前置通知
- 在目标方法执行之前进行操作
- 也就是在目标方法执行之前执行你指定的方法,可以在目标方法执行之前你可以做一些操作
<bean id="goodsDao" class="top.it6666.dao.impl.GoodsDaoImpl"/>
<bean id="myAspect" class="top.it6666.aspect.MyAspect"/>
<aop:config>
<aop:pointcut id="pointcut" expression="execution(* top.it6666.dao.impl.GoodsDaoImpl.save(..))"/>
<aop:aspect ref="myAspect">
<aop:before method="checkPrivilege" pointcut-ref="pointcut"/>
</aop:aspect>
</aop:config>
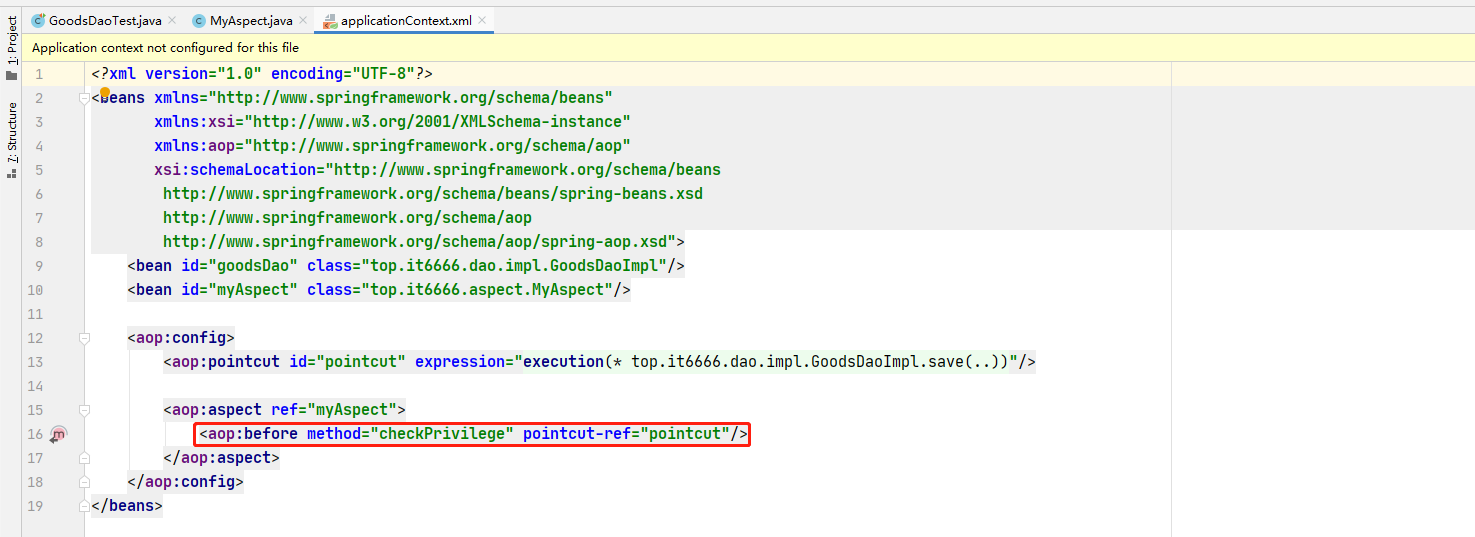
后置通知
- 在目标方法执行之后进行操作
- 修改
MyAspect
加入一下内容:
/**
* @author: BNTang
**/
public class MyAspect {
public void writeLog(){
System.out.println("record log");
}
}
- 修改
applicationContext.xml
<bean id="goodsDao" class="top.it6666.dao.impl.GoodsDaoImpl"/>
<bean id="myAspect" class="top.it6666.aspect.MyAspect"/>
<aop:config>
