实验一作业
1 #include <iostream> 2 using namespace std; 3 // 定义Point类 4 class Point 5 { 6 public: 7 Point(int x0 = 0, int y0 = 0); 8 Point(const Point &p); 9 ~Point() = default; 10 int get_x() const 11 { 12 return x; 13 } 14 int get_y() const 15 { 16 return y; 17 } 18 void show() const; 19 private: 20 int x, y; 21 }; 22 Point::Point(int x0, int y0) :x{ x0 }, y{ y0 }//初始化列表 23 { 24 cout << "constructor called." << endl; 25 } 26 Point::Point(const Point &p) 27 { 28 x = p.x; 29 y = p.y; 30 cout << "copy constructor called." << endl; 31 } 32 void Point::show() const 33 { 34 cout << "(" << x << ", "<< y << ")" << endl; 35 } 36 37 int main() 38 { 39 Point p1(10, 18); // 构造函数被调用 40 p1.show(); 41 Point p2 = p1; // 复制构造函数被调用 42 p2.show(); 43 Point p3{ p2 }; // 复制构造函数被调用 44 p3.show(); 45 cout << p3.get_x() << endl; 46 }
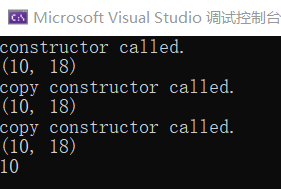
1 #include <iostream> 2 #include <iomanip> 3 using namespace std; 4 // 定义时钟类Clock 5 class Clock 6 { 7 public: 8 Clock(int h = 0, int m = 0, int s = 0); 9 Clock(const Clock &p); 10 ~Clock() = default; 11 void set_time(int h, int m = 0, int s = 0); 12 void show_time() const; 13 private: 14 int hour, minute, second; 15 }; 16 // 类Clock实现 17 Clock::Clock(int h, int m, int s) : hour{ h }, minute{ m }, second{ s } 18 { 19 cout << "constructor called" << endl; 20 } 21 Clock::Clock(const Clock &p) : hour{ p.hour }, minute{ p.minute },second{ p.second } 22 { 23 cout << "copy constructor called" << endl; 24 } 25 void Clock::set_time(int h, int m, int s) 26 { 27 hour = h; 28 minute = m; 29 second = s; 30 } 31 void Clock::show_time() const 32 { 33 cout << setfill('0') << setw(2) << hour << ":"<< setw(2) << minute << ":"<< setw(2) << second << endl; 34 } 35 // 普通函数定义 36 Clock reset() 37 { 38 return Clock(0, 0, 0); // 构造函数被调用 39 } 40 int main() 41 { 42 Clock c1(20, 22, 30); // 构造函数被调用 43 c1.show_time(); 44 c1 = reset(); // 理论上:复制构造函数被调用 45 c1.show_time(); 46 Clock c2(c1); // 复制构造函数被调用 47 c2.set_time(12); 48 c2.show_time(); 49 return 0; 50 }
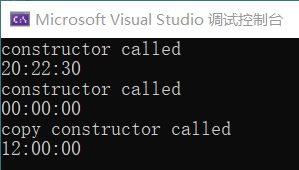
1 #include <iostream> 2 using namespace std; 3 // 定义一个简单抽象类 4 class X 5 { 6 public: 7 X(); // 默认构造函数 8 ~X(); // 析构函数 9 X(int m); // 构造函数 10 X(const X &obj); // 复制构造函数 11 X(X &&obj) noexcept; // 移动构造函数 12 void show() const; // 显示数据 13 private: 14 int data; 15 }; 16 X::X() : data{ 20 } 17 { 18 cout << "default constructor called.\n"; 19 } 20 X::~X() 21 { 22 cout << "destructor called.\n"; 23 } 24 X::X(int m) : data{ m } 25 { 26 cout << "constructor called.\n"; 27 } 28 X::X(const X &obj) : data{ obj.data } 29 { 30 cout << "copy constructor called.