自学python-opencv(5)图像梯度
import cv2
import numpy
def lapalac_demo(image):
#dst=cv2.Laplacian(image,cv2.CV_64F)
#laps=cv2.convertScaleAbs(dst)
#自适应的卷积核
kernel=numpy.array([[0,1,0],[1,-4,1],[0,1,0]])
dst=cv2.filter2D(image,cv2.CV_64F,kernel=kernel)
laps=cv2.convertScaleAbs(dst)
cv2.imshow("lapalac_demo",laps)
def sobel_demo(image):
#sobel_x=cv2.Sobel(image,cv2.CV_64F,1,0)
#sobel_y=cv2.Sobel(image,cv2.CV_64F,0,1)
sobel_x=cv2.Scharr(image,cv2.CV_64F,1,0)
sobel_y=cv2.Scharr(image,cv2.CV_64F,0,1)
sobelx=cv2.convertScaleAbs(sobel_x)
sobely=cv2.convertScaleAbs(sobel_y)
cv2.imshow("sobelx",sobelx)
cv2.imshow("sobely",sobely)
#gramma 修正值 G=根号(Gx^2+Gy^2)
sobelxy=cv2.addWeighted(sobelx,0.5,sobely,0.5,0)
cv2.imshow("sobelxy",sobelxy)
print("-----------hi python-------------")
img=cv2.imread("./images/lantian1.jpg")
cv2.namedWindow("imput images",cv2.WINDOW_AUTOSIZE)
cv2.imshow("imput images",img)
lapalac_demo(img)
cv2.waitKey()
cv2.destroyWindow('all')
------------------------------------------------------------------------------------------------------
图像梯度
1】sobel算子的理论基础
sobel算子
dst=cv2.Sobel(src,ddepth,dx,dy,[ksize])
dst:计算结果
src:原始图像
ddepth:处理结果图像深度
dx:x轴方向
dy:y轴方向
ksize:核大小
dst=cv2.convertScaleAbs(src,[,alpha[,beta]])
作用:将原始图像src转换为256色位图
目标图像=cv2.convertScaleAbs(原始图像)
最好是分别计算
dst=dx+dy
函数
dst=cv2.addWeighted(src1,alpha,src2,beta,gamma)
功能:计算两幅图像的权重和
dst:计算结果
src1:源图像1
alpha:源图像1的系数
src2:源图像2
beta:源图像2的系数
gamma:修正值
--import cv2
import numpy as np
def sober_xy(image):
soberx=cv2.Sobel(image,cv2.CV_64F,1,0)
soberx=cv2.convertScaleAbs(soberx)
sobery=cv2.Sobel(image,cv2.CV_64F,0,1)
sobery=cv2.convertScaleAbs(sobery)
xy=cv2.addWeighted(soberx,0.5,sobery,0.5,0)
cv2.imshow("soberxy",xy)
1】sobel算子的理论基础
sobel算子
dst=cv2.Sobel(src,ddepth,dx,dy,[ksize])
dst:计算结果
src:原始图像
ddepth:处理结果图像深度
dx:x轴方向
dy:y轴方向
ksize:核大小
dst=cv2.convertScaleAbs(src,[,alpha[,beta]])
作用:将原始图像src转换为256色位图
目标图像=cv2.convertScaleAbs(原始图像)
最好是分别计算
dst=dx+dy
函数
dst=cv2.addWeighted(src1,alpha,src2,beta,gamma)
功能:计算两幅图像的权重和
dst:计算结果
src1:源图像1
alpha:源图像1的系数
src2:源图像2
beta:源图像2的系数
gamma:修正值
--import cv2
import numpy as np
