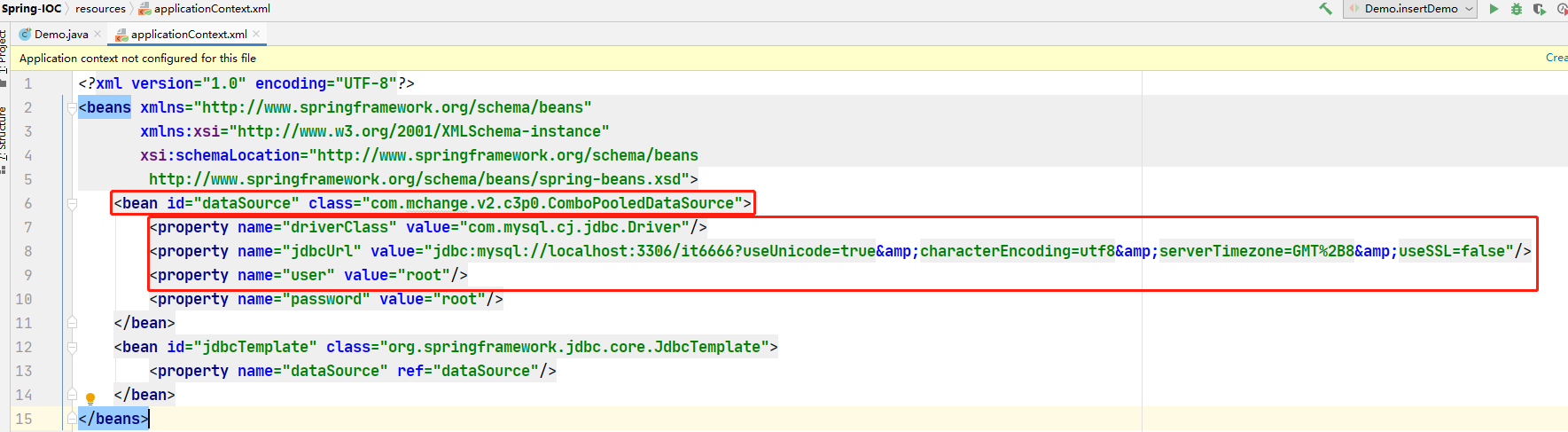
...
<bean id="dataSource" class="com.mchange.v2.c3p0.ComboPooledDataSource">
<property name="driverClass" value="com.mysql.cj.jdbc.Driver"/>
<property name="jdbcUrl" value="jdbc:mysql://localhost:3306/it6666?useUnicode=true&characterEncoding=utf8&serverTimezone=GMT%2B8&useSSL=false"/>
<property name="user" value="root"/>
...
</bean>
...
Druid
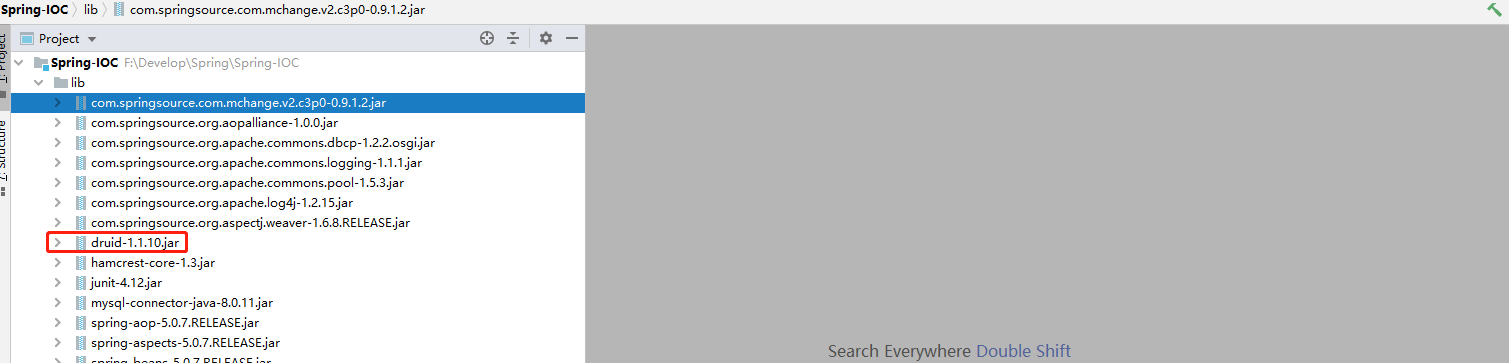