<aop:pointcut id="pointcut" expression="execution(* top.it6666.dao.impl.GoodsDaoImpl.save(..))"/>
<aop:aspect ref="myAspect">
<aop:after-returning method="writeLog" pointcut-ref="pointcut"/>
</aop:aspect>
</aop:config>
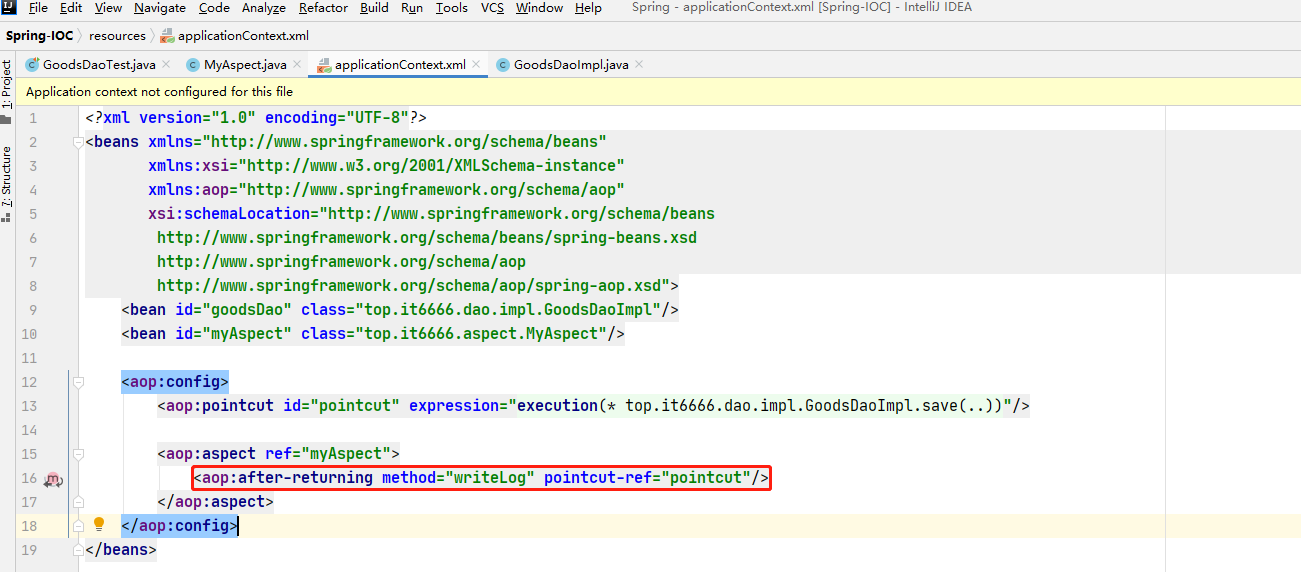
环绕通知
- 在目标方法执行之前和之后进行操作
- 在目标方法执行之前和之后做什么操作
- 修改
MyAspect
,修改之后的内容如下:
/**
* @author: BNTang
**/
public class MyAspect {
public Object around(ProceedingJoinPoint joinPoint) throws Throwable {
System.out.println("before method");
Object proceed = joinPoint.proceed();
System.out.println("after method");
return proceed;
}
}
- 修改
applicationContext.xml
<bean id="goodsDao" class="top.it6666.dao.impl.GoodsDaoImpl"/>
<bean id="myAspect" class="top.it6666.aspect.MyAspect"/>
<aop:config>
<aop:pointcut id="pointcut" expression="execution(* top.it6666.dao.impl.GoodsDaoImpl.save(..))"/>
<aop:aspect ref="myAspect">