def sober_xy(image):
soberx=cv2.Sobel(image,cv2.CV_64F,1,0)
soberx=cv2.convertScaleAbs(soberx)
sobery=cv2.Sobel(image,cv2.CV_64F,0,1)
sobery=cv2.convertScaleAbs(sobery)
xy=cv2.addWeighted(soberx,0.5,sobery,0.5,0)
cv2.imshow("soberxy",xy)
def scharr_xy(image):
#x的方向
scharrx=cv2.Scharr(image,cv2.CV_64F,1,0)
#y的方向
scharry=cv2.Scharr(image,cv2.CV_64F,1,0)
scharrx=cv2.convertScaleAbs(scharrx)#转回uint8
scharry=cv2.convertScaleAbs(scharry)
#权重
scharrxy=cv2.addWeighted(scharrx,0.5,scharry,0.5,0)
cv2.imshow("scharrxy", scharrxy)
img=cv2.imread("./images/lantian1.jpg")
cv2.imshow("liaoxiansheng",img)
sober_xy(img)
scharr_xy(img)
cv2.waitKey()
cv2.destroyAllWindows()
----------------------------
----------------------------------
2】scharr算子函数
水平 |左边减去右边|
[-3, 0, -3]
[-10,0, 10]
[-3, 0, 3]
垂直 |下边减去上边|
[-3,-10,-3]
[0, 0, 0]
[3, 10, 3]
sobel算子
水平 |左边减去右边|
[-1,0, -1]
[-2,0, 2]
[-1,0, 1]
垂直 |下边减去上边|
[-1, -2,-1]
[0, 0, 0]
[1, 2, 1]
------------------------------------
dst=Scharr(src,ddpeth,dx,dy)
dst,计算结果
src,原始图像
ddepth,处理结果图像深度
dx,x轴方向
dy,y轴方向
dst=cv2.converScaleAbs(dst)
scharrxy=cv2.addWeighted(scharrx,alpha,sharry,beta,gamma)
--------------------------------------
dst=cv2.Sobel(src,ddepth,dx,dy,-1) 注:卷积核不可能为负数的
等价于dst=Scharr(src,ddpeth,dx,dy)
------------------------------
import cv2
import numpy as np
img=cv2.imread("./images/lantian1.jpg")
#x的方向
scharrx=cv2.Scharr(img,cv2.CV_64F,1,0)
#y的方向
scharry=cv2.Scharr(img,cv2.CV_64F,1,0)
scharrx=cv2.convertScaleAbs(scharrx)#转回uint8
scharry=cv2.convertScaleAbs(scharry)
#权重
scharrxy=cv2.addWeighted(scharrx,0.5,scharry,0.5,0)
cv2.imshow("liaoxiansheng",img)
cv2.imshow("x",scharrx)
cv2.imshow("y",scharry)
cv2.imshow("xy",scharrxy)
cv2.waitKey()
cv2.destroyAllWindows()
---------------
比较:
梯度就是计算边界值
-------------------
3】laplacian算子 类似二阶sobel导数 两次
卷积核为:
[0, 1, 0]
[1,-4, 1]
[0, 1, 0]
------------------
[p1, p2, p3]
[p4, p5, p6]
[p7, p8, p9]
p5新=(p2+p4+p6+p8)-4*p5图中
---------------------------------
二阶导数:|左-右|+|左-右|+|下-上|-|下-上|
dst=cv2.Laplacian(src,ddepth)
dst:结果图像
src:原始图像
ddepth:图像深度
这里使用cv2.CV_64F使得结果可以是负值,
dst=cv2.convertScaleAbs(src)
