WPF系列
一、ListView绑定数据源XML
//前端代码
1 <Window x:Class="ListView读取XML数据.MainWindow" xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation" 2 xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml" Title="MainWindow" 3 Height="350" Width="525"> 4 <Window.Resources> 5 <XmlDataProvider x:Key="Student" Source="Book.xml"> 6 </XmlDataProvider> 7 </Window.Resources> 8 <Grid> 9 <ListView ItemsSource="{Binding Source={StaticResource Student},XPath=/Person/Information}"> 10 </ListView> 11 </Grid> 12 </Window>
//XML文件
1 <?xml version="1.0" encoding="utf-8" ?> 2 <Person> 3 <Information> 4 <Name>张三</Name> 5 <Age>22</Age> 6 <Sex>男</Sex> 7 </Information> 8 <Information> 9 <Name>李四</Name> 10 <Age>25</Age> 11 <Sex>男</Sex> 12 </Information> 13 </Person>
//效果截图
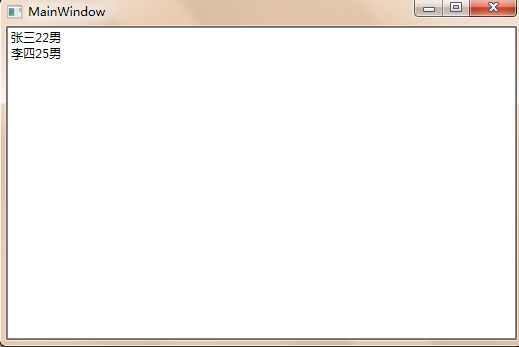
二、保存数据到XML
1 XmlDocument xml = new XmlDocument(); 2 string str_path = @"J:\WPF\test\XMLData.xml"; 3 XmlNode root = null; 4 if (File.Exists(str_path)) 5 { 6 xml.Load(str_path); 7 root = xml.SelectSingleNode("Person"); 8 } 9 10 XmlNodeList nodelist = xml.SelectNodes("/Person/Student/Infor"); 11 XmlElement inf,adr, stu, info, UN, IP, DB; 12 bool bl_exist = true; 13 foreach (XmlNode node in nodelist) 14 { 15 if (node.ChildNodes[1].InnerText == (ServerName.Text + ".").ToString() && 16 node.ChildNodes[0].InnerText == (UserName.Text + ".").ToString() && 17 node.ChildNodes[2].InnerText ==DataName.Text) 18 { 19 bl_exist = false; 20 break; 21 } 22 } 23 if (bl_exist) 24 { 25 stu = xml.CreateElement("Student"); //创建元素 26 info = xml.CreateElement("Infor"); 27 UN = xml.CreateElement("UserName"); 28 IP = xml.CreateElement("IP"); 29 DB = xml.CreateElement("DataBaseName"); 30 31 inf = xml.CreateElement("Information"); 32 adr = xml.CreateElement("Address"); 33 adr.InnerText = (UserName.Text + "." + ServerName.Text + "." + DataName.Text).ToString(); 34 root.AppendChild(inf); 35 inf.AppendChild(adr); 36 37 UN.InnerText = (UserName.Text + ".").ToString(); 38 IP.InnerText = (ServerName.Text + ".").ToString(); 39 DB.InnerText = DataName.Text; 40 stu.AppendChild(info); 41 info.AppendChild(UN); 42 info.AppendChild(IP); 43 info.AppendChild(DB); 44 root.AppendChild(stu); 45 MessageBox.Show("数据保存成功"); 46 } 47 xml.Save(str_path);
三、页面切换出现淡入淡出效果和图表控件页面重新加载动画
1、把Window窗口改为NavigationWindow导航切换窗口,添加源文件Market.xaml <NavigationWindow x:Class="MainWindow" xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation" xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml" Title="MainWindow" Height="1080" Width="3840" WindowState="Maximized" Source="Market.xaml" WindowStyle="None" ShowsNavigationUI="False" ResizeMode="NoResize" Navigating="NavigationWindow_Navigating" MouseLeftButtonDown="NavigationWindow_MouseLeftButtonDown"> </NavigationWindow> 2、下一个页面切换的时候用以下代码就可实现: NavigationService.Navigate(new Uri("Power.xaml", UriKind.Relative));
3、淡入淡出效果
private void NavigationWindow_Navigating(object sender, NavigatingCancelEventArgs e) { if (Content != null && !_allowDirectNavigation) { e.Cancel = true; _navArgs = e; this.IsHitTestVisible = false; DoubleAnimation da = new DoubleAnimation(0.3d, new Duration(TimeSpan.FromMilliseconds(100))); da.Completed += FadeOutCompleted; this.BeginAnimation(OpacityProperty, da); } _allowDirectNavigation = false; } private void FadeOutCompleted(object sender, EventArgs e) { (sender as AnimationClock).Completed -= FadeOutCompleted; this.IsHitTestVisible = true; _allowDirectNavigation = true; switch (_navArgs.NavigationMode) { case NavigationMode.New: if (_navArgs.Uri == null) { NavigationService.Navigate(_navArgs.Content); } else { NavigationService.Navigate(_navArgs.Uri); } break; case NavigationMode.Back: NavigationService.GoBack(); break; case NavigationMode.Forward: NavigationService.GoForward(); break; case NavigationMode.Refresh: NavigationService.Refresh(); break; } Dispatcher.BeginInvoke(DispatcherPriority.Loaded, (ThreadStart)delegate() { DoubleAnimation da = new DoubleAnimation(1.0d, new Duration(TimeSpan.FromMilliseconds(100))); this.BeginAnimation(OpacityProperty, da); }); } private bool _allowDirectNavigation = false; private NavigatingCancelEventArgs _navArgs = null;