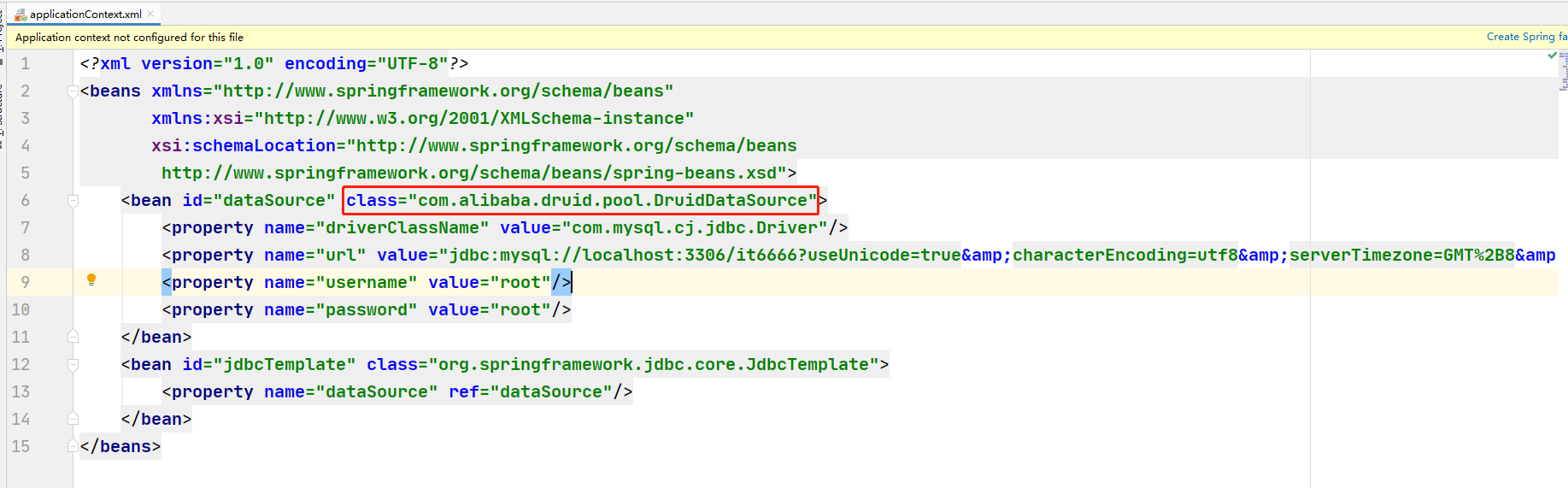
...
<bean id="dataSource" class="com.alibaba.druid.pool.DruidDataSource">
</bean>
...
使用属性文件配置数据库连接信息

jdbc.driverClassName=com.mysql.cj.jdbc.Driver
jdbc.url=jdbc:mysql://localhost:3306/it6666?useUnicode=true&characterEncoding=utf8&serverTimezone=GMT%2B8&useSSL=false
jdbc.username=root
jdbc.password=root
- 改造
applicationContext.xml
- 引入属性配置文件
<bean>的方式配置
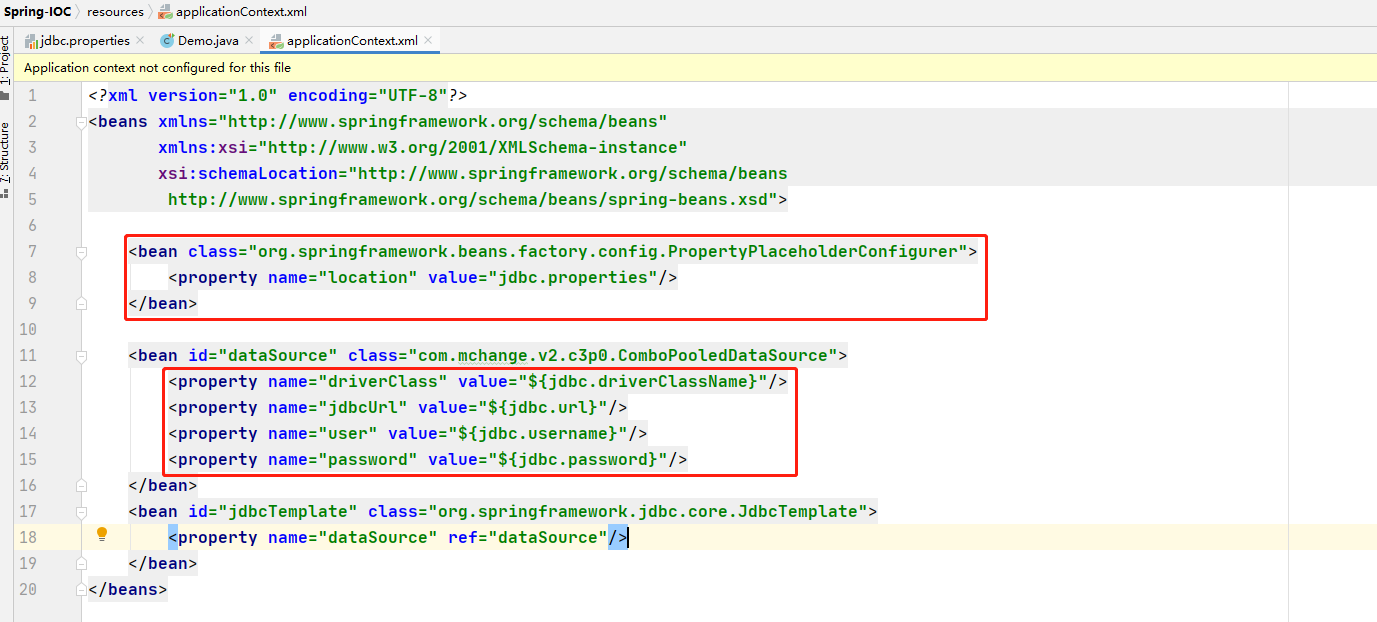
...