将原始图像调整256色位图 可以取绝对值
示例:目标图像=cv2.convertScaleAbs(原始图像)
scharrx=cv2.Scharr(image,cv2.CV_64F,1,0)
#y的方向
scharry=cv2.Scharr(image,cv2.CV_64F,1,0)
scharrx=cv2.convertScaleAbs(scharrx)#转回uint8
scharry=cv2.convertScaleAbs(scharry)
#权重
scharrxy=cv2.addWeighted(scharrx,0.5,scharry,0.5,0)
cv2.imshow("scharrxy", scharrxy)
img=cv2.imread("./images/lantian1.jpg")
cv2.imshow("liaoxiansheng",img)
sober_xy(img)
scharr_xy(img)
cv2.waitKey()
cv2.destroyAllWindows()
----------------------------
----------------------------------
2】scharr算子函数
水平 |左边减去右边|
[-3, 0, -3]
[-10,0, 10]
[-3, 0, 3]
垂直 |下边减去上边|
[-3,-10,-3]
[0, 0, 0]
[3, 10, 3]
sobel算子
水平 |左边减去右边|
[-1,0, -1]
[-2,0, 2]
[-1,0, 1]
垂直 |下边减去上边|
[-1, -2,-1]
[0, 0, 0]
[1, 2, 1]
------------------------------------
dst=Scharr(src,ddpeth,dx,dy)
dst,计算结果
src,原始图像
ddepth,处理结果图像深度
dx,x轴方向
dy,y轴方向
dst=cv2.converScaleAbs(dst)
scharrxy=cv2.addWeighted(scharrx,alpha,sharry,beta,gamma)
--------------------------------------
dst=cv2.Sobel(src,ddepth,dx,dy,-1) 注:卷积核不可能为负数的
等价于dst=Scharr(src,ddpeth,dx,dy)
------------------------------
import cv2
import numpy as np
img=cv2.imread("./images/lantian1.jpg")
#x的方向
scharrx=cv2.Scharr(img,cv2.CV_64F,1,0)
#y的方向
scharry=cv2.Scharr(img,cv2.CV_64F,1,0)
scharrx=cv2.convertScaleAbs(scharrx)#转回uint8
scharry=cv2.convertScaleAbs(scharry)
#权重
scharrxy=cv2.addWeighted(scharrx,0.5,scharry,0.5,0)
cv2.imshow("liaoxiansheng",img)
cv2.imshow("x",scharrx)
cv2.imshow("y",scharry)
cv2.imshow("xy",scharrxy)
cv2.waitKey()
cv2.destroyAllWindows()
---------------
比较:
梯度就是计算边界值
-------------------
3】laplacian算子 类似二阶sobel导数 两次
卷积核为:
[0, 1, 0]
[1,-4, 1]
[0, 1, 0]
------------------
[p1, p2, p3]
[p4, p5, p6]
[p7, p8, p9]
p5新=(p2+p4+p6+p8)-4*p5图中
---------------------------------
二阶导数:|左-右|+|左-右|+|下-上|-|下-上|
dst=cv2.Laplacian(src,ddepth)
dst:结果图像
src:原始图像
ddepth:图像深度
这里使用cv2.CV_64F使得结果可以是负值,
dst=cv2.convertScaleAbs(src)
将原始图像调整256色位图 可以取绝对值
示例:目标图像=cv2.convertScaleAbs(原始图像)
-------------------------------------------------------------
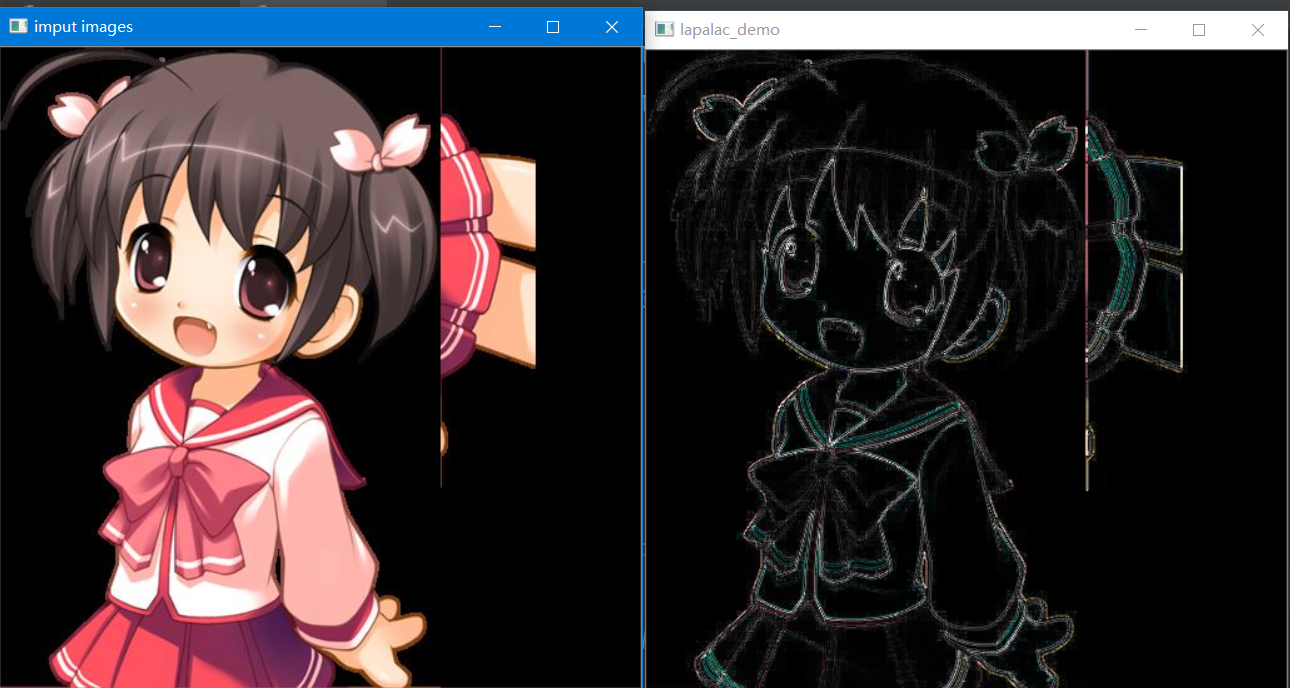
其实你不知道
在你给我糖的时候
有人给了我蛋糕
我没要
我没要蛋糕我不后悔
后悔的是
我以为这颗糖会一直甜