<aop:around method="around" pointcut-ref="pointcut"/>
</aop:aspect>
</aop:config>
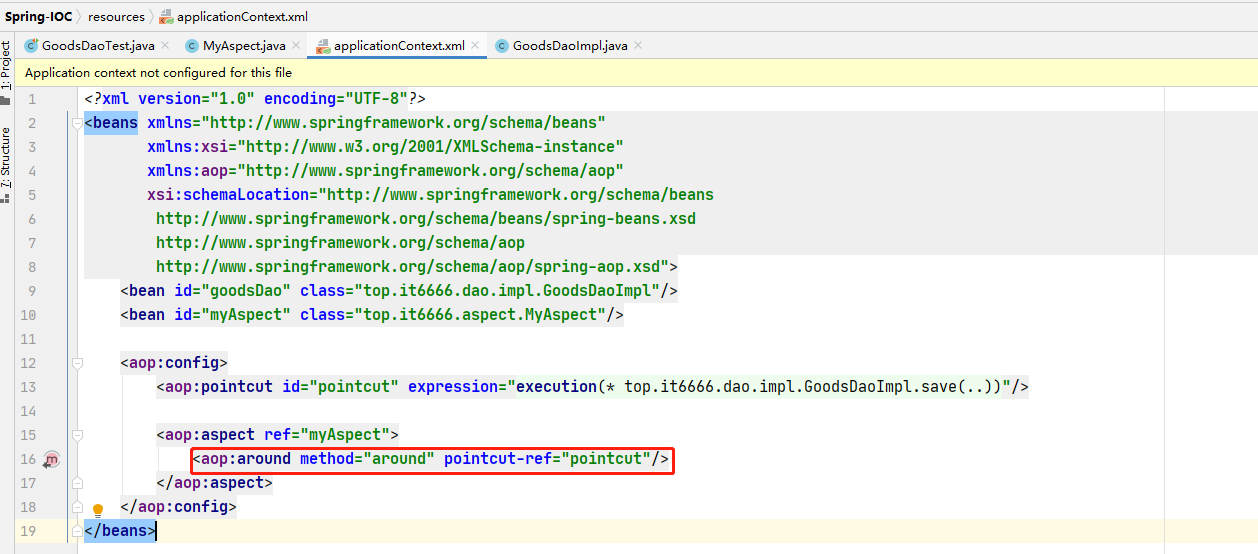
异常抛出通知
- 程序出现异常时进行操作
- 程序出现了异常要做什么操作
- 修改
MyAspect
,修改过后内容如下:
/**
* @author: BNTang
**/
public class MyAspect {
public void exceptionNotice(Throwable throwable){
System.out.println("happen exception = " + throwable.getMessage());
}
}
- 修改
applicationContext.xml
<bean id="goodsDao" class="top.it6666.dao.impl.GoodsDaoImpl"/>
<bean id="myAspect" class="top.it6666.aspect.MyAspect"/>
<aop:config>
<aop:pointcut id="pointcut" expression="execution(* top.it6666.dao.impl.GoodsDaoImpl.save(..))"/>
<aop:aspect ref="myAspect">
<aop:after-throwing method="exceptionNotice" pointcut-ref="pointcut" throwing="throwable"/>
</aop:aspect>
</aop:config>
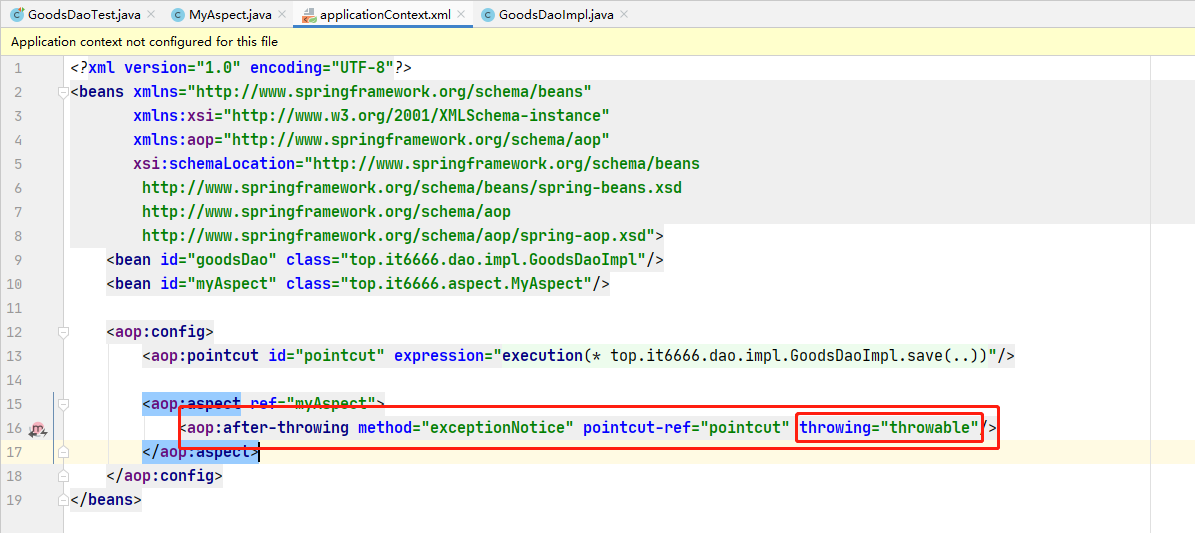