\n"; 31 } 32 X::X(X &&obj) noexcept : data{ obj.data } 33 { 34 cout << "move constructor called.\n"; 35 } 36 void X::show() const 37 { 38 cout << data << std::endl; 39 } 40 int main() 41 { 42 X x1; //默认构造函数被编译器自动调用 43 x1.show(); 44 X x2{ 2022 }; 45 x2.show(); // 构造函数被编译器自动调用 46 X x3{ x1 }; // 复制构造函数被编译器自动调用 47 x3.show(); 48 X x4{ std::move(x2) }; // 移动构造函数被编译器调用 49 x4.show(); 50 return 0; 51 }
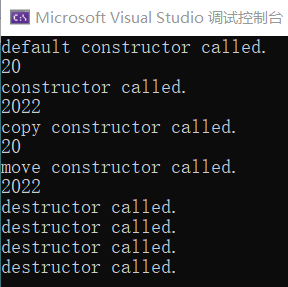
构造函数(按照代码执行顺序):
(1)执行X x1代码时,默认构造函数X()被调用
(2)执行X x2{2022}代码时,构造函数X(int m)被调用,将2022赋值给x2.data
(3)执行X x3{x1}代码时,复制构造函数被调用,将x1.data赋值给x3.data
(4)执行X x4{std::move(x2)}代码时,移动构造函数被调用,将x2.data赋值给x4.data
析构函数:
对象生命周期结束时,析构函数被调用
析构函数被调用的顺序与构造函数相反,x4首先调用析构函数,其次x3,然后x2,最后x1
1 #include <iostream> 2 #include <iomanip> 3 // 矩形类Rectangle的定义和实现 4 class Rectangle 5 { 6 public: 7 Rectangle();//默认构造函数 8 Rectangle(double l, double w);//有参构造函数 9 Rectangle(const Rectangle &p);//复制构造函数 10 double len(); 11 double wide(); 12 double area(); 13 double circumference(); 14 void resize(int times); 15 void resize(int l_times, int w_times); 16 ~Rectangle() 17 { 18 19 }//析构函数 20 private: 21 double length; 22 double width; 23 }; 24 Rectangle::Rectangle(double l, double w) 25 { 26 length = l; 27 width = w; 28 } 29 Rectangle::Rectangle() :length(2.0), width(1.0) 30 { 31 32 } 33 Rectangle::Rectangle(const Rectangle &p) 34 { 35 length = p.length; 36 width = p.width; 37 } 38 double Rectangle::len() 39 { 40 return length; 41 } 42 double Rectangle::wide() 43 { 44 return width; 45 } 46 double Rectangle::area() 47 { 48 return (length * width); 49 } 50 double Rectangle::circumference() 51 { 52 return 2*(length + width); 53 } 54 void Rectangle::resize(int times) 55 { 56 length = length * times; 57 width = width * times; 58 } 59 void Rectangle::resize(int l_times, int w_times) 60 { 61 length = length * l_times; 62 width = width * w_times; 63 } 64 // 普通函数, 用于输出矩形信息 65 void output(Rectangle &rect) 66 { 67 using namespace std; 68 cout<< "矩形信息:"<<endl; 69 cout<< fixed << setprecision(2); // 控制输出格式:以浮点数形式输出、小数部分保留两位 70 // 补足代码:分行输出矩形长、宽、面积、周长 71 cout<< rect.len() << endl; 72 cout << rect.wide() << endl; 73 cout << rect.area() << endl; 74 cout << rect.circumference() << endl; 75 cout << endl; 76 } 77 // 主函数,测试Rectangle类 78 int main() 79 { 80 Rectangle rect1; // 默认构造函数被调用 81 output(rect1); 82 Rectangle rect2(10, 5); // 带有两个参数的构造函数被调用 83 output(rect2); 84 Rectangle rect3(rect1); // 复制构造函数被调用 85 rect3.resize(2); // 矩形rect3的长和宽同时缩放2倍 86 output(rect3); 87 rect3.resize(5, 2); // 矩形rect3的长缩放5倍, 宽缩放2倍 88 output(rect3); 89 return 0; 90 }