<bean class="org.springframework.beans.factory.config.PropertyPlaceholderConfigurer">
<property name="location" value="jdbc.properties"/>
</bean>
<bean id="dataSource" class="com.mchange.v2.c3p0.ComboPooledDataSource">
<property name="driverClass" value="${jdbc.driverClassName}"/>
<property name="jdbcUrl" value="${jdbc.url}"/>
<property name="user" value="${jdbc.username}"/>
<property name="password" value="${jdbc.password}"/>
</bean>
...
<context/>的方式配置
- 修改
applicationContext.xml
- 加入
context
约束
xmlns:context="http://www.springframework.org/schema/context"
http://www.springframework.org/schema/context
http://www.springframework.org/schema/context/spring-context.xsd
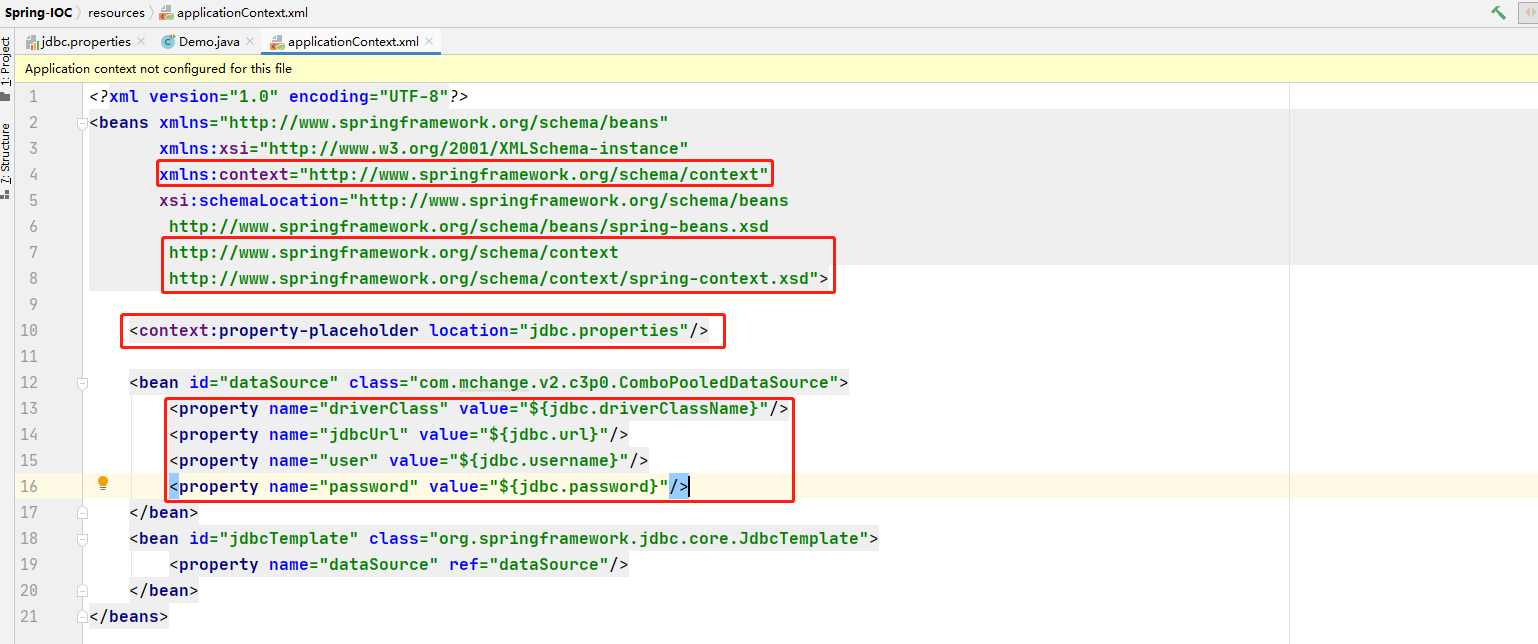
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:context="http://www.springframework.org/schema/context"
xsi:schemaLocation="http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans.xsd
http://www.springframework.org/schema/context
http://www.springframework.org/schema/context/spring-context.xsd">
<context:property-placeholder location="jdbc.properties"/>
<bean id="dataSource" class="com.mchange.v2.c3p0.ComboPooledDataSource">
<property name="driverClass" value="${jdbc.driverClassName}"/>
<property name="jdbcUrl" value="${jdbc.url}"/>
<property name="user" value="${jdbc.username}"/>
<property name="password" value="${jdbc.password}"/>
</bean>
...