/**
* @author: BNTang
**/
public class GoodsDaoImpl implements GoodsDao {
@Override
public void save() {
System.out.println(1 / 0);
System.out.println("save goods");
}
}
- 测试类代码还是之前的,这里只是为了演示这个异常抛出通知特意抛出的
最终通知
- 无论代码是否有异常,都会执行
- 也就是目标方法执行之后,在执行的过程当中不管有没有异常都会执行的方法,也就是要做的操作
- 修改
MyAspect
,修改之后的内容如下:
/**
* @author: BNTang
**/
public class MyAspect {
public void end(){
System.out.println("end inform");
}
}
- 修改
applicationContext.xml
<bean id="goodsDao" class="top.it6666.dao.impl.GoodsDaoImpl"/>
<bean id="myAspect" class="top.it6666.aspect.MyAspect"/>
<aop:config>
<aop:pointcut id="pointcut" expression="execution(* top.it6666.dao.impl.GoodsDaoImpl.save(..))"/>
<aop:aspect ref="myAspect">
<aop:after method="end" pointcut-ref="pointcut"/>
</aop:aspect>
</aop:config>
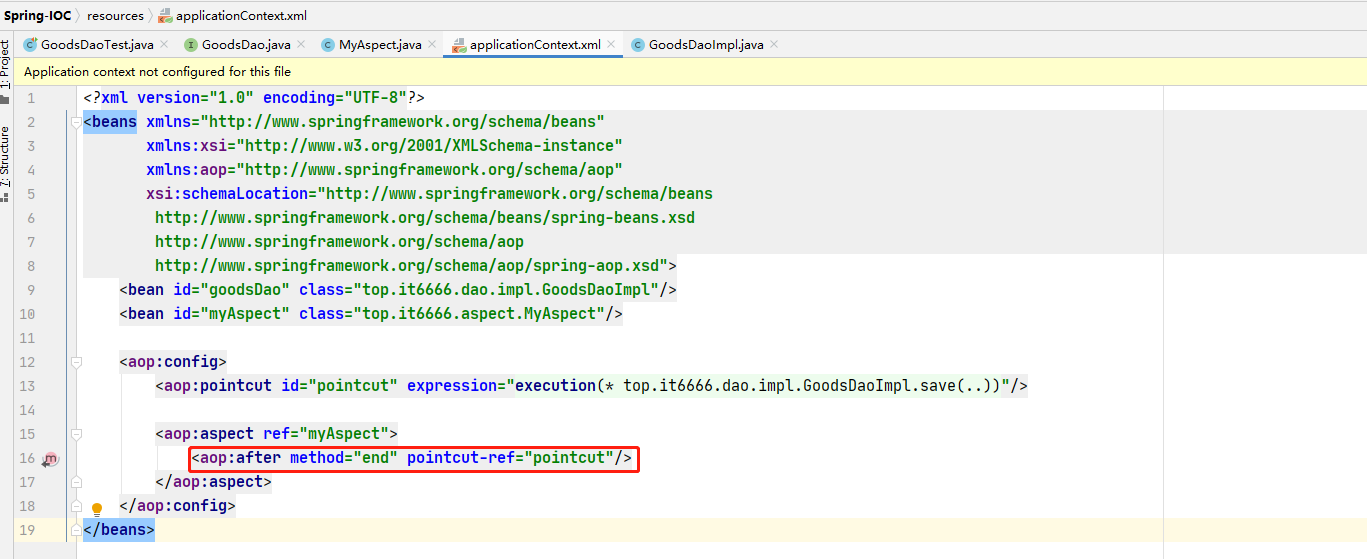