C语言:汉诺塔(Hanoi)问题
编程求解汉诺塔问题。
汉诺塔(Hanoi)是必须用递归方法才能解决的经典问题。它来自于印度神话。上帝创造世界时
作了三根金刚石柱子,在第一根柱子上从下往上按大小顺序摞着64片黄金圆盘,如图所示。上帝
命令婆罗门把圆盘从下面开始按大小顺序重新摆放到第二根柱子上,并且规定,每次只能移动一
个圆盘,在小圆盘上不能放大圆盘。有人预言说,这件事完成时宇宙会在一瞬间闪电式毁灭,也
有人相信婆罗门至今仍在一刻不停地搬动着圆盘。
**输入格式要求:"%d" 提示信息:"Input the number of disks:"
**输出格式要求:"Steps of moving %d disks from A to B by means of C:\n"
"Move %d: from %c to %c\n"
程序运行示例如下:
Input the number of disks:3
Steps of moving 3 disks from A to B by means of C:
Move 1: from A to B
Move 2: from A to C
Move 1: from B to C
Move 3: from A to B
Move 1: from C to A
Move 2: from C to B
Move 1: from A to B
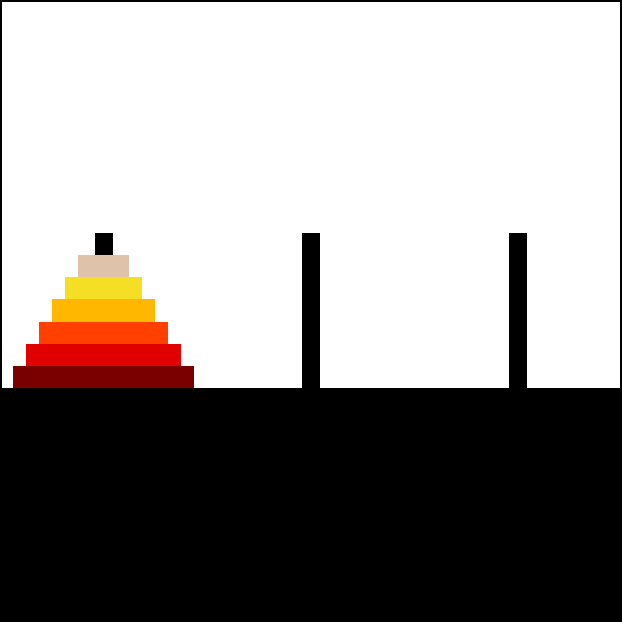
1 #include <stdio.h> 2 void Hanoi(int n, char a, char b, char c); 3 void Move(int n, char a, char b); 4 int main() 5 { 6 int n; 7 printf("Input the number of disks:"); 8 scanf("%d", &n); 9 printf("Steps of moving %d disks from A to B by means of C:\n", n); 10 Hanoi(n, 'A', 'B', 'C'); /*调用递归函数Hanoi()将n个圆盘借助于C由A移动到B*/ 11 return 0; 12 } 13 /* 函数功能:用递归方法将n个圆盘借助于柱子c从源柱子a移动到目标柱子b上 */ 14 void Hanoi(int n, char a, char b, char c) 15 { 16 if (n == 1) 17 { 18 Move(n, a, b); /* 将第n个圆盘由a移到b */ 19 } 20 else 21 { 22 Hanoi(n - 1, a, c, b); /* 递归调用Hanoi(),将第n-1个圆盘借助于b由a移动到c*/ 23 Move(n, a, b); /* 第n个圆盘由a移到b */ 24 Hanoi(n - 1, c, b, a); /*递归调用Hanoi(),将第n-1个圆盘借助于a由c移动到b*/ 25 } 26 } 27 /* 函数功能: 将第n个圆盘从源柱子a移到目标柱子b上 */ 28 void Move(int n, char a, char b) 29 { 30 printf("Move %d: from %c to %c\n", n, a, b); 31 }